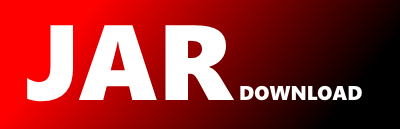
jp.co.yahoo.adsdisplayapi.v13.model.AdGroupTargetServiceGeoTarget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v13
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v13.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import jp.co.yahoo.adsdisplayapi.v13.model.AdGroupTargetServiceAreaSearchType;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\"> AdGroupTargetServiceGeoTargetオブジェクトは、地域ターゲティングの設定情報を保持します。<br> ADD、SETおよびREPLACE時、このフィールドは省略可能となります。<br> ※targetTypeがGEO_TARGETの場合は必須です。 </div> <div lang=\"en\"> AdGroupTargetServiceGeoTarget object is a container for storing geological targeting settings.<br> This field is optional in ADD, SET and REPLACE operation.<br> ∗If targetType is GEO_TARGET, this field is required. </div>
*/
@ApiModel(description = " AdGroupTargetServiceGeoTargetオブジェクトは、地域ターゲティングの設定情報を保持します。
ADD、SETおよびREPLACE時、このフィールドは省略可能となります。
※targetTypeがGEO_TARGETの場合は必須です。 AdGroupTargetServiceGeoTarget object is a container for storing geological targeting settings.
This field is optional in ADD, SET and REPLACE operation.
∗If targetType is GEO_TARGET, this field is required. ")
@JsonPropertyOrder({
AdGroupTargetServiceGeoTarget.JSON_PROPERTY_AREA_SEARCH_TYPE,
AdGroupTargetServiceGeoTarget.JSON_PROPERTY_LATITUDE_IN_MICRO_DEGREES,
AdGroupTargetServiceGeoTarget.JSON_PROPERTY_LONGITUDE_IN_MICRO_DEGREES,
AdGroupTargetServiceGeoTarget.JSON_PROPERTY_RADIUS,
AdGroupTargetServiceGeoTarget.JSON_PROPERTY_DESCRIPTION,
AdGroupTargetServiceGeoTarget.JSON_PROPERTY_GEO_NAME_EN,
AdGroupTargetServiceGeoTarget.JSON_PROPERTY_GEO_NAME_JA
})
@JsonTypeName("AdGroupTargetServiceGeoTarget")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class AdGroupTargetServiceGeoTarget {
public static final String JSON_PROPERTY_AREA_SEARCH_TYPE = "areaSearchType";
private AdGroupTargetServiceAreaSearchType areaSearchType;
public static final String JSON_PROPERTY_LATITUDE_IN_MICRO_DEGREES = "latitudeInMicroDegrees";
private Integer latitudeInMicroDegrees;
public static final String JSON_PROPERTY_LONGITUDE_IN_MICRO_DEGREES = "longitudeInMicroDegrees";
private Integer longitudeInMicroDegrees;
public static final String JSON_PROPERTY_RADIUS = "radius";
private Integer radius;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_GEO_NAME_EN = "geoNameEn";
private String geoNameEn;
public static final String JSON_PROPERTY_GEO_NAME_JA = "geoNameJa";
private String geoNameJa;
public AdGroupTargetServiceGeoTarget() {
}
public AdGroupTargetServiceGeoTarget areaSearchType(AdGroupTargetServiceAreaSearchType areaSearchType) {
this.areaSearchType = areaSearchType;
return this;
}
/**
* Get areaSearchType
* @return areaSearchType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AREA_SEARCH_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AdGroupTargetServiceAreaSearchType getAreaSearchType() {
return areaSearchType;
}
@JsonProperty(JSON_PROPERTY_AREA_SEARCH_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAreaSearchType(AdGroupTargetServiceAreaSearchType areaSearchType) {
this.areaSearchType = areaSearchType;
}
public AdGroupTargetServiceGeoTarget latitudeInMicroDegrees(Integer latitudeInMicroDegrees) {
this.latitudeInMicroDegrees = latitudeInMicroDegrees;
return this;
}
/**
* <div lang=\"ja\">マイクロ表記の緯度です。<br> 1度と指定したい場合は、1000000を指定します。<br> ADおよびREPLACE時、areaSearchTypeがRADIUSの場合は必須です。areaSearchTypeがGEOの場合は指定できません。<br> 日本国内のみ指定できます。</div> <div lang=\"en\">Micro degrees for the latitude.<br> To specify 1 degree, specify 1000000.<br> In ADD and REPLACE operation, Required if areaSearchType is RADIUS, and cannot be specified if areaSearchType is GEO.<br> Can be specified only in Japan.</div>
* @return latitudeInMicroDegrees
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "マイクロ表記の緯度です。
1度と指定したい場合は、1000000を指定します。
ADおよびREPLACE時、areaSearchTypeがRADIUSの場合は必須です。areaSearchTypeがGEOの場合は指定できません。
日本国内のみ指定できます。 Micro degrees for the latitude.
To specify 1 degree, specify 1000000.
In ADD and REPLACE operation, Required if areaSearchType is RADIUS, and cannot be specified if areaSearchType is GEO.
Can be specified only in Japan. ")
@JsonProperty(JSON_PROPERTY_LATITUDE_IN_MICRO_DEGREES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getLatitudeInMicroDegrees() {
return latitudeInMicroDegrees;
}
@JsonProperty(JSON_PROPERTY_LATITUDE_IN_MICRO_DEGREES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLatitudeInMicroDegrees(Integer latitudeInMicroDegrees) {
this.latitudeInMicroDegrees = latitudeInMicroDegrees;
}
public AdGroupTargetServiceGeoTarget longitudeInMicroDegrees(Integer longitudeInMicroDegrees) {
this.longitudeInMicroDegrees = longitudeInMicroDegrees;
return this;
}
/**
* <div lang=\"ja\">マイクロ表記の経度です。<br> 1度と指定したい場合は、1000000を指定します。<br> ADおよびREPLACE時、areaSearchTypeがRADIUSの場合は必須です。areaSearchTypeがGEOの場合は指定できません。<br> 日本国内のみ指定できます。</div> <div lang=\"en\">Micro degrees for the longitude.<br> To specify 1 degree, specify 1000000.<br> In ADD and REPLACE operation, Required if areaSearchType is RADIUS, and cannot be specified if areaSearchType is GEO.<br> Can be specified only in Japan.</div>
* @return longitudeInMicroDegrees
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "マイクロ表記の経度です。
1度と指定したい場合は、1000000を指定します。
ADおよびREPLACE時、areaSearchTypeがRADIUSの場合は必須です。areaSearchTypeがGEOの場合は指定できません。
日本国内のみ指定できます。 Micro degrees for the longitude.
To specify 1 degree, specify 1000000.
In ADD and REPLACE operation, Required if areaSearchType is RADIUS, and cannot be specified if areaSearchType is GEO.
Can be specified only in Japan. ")
@JsonProperty(JSON_PROPERTY_LONGITUDE_IN_MICRO_DEGREES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getLongitudeInMicroDegrees() {
return longitudeInMicroDegrees;
}
@JsonProperty(JSON_PROPERTY_LONGITUDE_IN_MICRO_DEGREES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLongitudeInMicroDegrees(Integer longitudeInMicroDegrees) {
this.longitudeInMicroDegrees = longitudeInMicroDegrees;
}
public AdGroupTargetServiceGeoTarget radius(Integer radius) {
this.radius = radius;
return this;
}
/**
* <div lang=\"ja\">半径(km)です。<br> ADDおよびREPLACE時、areaSearchTypeがRADIUSの場合は必須です。areaSearchTypeがGEOの場合は指定できません。<br> 最大80kmまで指定できます。</div> <div lang=\"en\">Radius(km).<br> In ADD and REPLACE operation, Required if areaSearchType is RADIUS, and cannot be specified if areaSearchType is GEO.<br> Can be specified up to 80km.</div>
* @return radius
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "半径(km)です。
ADDおよびREPLACE時、areaSearchTypeがRADIUSの場合は必須です。areaSearchTypeがGEOの場合は指定できません。
最大80kmまで指定できます。 Radius(km).
In ADD and REPLACE operation, Required if areaSearchType is RADIUS, and cannot be specified if areaSearchType is GEO.
Can be specified up to 80km. ")
@JsonProperty(JSON_PROPERTY_RADIUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getRadius() {
return radius;
}
@JsonProperty(JSON_PROPERTY_RADIUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRadius(Integer radius) {
this.radius = radius;
}
public AdGroupTargetServiceGeoTarget description(String description) {
this.description = description;
return this;
}
/**
* <div lang=\"ja\">指定した地点の説明です。<br> ADDおよびREPLACE時、areaSearchTypeがRADIUSの場合は任意で指定できます。areaSearchTypeがGEOの場合は指定できません。</div> <div lang=\"en\">A description of the specified location.<br> In ADD and REPLACE operation, Optional if areaSearchType is RADIUS, and cannot be specified if areaSearchType is GEO.</div>
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "指定した地点の説明です。
ADDおよびREPLACE時、areaSearchTypeがRADIUSの場合は任意で指定できます。areaSearchTypeがGEOの場合は指定できません。 A description of the specified location.
In ADD and REPLACE operation, Optional if areaSearchType is RADIUS, and cannot be specified if areaSearchType is GEO. ")
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public AdGroupTargetServiceGeoTarget geoNameEn(String geoNameEn) {
this.geoNameEn = geoNameEn;
return this;
}
/**
* <div lang=\"ja\"> 地域名(英語)です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。<br> areaSearchTypeがRADIUSの場合はレスポンスの際に返却されません。 </div> <div lang=\"en\"> Region name (English).<br> Although this field will be returned in the response, it will be ignored on input.<br> If areaSearchType is RADIUS, it will not be returned. </div>
* @return geoNameEn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 地域名(英語)です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。
areaSearchTypeがRADIUSの場合はレスポンスの際に返却されません。 Region name (English).
Although this field will be returned in the response, it will be ignored on input.
If areaSearchType is RADIUS, it will not be returned. ")
@JsonProperty(JSON_PROPERTY_GEO_NAME_EN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getGeoNameEn() {
return geoNameEn;
}
@JsonProperty(JSON_PROPERTY_GEO_NAME_EN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGeoNameEn(String geoNameEn) {
this.geoNameEn = geoNameEn;
}
public AdGroupTargetServiceGeoTarget geoNameJa(String geoNameJa) {
this.geoNameJa = geoNameJa;
return this;
}
/**
* <div lang=\"ja\"> 地域名(日本語)です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。<br> areaSearchTypeがRADIUSの場合はレスポンスの際に返却されません。 </div> <div lang=\"en\">Region name (Japanese).<br> Although this field will be returned in the response, it will be ignored on input.<br> If areaSearchType is RADIUS, it will not be returned. </div>
* @return geoNameJa
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 地域名(日本語)です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。
areaSearchTypeがRADIUSの場合はレスポンスの際に返却されません。 Region name (Japanese).
Although this field will be returned in the response, it will be ignored on input.
If areaSearchType is RADIUS, it will not be returned. ")
@JsonProperty(JSON_PROPERTY_GEO_NAME_JA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getGeoNameJa() {
return geoNameJa;
}
@JsonProperty(JSON_PROPERTY_GEO_NAME_JA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGeoNameJa(String geoNameJa) {
this.geoNameJa = geoNameJa;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AdGroupTargetServiceGeoTarget adGroupTargetServiceGeoTarget = (AdGroupTargetServiceGeoTarget) o;
return Objects.equals(this.areaSearchType, adGroupTargetServiceGeoTarget.areaSearchType) &&
Objects.equals(this.latitudeInMicroDegrees, adGroupTargetServiceGeoTarget.latitudeInMicroDegrees) &&
Objects.equals(this.longitudeInMicroDegrees, adGroupTargetServiceGeoTarget.longitudeInMicroDegrees) &&
Objects.equals(this.radius, adGroupTargetServiceGeoTarget.radius) &&
Objects.equals(this.description, adGroupTargetServiceGeoTarget.description) &&
Objects.equals(this.geoNameEn, adGroupTargetServiceGeoTarget.geoNameEn) &&
Objects.equals(this.geoNameJa, adGroupTargetServiceGeoTarget.geoNameJa);
}
@Override
public int hashCode() {
return Objects.hash(areaSearchType, latitudeInMicroDegrees, longitudeInMicroDegrees, radius, description, geoNameEn, geoNameJa);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AdGroupTargetServiceGeoTarget {\n");
sb.append(" areaSearchType: ").append(toIndentedString(areaSearchType)).append("\n");
sb.append(" latitudeInMicroDegrees: ").append(toIndentedString(latitudeInMicroDegrees)).append("\n");
sb.append(" longitudeInMicroDegrees: ").append(toIndentedString(longitudeInMicroDegrees)).append("\n");
sb.append(" radius: ").append(toIndentedString(radius)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" geoNameEn: ").append(toIndentedString(geoNameEn)).append("\n");
sb.append(" geoNameJa: ").append(toIndentedString(geoNameJa)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy