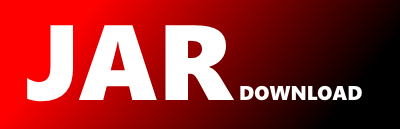
com.sun.ws.rest.impl.provider.entity.JSONRootElementProvider Maven / Gradle / Ivy
Go to download
Open source JAX-RS (JSR 311) Reference Implementation for building
RESTful Web services
The newest version!
/*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS HEADER.
*
* Copyright 2007 Sun Microsystems, Inc. All rights reserved.
*
* The contents of this file are subject to the terms of the Common Development
* and Distribution License("CDDL") (the "License"). You may not use this file
* except in compliance with the License.
*
* You can obtain a copy of the License at:
* https://jersey.dev.java.net/license.txt
* See the License for the specific language governing permissions and
* limitations under the License.
*
* When distributing the Covered Code, include this CDDL Header Notice in each
* file and include the License file at:
* https://jersey.dev.java.net/license.txt
* If applicable, add the following below this CDDL Header, with the fields
* enclosed by brackets [] replaced by your own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*/
package com.sun.ws.rest.impl.provider.entity;
import com.sun.ws.rest.impl.ImplMessages;
import com.sun.ws.rest.impl.json.JSONJAXBContext;
import com.sun.ws.rest.impl.json.JSONMarshaller;
import com.sun.ws.rest.impl.json.JSONUnmarshaller;
import com.sun.ws.rest.impl.json.reader.JsonXmlStreamReader;
import com.sun.ws.rest.impl.json.writer.JsonXmlStreamWriter;
import com.sun.ws.rest.impl.util.ThrowHelper;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
import javax.ws.rs.ConsumeMime;
import javax.ws.rs.ProduceMime;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.MultivaluedMap;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
/**
*
* @author [email protected]
*/
@ProduceMime("application/json")
@ConsumeMime("application/json")
public final class JSONRootElementProvider extends AbstractRootElementProvider {
public JSONRootElementProvider() {
Class> c = JAXBContext.class;
}
public Object readFrom(
Class
© 2015 - 2024 Weber Informatics LLC | Privacy Policy