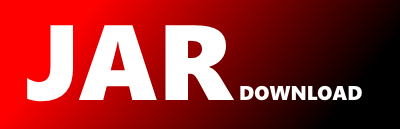
jp.co.yahoo.adsdisplayapi.v13.model.PlacementUrlList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v13
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v13.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import jp.co.yahoo.adsdisplayapi.v13.model.PlacementUrlListServiceIsRemoveFlg;
import jp.co.yahoo.adsdisplayapi.v13.model.PlacementUrlListServiceUnknownDomainFlg;
import jp.co.yahoo.adsdisplayapi.v13.model.PlacementUrlListServiceUrlList;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\">PlacementUrlListオブジェクトは、プレイスメントUrl情報を保持するオブジェクトです。</div> <div lang=\"en\">The objects to keep Placement Url Information.</div>
*/
@ApiModel(description = "PlacementUrlListオブジェクトは、プレイスメントUrl情報を保持するオブジェクトです。 The objects to keep Placement Url Information. ")
@JsonPropertyOrder({
PlacementUrlList.JSON_PROPERTY_ACCOUNT_ID,
PlacementUrlList.JSON_PROPERTY_DESCRIPTION,
PlacementUrlList.JSON_PROPERTY_IS_REMOVE_DESCRIPTION,
PlacementUrlList.JSON_PROPERTY_UNKNOWN_DOMAIN_FLG,
PlacementUrlList.JSON_PROPERTY_URL_LIST_ID,
PlacementUrlList.JSON_PROPERTY_URL_LIST_NAME,
PlacementUrlList.JSON_PROPERTY_BRAND_SAFETY_DENY_LIST_FLG,
PlacementUrlList.JSON_PROPERTY_URLS
})
@JsonTypeName("PlacementUrlList")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PlacementUrlList {
public static final String JSON_PROPERTY_ACCOUNT_ID = "accountId";
private Long accountId;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_IS_REMOVE_DESCRIPTION = "isRemoveDescription";
private PlacementUrlListServiceIsRemoveFlg isRemoveDescription;
public static final String JSON_PROPERTY_UNKNOWN_DOMAIN_FLG = "unknownDomainFlg";
private PlacementUrlListServiceUnknownDomainFlg unknownDomainFlg;
public static final String JSON_PROPERTY_URL_LIST_ID = "urlListId";
private Long urlListId;
public static final String JSON_PROPERTY_URL_LIST_NAME = "urlListName";
private String urlListName;
public static final String JSON_PROPERTY_BRAND_SAFETY_DENY_LIST_FLG = "brandSafetyDenyListFlg";
private Boolean brandSafetyDenyListFlg;
public static final String JSON_PROPERTY_URLS = "urls";
private List urls = null;
public PlacementUrlList() {
}
public PlacementUrlList accountId(Long accountId) {
this.accountId = accountId;
return this;
}
/**
* <div lang=\"ja\"> アカウントIDです。<br> このフィールドは、リクエストの場合は必須です。 </div> <div lang=\"en\"> Account ID.<br> This field is required in requests. </div>
* @return accountId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = " アカウントIDです。
このフィールドは、リクエストの場合は必須です。 Account ID.
This field is required in requests. ")
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getAccountId() {
return accountId;
}
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAccountId(Long accountId) {
this.accountId = accountId;
}
public PlacementUrlList description(String description) {
this.description = description;
return this;
}
/**
* <div lang=\"ja\"> urlリストの説明です。<br> このフィールドは、ADDおよびSET時に省略可能となります。 </div> <div lang=\"en\"> Url list description.<br> This field is optional in ADD and SET operation. </div>
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " urlリストの説明です。
このフィールドは、ADDおよびSET時に省略可能となります。 Url list description.
This field is optional in ADD and SET operation. ")
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public PlacementUrlList isRemoveDescription(PlacementUrlListServiceIsRemoveFlg isRemoveDescription) {
this.isRemoveDescription = isRemoveDescription;
return this;
}
/**
* Get isRemoveDescription
* @return isRemoveDescription
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_IS_REMOVE_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PlacementUrlListServiceIsRemoveFlg getIsRemoveDescription() {
return isRemoveDescription;
}
@JsonProperty(JSON_PROPERTY_IS_REMOVE_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsRemoveDescription(PlacementUrlListServiceIsRemoveFlg isRemoveDescription) {
this.isRemoveDescription = isRemoveDescription;
}
public PlacementUrlList unknownDomainFlg(PlacementUrlListServiceUnknownDomainFlg unknownDomainFlg) {
this.unknownDomainFlg = unknownDomainFlg;
return this;
}
/**
* Get unknownDomainFlg
* @return unknownDomainFlg
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_UNKNOWN_DOMAIN_FLG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PlacementUrlListServiceUnknownDomainFlg getUnknownDomainFlg() {
return unknownDomainFlg;
}
@JsonProperty(JSON_PROPERTY_UNKNOWN_DOMAIN_FLG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUnknownDomainFlg(PlacementUrlListServiceUnknownDomainFlg unknownDomainFlg) {
this.unknownDomainFlg = unknownDomainFlg;
}
public PlacementUrlList urlListId(Long urlListId) {
this.urlListId = urlListId;
return this;
}
/**
* <div lang=\"ja\"> urlリストIDです。<br> このフィールドは、SETおよびREMOVE時に必須となります。 </div> <div lang=\"en\"> Url list ID. <br> This field is required in SET and REMOVE operation. </div>
* @return urlListId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " urlリストIDです。
このフィールドは、SETおよびREMOVE時に必須となります。 Url list ID.
This field is required in SET and REMOVE operation. ")
@JsonProperty(JSON_PROPERTY_URL_LIST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getUrlListId() {
return urlListId;
}
@JsonProperty(JSON_PROPERTY_URL_LIST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUrlListId(Long urlListId) {
this.urlListId = urlListId;
}
public PlacementUrlList urlListName(String urlListName) {
this.urlListName = urlListName;
return this;
}
/**
* <div lang=\"ja\"> urlリスト名です。<br> このフィールドは、ADD時に必須となり、SET時に省略可能となります。 </div> <div lang=\"en\"> Url list name. <br> This field is required in ADD operation, and is optional in SET operation. </div>
* @return urlListName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " urlリスト名です。
このフィールドは、ADD時に必須となり、SET時に省略可能となります。 Url list name.
This field is required in ADD operation, and is optional in SET operation. ")
@JsonProperty(JSON_PROPERTY_URL_LIST_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUrlListName() {
return urlListName;
}
@JsonProperty(JSON_PROPERTY_URL_LIST_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUrlListName(String urlListName) {
this.urlListName = urlListName;
}
public PlacementUrlList brandSafetyDenyListFlg(Boolean brandSafetyDenyListFlg) {
this.brandSafetyDenyListFlg = brandSafetyDenyListFlg;
return this;
}
/**
* <div lang=\"ja\"> 除外専用リストかどうかのフラグです。<br> 除外専用リストには下記の制約があります。<br> ・1アカウントにつき1つ作成が可能です。<br> ・除外にのみ紐付け可能です。<br> このフィールドは、ADD時に省略可能(デフォルトの値はFALSE)、SET時に指定不可となります。 </div> <div lang=\"en\"> A flag that indicates whether it is an exclusion list. <br> Exclusion list includes the following restrictions: <br> *Only one list can be created per account. <br> *Can be linked only to exclusion. <br> This field is optional in ADD operation (default value will be FALSE), and cannot be specified in SET operation. </div> <dl class=term> <dt class=\"term__item\">TRUE</dt> <dd class=\"term__desc\"><span lang=\"ja\">除外専用リストです。</span><span lang=\"en\">Exclusion list.</span></dd> <dt class=\"term__item\">FALSE</dt> <dd class=\"term__desc\"><span lang=\"ja\">通常のurlリストです。</span><span lang=\"en\">Standard URL list.</span></dd> </dl>
* @return brandSafetyDenyListFlg
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 除外専用リストかどうかのフラグです。
除外専用リストには下記の制約があります。
・1アカウントにつき1つ作成が可能です。
・除外にのみ紐付け可能です。
このフィールドは、ADD時に省略可能(デフォルトの値はFALSE)、SET時に指定不可となります。 A flag that indicates whether it is an exclusion list.
Exclusion list includes the following restrictions:
*Only one list can be created per account.
*Can be linked only to exclusion.
This field is optional in ADD operation (default value will be FALSE), and cannot be specified in SET operation. - TRUE
- 除外専用リストです。Exclusion list.
- FALSE
- 通常のurlリストです。Standard URL list.
")
@JsonProperty(JSON_PROPERTY_BRAND_SAFETY_DENY_LIST_FLG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getBrandSafetyDenyListFlg() {
return brandSafetyDenyListFlg;
}
@JsonProperty(JSON_PROPERTY_BRAND_SAFETY_DENY_LIST_FLG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBrandSafetyDenyListFlg(Boolean brandSafetyDenyListFlg) {
this.brandSafetyDenyListFlg = brandSafetyDenyListFlg;
}
public PlacementUrlList urls(List urls) {
this.urls = urls;
return this;
}
public PlacementUrlList addUrlsItem(PlacementUrlListServiceUrlList urlsItem) {
if (this.urls == null) {
this.urls = new ArrayList<>();
}
this.urls.add(urlsItem);
return this;
}
/**
* Get urls
* @return urls
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_URLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getUrls() {
return urls;
}
@JsonProperty(JSON_PROPERTY_URLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUrls(List urls) {
this.urls = urls;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PlacementUrlList placementUrlList = (PlacementUrlList) o;
return Objects.equals(this.accountId, placementUrlList.accountId) &&
Objects.equals(this.description, placementUrlList.description) &&
Objects.equals(this.isRemoveDescription, placementUrlList.isRemoveDescription) &&
Objects.equals(this.unknownDomainFlg, placementUrlList.unknownDomainFlg) &&
Objects.equals(this.urlListId, placementUrlList.urlListId) &&
Objects.equals(this.urlListName, placementUrlList.urlListName) &&
Objects.equals(this.brandSafetyDenyListFlg, placementUrlList.brandSafetyDenyListFlg) &&
Objects.equals(this.urls, placementUrlList.urls);
}
@Override
public int hashCode() {
return Objects.hash(accountId, description, isRemoveDescription, unknownDomainFlg, urlListId, urlListName, brandSafetyDenyListFlg, urls);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PlacementUrlList {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" isRemoveDescription: ").append(toIndentedString(isRemoveDescription)).append("\n");
sb.append(" unknownDomainFlg: ").append(toIndentedString(unknownDomainFlg)).append("\n");
sb.append(" urlListId: ").append(toIndentedString(urlListId)).append("\n");
sb.append(" urlListName: ").append(toIndentedString(urlListName)).append("\n");
sb.append(" brandSafetyDenyListFlg: ").append(toIndentedString(brandSafetyDenyListFlg)).append("\n");
sb.append(" urls: ").append(toIndentedString(urls)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy