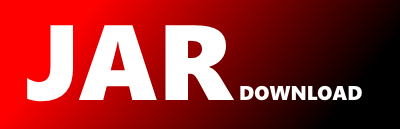
jp.co.yahoo.adsdisplayapi.v14.model.MediaServiceImageMedia Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v14
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v14.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import jp.co.yahoo.adsdisplayapi.v14.model.MediaServiceFileType;
import jp.co.yahoo.adsdisplayapi.v14.model.MediaServiceType;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\"> MediaServiceImageMediaオブジェクトは、画像を格納するコンテナです。<br> このフィールドは、SET時に必須となります。 </div> <div lang=\"en\"> The MediaServiceImageMedia object is a container for storing image. <br> This field is required in SET operation. </div>
*/
@ApiModel(description = " MediaServiceImageMediaオブジェクトは、画像を格納するコンテナです。
このフィールドは、SET時に必須となります。 The MediaServiceImageMedia object is a container for storing image.
This field is required in SET operation. ")
@JsonPropertyOrder({
MediaServiceImageMedia.JSON_PROPERTY_MEDIA_TYPE,
MediaServiceImageMedia.JSON_PROPERTY_ASPECT_RATIO,
MediaServiceImageMedia.JSON_PROPERTY_DATA,
MediaServiceImageMedia.JSON_PROPERTY_FILE_SIZE,
MediaServiceImageMedia.JSON_PROPERTY_HEIGHT,
MediaServiceImageMedia.JSON_PROPERTY_MEDIA_AD_FORMAT,
MediaServiceImageMedia.JSON_PROPERTY_MEDIA_FILE_TYPE,
MediaServiceImageMedia.JSON_PROPERTY_WIDTH,
MediaServiceImageMedia.JSON_PROPERTY_UPSCALE_IMAGE_ENABLED
})
@JsonTypeName("MediaServiceImageMedia")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class MediaServiceImageMedia {
public static final String JSON_PROPERTY_MEDIA_TYPE = "mediaType";
private MediaServiceType mediaType;
public static final String JSON_PROPERTY_ASPECT_RATIO = "aspectRatio";
private String aspectRatio;
public static final String JSON_PROPERTY_DATA = "data";
private byte[] data;
public static final String JSON_PROPERTY_FILE_SIZE = "fileSize";
private Long fileSize;
public static final String JSON_PROPERTY_HEIGHT = "height";
private Long height;
public static final String JSON_PROPERTY_MEDIA_AD_FORMAT = "mediaAdFormat";
private String mediaAdFormat;
public static final String JSON_PROPERTY_MEDIA_FILE_TYPE = "mediaFileType";
private MediaServiceFileType mediaFileType;
public static final String JSON_PROPERTY_WIDTH = "width";
private Long width;
public static final String JSON_PROPERTY_UPSCALE_IMAGE_ENABLED = "upscaleImageEnabled";
private Boolean upscaleImageEnabled;
public MediaServiceImageMedia() {
}
public MediaServiceImageMedia mediaType(MediaServiceType mediaType) {
this.mediaType = mediaType;
return this;
}
/**
* Get mediaType
* @return mediaType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_MEDIA_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MediaServiceType getMediaType() {
return mediaType;
}
@JsonProperty(JSON_PROPERTY_MEDIA_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMediaType(MediaServiceType mediaType) {
this.mediaType = mediaType;
}
public MediaServiceImageMedia aspectRatio(String aspectRatio) {
this.aspectRatio = aspectRatio;
return this;
}
/**
* <div lang=\"ja\"> 画像アスペクト比の種類です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。<br> ※指定可能な値は、DictionaryServiceのgetMediaAdFormatで取得されるDictionaryServiceMediaAdFormatのaspectRatioフィールドをご確認ください。 </div> <div lang=\"en\"> The type of aspect ratio.<br> Although this field will be returned in the response, it will be ignored on input. <br> * Available values can be referred to aspectRatio field of DictionaryServiceMediaAdFormat object obtained by getMediaAdFormat operation of DictionaryService. </div>
* @return aspectRatio
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 画像アスペクト比の種類です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。
※指定可能な値は、DictionaryServiceのgetMediaAdFormatで取得されるDictionaryServiceMediaAdFormatのaspectRatioフィールドをご確認ください。 The type of aspect ratio.
Although this field will be returned in the response, it will be ignored on input.
* Available values can be referred to aspectRatio field of DictionaryServiceMediaAdFormat object obtained by getMediaAdFormat operation of DictionaryService. ")
@JsonProperty(JSON_PROPERTY_ASPECT_RATIO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAspectRatio() {
return aspectRatio;
}
@JsonProperty(JSON_PROPERTY_ASPECT_RATIO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAspectRatio(String aspectRatio) {
this.aspectRatio = aspectRatio;
}
public MediaServiceImageMedia data(byte[] data) {
this.data = data;
return this;
}
/**
* <div lang=\"ja\">画像ファイルのbase64エンコードです。ADD時のみ指定可能で、GET時のレスポンスでは値は取得されません。</div> <div lang=\"en\">The image file in base64 encode. It can be specified on ADD, however no value returns on the response of GET.</div>
* @return data
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "画像ファイルのbase64エンコードです。ADD時のみ指定可能で、GET時のレスポンスでは値は取得されません。 The image file in base64 encode. It can be specified on ADD, however no value returns on the response of GET. ")
@JsonProperty(JSON_PROPERTY_DATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public byte[] getData() {
return data;
}
@JsonProperty(JSON_PROPERTY_DATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setData(byte[] data) {
this.data = data;
}
public MediaServiceImageMedia fileSize(Long fileSize) {
this.fileSize = fileSize;
return this;
}
/**
* <div lang=\"ja\"> ファイルサイズです。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> The file size of image. <br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return fileSize
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " ファイルサイズです。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 The file size of image.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_FILE_SIZE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getFileSize() {
return fileSize;
}
@JsonProperty(JSON_PROPERTY_FILE_SIZE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFileSize(Long fileSize) {
this.fileSize = fileSize;
}
public MediaServiceImageMedia height(Long height) {
this.height = height;
return this;
}
/**
* <div lang=\"ja\"> 縦の長さです。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> The height of image. <br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return height
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 縦の長さです。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 The height of image.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_HEIGHT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getHeight() {
return height;
}
@JsonProperty(JSON_PROPERTY_HEIGHT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHeight(Long height) {
this.height = height;
}
public MediaServiceImageMedia mediaAdFormat(String mediaAdFormat) {
this.mediaAdFormat = mediaAdFormat;
return this;
}
/**
* <div lang=\"ja\"> 画像フォーマットの種類です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。<br> ※指定可能な値は、DictionaryServiceのgetMediaAdFormatで取得されるDictionaryServiceMediaAdFormatのadFormatフィールドをご確認ください。 </div> <div lang=\"en\"> The type of image format.<br> Although this field will be returned in the response, it will be ignored on input. <br> * Available values can be referred to adFormat field of DictionaryServiceMediaAdFormat object obtained by getMediaAdFormat operation of DictionaryService. </div>
* @return mediaAdFormat
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 画像フォーマットの種類です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。
※指定可能な値は、DictionaryServiceのgetMediaAdFormatで取得されるDictionaryServiceMediaAdFormatのadFormatフィールドをご確認ください。 The type of image format.
Although this field will be returned in the response, it will be ignored on input.
* Available values can be referred to adFormat field of DictionaryServiceMediaAdFormat object obtained by getMediaAdFormat operation of DictionaryService. ")
@JsonProperty(JSON_PROPERTY_MEDIA_AD_FORMAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMediaAdFormat() {
return mediaAdFormat;
}
@JsonProperty(JSON_PROPERTY_MEDIA_AD_FORMAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMediaAdFormat(String mediaAdFormat) {
this.mediaAdFormat = mediaAdFormat;
}
public MediaServiceImageMedia mediaFileType(MediaServiceFileType mediaFileType) {
this.mediaFileType = mediaFileType;
return this;
}
/**
* Get mediaFileType
* @return mediaFileType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_MEDIA_FILE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MediaServiceFileType getMediaFileType() {
return mediaFileType;
}
@JsonProperty(JSON_PROPERTY_MEDIA_FILE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMediaFileType(MediaServiceFileType mediaFileType) {
this.mediaFileType = mediaFileType;
}
public MediaServiceImageMedia width(Long width) {
this.width = width;
return this;
}
/**
* <div lang=\"ja\"> 横幅です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> The width of image.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return width
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 横幅です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 The width of image.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_WIDTH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getWidth() {
return width;
}
@JsonProperty(JSON_PROPERTY_WIDTH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setWidth(Long width) {
this.width = width;
}
public MediaServiceImageMedia upscaleImageEnabled(Boolean upscaleImageEnabled) {
this.upscaleImageEnabled = upscaleImageEnabled;
return this;
}
/**
* <div lang=\"ja\"> MediaServiceUpscaleImageEnabledは画像の自動アップコンバートの設定フラグを表します。<br> このフィールドは、ADD時に省略可能となります。その際、デフォルト値はtrueになります。 </div> <div lang=\"en\"> MediaServiceUpscaleImageEnabled displays the flag settings of automatic image up-conversion. <br> This field is optional in ADD operation. The default value will be true. </div>
* @return upscaleImageEnabled
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " MediaServiceUpscaleImageEnabledは画像の自動アップコンバートの設定フラグを表します。
このフィールドは、ADD時に省略可能となります。その際、デフォルト値はtrueになります。 MediaServiceUpscaleImageEnabled displays the flag settings of automatic image up-conversion.
This field is optional in ADD operation. The default value will be true. ")
@JsonProperty(JSON_PROPERTY_UPSCALE_IMAGE_ENABLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getUpscaleImageEnabled() {
return upscaleImageEnabled;
}
@JsonProperty(JSON_PROPERTY_UPSCALE_IMAGE_ENABLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUpscaleImageEnabled(Boolean upscaleImageEnabled) {
this.upscaleImageEnabled = upscaleImageEnabled;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MediaServiceImageMedia mediaServiceImageMedia = (MediaServiceImageMedia) o;
return Objects.equals(this.mediaType, mediaServiceImageMedia.mediaType) &&
Objects.equals(this.aspectRatio, mediaServiceImageMedia.aspectRatio) &&
Arrays.equals(this.data, mediaServiceImageMedia.data) &&
Objects.equals(this.fileSize, mediaServiceImageMedia.fileSize) &&
Objects.equals(this.height, mediaServiceImageMedia.height) &&
Objects.equals(this.mediaAdFormat, mediaServiceImageMedia.mediaAdFormat) &&
Objects.equals(this.mediaFileType, mediaServiceImageMedia.mediaFileType) &&
Objects.equals(this.width, mediaServiceImageMedia.width) &&
Objects.equals(this.upscaleImageEnabled, mediaServiceImageMedia.upscaleImageEnabled);
}
@Override
public int hashCode() {
return Objects.hash(mediaType, aspectRatio, Arrays.hashCode(data), fileSize, height, mediaAdFormat, mediaFileType, width, upscaleImageEnabled);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MediaServiceImageMedia {\n");
sb.append(" mediaType: ").append(toIndentedString(mediaType)).append("\n");
sb.append(" aspectRatio: ").append(toIndentedString(aspectRatio)).append("\n");
sb.append(" data: ").append(toIndentedString(data)).append("\n");
sb.append(" fileSize: ").append(toIndentedString(fileSize)).append("\n");
sb.append(" height: ").append(toIndentedString(height)).append("\n");
sb.append(" mediaAdFormat: ").append(toIndentedString(mediaAdFormat)).append("\n");
sb.append(" mediaFileType: ").append(toIndentedString(mediaFileType)).append("\n");
sb.append(" width: ").append(toIndentedString(width)).append("\n");
sb.append(" upscaleImageEnabled: ").append(toIndentedString(upscaleImageEnabled)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy