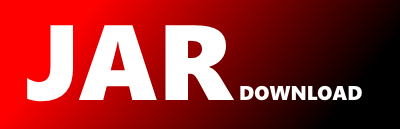
javax.ws.rs.core.UriInfo Maven / Gradle / Ivy
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* http://www.opensource.org/licenses/cddl1.php
* See the License for the specific language governing
* permissions and limitations under the License.
*/
/*
* UriInfo.java
*
* Created on April 13, 2007, 2:55 PM
*
*/
package javax.ws.rs.core;
import java.net.URI;
import java.util.List;
/**
* An injectable interface that provides access to request URI information
* @see HttpContext
* @author mh124079
*/
public interface UriInfo {
/**
* Get the path of the current request relative to the base URI as
* a string. All sequences of escaped octets are decoded, equivalent to
* getPath(true)
.
* @return the relative URI path.
*/
public String getPath();
/**
* Get the path of the current request relative to the base URI as
* a string.
*
* @param decode controls whether sequences of escaped octets are decoded
* (true) or not (false).
* @return the relative URI path.
*/
public String getPath(boolean decode);
/**
* Get the path of the current request relative to the base URI as a
* list of {@link PathSegment}. This method is useful when the
* path needs to be parsed, particularly when matrix parameters may be
* present in the path. All sequences of escaped octets are decoded,
* equivalent to getPathSegments(true)
.
* @return the list of {@link PathSegment}.
* @see PathSegment
*/
public List getPathSegments();
/**
* Get the path of the current request relative to the base URI as a
* list of {@link PathSegment}. This method is useful when the
* path needs to be parsed, particularly when matrix parameters may be
* present in the path.
* @param decode controls whether sequences of escaped octets are decoded
* (true) or not (false).
* @return the list of {@link PathSegment}.
* @see PathSegment
*/
public List getPathSegments(boolean decode);
/**
* Get the absolute URI of the request. This is a shortcut for
* uriInfo.getBase().resolve(uriInfo.getPath()).
* @return the absolute URI of the request
*/
public URI getAbsolute();
/**
* Get the absolute URI of the current request in the form of a UriBuilder.
* @return a UriBuilder initialized with the current request URI.
*/
public UriBuilder getBuilder();
/**
* Get the base URI of the application. URIs of resource beans
* are all relative to this base URI.
* @return the base URI of the application
*/
public URI getBase();
/**
* Get the base URI of the application in the form of a UriBuilder.
* @return a UriBuilder initialized with the base URI of the application.
*/
public UriBuilder getBaseBuilder();
/**
* Get the values of any embedded URI template parameters.
* All sequences of escaped octets are decoded,
* equivalent to getURIParameters(true)
.
* @return a map of parameter names and values.
* @see javax.ws.rs.UriTemplate
*/
public MultivaluedMap getTemplateParameters();
/**
* Get the values of any embedded URI template parameters.
*
* @param decode controls whether sequences of escaped octets are decoded
* (true) or not (false).
* @return a map of parameter names and values.
* @see javax.ws.rs.UriTemplate
*/
public MultivaluedMap getTemplateParameters(boolean decode);
/**
* Get the URI query parameters of the current request.
* All sequences of escaped octets are decoded,
* equivalent to getQueryParameters(true)
.
* @return a map of query parameter names and values.
*/
public MultivaluedMap getQueryParameters();
/**
* Get the URI query parameters of the current request.
* @param decode controls whether sequences of escaped octets are decoded
* (true) or not (false).
* @return a map of query parameter names and values.
*/
public MultivaluedMap getQueryParameters(boolean decode);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy