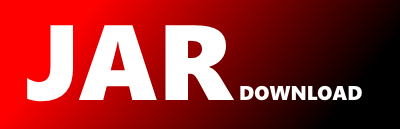
javax.slee.resource.ResourceAdaptorContext Maven / Gradle / Ivy
package javax.slee.resource;
import java.util.Timer;
import javax.slee.SLEEException;
import javax.slee.ServiceID;
import javax.slee.facilities.AlarmFacility;
import javax.slee.facilities.EventLookupFacility;
import javax.slee.facilities.ServiceLookupFacility;
import javax.slee.facilities.Tracer;
import javax.slee.profile.ProfileTable;
import javax.slee.profile.UnrecognizedProfileTableNameException;
import javax.slee.transaction.SleeTransactionManager;
import javax.slee.usage.NoUsageParametersInterfaceDefinedException;
import javax.slee.usage.UnrecognizedUsageParameterSetNameException;
/**
* The ResourceAdaptorContext
interface provides a resource adaptor object
* with access to SLEE Facilities and other capabilities and objects implemented by the
* SLEE on behalf of a Resource Adaptor.
*
* A ResourceAdaptorContext
object is given to a resource adaptor object after it
* is created via the {@link ResourceAdaptor#setResourceAdaptorContext setResourceAdaptorContext}
* method. The ResourceAdaptorContext
object remains associated with the resource
* adaptor object for the lifetime of that resource adaptor object.
* @since SLEE 1.1
*/
public interface ResourceAdaptorContext {
/**
* Get the component identifier for this resource adaptor.
*
* This method is a non-transactional method.
* @return the component identifier for the resource adaptor.
*/
public ResourceAdaptorID getResourceAdaptor();
/**
* Get the component identifiers of the resource adaptor types implemented
* by this resource adaptor.
*
* This method is a non-transactional method.
* @return the component identifiers of the resource adaptor types implemented
* by this resource adaptor. This will always be a non-null value.
*/
public ResourceAdaptorTypeID[] getResourceAdaptorTypes();
/**
* Get the name of the resource adaptor entity the resource adaptor object was
* created for.
*
* This method is a non-transactional method.
* @return the name of the resource adaptor entity.
*/
public String getEntityName();
/**
* Get the SLEE Endpoint object used to notify the SLEE about activities that are
* starting and ending, and to fire events on those activities to the SLEE.
*
* This method is a non-transactional method.
*/
public SleeEndpoint getSleeEndpoint();
/**
* Get the alarm facility used to raise, update, and clear alarms in the SLEE.
* The notification source used by this alarm facility is a
* {@link javax.slee.management.ResourceAdaptorEntityNotification} that contains the
* name of the resource adaptor entity as identified by {@link #getEntityName()}.
*
* Alarm notifications generated by this alarm facility are of the type
* {@link javax.slee.management.ResourceAdaptorEntityNotification#ALARM_NOTIFICATION_TYPE}.
*
* This method is a non-transactional method.
* @return the alarm facility.
*/
public AlarmFacility getAlarmFacility();
/**
* Get a tracer for the specified tracer name. The notification source used by the tracer
* is a {@link javax.slee.management.ResourceAdaptorEntityNotification} that contains the
* name of the resource adaptor entity as identified by {@link #getEntityName()}.
*
* Refer {@link javax.slee.facilities.Tracer} for a complete discussion on tracers and
* tracer names.
*
* Trace notifications generated by a tracer obtained using this method are of the type
* {@link javax.slee.management.ResourceAdaptorEntityNotification#TRACE_NOTIFICATION_TYPE}.
*
* This method is a non-transactional method.
* @param tracerName the name of the tracer.
* @return a tracer for the specified tracer name. Trace messages generated by this tracer
* will contain a notification source that is a ResourceAdaptorEntityNotification
* object containing a resource adaptor entity name equal to that obtained from the
* {@link #getEntityName()} method on this ResourceAdaptorContext
.
* @throws NullPointerException if tracerName
is null
.
* @throws IllegalArgumentException if tracerName
is an invalid name. Name
* components within a tracer name must have at least one character. For example,
* "com.mycompany"
is a valid tracer name, whereas "com..mycompany"
* is not.
* @throws SLEEException if the tracer could not be obtained due to a system-level failure.
*/
public Tracer getTracer(String tracerName)
throws NullPointerException, IllegalArgumentException, SLEEException;
/**
* Get the event lookup facility used to obtain the {@link FireableEventType} objects
* required for the resource adaptor to fire events to the SLEE.
*
* This method is a non-transactional method.
* @return the event lookup facility.
*/
public EventLookupFacility getEventLookupFacility();
/**
* Get the service lookup facility used obtain information about the event types
* that may be received by SBBs in a service.
*
* This method is a non-transactional method.
* @return the service lookup facility.
*/
public ServiceLookupFacility getServiceLookupFacility();
/**
* Get the SLEE transaction manager that allows a resource adaptor to demarcate
* transaction boundaries.
*
* This method is a non-transactional method.
* @return the SLEE transaction manager.
*/
public SleeTransactionManager getSleeTransactionManager();
/**
* Get the shared Java timer that can be used to schedule timer tasks.
*
* A Resource Adaptor is not permitted to use the {@link Timer#cancel} method on
* the Timer
object returned from this method. Attempting to do so
* will cause a java.lang.UnsupportedOperationException
to be thrown.
*
* This method is a non-transactional method.
* @return the shared Java timer.
*/
public Timer getTimer();
/**
* Get a ProfileTable
object for a profile table. The object returned
* by this method may be safely typecast to the Profile Table interface defined by
* the profile specification of the profile table if the resource adaptor has the
* appropriate classes in its classloader to do so, for example by declaring a
* profile-spec-ref
in its deployment descriptor for the profile
* specification of the Profile Table.
*
* This method is a non-transactional method.
* @param profileTableName the name of the profile table.
* @return a {@link ProfileTable} object for the profile table.
* @throws NullPointerException if profileTableName
is null
.
* @throws UnrecognizedProfileTableNameException if a profile table with the
* specified name does not exist.
* @throws SLEEException if the ProfileTable
object could not be
* obtained due to a system-level-failure.
*/
public ProfileTable getProfileTable(String profileTableName)
throws NullPointerException, UnrecognizedProfileTableNameException, SLEEException;
/**
* Get the unnamed usage parameter set for the resource adaptor entity. The object
* returned by this method may be safely typecast to the Usage Parameters Interface
* defined by the Resource Adaptor.
*
* This method can only be invoked by resource adaptor entities of a Resource Adaptor
* that defines a Usage Parameters Interface. If a resource adaptor entity of a
* Resource Adaptor that doesn't define a Usage Parameters Interface attempts to invoke
* this method, a NoUsageParametersInterfaceDefinedException
is thrown.
*
* This method is a non-transactional method.
* @return an object that implements the Usage Parameters Interface of the Resource
* Adaptor that represents the unnamed usage parameter set for the resource
* adaptor entity.
* @throws NoUsageParametersInterfaceDefinedException if the Resource Adaptor did not
* define a Usage Parameters Interface.
* @throws SLEEException if the usage parameter set could not be obtained due to a
* system-level failure.
*/
public Object getDefaultUsageParameterSet()
throws NoUsageParametersInterfaceDefinedException, SLEEException;
/**
* Get a named usage parameter set for the resource adaptor entity. The object
* returned by this method may be safely typecast to the Usage Parameters Interface
* defined by the Resource Adaptor.
*
* This method can only be invoked by resource adaptor entities of a Resource Adaptor
* that defines a Usage Parameters Interface. If a resource adaptor entity of a
* Resource Adaptor that doesn't define a Usage Parameters Interface attempts to invoke
* this method, a NoUsageParametersInterfaceDefinedException
is thrown.
*
* This method is a non-transactional method.
* @param paramSetName the name of the usage parameter set to return.
* @return an object that implements the Usage Parameters Interface of the Resource
* Adaptor that represents the specified named usage parameter set for the
* resource adaptor entity.
* @throws NullPointerException if paramSetName
is null
.
* @throws NoUsageParametersInterfaceDefinedException if the Resource Adaptor did not
* define a Usage Parameters Interface.
* @throws UnrecognizedUsageParameterSetNameException if the name does not identify a
* usage parameter set that has been created for the resource adaptor entity.
* @throws SLEEException if the usage parameter set could not be obtained due to a
* system-level failure.
*/
public Object getUsageParameterSet(String paramSetName)
throws NullPointerException, NoUsageParametersInterfaceDefinedException,
UnrecognizedUsageParameterSetNameException, SLEEException;
/**
* Get the service component identifier of the service currently being invoked by
* the SLEE in the current thread. The purpose of this method is to allow resource
* adaptors to determine which service an SBB belongs to when that SBB invokes a
* downcall on the resource adaptor, for example by invoking a method on the resource
* adaptor interface or on an activity object.
* @return the service component identifier of the service currently being invoked by
* the SLEE in the current thread, or null
if there is no such
* service.
*/
public ServiceID getInvokingService();
}