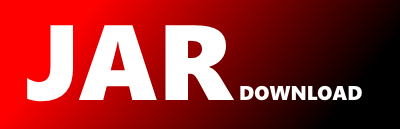
jp.co.yahoo.adsdisplayapi.v14.model.CampaignServiceViewableFrequencyCap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v14
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v14.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import jp.co.yahoo.adsdisplayapi.v14.model.CampaignServiceFrequencyLevel;
import jp.co.yahoo.adsdisplayapi.v14.model.CampaignServiceFrequencyTimeUnit;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\"> CampaignServiceViewableFrequencyCapは、ビューアブルフリークエンシー制御を表します。<br> ADDおよびSET時、このフィールドは省略可能となります。<br> REMOVE時は無視されます。<br> ※ADD時は全ての項目の指定が必須です。<br> ※SET時は更新する項目のみのリクエストが可能です。<br> ※ビューアブルフリークエンシーキャップの解除方法は、以下の通りです: </div> <div lang=\"en\"> CampaignServiceViewableFrequencyCap object describes viewable frequency restriction.<br> This field is optional in ADD and SET operation.<br> In REMOVE operation, this field will be ignored.<br> *All items must be specified in ADD operation.<br> *Only update items can be requested in SET operation.<br> *Method to remove the viewable frequency cap: </div> <code> { \"viewableFrequencyCap\": { \"vImps\": 0 } } </code>
*/
@ApiModel(description = " CampaignServiceViewableFrequencyCapは、ビューアブルフリークエンシー制御を表します。
ADDおよびSET時、このフィールドは省略可能となります。
REMOVE時は無視されます。
※ADD時は全ての項目の指定が必須です。
※SET時は更新する項目のみのリクエストが可能です。
※ビューアブルフリークエンシーキャップの解除方法は、以下の通りです: CampaignServiceViewableFrequencyCap object describes viewable frequency restriction.
This field is optional in ADD and SET operation.
In REMOVE operation, this field will be ignored.
*All items must be specified in ADD operation.
*Only update items can be requested in SET operation.
*Method to remove the viewable frequency cap: { \"viewableFrequencyCap\": { \"vImps\": 0 } }
")
@JsonPropertyOrder({
CampaignServiceViewableFrequencyCap.JSON_PROPERTY_FREQUENCY_LEVEL,
CampaignServiceViewableFrequencyCap.JSON_PROPERTY_FREQUENCY_TIME_UNIT,
CampaignServiceViewableFrequencyCap.JSON_PROPERTY_V_IMPS
})
@JsonTypeName("CampaignServiceViewableFrequencyCap")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class CampaignServiceViewableFrequencyCap {
public static final String JSON_PROPERTY_FREQUENCY_LEVEL = "frequencyLevel";
private CampaignServiceFrequencyLevel frequencyLevel;
public static final String JSON_PROPERTY_FREQUENCY_TIME_UNIT = "frequencyTimeUnit";
private CampaignServiceFrequencyTimeUnit frequencyTimeUnit;
public static final String JSON_PROPERTY_V_IMPS = "vImps";
private Long vImps;
public CampaignServiceViewableFrequencyCap() {
}
public CampaignServiceViewableFrequencyCap frequencyLevel(CampaignServiceFrequencyLevel frequencyLevel) {
this.frequencyLevel = frequencyLevel;
return this;
}
/**
* Get frequencyLevel
* @return frequencyLevel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_FREQUENCY_LEVEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CampaignServiceFrequencyLevel getFrequencyLevel() {
return frequencyLevel;
}
@JsonProperty(JSON_PROPERTY_FREQUENCY_LEVEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFrequencyLevel(CampaignServiceFrequencyLevel frequencyLevel) {
this.frequencyLevel = frequencyLevel;
}
public CampaignServiceViewableFrequencyCap frequencyTimeUnit(CampaignServiceFrequencyTimeUnit frequencyTimeUnit) {
this.frequencyTimeUnit = frequencyTimeUnit;
return this;
}
/**
* Get frequencyTimeUnit
* @return frequencyTimeUnit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_FREQUENCY_TIME_UNIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CampaignServiceFrequencyTimeUnit getFrequencyTimeUnit() {
return frequencyTimeUnit;
}
@JsonProperty(JSON_PROPERTY_FREQUENCY_TIME_UNIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFrequencyTimeUnit(CampaignServiceFrequencyTimeUnit frequencyTimeUnit) {
this.frequencyTimeUnit = frequencyTimeUnit;
}
public CampaignServiceViewableFrequencyCap vImps(Long vImps) {
this.vImps = vImps;
return this;
}
/**
* <div lang=\"ja\"> 同一ユーザに対する広告の最大ビューアブルインプレッション数です。<br> このフィールドは、ADDおよびSET時に省略可能となります。 </div> <div lang=\"en\"> Maximum number of ad viewable impressions to same user.<br> This field is optional in ADD and SET operation. </div>
* @return vImps
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 同一ユーザに対する広告の最大ビューアブルインプレッション数です。
このフィールドは、ADDおよびSET時に省略可能となります。 Maximum number of ad viewable impressions to same user.
This field is optional in ADD and SET operation. ")
@JsonProperty(JSON_PROPERTY_V_IMPS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getvImps() {
return vImps;
}
@JsonProperty(JSON_PROPERTY_V_IMPS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setvImps(Long vImps) {
this.vImps = vImps;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CampaignServiceViewableFrequencyCap campaignServiceViewableFrequencyCap = (CampaignServiceViewableFrequencyCap) o;
return Objects.equals(this.frequencyLevel, campaignServiceViewableFrequencyCap.frequencyLevel) &&
Objects.equals(this.frequencyTimeUnit, campaignServiceViewableFrequencyCap.frequencyTimeUnit) &&
Objects.equals(this.vImps, campaignServiceViewableFrequencyCap.vImps);
}
@Override
public int hashCode() {
return Objects.hash(frequencyLevel, frequencyTimeUnit, vImps);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CampaignServiceViewableFrequencyCap {\n");
sb.append(" frequencyLevel: ").append(toIndentedString(frequencyLevel)).append("\n");
sb.append(" frequencyTimeUnit: ").append(toIndentedString(frequencyTimeUnit)).append("\n");
sb.append(" vImps: ").append(toIndentedString(vImps)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy