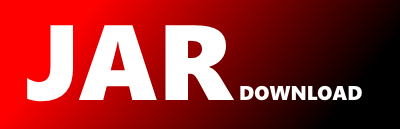
javax.slee.profile.query.RangeMatch Maven / Gradle / Ivy
package javax.slee.profile.query;
/**
* The RangeMatch
class represents a dynamic query expression that checks
* whether the value of a profile attribute lies within a specified range. A profile
* matches the expression if the profile attribute value is greater than or equal to
* the lower bound value and less than or equal to the upper bound value.
*
* This query expression can only be used with profile attributes whose class
* implements the {@link Comparable} interface.
*/
public final class RangeMatch extends QueryExpression {
/**
* Create a RangeMatch
query expression. A profile will match the
* expression criteria if the value of the attrName
attribute is
* greater than or equal to fromValue
and less than or equal to
* toValue
, as determined by {@link Comparable#compareTo(Object)}.
* @param attrName the name of the profile attribute to compare.
* @param fromValue the lower bound of the range.
* @param toValue the upper bound of the range.
* @throws NullPointerException if any argument is null
.
* @throws IllegalArgumentException if the class of fromValue
or
* toValue
does not implement the java.lang.Comparable
* interface.
*/
public RangeMatch(String attrName, Object fromValue, Object toValue) {
if (attrName == null) throw new NullPointerException("attrName is null");
if (fromValue == null) throw new NullPointerException("fromValue is null");
if (toValue == null) throw new NullPointerException("toValue is null");
// implement in terms of other expressions
lowerBound = new GreaterThanOrEquals(attrName, fromValue);
upperBound = new LessThanOrEquals(attrName, toValue);
}
/**
* Create a RangeMatch
query expression. A profile will match the
* expression criteria if the value of the attrName
attribute is
* greater than or equal to fromValue
and less than or equal to
* toValue
, as determined by {@link java.text.Collator#compare(String, String)},
* where the collator is obtained from the specified QueryCollator
.
* @param attrName the name of the profile attribute to compare.
* @param fromValue the lower bound of the range.
* @param toValue the upper bound of the range.
* @param collator the collator to use for the comparison. May be null
.
* @throws NullPointerException if attrName
, fromValue
,
* or toValue
is null
.
* @throws IllegalArgumentException if the class of fromValue
or
* toValue
does not implement the java.lang.Comparable
* interface.
*/
public RangeMatch(String attrName, String fromValue, String toValue, QueryCollator collator) {
if (fromValue == null) throw new NullPointerException("fromValue is null");
if (toValue == null) throw new NullPointerException("toValue is null");
// implement in terms of other expressions
lowerBound = new GreaterThanOrEquals(attrName, fromValue, collator);
upperBound = new LessThanOrEquals(attrName, toValue, collator);
}
/**
* Get the name of the profile attribute used by this query expression.
* @return the name of the profile attribute.
*/
public String getAttributeName() {
return lowerBound.getAttributeName();
}
/**
* Get the lower-bound value the profile attribute will be compared to.
* @return the lower-bound value the profile attribute will be compared to.
*/
public Object getFromValue() {
return lowerBound.getAttributeValue();
}
/**
* Get the upper-bound value the profile attribute will be compared to.
* @return the upper-bound value the profile attribute will be compared to.
*/
public Object getToValue() {
return upperBound.getAttributeValue();
}
/**
* Get the query collator used by this query expression.
* @return the query collator, or null
if one has not been
* specified for this query expression.
*/
public QueryCollator getCollator() { return lowerBound.getCollator(); }
// protected
// javadoc copied from parent
protected void toString(StringBuffer buf) {
buf.append(lowerBound.getAttributeValue()).append(" <= ").
append(getAttributeName()).append(" <= ").append(upperBound.getAttributeValue());
}
private final GreaterThanOrEquals lowerBound;
private final LessThanOrEquals upperBound;
}