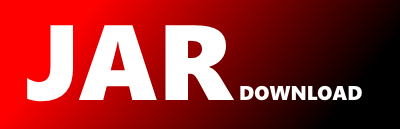
jp.co.yahoo.adsdisplayapi.v14.api.CampaignServiceApi Maven / Gradle / Ivy
Show all versions of ads-display-api-lib Show documentation
package jp.co.yahoo.adsdisplayapi.v14.api;
import jp.co.yahoo.adsdisplayapi.v14.ApiClient;
import jp.co.yahoo.adsdisplayapi.v14.model.CampaignServiceGetResponse;
import jp.co.yahoo.adsdisplayapi.v14.model.CampaignServiceMutateResponse;
import jp.co.yahoo.adsdisplayapi.v14.model.CampaignServiceOperation;
import jp.co.yahoo.adsdisplayapi.v14.model.CampaignServiceSelector;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.stream.Collectors;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestClientException;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
@Component("jp.co.yahoo.adsdisplayapi.v14.api.CampaignServiceApi")
public class CampaignServiceApi {
private ApiClient apiClient;
public CampaignServiceApi() {
this(new ApiClient());
}
@Autowired
public CampaignServiceApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
*
* <div lang=\"ja\"> キャンペーン情報を追加します。<br> </div> <div lang=\"en\"> Adds campaign information.<br> </div>
* 503 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0099</td><td>Out of service.</td><td><div lang=\"ja\">APIがメンテナンス中、またはご利用できません。</div><div lang=\"en\">API is under maintenance or not available to use.</div></td></tr> </tbody> </table> </div>
*
500 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0002</td><td>An internal error has occurred.</td><td><div lang=\"ja\">内部エラーが発生しました。再度操作を実行してください。 <br>もし、問題が解決しない場合は、お問い合わせページをご利用ください。</div><div lang=\"en\">An internal error has occurred. Please try again later. <br>If the problem continues, please contact the support team via Inquiry page for assistance. </div></td></tr> </tbody> </table> </div>
*
403 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0098</td><td>Permission denied.</td><td><div lang=\"ja\">権限がありません。</div><div lang=\"en\">Permission denied.</div></td></tr> <tr><td>0003</td><td>Frequency limit exceeded. Please try your request again later</td><td><div lang=\"ja\">アクセス頻度が上限値に達しました。時間をおいて再度実行してください。</div><div lang=\"en\">Frequency limit exceeded. Please try your request again later.</div></td></tr> </tbody> </table> </div>
*
401 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0110</td><td>Require access token.</td><td><div lang=\"ja\">アクセストークンがリクエストヘッダに存在していません。</div><div lang=\"en\">There is no access token in the request header.</div></td></tr> <tr><td>0111</td><td>Authentication failed.</td><td><div lang=\"ja\">アクセストークンの認証に失敗しました。</div><div lang=\"en\">The access token authentication failed.</div></td></tr> <tr><td>0112</td><td>Account not found.</td><td><div lang=\"ja\">アカウントが見つかりませんでした。</div><div lang=\"en\">The account can not found.</div></td></tr> <tr><td>0113</td><td>Deactivated account.</td><td><div lang=\"ja\">アカウントが無効です。</div><div lang=\"en\">The account is deactivated.</div></td></tr> </tbody> </table> </div>
*
400 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0114</td><td>Invalid scope.</td><td><div lang=\"ja\">アクセストークンが無効です。</div><div lang=\"en\">The access token is invalid.</div></td></tr> </tbody> </table> </div>
*
200 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0001</td><td>Invalid Request.</td><td><div lang=\"ja\">さまざまな要因が考えられます。<br>主な要因は、パラメータの値が不正か、誤りがあるためにオペレーションが完了できません。</div><div lang=\"en\">This error can result for a variety of reasons. <br>Typically because one of the parameter values in the request is wrong or invalid and the operation cannot be completed.</div></td></tr> <tr><td>R0001</td><td>Require.</td><td><div lang=\"ja\">必須です。</div><div lang=\"en\">It is missing required parameter.</div></td></tr> <tr><td>V0001</td><td>Invalid value.</td><td><div lang=\"ja\">値が無効です。</div><div lang=\"en\">The value is invalid.</div></td></tr> <tr><td>I0001</td><td>Deactivated Id.</td><td><div lang=\"ja\">IDが無効です。</div><div lang=\"en\">The ID is Deactivated.</div></td></tr> <tr><td>F0001</td><td>Invalid format.</td><td><div lang=\"ja\">値の形式が不正です。</div><div lang=\"en\">The value format is invalid.</div></td></tr> <tr><td>110005</td><td>Not a valid id.</td><td><div lang=\"ja\">IDが有効ではありません。</div><div lang=\"en\">Not a valid id.</div></td></tr> <tr><td>L0002</td><td>Over list size.</td><td><div lang=\"ja\">配列の要素数が上限値を超過しています。</div><div lang=\"en\">The number of elements in the array exceeds the upper limit.</div></td></tr> <tr><td>D0001</td><td>Duplicate.</td><td><div lang=\"ja\">一意な値が重複しています。</div><div lang=\"en\">The unique value is duplicated.</div></td></tr> <tr><td>RL001</td><td>Invalid relation.</td><td><div lang=\"ja\">リクエストの関連性が矛盾しています。<br> たとえば、開始>終了の日付指定を行なっているなど</div><div lang=\"en\">The relation of the request is contradictory.<br> For example, you are specifying the date of start > end.</div></td></tr> <tr><td>L0001</td><td>Lower list size.</td><td><div lang=\"ja\">配列の要素数が下限値を下回っています。</div><div lang=\"en\">The number of elements in the array is below the lower limit.</div></td></tr> <tr><td>120020</td><td>Can not set value.</td><td><div lang=\"ja\">値を設定することができません。</div><div lang=\"en\">Value cannot be set.</div></td></tr> <tr><td>220014</td><td>Budget is lower than bidding price.</td><td><div lang=\"ja\">予算額が入札価格を下回っています。</div><div lang=\"en\">The bidding price has been set higher than the budget.</div></td></tr> <tr><td>LT001</td><td>Over limit.</td><td><div lang=\"ja\">登録できる上限値を超過しています。</div><div lang=\"en\">The upper limit value that can be registered is exceeded.</div></td></tr> <tr><td>220121</td><td>Frequency setting was made to the product that is not available.</td><td><div lang=\"ja\">指定された広告商品ではフリークエンシー設定できません。</div><div lang=\"en\">You can not set frequency ads instruments designated.</div></td></tr> <tr><td>220122</td><td>Unavailable combination of frequency settings.</td><td><div lang=\"ja\">フリークエンシー設定の組み合わせが不正です。</div><div lang=\"en\">Combination of frequency setting is invalid.</div></td></tr> <tr><td>220123</td><td>Unavailable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの設定が出来ない商品です。</div><div lang=\"en\">Conversion optimization setting is not possible due to the non-eligible Ad distribution.</div></td></tr> <tr><td>220124</td><td>Disable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの有効設定が不正です。</div><div lang=\"en\">Target CPA cannot be set</div></td></tr> <tr><td>220144</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">企業の配下でSKAdNetwork計測できる広告グループは最大30件までです。</div><div lang=\"en\">The maximum number of ad groups that can be optimized for SKAdNetwork under the control of a company is 30.</div></td></tr> <tr><td>220145</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">広告アカウント配下でSKAdNetwork計測できるのは最大30件までです。</div><div lang=\"en\">The maximum number of ad groups that can be optimized for SKAdNetwork under the control of an ad account is 30.</div></td></tr> <tr><td>220147</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">SKAdNetwork計測利用時の制限により、作成および変更できません。SKAdNetwork計測利用時は、配信オンの広告グループが上限数に満たない場合でも設定変更できないケースがあります。お手数ですが数日後(最大5日間)に再試行してください。</div><div lang=\"en\">Due to the limitations of SKAdNetwork, you can't create or change the settings for ad groups. When using SKAdNetwork, you may not be able to change settings even if you don't have a maximum number of ad groups. Try again in a few days (up to 5 days).</div></td></tr> </tbody> </table> </div>
* @param xZBaseAccountId <div lang=\"ja\">アカウントIDを指定してください。</div> <div lang=\"en\">Specify Account ID.</div> (required)
* @param campaignServiceOperation (optional)
* @return CampaignServiceMutateResponse
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public CampaignServiceMutateResponse campaignServiceAddPost(Long xZBaseAccountId, CampaignServiceOperation campaignServiceOperation) throws RestClientException {
return campaignServiceAddPostWithHttpInfo(xZBaseAccountId, campaignServiceOperation).getBody();
}
/**
*
* <div lang=\"ja\"> キャンペーン情報を追加します。<br> </div> <div lang=\"en\"> Adds campaign information.<br> </div>
*
503 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0099</td><td>Out of service.</td><td><div lang=\"ja\">APIがメンテナンス中、またはご利用できません。</div><div lang=\"en\">API is under maintenance or not available to use.</div></td></tr> </tbody> </table> </div>
*
500 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0002</td><td>An internal error has occurred.</td><td><div lang=\"ja\">内部エラーが発生しました。再度操作を実行してください。 <br>もし、問題が解決しない場合は、お問い合わせページをご利用ください。</div><div lang=\"en\">An internal error has occurred. Please try again later. <br>If the problem continues, please contact the support team via Inquiry page for assistance. </div></td></tr> </tbody> </table> </div>
*
403 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0098</td><td>Permission denied.</td><td><div lang=\"ja\">権限がありません。</div><div lang=\"en\">Permission denied.</div></td></tr> <tr><td>0003</td><td>Frequency limit exceeded. Please try your request again later</td><td><div lang=\"ja\">アクセス頻度が上限値に達しました。時間をおいて再度実行してください。</div><div lang=\"en\">Frequency limit exceeded. Please try your request again later.</div></td></tr> </tbody> </table> </div>
*
401 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0110</td><td>Require access token.</td><td><div lang=\"ja\">アクセストークンがリクエストヘッダに存在していません。</div><div lang=\"en\">There is no access token in the request header.</div></td></tr> <tr><td>0111</td><td>Authentication failed.</td><td><div lang=\"ja\">アクセストークンの認証に失敗しました。</div><div lang=\"en\">The access token authentication failed.</div></td></tr> <tr><td>0112</td><td>Account not found.</td><td><div lang=\"ja\">アカウントが見つかりませんでした。</div><div lang=\"en\">The account can not found.</div></td></tr> <tr><td>0113</td><td>Deactivated account.</td><td><div lang=\"ja\">アカウントが無効です。</div><div lang=\"en\">The account is deactivated.</div></td></tr> </tbody> </table> </div>
*
400 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0114</td><td>Invalid scope.</td><td><div lang=\"ja\">アクセストークンが無効です。</div><div lang=\"en\">The access token is invalid.</div></td></tr> </tbody> </table> </div>
*
200 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0001</td><td>Invalid Request.</td><td><div lang=\"ja\">さまざまな要因が考えられます。<br>主な要因は、パラメータの値が不正か、誤りがあるためにオペレーションが完了できません。</div><div lang=\"en\">This error can result for a variety of reasons. <br>Typically because one of the parameter values in the request is wrong or invalid and the operation cannot be completed.</div></td></tr> <tr><td>R0001</td><td>Require.</td><td><div lang=\"ja\">必須です。</div><div lang=\"en\">It is missing required parameter.</div></td></tr> <tr><td>V0001</td><td>Invalid value.</td><td><div lang=\"ja\">値が無効です。</div><div lang=\"en\">The value is invalid.</div></td></tr> <tr><td>I0001</td><td>Deactivated Id.</td><td><div lang=\"ja\">IDが無効です。</div><div lang=\"en\">The ID is Deactivated.</div></td></tr> <tr><td>F0001</td><td>Invalid format.</td><td><div lang=\"ja\">値の形式が不正です。</div><div lang=\"en\">The value format is invalid.</div></td></tr> <tr><td>110005</td><td>Not a valid id.</td><td><div lang=\"ja\">IDが有効ではありません。</div><div lang=\"en\">Not a valid id.</div></td></tr> <tr><td>L0002</td><td>Over list size.</td><td><div lang=\"ja\">配列の要素数が上限値を超過しています。</div><div lang=\"en\">The number of elements in the array exceeds the upper limit.</div></td></tr> <tr><td>D0001</td><td>Duplicate.</td><td><div lang=\"ja\">一意な値が重複しています。</div><div lang=\"en\">The unique value is duplicated.</div></td></tr> <tr><td>RL001</td><td>Invalid relation.</td><td><div lang=\"ja\">リクエストの関連性が矛盾しています。<br> たとえば、開始>終了の日付指定を行なっているなど</div><div lang=\"en\">The relation of the request is contradictory.<br> For example, you are specifying the date of start > end.</div></td></tr> <tr><td>L0001</td><td>Lower list size.</td><td><div lang=\"ja\">配列の要素数が下限値を下回っています。</div><div lang=\"en\">The number of elements in the array is below the lower limit.</div></td></tr> <tr><td>120020</td><td>Can not set value.</td><td><div lang=\"ja\">値を設定することができません。</div><div lang=\"en\">Value cannot be set.</div></td></tr> <tr><td>220014</td><td>Budget is lower than bidding price.</td><td><div lang=\"ja\">予算額が入札価格を下回っています。</div><div lang=\"en\">The bidding price has been set higher than the budget.</div></td></tr> <tr><td>LT001</td><td>Over limit.</td><td><div lang=\"ja\">登録できる上限値を超過しています。</div><div lang=\"en\">The upper limit value that can be registered is exceeded.</div></td></tr> <tr><td>220121</td><td>Frequency setting was made to the product that is not available.</td><td><div lang=\"ja\">指定された広告商品ではフリークエンシー設定できません。</div><div lang=\"en\">You can not set frequency ads instruments designated.</div></td></tr> <tr><td>220122</td><td>Unavailable combination of frequency settings.</td><td><div lang=\"ja\">フリークエンシー設定の組み合わせが不正です。</div><div lang=\"en\">Combination of frequency setting is invalid.</div></td></tr> <tr><td>220123</td><td>Unavailable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの設定が出来ない商品です。</div><div lang=\"en\">Conversion optimization setting is not possible due to the non-eligible Ad distribution.</div></td></tr> <tr><td>220124</td><td>Disable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの有効設定が不正です。</div><div lang=\"en\">Target CPA cannot be set</div></td></tr> <tr><td>220144</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">企業の配下でSKAdNetwork計測できる広告グループは最大30件までです。</div><div lang=\"en\">The maximum number of ad groups that can be optimized for SKAdNetwork under the control of a company is 30.</div></td></tr> <tr><td>220145</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">広告アカウント配下でSKAdNetwork計測できるのは最大30件までです。</div><div lang=\"en\">The maximum number of ad groups that can be optimized for SKAdNetwork under the control of an ad account is 30.</div></td></tr> <tr><td>220147</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">SKAdNetwork計測利用時の制限により、作成および変更できません。SKAdNetwork計測利用時は、配信オンの広告グループが上限数に満たない場合でも設定変更できないケースがあります。お手数ですが数日後(最大5日間)に再試行してください。</div><div lang=\"en\">Due to the limitations of SKAdNetwork, you can't create or change the settings for ad groups. When using SKAdNetwork, you may not be able to change settings even if you don't have a maximum number of ad groups. Try again in a few days (up to 5 days).</div></td></tr> </tbody> </table> </div>
* @param xZBaseAccountId <div lang=\"ja\">アカウントIDを指定してください。</div> <div lang=\"en\">Specify Account ID.</div> (required)
* @param campaignServiceOperation (optional)
* @return ResponseEntity<CampaignServiceMutateResponse>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity campaignServiceAddPostWithHttpInfo(Long xZBaseAccountId, CampaignServiceOperation campaignServiceOperation) throws RestClientException {
Object postBody = campaignServiceOperation;
// verify the required parameter 'xZBaseAccountId' is set
if (xZBaseAccountId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xZBaseAccountId' when calling campaignServiceAddPost");
}
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xZBaseAccountId != null)
headerParams.add("x-z-base-account-id", apiClient.parameterToString(xZBaseAccountId));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] contentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "oAuth" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/CampaignService/add", HttpMethod.POST, Collections.emptyMap(), queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, authNames, returnType);
}
/**
*
* <div lang=\"ja\">キャンペーン情報を取得します。</div> <div lang=\"en\">Gets information related to campaigns.</div>
* 503 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0099</td><td>Out of service.</td><td><div lang=\"ja\">APIがメンテナンス中、またはご利用できません。</div><div lang=\"en\">API is under maintenance or not available to use.</div></td></tr> </tbody> </table> </div>
*
500 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0002</td><td>An internal error has occurred.</td><td><div lang=\"ja\">内部エラーが発生しました。再度操作を実行してください。 <br>もし、問題が解決しない場合は、お問い合わせページをご利用ください。</div><div lang=\"en\">An internal error has occurred. Please try again later. <br>If the problem continues, please contact the support team via Inquiry page for assistance. </div></td></tr> </tbody> </table> </div>
*
403 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0098</td><td>Permission denied.</td><td><div lang=\"ja\">権限がありません。</div><div lang=\"en\">Permission denied.</div></td></tr> <tr><td>0003</td><td>Frequency limit exceeded. Please try your request again later</td><td><div lang=\"ja\">アクセス頻度が上限値に達しました。時間をおいて再度実行してください。</div><div lang=\"en\">Frequency limit exceeded. Please try your request again later.</div></td></tr> </tbody> </table> </div>
*
401 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0110</td><td>Require access token.</td><td><div lang=\"ja\">アクセストークンがリクエストヘッダに存在していません。</div><div lang=\"en\">There is no access token in the request header.</div></td></tr> <tr><td>0111</td><td>Authentication failed.</td><td><div lang=\"ja\">アクセストークンの認証に失敗しました。</div><div lang=\"en\">The access token authentication failed.</div></td></tr> <tr><td>0112</td><td>Account not found.</td><td><div lang=\"ja\">アカウントが見つかりませんでした。</div><div lang=\"en\">The account can not found.</div></td></tr> <tr><td>0113</td><td>Deactivated account.</td><td><div lang=\"ja\">アカウントが無効です。</div><div lang=\"en\">The account is deactivated.</div></td></tr> </tbody> </table> </div>
*
400 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0114</td><td>Invalid scope.</td><td><div lang=\"ja\">アクセストークンが無効です。</div><div lang=\"en\">The access token is invalid.</div></td></tr> </tbody> </table> </div>
*
200 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0001</td><td>Invalid Request.</td><td><div lang=\"ja\">さまざまな要因が考えられます。<br>主な要因は、パラメータの値が不正か、誤りがあるためにオペレーションが完了できません。</div><div lang=\"en\">This error can result for a variety of reasons. <br>Typically because one of the parameter values in the request is wrong or invalid and the operation cannot be completed.</div></td></tr> <tr><td>R0001</td><td>Require.</td><td><div lang=\"ja\">必須です。</div><div lang=\"en\">It is missing required parameter.</div></td></tr> <tr><td>V0001</td><td>Invalid value.</td><td><div lang=\"ja\">値が無効です。</div><div lang=\"en\">The value is invalid.</div></td></tr> <tr><td>I0001</td><td>Deactivated Id.</td><td><div lang=\"ja\">IDが無効です。</div><div lang=\"en\">The ID is Deactivated.</div></td></tr> <tr><td>F0001</td><td>Invalid format.</td><td><div lang=\"ja\">値の形式が不正です。</div><div lang=\"en\">The value format is invalid.</div></td></tr> <tr><td>110005</td><td>Not a valid id.</td><td><div lang=\"ja\">IDが有効ではありません。</div><div lang=\"en\">Not a valid id.</div></td></tr> <tr><td>L0002</td><td>Over list size.</td><td><div lang=\"ja\">配列の要素数が上限値を超過しています。</div><div lang=\"en\">The number of elements in the array exceeds the upper limit.</div></td></tr> <tr><td>D0001</td><td>Duplicate.</td><td><div lang=\"ja\">一意な値が重複しています。</div><div lang=\"en\">The unique value is duplicated.</div></td></tr> <tr><td>RL001</td><td>Invalid relation.</td><td><div lang=\"ja\">リクエストの関連性が矛盾しています。<br> たとえば、開始>終了の日付指定を行なっているなど</div><div lang=\"en\">The relation of the request is contradictory.<br> For example, you are specifying the date of start > end.</div></td></tr> <tr><td>L0001</td><td>Lower list size.</td><td><div lang=\"ja\">配列の要素数が下限値を下回っています。</div><div lang=\"en\">The number of elements in the array is below the lower limit.</div></td></tr> <tr><td>120020</td><td>Can not set value.</td><td><div lang=\"ja\">値を設定することができません。</div><div lang=\"en\">Value cannot be set.</div></td></tr> <tr><td>220014</td><td>Budget is lower than bidding price.</td><td><div lang=\"ja\">予算額が入札価格を下回っています。</div><div lang=\"en\">The bidding price has been set higher than the budget.</div></td></tr> <tr><td>LT001</td><td>Over limit.</td><td><div lang=\"ja\">登録できる上限値を超過しています。</div><div lang=\"en\">The upper limit value that can be registered is exceeded.</div></td></tr> <tr><td>220121</td><td>Frequency setting was made to the product that is not available.</td><td><div lang=\"ja\">指定された広告商品ではフリークエンシー設定できません。</div><div lang=\"en\">You can not set frequency ads instruments designated.</div></td></tr> <tr><td>220122</td><td>Unavailable combination of frequency settings.</td><td><div lang=\"ja\">フリークエンシー設定の組み合わせが不正です。</div><div lang=\"en\">Combination of frequency setting is invalid.</div></td></tr> <tr><td>220123</td><td>Unavailable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの設定が出来ない商品です。</div><div lang=\"en\">Conversion optimization setting is not possible due to the non-eligible Ad distribution.</div></td></tr> <tr><td>220124</td><td>Disable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの有効設定が不正です。</div><div lang=\"en\">Target CPA cannot be set</div></td></tr> </tbody> </table> </div>
* @param xZBaseAccountId <div lang=\"ja\">アカウントIDを指定してください。</div> <div lang=\"en\">Specify Account ID.</div> (required)
* @param campaignServiceSelector (optional)
* @return CampaignServiceGetResponse
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public CampaignServiceGetResponse campaignServiceGetPost(Long xZBaseAccountId, CampaignServiceSelector campaignServiceSelector) throws RestClientException {
return campaignServiceGetPostWithHttpInfo(xZBaseAccountId, campaignServiceSelector).getBody();
}
/**
*
* <div lang=\"ja\">キャンペーン情報を取得します。</div> <div lang=\"en\">Gets information related to campaigns.</div>
*
503 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0099</td><td>Out of service.</td><td><div lang=\"ja\">APIがメンテナンス中、またはご利用できません。</div><div lang=\"en\">API is under maintenance or not available to use.</div></td></tr> </tbody> </table> </div>
*
500 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0002</td><td>An internal error has occurred.</td><td><div lang=\"ja\">内部エラーが発生しました。再度操作を実行してください。 <br>もし、問題が解決しない場合は、お問い合わせページをご利用ください。</div><div lang=\"en\">An internal error has occurred. Please try again later. <br>If the problem continues, please contact the support team via Inquiry page for assistance. </div></td></tr> </tbody> </table> </div>
*
403 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0098</td><td>Permission denied.</td><td><div lang=\"ja\">権限がありません。</div><div lang=\"en\">Permission denied.</div></td></tr> <tr><td>0003</td><td>Frequency limit exceeded. Please try your request again later</td><td><div lang=\"ja\">アクセス頻度が上限値に達しました。時間をおいて再度実行してください。</div><div lang=\"en\">Frequency limit exceeded. Please try your request again later.</div></td></tr> </tbody> </table> </div>
*
401 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0110</td><td>Require access token.</td><td><div lang=\"ja\">アクセストークンがリクエストヘッダに存在していません。</div><div lang=\"en\">There is no access token in the request header.</div></td></tr> <tr><td>0111</td><td>Authentication failed.</td><td><div lang=\"ja\">アクセストークンの認証に失敗しました。</div><div lang=\"en\">The access token authentication failed.</div></td></tr> <tr><td>0112</td><td>Account not found.</td><td><div lang=\"ja\">アカウントが見つかりませんでした。</div><div lang=\"en\">The account can not found.</div></td></tr> <tr><td>0113</td><td>Deactivated account.</td><td><div lang=\"ja\">アカウントが無効です。</div><div lang=\"en\">The account is deactivated.</div></td></tr> </tbody> </table> </div>
*
400 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0114</td><td>Invalid scope.</td><td><div lang=\"ja\">アクセストークンが無効です。</div><div lang=\"en\">The access token is invalid.</div></td></tr> </tbody> </table> </div>
*
200 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0001</td><td>Invalid Request.</td><td><div lang=\"ja\">さまざまな要因が考えられます。<br>主な要因は、パラメータの値が不正か、誤りがあるためにオペレーションが完了できません。</div><div lang=\"en\">This error can result for a variety of reasons. <br>Typically because one of the parameter values in the request is wrong or invalid and the operation cannot be completed.</div></td></tr> <tr><td>R0001</td><td>Require.</td><td><div lang=\"ja\">必須です。</div><div lang=\"en\">It is missing required parameter.</div></td></tr> <tr><td>V0001</td><td>Invalid value.</td><td><div lang=\"ja\">値が無効です。</div><div lang=\"en\">The value is invalid.</div></td></tr> <tr><td>I0001</td><td>Deactivated Id.</td><td><div lang=\"ja\">IDが無効です。</div><div lang=\"en\">The ID is Deactivated.</div></td></tr> <tr><td>F0001</td><td>Invalid format.</td><td><div lang=\"ja\">値の形式が不正です。</div><div lang=\"en\">The value format is invalid.</div></td></tr> <tr><td>110005</td><td>Not a valid id.</td><td><div lang=\"ja\">IDが有効ではありません。</div><div lang=\"en\">Not a valid id.</div></td></tr> <tr><td>L0002</td><td>Over list size.</td><td><div lang=\"ja\">配列の要素数が上限値を超過しています。</div><div lang=\"en\">The number of elements in the array exceeds the upper limit.</div></td></tr> <tr><td>D0001</td><td>Duplicate.</td><td><div lang=\"ja\">一意な値が重複しています。</div><div lang=\"en\">The unique value is duplicated.</div></td></tr> <tr><td>RL001</td><td>Invalid relation.</td><td><div lang=\"ja\">リクエストの関連性が矛盾しています。<br> たとえば、開始>終了の日付指定を行なっているなど</div><div lang=\"en\">The relation of the request is contradictory.<br> For example, you are specifying the date of start > end.</div></td></tr> <tr><td>L0001</td><td>Lower list size.</td><td><div lang=\"ja\">配列の要素数が下限値を下回っています。</div><div lang=\"en\">The number of elements in the array is below the lower limit.</div></td></tr> <tr><td>120020</td><td>Can not set value.</td><td><div lang=\"ja\">値を設定することができません。</div><div lang=\"en\">Value cannot be set.</div></td></tr> <tr><td>220014</td><td>Budget is lower than bidding price.</td><td><div lang=\"ja\">予算額が入札価格を下回っています。</div><div lang=\"en\">The bidding price has been set higher than the budget.</div></td></tr> <tr><td>LT001</td><td>Over limit.</td><td><div lang=\"ja\">登録できる上限値を超過しています。</div><div lang=\"en\">The upper limit value that can be registered is exceeded.</div></td></tr> <tr><td>220121</td><td>Frequency setting was made to the product that is not available.</td><td><div lang=\"ja\">指定された広告商品ではフリークエンシー設定できません。</div><div lang=\"en\">You can not set frequency ads instruments designated.</div></td></tr> <tr><td>220122</td><td>Unavailable combination of frequency settings.</td><td><div lang=\"ja\">フリークエンシー設定の組み合わせが不正です。</div><div lang=\"en\">Combination of frequency setting is invalid.</div></td></tr> <tr><td>220123</td><td>Unavailable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの設定が出来ない商品です。</div><div lang=\"en\">Conversion optimization setting is not possible due to the non-eligible Ad distribution.</div></td></tr> <tr><td>220124</td><td>Disable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの有効設定が不正です。</div><div lang=\"en\">Target CPA cannot be set</div></td></tr> </tbody> </table> </div>
* @param xZBaseAccountId <div lang=\"ja\">アカウントIDを指定してください。</div> <div lang=\"en\">Specify Account ID.</div> (required)
* @param campaignServiceSelector (optional)
* @return ResponseEntity<CampaignServiceGetResponse>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity campaignServiceGetPostWithHttpInfo(Long xZBaseAccountId, CampaignServiceSelector campaignServiceSelector) throws RestClientException {
Object postBody = campaignServiceSelector;
// verify the required parameter 'xZBaseAccountId' is set
if (xZBaseAccountId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xZBaseAccountId' when calling campaignServiceGetPost");
}
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xZBaseAccountId != null)
headerParams.add("x-z-base-account-id", apiClient.parameterToString(xZBaseAccountId));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] contentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "oAuth" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/CampaignService/get", HttpMethod.POST, Collections.emptyMap(), queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, authNames, returnType);
}
/**
*
* <div lang=\"ja\"> キャンペーン情報を削除します。<br> </div> <div lang=\"en\"> Deletes campaign information.<br> </div>
* 503 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0099</td><td>Out of service.</td><td><div lang=\"ja\">APIがメンテナンス中、またはご利用できません。</div><div lang=\"en\">API is under maintenance or not available to use.</div></td></tr> </tbody> </table> </div>
*
500 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0002</td><td>An internal error has occurred.</td><td><div lang=\"ja\">内部エラーが発生しました。再度操作を実行してください。 <br>もし、問題が解決しない場合は、お問い合わせページをご利用ください。</div><div lang=\"en\">An internal error has occurred. Please try again later. <br>If the problem continues, please contact the support team via Inquiry page for assistance. </div></td></tr> </tbody> </table> </div>
*
403 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0098</td><td>Permission denied.</td><td><div lang=\"ja\">権限がありません。</div><div lang=\"en\">Permission denied.</div></td></tr> <tr><td>0003</td><td>Frequency limit exceeded. Please try your request again later</td><td><div lang=\"ja\">アクセス頻度が上限値に達しました。時間をおいて再度実行してください。</div><div lang=\"en\">Frequency limit exceeded. Please try your request again later.</div></td></tr> </tbody> </table> </div>
*
401 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0110</td><td>Require access token.</td><td><div lang=\"ja\">アクセストークンがリクエストヘッダに存在していません。</div><div lang=\"en\">There is no access token in the request header.</div></td></tr> <tr><td>0111</td><td>Authentication failed.</td><td><div lang=\"ja\">アクセストークンの認証に失敗しました。</div><div lang=\"en\">The access token authentication failed.</div></td></tr> <tr><td>0112</td><td>Account not found.</td><td><div lang=\"ja\">アカウントが見つかりませんでした。</div><div lang=\"en\">The account can not found.</div></td></tr> <tr><td>0113</td><td>Deactivated account.</td><td><div lang=\"ja\">アカウントが無効です。</div><div lang=\"en\">The account is deactivated.</div></td></tr> </tbody> </table> </div>
*
400 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0114</td><td>Invalid scope.</td><td><div lang=\"ja\">アクセストークンが無効です。</div><div lang=\"en\">The access token is invalid.</div></td></tr> </tbody> </table> </div>
*
200 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0001</td><td>Invalid Request.</td><td><div lang=\"ja\">さまざまな要因が考えられます。<br>主な要因は、パラメータの値が不正か、誤りがあるためにオペレーションが完了できません。</div><div lang=\"en\">This error can result for a variety of reasons. <br>Typically because one of the parameter values in the request is wrong or invalid and the operation cannot be completed.</div></td></tr> <tr><td>R0001</td><td>Require.</td><td><div lang=\"ja\">必須です。</div><div lang=\"en\">It is missing required parameter.</div></td></tr> <tr><td>V0001</td><td>Invalid value.</td><td><div lang=\"ja\">値が無効です。</div><div lang=\"en\">The value is invalid.</div></td></tr> <tr><td>I0001</td><td>Deactivated Id.</td><td><div lang=\"ja\">IDが無効です。</div><div lang=\"en\">The ID is Deactivated.</div></td></tr> <tr><td>F0001</td><td>Invalid format.</td><td><div lang=\"ja\">値の形式が不正です。</div><div lang=\"en\">The value format is invalid.</div></td></tr> <tr><td>110005</td><td>Not a valid id.</td><td><div lang=\"ja\">IDが有効ではありません。</div><div lang=\"en\">Not a valid id.</div></td></tr> <tr><td>L0002</td><td>Over list size.</td><td><div lang=\"ja\">配列の要素数が上限値を超過しています。</div><div lang=\"en\">The number of elements in the array exceeds the upper limit.</div></td></tr> <tr><td>D0001</td><td>Duplicate.</td><td><div lang=\"ja\">一意な値が重複しています。</div><div lang=\"en\">The unique value is duplicated.</div></td></tr> <tr><td>RL001</td><td>Invalid relation.</td><td><div lang=\"ja\">リクエストの関連性が矛盾しています。<br> たとえば、開始>終了の日付指定を行なっているなど</div><div lang=\"en\">The relation of the request is contradictory.<br> For example, you are specifying the date of start > end.</div></td></tr> <tr><td>L0001</td><td>Lower list size.</td><td><div lang=\"ja\">配列の要素数が下限値を下回っています。</div><div lang=\"en\">The number of elements in the array is below the lower limit.</div></td></tr> <tr><td>120020</td><td>Can not set value.</td><td><div lang=\"ja\">値を設定することができません。</div><div lang=\"en\">Value cannot be set.</div></td></tr> <tr><td>220014</td><td>Budget is lower than bidding price.</td><td><div lang=\"ja\">予算額が入札価格を下回っています。</div><div lang=\"en\">The bidding price has been set higher than the budget.</div></td></tr> <tr><td>LT001</td><td>Over limit.</td><td><div lang=\"ja\">登録できる上限値を超過しています。</div><div lang=\"en\">The upper limit value that can be registered is exceeded.</div></td></tr> <tr><td>220121</td><td>Frequency setting was made to the product that is not available.</td><td><div lang=\"ja\">指定された広告商品ではフリークエンシー設定できません。</div><div lang=\"en\">You can not set frequency ads instruments designated.</div></td></tr> <tr><td>220122</td><td>Unavailable combination of frequency settings.</td><td><div lang=\"ja\">フリークエンシー設定の組み合わせが不正です。</div><div lang=\"en\">Combination of frequency setting is invalid.</div></td></tr> <tr><td>220123</td><td>Unavailable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの設定が出来ない商品です。</div><div lang=\"en\">Conversion optimization setting is not possible due to the non-eligible Ad distribution.</div></td></tr> <tr><td>220124</td><td>Disable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの有効設定が不正です。</div><div lang=\"en\">Target CPA cannot be set</div></td></tr> </tbody> </table> </div>
* @param xZBaseAccountId <div lang=\"ja\">アカウントIDを指定してください。</div> <div lang=\"en\">Specify Account ID.</div> (required)
* @param campaignServiceOperation (optional)
* @return CampaignServiceMutateResponse
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public CampaignServiceMutateResponse campaignServiceRemovePost(Long xZBaseAccountId, CampaignServiceOperation campaignServiceOperation) throws RestClientException {
return campaignServiceRemovePostWithHttpInfo(xZBaseAccountId, campaignServiceOperation).getBody();
}
/**
*
* <div lang=\"ja\"> キャンペーン情報を削除します。<br> </div> <div lang=\"en\"> Deletes campaign information.<br> </div>
*
503 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0099</td><td>Out of service.</td><td><div lang=\"ja\">APIがメンテナンス中、またはご利用できません。</div><div lang=\"en\">API is under maintenance or not available to use.</div></td></tr> </tbody> </table> </div>
*
500 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0002</td><td>An internal error has occurred.</td><td><div lang=\"ja\">内部エラーが発生しました。再度操作を実行してください。 <br>もし、問題が解決しない場合は、お問い合わせページをご利用ください。</div><div lang=\"en\">An internal error has occurred. Please try again later. <br>If the problem continues, please contact the support team via Inquiry page for assistance. </div></td></tr> </tbody> </table> </div>
*
403 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0098</td><td>Permission denied.</td><td><div lang=\"ja\">権限がありません。</div><div lang=\"en\">Permission denied.</div></td></tr> <tr><td>0003</td><td>Frequency limit exceeded. Please try your request again later</td><td><div lang=\"ja\">アクセス頻度が上限値に達しました。時間をおいて再度実行してください。</div><div lang=\"en\">Frequency limit exceeded. Please try your request again later.</div></td></tr> </tbody> </table> </div>
*
401 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0110</td><td>Require access token.</td><td><div lang=\"ja\">アクセストークンがリクエストヘッダに存在していません。</div><div lang=\"en\">There is no access token in the request header.</div></td></tr> <tr><td>0111</td><td>Authentication failed.</td><td><div lang=\"ja\">アクセストークンの認証に失敗しました。</div><div lang=\"en\">The access token authentication failed.</div></td></tr> <tr><td>0112</td><td>Account not found.</td><td><div lang=\"ja\">アカウントが見つかりませんでした。</div><div lang=\"en\">The account can not found.</div></td></tr> <tr><td>0113</td><td>Deactivated account.</td><td><div lang=\"ja\">アカウントが無効です。</div><div lang=\"en\">The account is deactivated.</div></td></tr> </tbody> </table> </div>
*
400 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0114</td><td>Invalid scope.</td><td><div lang=\"ja\">アクセストークンが無効です。</div><div lang=\"en\">The access token is invalid.</div></td></tr> </tbody> </table> </div>
*
200 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0001</td><td>Invalid Request.</td><td><div lang=\"ja\">さまざまな要因が考えられます。<br>主な要因は、パラメータの値が不正か、誤りがあるためにオペレーションが完了できません。</div><div lang=\"en\">This error can result for a variety of reasons. <br>Typically because one of the parameter values in the request is wrong or invalid and the operation cannot be completed.</div></td></tr> <tr><td>R0001</td><td>Require.</td><td><div lang=\"ja\">必須です。</div><div lang=\"en\">It is missing required parameter.</div></td></tr> <tr><td>V0001</td><td>Invalid value.</td><td><div lang=\"ja\">値が無効です。</div><div lang=\"en\">The value is invalid.</div></td></tr> <tr><td>I0001</td><td>Deactivated Id.</td><td><div lang=\"ja\">IDが無効です。</div><div lang=\"en\">The ID is Deactivated.</div></td></tr> <tr><td>F0001</td><td>Invalid format.</td><td><div lang=\"ja\">値の形式が不正です。</div><div lang=\"en\">The value format is invalid.</div></td></tr> <tr><td>110005</td><td>Not a valid id.</td><td><div lang=\"ja\">IDが有効ではありません。</div><div lang=\"en\">Not a valid id.</div></td></tr> <tr><td>L0002</td><td>Over list size.</td><td><div lang=\"ja\">配列の要素数が上限値を超過しています。</div><div lang=\"en\">The number of elements in the array exceeds the upper limit.</div></td></tr> <tr><td>D0001</td><td>Duplicate.</td><td><div lang=\"ja\">一意な値が重複しています。</div><div lang=\"en\">The unique value is duplicated.</div></td></tr> <tr><td>RL001</td><td>Invalid relation.</td><td><div lang=\"ja\">リクエストの関連性が矛盾しています。<br> たとえば、開始>終了の日付指定を行なっているなど</div><div lang=\"en\">The relation of the request is contradictory.<br> For example, you are specifying the date of start > end.</div></td></tr> <tr><td>L0001</td><td>Lower list size.</td><td><div lang=\"ja\">配列の要素数が下限値を下回っています。</div><div lang=\"en\">The number of elements in the array is below the lower limit.</div></td></tr> <tr><td>120020</td><td>Can not set value.</td><td><div lang=\"ja\">値を設定することができません。</div><div lang=\"en\">Value cannot be set.</div></td></tr> <tr><td>220014</td><td>Budget is lower than bidding price.</td><td><div lang=\"ja\">予算額が入札価格を下回っています。</div><div lang=\"en\">The bidding price has been set higher than the budget.</div></td></tr> <tr><td>LT001</td><td>Over limit.</td><td><div lang=\"ja\">登録できる上限値を超過しています。</div><div lang=\"en\">The upper limit value that can be registered is exceeded.</div></td></tr> <tr><td>220121</td><td>Frequency setting was made to the product that is not available.</td><td><div lang=\"ja\">指定された広告商品ではフリークエンシー設定できません。</div><div lang=\"en\">You can not set frequency ads instruments designated.</div></td></tr> <tr><td>220122</td><td>Unavailable combination of frequency settings.</td><td><div lang=\"ja\">フリークエンシー設定の組み合わせが不正です。</div><div lang=\"en\">Combination of frequency setting is invalid.</div></td></tr> <tr><td>220123</td><td>Unavailable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの設定が出来ない商品です。</div><div lang=\"en\">Conversion optimization setting is not possible due to the non-eligible Ad distribution.</div></td></tr> <tr><td>220124</td><td>Disable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの有効設定が不正です。</div><div lang=\"en\">Target CPA cannot be set</div></td></tr> </tbody> </table> </div>
* @param xZBaseAccountId <div lang=\"ja\">アカウントIDを指定してください。</div> <div lang=\"en\">Specify Account ID.</div> (required)
* @param campaignServiceOperation (optional)
* @return ResponseEntity<CampaignServiceMutateResponse>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity campaignServiceRemovePostWithHttpInfo(Long xZBaseAccountId, CampaignServiceOperation campaignServiceOperation) throws RestClientException {
Object postBody = campaignServiceOperation;
// verify the required parameter 'xZBaseAccountId' is set
if (xZBaseAccountId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xZBaseAccountId' when calling campaignServiceRemovePost");
}
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xZBaseAccountId != null)
headerParams.add("x-z-base-account-id", apiClient.parameterToString(xZBaseAccountId));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] contentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "oAuth" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/CampaignService/remove", HttpMethod.POST, Collections.emptyMap(), queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, authNames, returnType);
}
/**
*
* <div lang=\"ja\"> キャンペーン情報を更新します。<br> </div> <div lang=\"en\"> Updates campaign information.<br> </div>
* 503 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0099</td><td>Out of service.</td><td><div lang=\"ja\">APIがメンテナンス中、またはご利用できません。</div><div lang=\"en\">API is under maintenance or not available to use.</div></td></tr> </tbody> </table> </div>
*
500 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0002</td><td>An internal error has occurred.</td><td><div lang=\"ja\">内部エラーが発生しました。再度操作を実行してください。 <br>もし、問題が解決しない場合は、お問い合わせページをご利用ください。</div><div lang=\"en\">An internal error has occurred. Please try again later. <br>If the problem continues, please contact the support team via Inquiry page for assistance. </div></td></tr> </tbody> </table> </div>
*
403 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0098</td><td>Permission denied.</td><td><div lang=\"ja\">権限がありません。</div><div lang=\"en\">Permission denied.</div></td></tr> <tr><td>0003</td><td>Frequency limit exceeded. Please try your request again later</td><td><div lang=\"ja\">アクセス頻度が上限値に達しました。時間をおいて再度実行してください。</div><div lang=\"en\">Frequency limit exceeded. Please try your request again later.</div></td></tr> </tbody> </table> </div>
*
401 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0110</td><td>Require access token.</td><td><div lang=\"ja\">アクセストークンがリクエストヘッダに存在していません。</div><div lang=\"en\">There is no access token in the request header.</div></td></tr> <tr><td>0111</td><td>Authentication failed.</td><td><div lang=\"ja\">アクセストークンの認証に失敗しました。</div><div lang=\"en\">The access token authentication failed.</div></td></tr> <tr><td>0112</td><td>Account not found.</td><td><div lang=\"ja\">アカウントが見つかりませんでした。</div><div lang=\"en\">The account can not found.</div></td></tr> <tr><td>0113</td><td>Deactivated account.</td><td><div lang=\"ja\">アカウントが無効です。</div><div lang=\"en\">The account is deactivated.</div></td></tr> </tbody> </table> </div>
*
400 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0114</td><td>Invalid scope.</td><td><div lang=\"ja\">アクセストークンが無効です。</div><div lang=\"en\">The access token is invalid.</div></td></tr> </tbody> </table> </div>
*
200 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0001</td><td>Invalid Request.</td><td><div lang=\"ja\">さまざまな要因が考えられます。<br>主な要因は、パラメータの値が不正か、誤りがあるためにオペレーションが完了できません。</div><div lang=\"en\">This error can result for a variety of reasons. <br>Typically because one of the parameter values in the request is wrong or invalid and the operation cannot be completed.</div></td></tr> <tr><td>R0001</td><td>Require.</td><td><div lang=\"ja\">必須です。</div><div lang=\"en\">It is missing required parameter.</div></td></tr> <tr><td>V0001</td><td>Invalid value.</td><td><div lang=\"ja\">値が無効です。</div><div lang=\"en\">The value is invalid.</div></td></tr> <tr><td>I0001</td><td>Deactivated Id.</td><td><div lang=\"ja\">IDが無効です。</div><div lang=\"en\">The ID is Deactivated.</div></td></tr> <tr><td>F0001</td><td>Invalid format.</td><td><div lang=\"ja\">値の形式が不正です。</div><div lang=\"en\">The value format is invalid.</div></td></tr> <tr><td>110005</td><td>Not a valid id.</td><td><div lang=\"ja\">IDが有効ではありません。</div><div lang=\"en\">Not a valid id.</div></td></tr> <tr><td>L0002</td><td>Over list size.</td><td><div lang=\"ja\">配列の要素数が上限値を超過しています。</div><div lang=\"en\">The number of elements in the array exceeds the upper limit.</div></td></tr> <tr><td>D0001</td><td>Duplicate.</td><td><div lang=\"ja\">一意な値が重複しています。</div><div lang=\"en\">The unique value is duplicated.</div></td></tr> <tr><td>RL001</td><td>Invalid relation.</td><td><div lang=\"ja\">リクエストの関連性が矛盾しています。<br> たとえば、開始>終了の日付指定を行なっているなど</div><div lang=\"en\">The relation of the request is contradictory.<br> For example, you are specifying the date of start > end.</div></td></tr> <tr><td>L0001</td><td>Lower list size.</td><td><div lang=\"ja\">配列の要素数が下限値を下回っています。</div><div lang=\"en\">The number of elements in the array is below the lower limit.</div></td></tr> <tr><td>120020</td><td>Can not set value.</td><td><div lang=\"ja\">値を設定することができません。</div><div lang=\"en\">Value cannot be set.</div></td></tr> <tr><td>220014</td><td>Budget is lower than bidding price.</td><td><div lang=\"ja\">予算額が入札価格を下回っています。</div><div lang=\"en\">The bidding price has been set higher than the budget.</div></td></tr> <tr><td>LT001</td><td>Over limit.</td><td><div lang=\"ja\">登録できる上限値を超過しています。</div><div lang=\"en\">The upper limit value that can be registered is exceeded.</div></td></tr> <tr><td>220121</td><td>Frequency setting was made to the product that is not available.</td><td><div lang=\"ja\">指定された広告商品ではフリークエンシー設定できません。</div><div lang=\"en\">You can not set frequency ads instruments designated.</div></td></tr> <tr><td>220122</td><td>Unavailable combination of frequency settings.</td><td><div lang=\"ja\">フリークエンシー設定の組み合わせが不正です。</div><div lang=\"en\">Combination of frequency setting is invalid.</div></td></tr> <tr><td>220123</td><td>Unavailable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの設定が出来ない商品です。</div><div lang=\"en\">Conversion optimization setting is not possible due to the non-eligible Ad distribution.</div></td></tr> <tr><td>220124</td><td>Disable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの有効設定が不正です。</div><div lang=\"en\">Target CPA cannot be set</div></td></tr> <tr><td>220144</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">企業の配下でSKAdNetwork計測できる広告グループは最大30件までです。</div><div lang=\"en\">The maximum number of ad groups that can be optimized for SKAdNetwork under the control of a company is 30.</div></td></tr> <tr><td>220145</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">広告アカウント配下でSKAdNetwork計測できるのは最大30件までです。</div><div lang=\"en\">The maximum number of ad groups that can be optimized for SKAdNetwork under the control of an ad account is 30.</div></td></tr> <tr><td>220147</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">SKAdNetwork計測利用時の制限により、作成および変更できません。SKAdNetwork計測利用時は、配信オンの広告グループが上限数に満たない場合でも設定変更できないケースがあります。お手数ですが数日後(最大5日間)に再試行してください。</div><div lang=\"en\">Due to the limitations of SKAdNetwork, you can't create or change the settings for ad groups. When using SKAdNetwork, you may not be able to change settings even if you don't have a maximum number of ad groups. Try again in a few days (up to 5 days).</div></td></tr> </tbody> </table> </div>
* @param xZBaseAccountId <div lang=\"ja\">アカウントIDを指定してください。</div> <div lang=\"en\">Specify Account ID.</div> (required)
* @param campaignServiceOperation (optional)
* @return CampaignServiceMutateResponse
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public CampaignServiceMutateResponse campaignServiceSetPost(Long xZBaseAccountId, CampaignServiceOperation campaignServiceOperation) throws RestClientException {
return campaignServiceSetPostWithHttpInfo(xZBaseAccountId, campaignServiceOperation).getBody();
}
/**
*
* <div lang=\"ja\"> キャンペーン情報を更新します。<br> </div> <div lang=\"en\"> Updates campaign information.<br> </div>
*
503 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0099</td><td>Out of service.</td><td><div lang=\"ja\">APIがメンテナンス中、またはご利用できません。</div><div lang=\"en\">API is under maintenance or not available to use.</div></td></tr> </tbody> </table> </div>
*
500 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0002</td><td>An internal error has occurred.</td><td><div lang=\"ja\">内部エラーが発生しました。再度操作を実行してください。 <br>もし、問題が解決しない場合は、お問い合わせページをご利用ください。</div><div lang=\"en\">An internal error has occurred. Please try again later. <br>If the problem continues, please contact the support team via Inquiry page for assistance. </div></td></tr> </tbody> </table> </div>
*
403 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0098</td><td>Permission denied.</td><td><div lang=\"ja\">権限がありません。</div><div lang=\"en\">Permission denied.</div></td></tr> <tr><td>0003</td><td>Frequency limit exceeded. Please try your request again later</td><td><div lang=\"ja\">アクセス頻度が上限値に達しました。時間をおいて再度実行してください。</div><div lang=\"en\">Frequency limit exceeded. Please try your request again later.</div></td></tr> </tbody> </table> </div>
*
401 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0110</td><td>Require access token.</td><td><div lang=\"ja\">アクセストークンがリクエストヘッダに存在していません。</div><div lang=\"en\">There is no access token in the request header.</div></td></tr> <tr><td>0111</td><td>Authentication failed.</td><td><div lang=\"ja\">アクセストークンの認証に失敗しました。</div><div lang=\"en\">The access token authentication failed.</div></td></tr> <tr><td>0112</td><td>Account not found.</td><td><div lang=\"ja\">アカウントが見つかりませんでした。</div><div lang=\"en\">The account can not found.</div></td></tr> <tr><td>0113</td><td>Deactivated account.</td><td><div lang=\"ja\">アカウントが無効です。</div><div lang=\"en\">The account is deactivated.</div></td></tr> </tbody> </table> </div>
*
400 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0114</td><td>Invalid scope.</td><td><div lang=\"ja\">アクセストークンが無効です。</div><div lang=\"en\">The access token is invalid.</div></td></tr> </tbody> </table> </div>
*
200 - <p class=\"cellContent__head\">Error codes</p> <div class=\"cellContent__body\"> <table class=\"apiTable\"> <colgroup span=\"1\" class=\"short\"></colgroup> <colgroup span=\"2\"></colgroup> <thead> <tr><th>Code</th><th>Message</th><th>Description</th></tr> </thead> <tbody> <tr><td>0001</td><td>Invalid Request.</td><td><div lang=\"ja\">さまざまな要因が考えられます。<br>主な要因は、パラメータの値が不正か、誤りがあるためにオペレーションが完了できません。</div><div lang=\"en\">This error can result for a variety of reasons. <br>Typically because one of the parameter values in the request is wrong or invalid and the operation cannot be completed.</div></td></tr> <tr><td>R0001</td><td>Require.</td><td><div lang=\"ja\">必須です。</div><div lang=\"en\">It is missing required parameter.</div></td></tr> <tr><td>V0001</td><td>Invalid value.</td><td><div lang=\"ja\">値が無効です。</div><div lang=\"en\">The value is invalid.</div></td></tr> <tr><td>I0001</td><td>Deactivated Id.</td><td><div lang=\"ja\">IDが無効です。</div><div lang=\"en\">The ID is Deactivated.</div></td></tr> <tr><td>F0001</td><td>Invalid format.</td><td><div lang=\"ja\">値の形式が不正です。</div><div lang=\"en\">The value format is invalid.</div></td></tr> <tr><td>110005</td><td>Not a valid id.</td><td><div lang=\"ja\">IDが有効ではありません。</div><div lang=\"en\">Not a valid id.</div></td></tr> <tr><td>L0002</td><td>Over list size.</td><td><div lang=\"ja\">配列の要素数が上限値を超過しています。</div><div lang=\"en\">The number of elements in the array exceeds the upper limit.</div></td></tr> <tr><td>D0001</td><td>Duplicate.</td><td><div lang=\"ja\">一意な値が重複しています。</div><div lang=\"en\">The unique value is duplicated.</div></td></tr> <tr><td>RL001</td><td>Invalid relation.</td><td><div lang=\"ja\">リクエストの関連性が矛盾しています。<br> たとえば、開始>終了の日付指定を行なっているなど</div><div lang=\"en\">The relation of the request is contradictory.<br> For example, you are specifying the date of start > end.</div></td></tr> <tr><td>L0001</td><td>Lower list size.</td><td><div lang=\"ja\">配列の要素数が下限値を下回っています。</div><div lang=\"en\">The number of elements in the array is below the lower limit.</div></td></tr> <tr><td>120020</td><td>Can not set value.</td><td><div lang=\"ja\">値を設定することができません。</div><div lang=\"en\">Value cannot be set.</div></td></tr> <tr><td>220014</td><td>Budget is lower than bidding price.</td><td><div lang=\"ja\">予算額が入札価格を下回っています。</div><div lang=\"en\">The bidding price has been set higher than the budget.</div></td></tr> <tr><td>LT001</td><td>Over limit.</td><td><div lang=\"ja\">登録できる上限値を超過しています。</div><div lang=\"en\">The upper limit value that can be registered is exceeded.</div></td></tr> <tr><td>220121</td><td>Frequency setting was made to the product that is not available.</td><td><div lang=\"ja\">指定された広告商品ではフリークエンシー設定できません。</div><div lang=\"en\">You can not set frequency ads instruments designated.</div></td></tr> <tr><td>220122</td><td>Unavailable combination of frequency settings.</td><td><div lang=\"ja\">フリークエンシー設定の組み合わせが不正です。</div><div lang=\"en\">Combination of frequency setting is invalid.</div></td></tr> <tr><td>220123</td><td>Unavailable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの設定が出来ない商品です。</div><div lang=\"en\">Conversion optimization setting is not possible due to the non-eligible Ad distribution.</div></td></tr> <tr><td>220124</td><td>Disable conversion optimizer.</td><td><div lang=\"ja\">コンバージョンオプティマイザーの有効設定が不正です。</div><div lang=\"en\">Target CPA cannot be set</div></td></tr> <tr><td>220144</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">企業の配下でSKAdNetwork計測できる広告グループは最大30件までです。</div><div lang=\"en\">The maximum number of ad groups that can be optimized for SKAdNetwork under the control of a company is 30.</div></td></tr> <tr><td>220145</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">広告アカウント配下でSKAdNetwork計測できるのは最大30件までです。</div><div lang=\"en\">The maximum number of ad groups that can be optimized for SKAdNetwork under the control of an ad account is 30.</div></td></tr> <tr><td>220147</td><td>Over limit of SKAdNetwork optimization.</td><td><div lang=\"ja\">SKAdNetwork計測利用時の制限により、作成および変更できません。SKAdNetwork計測利用時は、配信オンの広告グループが上限数に満たない場合でも設定変更できないケースがあります。お手数ですが数日後(最大5日間)に再試行してください。</div><div lang=\"en\">Due to the limitations of SKAdNetwork, you can't create or change the settings for ad groups. When using SKAdNetwork, you may not be able to change settings even if you don't have a maximum number of ad groups. Try again in a few days (up to 5 days).</div></td></tr> </tbody> </table> </div>
* @param xZBaseAccountId <div lang=\"ja\">アカウントIDを指定してください。</div> <div lang=\"en\">Specify Account ID.</div> (required)
* @param campaignServiceOperation (optional)
* @return ResponseEntity<CampaignServiceMutateResponse>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity campaignServiceSetPostWithHttpInfo(Long xZBaseAccountId, CampaignServiceOperation campaignServiceOperation) throws RestClientException {
Object postBody = campaignServiceOperation;
// verify the required parameter 'xZBaseAccountId' is set
if (xZBaseAccountId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xZBaseAccountId' when calling campaignServiceSetPost");
}
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xZBaseAccountId != null)
headerParams.add("x-z-base-account-id", apiClient.parameterToString(xZBaseAccountId));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] contentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "oAuth" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/CampaignService/set", HttpMethod.POST, Collections.emptyMap(), queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, authNames, returnType);
}
}