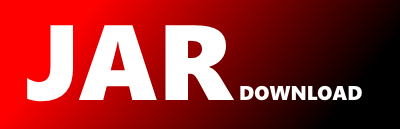
jp.co.yahoo.adsdisplayapi.v13.model.DictionaryServiceSharedAudienceListInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v13
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v13.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import jp.co.yahoo.adsdisplayapi.v13.model.DictionaryServiceSharedAudienceListType;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\">DictionaryServiceSharedAudienceListInfoオブジェクトは、共通オーディエンスリストの情報を表します。</div> <div lang=\"en\">DictionaryServiceSharedAudienceListInfo object describes information of shared audience list.</div>
*/
@ApiModel(description = "DictionaryServiceSharedAudienceListInfoオブジェクトは、共通オーディエンスリストの情報を表します。 DictionaryServiceSharedAudienceListInfo object describes information of shared audience list. ")
@JsonPropertyOrder({
DictionaryServiceSharedAudienceListInfo.JSON_PROPERTY_AUDIENCE_LIST_ID,
DictionaryServiceSharedAudienceListInfo.JSON_PROPERTY_AUDIENCE_LIST_NAME_JA,
DictionaryServiceSharedAudienceListInfo.JSON_PROPERTY_AUDIENCE_LIST_NAME_EN,
DictionaryServiceSharedAudienceListInfo.JSON_PROPERTY_AUDIENCE_LIST_TYPE,
DictionaryServiceSharedAudienceListInfo.JSON_PROPERTY_REACH
})
@JsonTypeName("DictionaryServiceSharedAudienceListInfo")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class DictionaryServiceSharedAudienceListInfo {
public static final String JSON_PROPERTY_AUDIENCE_LIST_ID = "audienceListId";
private Long audienceListId;
public static final String JSON_PROPERTY_AUDIENCE_LIST_NAME_JA = "audienceListNameJa";
private String audienceListNameJa;
public static final String JSON_PROPERTY_AUDIENCE_LIST_NAME_EN = "audienceListNameEn";
private String audienceListNameEn;
public static final String JSON_PROPERTY_AUDIENCE_LIST_TYPE = "audienceListType";
private DictionaryServiceSharedAudienceListType audienceListType;
public static final String JSON_PROPERTY_REACH = "reach";
private Long reach;
public DictionaryServiceSharedAudienceListInfo() {
}
public DictionaryServiceSharedAudienceListInfo audienceListId(Long audienceListId) {
this.audienceListId = audienceListId;
return this;
}
/**
* <div lang=\"ja\"> オーディエンスリストIDです。<br> </div> <div lang=\"en\"> Audience list ID. </div>
* @return audienceListId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " オーディエンスリストIDです。
Audience list ID. ")
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getAudienceListId() {
return audienceListId;
}
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAudienceListId(Long audienceListId) {
this.audienceListId = audienceListId;
}
public DictionaryServiceSharedAudienceListInfo audienceListNameJa(String audienceListNameJa) {
this.audienceListNameJa = audienceListNameJa;
return this;
}
/**
* <div lang=\"ja\"> オーディエンスリスト名(日本語)です。 </div> <div lang=\"en\"> Audience list name(Japanese). </div>
* @return audienceListNameJa
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " オーディエンスリスト名(日本語)です。 Audience list name(Japanese). ")
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_NAME_JA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAudienceListNameJa() {
return audienceListNameJa;
}
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_NAME_JA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAudienceListNameJa(String audienceListNameJa) {
this.audienceListNameJa = audienceListNameJa;
}
public DictionaryServiceSharedAudienceListInfo audienceListNameEn(String audienceListNameEn) {
this.audienceListNameEn = audienceListNameEn;
return this;
}
/**
* <div lang=\"ja\"> オーディエンスリスト名(英語)です。 </div> <div lang=\"en\"> Audience list name(English). </div>
* @return audienceListNameEn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " オーディエンスリスト名(英語)です。 Audience list name(English). ")
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_NAME_EN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAudienceListNameEn() {
return audienceListNameEn;
}
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_NAME_EN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAudienceListNameEn(String audienceListNameEn) {
this.audienceListNameEn = audienceListNameEn;
}
public DictionaryServiceSharedAudienceListInfo audienceListType(DictionaryServiceSharedAudienceListType audienceListType) {
this.audienceListType = audienceListType;
return this;
}
/**
* Get audienceListType
* @return audienceListType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DictionaryServiceSharedAudienceListType getAudienceListType() {
return audienceListType;
}
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAudienceListType(DictionaryServiceSharedAudienceListType audienceListType) {
this.audienceListType = audienceListType;
}
public DictionaryServiceSharedAudienceListInfo reach(Long reach) {
this.reach = reach;
return this;
}
/**
* <div lang=\"ja\"> リーチ数です。 </div> <div lang=\"en\"> Number of reaches. </div>
* @return reach
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " リーチ数です。 Number of reaches. ")
@JsonProperty(JSON_PROPERTY_REACH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getReach() {
return reach;
}
@JsonProperty(JSON_PROPERTY_REACH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReach(Long reach) {
this.reach = reach;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DictionaryServiceSharedAudienceListInfo dictionaryServiceSharedAudienceListInfo = (DictionaryServiceSharedAudienceListInfo) o;
return Objects.equals(this.audienceListId, dictionaryServiceSharedAudienceListInfo.audienceListId) &&
Objects.equals(this.audienceListNameJa, dictionaryServiceSharedAudienceListInfo.audienceListNameJa) &&
Objects.equals(this.audienceListNameEn, dictionaryServiceSharedAudienceListInfo.audienceListNameEn) &&
Objects.equals(this.audienceListType, dictionaryServiceSharedAudienceListInfo.audienceListType) &&
Objects.equals(this.reach, dictionaryServiceSharedAudienceListInfo.reach);
}
@Override
public int hashCode() {
return Objects.hash(audienceListId, audienceListNameJa, audienceListNameEn, audienceListType, reach);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DictionaryServiceSharedAudienceListInfo {\n");
sb.append(" audienceListId: ").append(toIndentedString(audienceListId)).append("\n");
sb.append(" audienceListNameJa: ").append(toIndentedString(audienceListNameJa)).append("\n");
sb.append(" audienceListNameEn: ").append(toIndentedString(audienceListNameEn)).append("\n");
sb.append(" audienceListType: ").append(toIndentedString(audienceListType)).append("\n");
sb.append(" reach: ").append(toIndentedString(reach)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy