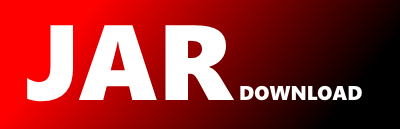
jp.co.yahoo.adsdisplayapi.v14.model.PlacementUrlIdea Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v14
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v14.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\">PlacementUrlIdeaオブジェクトは、プレイスメントUrl情報を保持するオブジェクトです。</div> <div lang=\"en\">An object that holds the placement Url information.</div>
*/
@ApiModel(description = "PlacementUrlIdeaオブジェクトは、プレイスメントUrl情報を保持するオブジェクトです。 An object that holds the placement Url information. ")
@JsonPropertyOrder({
PlacementUrlIdea.JSON_PROPERTY_KEYWORD,
PlacementUrlIdea.JSON_PROPERTY_SITE_CATEGORY,
PlacementUrlIdea.JSON_PROPERTY_SEARCH_URL,
PlacementUrlIdea.JSON_PROPERTY_DESKTOP_REACHES,
PlacementUrlIdea.JSON_PROPERTY_DESKTOP_AD_REQUESTS,
PlacementUrlIdea.JSON_PROPERTY_SMART_PHONE_REACHES,
PlacementUrlIdea.JSON_PROPERTY_SMART_PHONE_AD_REQUESTS,
PlacementUrlIdea.JSON_PROPERTY_TABLET_REACHES,
PlacementUrlIdea.JSON_PROPERTY_TABLET_AD_REQUESTS
})
@JsonTypeName("PlacementUrlIdea")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PlacementUrlIdea {
public static final String JSON_PROPERTY_KEYWORD = "keyword";
private String keyword;
public static final String JSON_PROPERTY_SITE_CATEGORY = "siteCategory";
private List siteCategory = null;
public static final String JSON_PROPERTY_SEARCH_URL = "searchUrl";
private String searchUrl;
public static final String JSON_PROPERTY_DESKTOP_REACHES = "desktopReaches";
private Long desktopReaches;
public static final String JSON_PROPERTY_DESKTOP_AD_REQUESTS = "desktopAdRequests";
private Long desktopAdRequests;
public static final String JSON_PROPERTY_SMART_PHONE_REACHES = "smartPhoneReaches";
private Long smartPhoneReaches;
public static final String JSON_PROPERTY_SMART_PHONE_AD_REQUESTS = "smartPhoneAdRequests";
private Long smartPhoneAdRequests;
public static final String JSON_PROPERTY_TABLET_REACHES = "tabletReaches";
private Long tabletReaches;
public static final String JSON_PROPERTY_TABLET_AD_REQUESTS = "tabletAdRequests";
private Long tabletAdRequests;
public PlacementUrlIdea() {
}
public PlacementUrlIdea keyword(String keyword) {
this.keyword = keyword;
return this;
}
/**
* <div lang=\"ja\">検索キーワードです。</div> <div lang=\"en\">Search keyword</div>
* @return keyword
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "検索キーワードです。 Search keyword ")
@JsonProperty(JSON_PROPERTY_KEYWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getKeyword() {
return keyword;
}
@JsonProperty(JSON_PROPERTY_KEYWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setKeyword(String keyword) {
this.keyword = keyword;
}
public PlacementUrlIdea siteCategory(List siteCategory) {
this.siteCategory = siteCategory;
return this;
}
public PlacementUrlIdea addSiteCategoryItem(String siteCategoryItem) {
if (this.siteCategory == null) {
this.siteCategory = new ArrayList<>();
}
this.siteCategory.add(siteCategoryItem);
return this;
}
/**
* <div lang=\"ja\">検索カテゴリです。</div> <div lang=\"en\">Search category</div>
* @return siteCategory
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "検索カテゴリです。 Search category ")
@JsonProperty(JSON_PROPERTY_SITE_CATEGORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSiteCategory() {
return siteCategory;
}
@JsonProperty(JSON_PROPERTY_SITE_CATEGORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSiteCategory(List siteCategory) {
this.siteCategory = siteCategory;
}
public PlacementUrlIdea searchUrl(String searchUrl) {
this.searchUrl = searchUrl;
return this;
}
/**
* <div lang=\"ja\"> 取得URLです。<br> 不明なURLの場合 UNKNOWN_URLが入ります。 </div> <div lang=\"en\">URL</div>
* @return searchUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 取得URLです。
不明なURLの場合 UNKNOWN_URLが入ります。 URL ")
@JsonProperty(JSON_PROPERTY_SEARCH_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSearchUrl() {
return searchUrl;
}
@JsonProperty(JSON_PROPERTY_SEARCH_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSearchUrl(String searchUrl) {
this.searchUrl = searchUrl;
}
public PlacementUrlIdea desktopReaches(Long desktopReaches) {
this.desktopReaches = desktopReaches;
return this;
}
/**
* <div lang=\"ja\"> PC でのリーチ数です。<br> ・1000未満の時は0が取得されます。 </div> <div lang=\"en\">Reach number of PC(1000 in the case of 0)</div>
* @return desktopReaches
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " PC でのリーチ数です。
・1000未満の時は0が取得されます。 Reach number of PC(1000 in the case of 0) ")
@JsonProperty(JSON_PROPERTY_DESKTOP_REACHES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getDesktopReaches() {
return desktopReaches;
}
@JsonProperty(JSON_PROPERTY_DESKTOP_REACHES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDesktopReaches(Long desktopReaches) {
this.desktopReaches = desktopReaches;
}
public PlacementUrlIdea desktopAdRequests(Long desktopAdRequests) {
this.desktopAdRequests = desktopAdRequests;
return this;
}
/**
* <div lang=\"ja\"> PC でのADリクエスト数です。<br> ・1000未満の時は0が取得されます。 </div> <div lang=\"en\">AD number of PC(1000 in the case of 0)</div>
* @return desktopAdRequests
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " PC でのADリクエスト数です。
・1000未満の時は0が取得されます。 AD number of PC(1000 in the case of 0) ")
@JsonProperty(JSON_PROPERTY_DESKTOP_AD_REQUESTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getDesktopAdRequests() {
return desktopAdRequests;
}
@JsonProperty(JSON_PROPERTY_DESKTOP_AD_REQUESTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDesktopAdRequests(Long desktopAdRequests) {
this.desktopAdRequests = desktopAdRequests;
}
public PlacementUrlIdea smartPhoneReaches(Long smartPhoneReaches) {
this.smartPhoneReaches = smartPhoneReaches;
return this;
}
/**
* <div lang=\"ja\"> SmartPhone でのリーチ数です。<br> ・1000未満の時は0が取得されます。 </div> <div lang=\"en\">Reach number of SmartPhone (1000 in the case of 0)</div>
* @return smartPhoneReaches
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " SmartPhone でのリーチ数です。
・1000未満の時は0が取得されます。 Reach number of SmartPhone (1000 in the case of 0) ")
@JsonProperty(JSON_PROPERTY_SMART_PHONE_REACHES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getSmartPhoneReaches() {
return smartPhoneReaches;
}
@JsonProperty(JSON_PROPERTY_SMART_PHONE_REACHES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSmartPhoneReaches(Long smartPhoneReaches) {
this.smartPhoneReaches = smartPhoneReaches;
}
public PlacementUrlIdea smartPhoneAdRequests(Long smartPhoneAdRequests) {
this.smartPhoneAdRequests = smartPhoneAdRequests;
return this;
}
/**
* <div lang=\"ja\"> SmartPhone でのADリクエスト数です。<br> ・1000未満の時は0が取得されます。 </div> <div lang=\"en\">AD number of SmartPhone(1000 in the case of 0)</div>
* @return smartPhoneAdRequests
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " SmartPhone でのADリクエスト数です。
・1000未満の時は0が取得されます。 AD number of SmartPhone(1000 in the case of 0) ")
@JsonProperty(JSON_PROPERTY_SMART_PHONE_AD_REQUESTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getSmartPhoneAdRequests() {
return smartPhoneAdRequests;
}
@JsonProperty(JSON_PROPERTY_SMART_PHONE_AD_REQUESTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSmartPhoneAdRequests(Long smartPhoneAdRequests) {
this.smartPhoneAdRequests = smartPhoneAdRequests;
}
public PlacementUrlIdea tabletReaches(Long tabletReaches) {
this.tabletReaches = tabletReaches;
return this;
}
/**
* <div lang=\"ja\"> Tablet でのリーチ数です。<br> ・1000未満の時は0が取得されます。 </div> <div lang=\"en\">Tablet(1000 in the case of 0)</div>
* @return tabletReaches
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " Tablet でのリーチ数です。
・1000未満の時は0が取得されます。 Tablet(1000 in the case of 0) ")
@JsonProperty(JSON_PROPERTY_TABLET_REACHES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getTabletReaches() {
return tabletReaches;
}
@JsonProperty(JSON_PROPERTY_TABLET_REACHES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTabletReaches(Long tabletReaches) {
this.tabletReaches = tabletReaches;
}
public PlacementUrlIdea tabletAdRequests(Long tabletAdRequests) {
this.tabletAdRequests = tabletAdRequests;
return this;
}
/**
* <div lang=\"ja\"> TabletでのADリクエスト数です。<br> ・1000未満の時は0が取得されます。 </div> <div lang=\"en\">AD number of Tablet (1000 in the case of 0)</div>
* @return tabletAdRequests
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " TabletでのADリクエスト数です。
・1000未満の時は0が取得されます。 AD number of Tablet (1000 in the case of 0) ")
@JsonProperty(JSON_PROPERTY_TABLET_AD_REQUESTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getTabletAdRequests() {
return tabletAdRequests;
}
@JsonProperty(JSON_PROPERTY_TABLET_AD_REQUESTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTabletAdRequests(Long tabletAdRequests) {
this.tabletAdRequests = tabletAdRequests;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PlacementUrlIdea placementUrlIdea = (PlacementUrlIdea) o;
return Objects.equals(this.keyword, placementUrlIdea.keyword) &&
Objects.equals(this.siteCategory, placementUrlIdea.siteCategory) &&
Objects.equals(this.searchUrl, placementUrlIdea.searchUrl) &&
Objects.equals(this.desktopReaches, placementUrlIdea.desktopReaches) &&
Objects.equals(this.desktopAdRequests, placementUrlIdea.desktopAdRequests) &&
Objects.equals(this.smartPhoneReaches, placementUrlIdea.smartPhoneReaches) &&
Objects.equals(this.smartPhoneAdRequests, placementUrlIdea.smartPhoneAdRequests) &&
Objects.equals(this.tabletReaches, placementUrlIdea.tabletReaches) &&
Objects.equals(this.tabletAdRequests, placementUrlIdea.tabletAdRequests);
}
@Override
public int hashCode() {
return Objects.hash(keyword, siteCategory, searchUrl, desktopReaches, desktopAdRequests, smartPhoneReaches, smartPhoneAdRequests, tabletReaches, tabletAdRequests);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PlacementUrlIdea {\n");
sb.append(" keyword: ").append(toIndentedString(keyword)).append("\n");
sb.append(" siteCategory: ").append(toIndentedString(siteCategory)).append("\n");
sb.append(" searchUrl: ").append(toIndentedString(searchUrl)).append("\n");
sb.append(" desktopReaches: ").append(toIndentedString(desktopReaches)).append("\n");
sb.append(" desktopAdRequests: ").append(toIndentedString(desktopAdRequests)).append("\n");
sb.append(" smartPhoneReaches: ").append(toIndentedString(smartPhoneReaches)).append("\n");
sb.append(" smartPhoneAdRequests: ").append(toIndentedString(smartPhoneAdRequests)).append("\n");
sb.append(" tabletReaches: ").append(toIndentedString(tabletReaches)).append("\n");
sb.append(" tabletAdRequests: ").append(toIndentedString(tabletAdRequests)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy