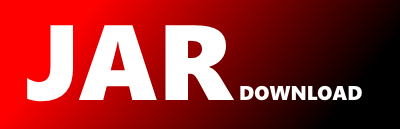
javax.slee.profile.query.GreaterThanOrEquals Maven / Gradle / Ivy
package javax.slee.profile.query;
/**
* The GreaterThanOrEquals
class represents a dynamic query expression
* that checks whether the value of a profile attribute is greater than or equal to a
* specified value.
*
* This query expression can only be used with profile attributes whose class
* implements the {@link Comparable} interface.
*/
public final class GreaterThanOrEquals extends OrderedQueryExpression {
/**
* Create a GreaterThanOrEquals
query expression. A profile will
* match the expression criteria if the value of the attrName
attribute
* is greater than or equal to attrValue
, as determined by
* {@link Comparable#compareTo(Object)}.
* @param attrName the name of the profile attribute to compare.
* @param attrValue the value of the attribute to compare with.
* @throws NullPointerException if either argument is null
.
* @throws IllegalArgumentException if the class of attrValue
does
* not implement the java.lang.Comparable
interface.
*/
public GreaterThanOrEquals(String attrName, Object attrValue) throws NullPointerException {
super(attrName, attrValue, null);
}
/**
* Create a GreaterThanOrEquals
query expression. A profile will
* match the expression criteria if the value of the attrName
attribute
* is greater than or equal to attrValue
, as determined by
* {@link java.text.Collator#compare(String, String)}, where the collator is obtained
* from the specified QueryCollator
.
* @param attrName the name of the profile attribute to compare.
* @param attrValue the value of the attribute to compare with.
* @param collator the collator to use for the comparison. May be null
.
* @throws NullPointerException if either attrName
or
* attrValue
is null
.
*/
public GreaterThanOrEquals(String attrName, String attrValue, QueryCollator collator) throws NullPointerException {
super(attrName, attrValue, collator);
}
// protected
// javadoc copied from parent
protected String getRelation() {
return ">=";
}
}