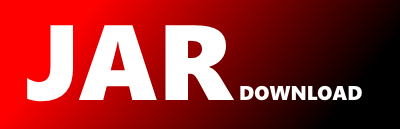
jp.co.yahoo.adsdisplayapi.v15.model.AdGroupAdServiceCarouselAd Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v15
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v15.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import jp.co.yahoo.adsdisplayapi.v15.model.AdGroupAdServiceButtonText;
import jp.co.yahoo.adsdisplayapi.v15.model.AdGroupAdServiceCarousel;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\"> AdGroupAdServiceCarouselAdオブジェクトはカルーセル広告の情報を表します。<br> このフィールドは、省略可能となります。<br> ※ADD時、adTypeがCAROUSEL_ADの場合は必須です。 </div> <div lang=\"en\"> AdGroupAdServiceCarouselAd object describes information of carousel ad.<br> This field is optional.<br> *If adType is CAROUSEL_AD, this field is required in ADD operation. </div>
*/
@ApiModel(description = " AdGroupAdServiceCarouselAdオブジェクトはカルーセル広告の情報を表します。
このフィールドは、省略可能となります。
※ADD時、adTypeがCAROUSEL_ADの場合は必須です。 AdGroupAdServiceCarouselAd object describes information of carousel ad.
This field is optional.
*If adType is CAROUSEL_AD, this field is required in ADD operation. ")
@JsonPropertyOrder({
AdGroupAdServiceCarouselAd.JSON_PROPERTY_BUTTON_TEXT,
AdGroupAdServiceCarouselAd.JSON_PROPERTY_LOGO_MEDIA_ID,
AdGroupAdServiceCarouselAd.JSON_PROPERTY_PRINCIPAL,
AdGroupAdServiceCarouselAd.JSON_PROPERTY_CAROUSELS
})
@JsonTypeName("AdGroupAdServiceCarouselAd")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class AdGroupAdServiceCarouselAd {
public static final String JSON_PROPERTY_BUTTON_TEXT = "buttonText";
private AdGroupAdServiceButtonText buttonText;
public static final String JSON_PROPERTY_LOGO_MEDIA_ID = "logoMediaId";
private Long logoMediaId;
public static final String JSON_PROPERTY_PRINCIPAL = "principal";
private String principal;
public static final String JSON_PROPERTY_CAROUSELS = "carousels";
private List carousels = null;
public AdGroupAdServiceCarouselAd() {
}
public AdGroupAdServiceCarouselAd buttonText(AdGroupAdServiceButtonText buttonText) {
this.buttonText = buttonText;
return this;
}
/**
* Get buttonText
* @return buttonText
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_BUTTON_TEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AdGroupAdServiceButtonText getButtonText() {
return buttonText;
}
@JsonProperty(JSON_PROPERTY_BUTTON_TEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setButtonText(AdGroupAdServiceButtonText buttonText) {
this.buttonText = buttonText;
}
public AdGroupAdServiceCarouselAd logoMediaId(Long logoMediaId) {
this.logoMediaId = logoMediaId;
return this;
}
/**
* <div lang=\"ja\"> ロゴの画像IDです。<br> このフィールドは、ADD時は必須となり、SET時は省略可能となります。<br> </div> <div lang=\"en\"> Logo image ID.<br> This field is required in ADD operation, and will be optional in SET operation.<br> </div>
* @return logoMediaId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " ロゴの画像IDです。
このフィールドは、ADD時は必須となり、SET時は省略可能となります。
Logo image ID.
This field is required in ADD operation, and will be optional in SET operation.
")
@JsonProperty(JSON_PROPERTY_LOGO_MEDIA_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getLogoMediaId() {
return logoMediaId;
}
@JsonProperty(JSON_PROPERTY_LOGO_MEDIA_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLogoMediaId(Long logoMediaId) {
this.logoMediaId = logoMediaId;
}
public AdGroupAdServiceCarouselAd principal(String principal) {
this.principal = principal;
return this;
}
/**
* <div lang=\"ja\"> 広告の主体者表記です。<br> このフィールドは、ADD時は必須となり、SET時は省略可能となります。<br> ただし、キャンペーン目的「アプリ訴求」の場合は、キャンペーンのアプリ名と同一の値が自動的に設定され、アプリ名以外の値は設定できません。 </div> <div lang=\"en\"> Advertiser indication of the ad.<br> This field is required in ADD operation, and will be optional in SET operation.<br> However, if campaignGoal is APP_PROMOTION, it will automatically be set to the same value as appName of campaign, and it cannot be set to any other value. </div>
* @return principal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 広告の主体者表記です。
このフィールドは、ADD時は必須となり、SET時は省略可能となります。
ただし、キャンペーン目的「アプリ訴求」の場合は、キャンペーンのアプリ名と同一の値が自動的に設定され、アプリ名以外の値は設定できません。 Advertiser indication of the ad.
This field is required in ADD operation, and will be optional in SET operation.
However, if campaignGoal is APP_PROMOTION, it will automatically be set to the same value as appName of campaign, and it cannot be set to any other value. ")
@JsonProperty(JSON_PROPERTY_PRINCIPAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPrincipal() {
return principal;
}
@JsonProperty(JSON_PROPERTY_PRINCIPAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPrincipal(String principal) {
this.principal = principal;
}
public AdGroupAdServiceCarouselAd carousels(List carousels) {
this.carousels = carousels;
return this;
}
public AdGroupAdServiceCarouselAd addCarouselsItem(AdGroupAdServiceCarousel carouselsItem) {
if (this.carousels == null) {
this.carousels = new ArrayList<>();
}
this.carousels.add(carouselsItem);
return this;
}
/**
* Get carousels
* @return carousels
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CAROUSELS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCarousels() {
return carousels;
}
@JsonProperty(JSON_PROPERTY_CAROUSELS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCarousels(List carousels) {
this.carousels = carousels;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AdGroupAdServiceCarouselAd adGroupAdServiceCarouselAd = (AdGroupAdServiceCarouselAd) o;
return Objects.equals(this.buttonText, adGroupAdServiceCarouselAd.buttonText) &&
Objects.equals(this.logoMediaId, adGroupAdServiceCarouselAd.logoMediaId) &&
Objects.equals(this.principal, adGroupAdServiceCarouselAd.principal) &&
Objects.equals(this.carousels, adGroupAdServiceCarouselAd.carousels);
}
@Override
public int hashCode() {
return Objects.hash(buttonText, logoMediaId, principal, carousels);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AdGroupAdServiceCarouselAd {\n");
sb.append(" buttonText: ").append(toIndentedString(buttonText)).append("\n");
sb.append(" logoMediaId: ").append(toIndentedString(logoMediaId)).append("\n");
sb.append(" principal: ").append(toIndentedString(principal)).append("\n");
sb.append(" carousels: ").append(toIndentedString(carousels)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy