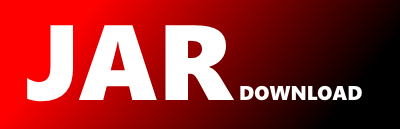
jp.co.yahoo.adsdisplayapi.v13.model.PlacementUrlIdeaServiceSelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v13
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v13.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\">PlacementUrlIdeaServiceSelectorオブジェクトは、getメソッドの検索条件(実行パラメータ)を保持するオブジェクトです。</div> <div lang=\"en\">The objects to keep get method search conditions (execution parameter).</div>
*/
@ApiModel(description = "PlacementUrlIdeaServiceSelectorオブジェクトは、getメソッドの検索条件(実行パラメータ)を保持するオブジェクトです。 The objects to keep get method search conditions (execution parameter). ")
@JsonPropertyOrder({
PlacementUrlIdeaServiceSelector.JSON_PROPERTY_KEYWORD,
PlacementUrlIdeaServiceSelector.JSON_PROPERTY_SITE_CATEGORIES,
PlacementUrlIdeaServiceSelector.JSON_PROPERTY_NUMBER_RESULTS,
PlacementUrlIdeaServiceSelector.JSON_PROPERTY_START_INDEX
})
@JsonTypeName("PlacementUrlIdeaServiceSelector")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PlacementUrlIdeaServiceSelector {
public static final String JSON_PROPERTY_KEYWORD = "keyword";
private String keyword;
public static final String JSON_PROPERTY_SITE_CATEGORIES = "siteCategories";
private List siteCategories = null;
public static final String JSON_PROPERTY_NUMBER_RESULTS = "numberResults";
private Integer numberResults = 500;
public static final String JSON_PROPERTY_START_INDEX = "startIndex";
private Integer startIndex = 1;
public PlacementUrlIdeaServiceSelector() {
}
public PlacementUrlIdeaServiceSelector keyword(String keyword) {
this.keyword = keyword;
return this;
}
/**
* <div lang=\"ja\"> 検索キーワードの配列です。<br> ・URLを検索するためのキーワードです。<br> ・部分一致です。<br> ・スペース区切りでAND検索です。<br> ・最大文字数250です。<br> ・スペース区切りでの単語数は最大10個です。 </div> <div lang=\"en\"> Array of Search keyword.<br> ・Keyword to search the URL<br> ・Broad match<br> ・Search all keywords (AND), separated by spaces<br> ・Maximum of 250 characters<br> ・Maximum of 10 spaces to separate the words </div>
* @return keyword
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 検索キーワードの配列です。
・URLを検索するためのキーワードです。
・部分一致です。
・スペース区切りでAND検索です。
・最大文字数250です。
・スペース区切りでの単語数は最大10個です。 Array of Search keyword.
・Keyword to search the URL
・Broad match
・Search all keywords (AND), separated by spaces
・Maximum of 250 characters
・Maximum of 10 spaces to separate the words ")
@JsonProperty(JSON_PROPERTY_KEYWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getKeyword() {
return keyword;
}
@JsonProperty(JSON_PROPERTY_KEYWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setKeyword(String keyword) {
this.keyword = keyword;
}
public PlacementUrlIdeaServiceSelector siteCategories(List siteCategories) {
this.siteCategories = siteCategories;
return this;
}
public PlacementUrlIdeaServiceSelector addSiteCategoriesItem(String siteCategoriesItem) {
if (this.siteCategories == null) {
this.siteCategories = new ArrayList<>();
}
this.siteCategories.add(siteCategoriesItem);
return this;
}
/**
* <div lang=\"ja\"> カテゴリの配列です。<br> ・URLのカテゴリです。<br> ・完全一致です。<br> ・複数指定でOR検索です。<br> ・TC-SC-xxxxxxで現される規定値です。<br> ・DicitonaryServiceから返ってくるTC-SC-xxxxxxをそのまま指定です。<br> ・最大50件です。 </div> <div lang=\"en\"> Array of categories.<br> ・Category of URL<br> ・Exact match<br> ・Search multiple specific keywords (OR)<br> ・From value: TC-SC-xxxxxx<br> ・Choose the TC-SC-xxxxxx value from DictionaryService<br> ・Maximum of 50 cases </div>
* @return siteCategories
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " カテゴリの配列です。
・URLのカテゴリです。
・完全一致です。
・複数指定でOR検索です。
・TC-SC-xxxxxxで現される規定値です。
・DicitonaryServiceから返ってくるTC-SC-xxxxxxをそのまま指定です。
・最大50件です。 Array of categories.
・Category of URL
・Exact match
・Search multiple specific keywords (OR)
・From value: TC-SC-xxxxxx
・Choose the TC-SC-xxxxxx value from DictionaryService
・Maximum of 50 cases ")
@JsonProperty(JSON_PROPERTY_SITE_CATEGORIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSiteCategories() {
return siteCategories;
}
@JsonProperty(JSON_PROPERTY_SITE_CATEGORIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSiteCategories(List siteCategories) {
this.siteCategories = siteCategories;
}
public PlacementUrlIdeaServiceSelector numberResults(Integer numberResults) {
this.numberResults = numberResults;
return this;
}
/**
* <div lang=\"ja\">開始位置から取得する結果の数。このフィールドは、1以上を指定する必要があります。</div> <div lang=\"en\">The number of results to retrieve starting from the initial position. This field must be greater than or equal to 1.</div>
* minimum: 1
* maximum: 500
* @return numberResults
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "開始位置から取得する結果の数。このフィールドは、1以上を指定する必要があります。 The number of results to retrieve starting from the initial position. This field must be greater than or equal to 1. ")
@JsonProperty(JSON_PROPERTY_NUMBER_RESULTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getNumberResults() {
return numberResults;
}
@JsonProperty(JSON_PROPERTY_NUMBER_RESULTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNumberResults(Integer numberResults) {
this.numberResults = numberResults;
}
public PlacementUrlIdeaServiceSelector startIndex(Integer startIndex) {
this.startIndex = startIndex;
return this;
}
/**
* <div lang=\"ja\">取得を開始する結果セット内の位置。このフィールドは、0以上を指定する必要があります。</div> <div lang=\"en\">The position within the result set where retrieval begins. This field must be greater than or equal to 0.</div>
* minimum: 1
* @return startIndex
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "取得を開始する結果セット内の位置。このフィールドは、0以上を指定する必要があります。 The position within the result set where retrieval begins. This field must be greater than or equal to 0. ")
@JsonProperty(JSON_PROPERTY_START_INDEX)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getStartIndex() {
return startIndex;
}
@JsonProperty(JSON_PROPERTY_START_INDEX)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStartIndex(Integer startIndex) {
this.startIndex = startIndex;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PlacementUrlIdeaServiceSelector placementUrlIdeaServiceSelector = (PlacementUrlIdeaServiceSelector) o;
return Objects.equals(this.keyword, placementUrlIdeaServiceSelector.keyword) &&
Objects.equals(this.siteCategories, placementUrlIdeaServiceSelector.siteCategories) &&
Objects.equals(this.numberResults, placementUrlIdeaServiceSelector.numberResults) &&
Objects.equals(this.startIndex, placementUrlIdeaServiceSelector.startIndex);
}
@Override
public int hashCode() {
return Objects.hash(keyword, siteCategories, numberResults, startIndex);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PlacementUrlIdeaServiceSelector {\n");
sb.append(" keyword: ").append(toIndentedString(keyword)).append("\n");
sb.append(" siteCategories: ").append(toIndentedString(siteCategories)).append("\n");
sb.append(" numberResults: ").append(toIndentedString(numberResults)).append("\n");
sb.append(" startIndex: ").append(toIndentedString(startIndex)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy