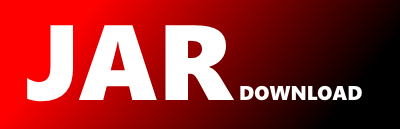
jp.co.yahoo.adsdisplayapi.v15.model.AudienceList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v15
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v15.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import jp.co.yahoo.adsdisplayapi.v15.model.AudienceListServiceContent;
import jp.co.yahoo.adsdisplayapi.v15.model.AudienceListServiceDataConnectionStopped;
import jp.co.yahoo.adsdisplayapi.v15.model.AudienceListServiceDeliveryStatus;
import jp.co.yahoo.adsdisplayapi.v15.model.AudienceListServiceIsRemoveFlg;
import jp.co.yahoo.adsdisplayapi.v15.model.AudienceListServiceSourceType;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\">AudienceListオブジェクトは、オーディエンスリストの情報を表します。</div> <div lang=\"en\">AudienceList object describes information of audience list.</div>
*/
@ApiModel(description = "AudienceListオブジェクトは、オーディエンスリストの情報を表します。 AudienceList object describes information of audience list. ")
@JsonPropertyOrder({
AudienceList.JSON_PROPERTY_ACCOUNT_ID,
AudienceList.JSON_PROPERTY_DELIVERY_STATUS,
AudienceList.JSON_PROPERTY_DESCRIPTION,
AudienceList.JSON_PROPERTY_IS_REMOVE_DESCRIPTION,
AudienceList.JSON_PROPERTY_REACH,
AudienceList.JSON_PROPERTY_AUDIENCE_LIST_CONTENT,
AudienceList.JSON_PROPERTY_AUDIENCE_LIST_ID,
AudienceList.JSON_PROPERTY_AUDIENCE_LIST_NAME,
AudienceList.JSON_PROPERTY_SOURCE_ACCOUNT_ID,
AudienceList.JSON_PROPERTY_SOURCE_ACCOUNT_NAME,
AudienceList.JSON_PROPERTY_SOURCE_TYPE,
AudienceList.JSON_PROPERTY_IS_SHARED,
AudienceList.JSON_PROPERTY_DATA_CONNECTION_STOPPED
})
@JsonTypeName("AudienceList")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class AudienceList {
public static final String JSON_PROPERTY_ACCOUNT_ID = "accountId";
private Long accountId;
public static final String JSON_PROPERTY_DELIVERY_STATUS = "deliveryStatus";
private AudienceListServiceDeliveryStatus deliveryStatus;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_IS_REMOVE_DESCRIPTION = "isRemoveDescription";
private AudienceListServiceIsRemoveFlg isRemoveDescription;
public static final String JSON_PROPERTY_REACH = "reach";
private Long reach;
public static final String JSON_PROPERTY_AUDIENCE_LIST_CONTENT = "audienceListContent";
private AudienceListServiceContent audienceListContent;
public static final String JSON_PROPERTY_AUDIENCE_LIST_ID = "audienceListId";
private Long audienceListId;
public static final String JSON_PROPERTY_AUDIENCE_LIST_NAME = "audienceListName";
private String audienceListName;
public static final String JSON_PROPERTY_SOURCE_ACCOUNT_ID = "sourceAccountId";
private Long sourceAccountId;
public static final String JSON_PROPERTY_SOURCE_ACCOUNT_NAME = "sourceAccountName";
private String sourceAccountName;
public static final String JSON_PROPERTY_SOURCE_TYPE = "sourceType";
private AudienceListServiceSourceType sourceType;
public static final String JSON_PROPERTY_IS_SHARED = "isShared";
private Boolean isShared;
public static final String JSON_PROPERTY_DATA_CONNECTION_STOPPED = "dataConnectionStopped";
private AudienceListServiceDataConnectionStopped dataConnectionStopped;
public AudienceList() {
}
public AudienceList accountId(Long accountId) {
this.accountId = accountId;
return this;
}
/**
* <div lang=\"ja\"> アカウントIDです。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> Account ID.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " アカウントIDです。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 Account ID.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getAccountId() {
return accountId;
}
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccountId(Long accountId) {
this.accountId = accountId;
}
public AudienceList deliveryStatus(AudienceListServiceDeliveryStatus deliveryStatus) {
this.deliveryStatus = deliveryStatus;
return this;
}
/**
* Get deliveryStatus
* @return deliveryStatus
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DELIVERY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AudienceListServiceDeliveryStatus getDeliveryStatus() {
return deliveryStatus;
}
@JsonProperty(JSON_PROPERTY_DELIVERY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeliveryStatus(AudienceListServiceDeliveryStatus deliveryStatus) {
this.deliveryStatus = deliveryStatus;
}
public AudienceList description(String description) {
this.description = description;
return this;
}
/**
* <div lang=\"ja\"> オーディエンスリストの説明です。<br> このフィールドは、ADDおよびSET時に省略可能となり、REMOVE時に無視されます。 </div> <div lang=\"en\"> Description of audience list.<br> This field is optional in ADD and SET operation, and will be ignored in REMOVE operation. </div>
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " オーディエンスリストの説明です。
このフィールドは、ADDおよびSET時に省略可能となり、REMOVE時に無視されます。 Description of audience list.
This field is optional in ADD and SET operation, and will be ignored in REMOVE operation. ")
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public AudienceList isRemoveDescription(AudienceListServiceIsRemoveFlg isRemoveDescription) {
this.isRemoveDescription = isRemoveDescription;
return this;
}
/**
* Get isRemoveDescription
* @return isRemoveDescription
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_IS_REMOVE_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AudienceListServiceIsRemoveFlg getIsRemoveDescription() {
return isRemoveDescription;
}
@JsonProperty(JSON_PROPERTY_IS_REMOVE_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsRemoveDescription(AudienceListServiceIsRemoveFlg isRemoveDescription) {
this.isRemoveDescription = isRemoveDescription;
}
public AudienceList reach(Long reach) {
this.reach = reach;
return this;
}
/**
* <div lang=\"ja\"> リーチ数です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> Number of reaches.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return reach
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " リーチ数です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 Number of reaches.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_REACH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getReach() {
return reach;
}
@JsonProperty(JSON_PROPERTY_REACH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReach(Long reach) {
this.reach = reach;
}
public AudienceList audienceListContent(AudienceListServiceContent audienceListContent) {
this.audienceListContent = audienceListContent;
return this;
}
/**
* Get audienceListContent
* @return audienceListContent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_CONTENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AudienceListServiceContent getAudienceListContent() {
return audienceListContent;
}
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_CONTENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAudienceListContent(AudienceListServiceContent audienceListContent) {
this.audienceListContent = audienceListContent;
}
public AudienceList audienceListId(Long audienceListId) {
this.audienceListId = audienceListId;
return this;
}
/**
* <div lang=\"ja\"> オーディエンスリストIDです。<br> このフィールドは、SETおよびREMOVE時に必須となり、ADD時には無視されます。 </div> <div lang=\"en\"> Audience list ID.<br> This field is required in SET and REMOVE operation, and will be ignored in ADD operation. </div>
* @return audienceListId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " オーディエンスリストIDです。
このフィールドは、SETおよびREMOVE時に必須となり、ADD時には無視されます。 Audience list ID.
This field is required in SET and REMOVE operation, and will be ignored in ADD operation. ")
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getAudienceListId() {
return audienceListId;
}
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAudienceListId(Long audienceListId) {
this.audienceListId = audienceListId;
}
public AudienceList audienceListName(String audienceListName) {
this.audienceListName = audienceListName;
return this;
}
/**
* <div lang=\"ja\"> オーディエンスリスト名です。<br> このフィールドは、ADD時は必須、SET時は省略可能となり、REMOVE時は無視されます。 </div> <div lang=\"en\"> Audience list name.<br> This field is required in ADD operation, optional in SET operation, and will be ignored in REMOVE operation. </div>
* @return audienceListName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " オーディエンスリスト名です。
このフィールドは、ADD時は必須、SET時は省略可能となり、REMOVE時は無視されます。 Audience list name.
This field is required in ADD operation, optional in SET operation, and will be ignored in REMOVE operation. ")
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAudienceListName() {
return audienceListName;
}
@JsonProperty(JSON_PROPERTY_AUDIENCE_LIST_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAudienceListName(String audienceListName) {
this.audienceListName = audienceListName;
}
public AudienceList sourceAccountId(Long sourceAccountId) {
this.sourceAccountId = sourceAccountId;
return this;
}
/**
* <div lang=\"ja\"> 共有元アカウントIDです。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> Account ID of the sharing source.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return sourceAccountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 共有元アカウントIDです。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 Account ID of the sharing source.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_SOURCE_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getSourceAccountId() {
return sourceAccountId;
}
@JsonProperty(JSON_PROPERTY_SOURCE_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSourceAccountId(Long sourceAccountId) {
this.sourceAccountId = sourceAccountId;
}
public AudienceList sourceAccountName(String sourceAccountName) {
this.sourceAccountName = sourceAccountName;
return this;
}
/**
* <div lang=\"ja\"> 共有元アカウント名です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> Account name of the sharing source.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return sourceAccountName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 共有元アカウント名です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 Account name of the sharing source.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_SOURCE_ACCOUNT_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSourceAccountName() {
return sourceAccountName;
}
@JsonProperty(JSON_PROPERTY_SOURCE_ACCOUNT_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSourceAccountName(String sourceAccountName) {
this.sourceAccountName = sourceAccountName;
}
public AudienceList sourceType(AudienceListServiceSourceType sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* Get sourceType
* @return sourceType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SOURCE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AudienceListServiceSourceType getSourceType() {
return sourceType;
}
@JsonProperty(JSON_PROPERTY_SOURCE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSourceType(AudienceListServiceSourceType sourceType) {
this.sourceType = sourceType;
}
public AudienceList isShared(Boolean isShared) {
this.isShared = isShared;
return this;
}
/**
* <div lang=\"ja\"> AudienceListServiceIsSharedは、オーディエンスリストの共有ステータスを示します。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> AudienceListServiceIsShared describes the sharing status of the audience list.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return isShared
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " AudienceListServiceIsSharedは、オーディエンスリストの共有ステータスを示します。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 AudienceListServiceIsShared describes the sharing status of the audience list.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_IS_SHARED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsShared() {
return isShared;
}
@JsonProperty(JSON_PROPERTY_IS_SHARED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsShared(Boolean isShared) {
this.isShared = isShared;
}
public AudienceList dataConnectionStopped(AudienceListServiceDataConnectionStopped dataConnectionStopped) {
this.dataConnectionStopped = dataConnectionStopped;
return this;
}
/**
* Get dataConnectionStopped
* @return dataConnectionStopped
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DATA_CONNECTION_STOPPED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AudienceListServiceDataConnectionStopped getDataConnectionStopped() {
return dataConnectionStopped;
}
@JsonProperty(JSON_PROPERTY_DATA_CONNECTION_STOPPED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataConnectionStopped(AudienceListServiceDataConnectionStopped dataConnectionStopped) {
this.dataConnectionStopped = dataConnectionStopped;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AudienceList audienceList = (AudienceList) o;
return Objects.equals(this.accountId, audienceList.accountId) &&
Objects.equals(this.deliveryStatus, audienceList.deliveryStatus) &&
Objects.equals(this.description, audienceList.description) &&
Objects.equals(this.isRemoveDescription, audienceList.isRemoveDescription) &&
Objects.equals(this.reach, audienceList.reach) &&
Objects.equals(this.audienceListContent, audienceList.audienceListContent) &&
Objects.equals(this.audienceListId, audienceList.audienceListId) &&
Objects.equals(this.audienceListName, audienceList.audienceListName) &&
Objects.equals(this.sourceAccountId, audienceList.sourceAccountId) &&
Objects.equals(this.sourceAccountName, audienceList.sourceAccountName) &&
Objects.equals(this.sourceType, audienceList.sourceType) &&
Objects.equals(this.isShared, audienceList.isShared) &&
Objects.equals(this.dataConnectionStopped, audienceList.dataConnectionStopped);
}
@Override
public int hashCode() {
return Objects.hash(accountId, deliveryStatus, description, isRemoveDescription, reach, audienceListContent, audienceListId, audienceListName, sourceAccountId, sourceAccountName, sourceType, isShared, dataConnectionStopped);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AudienceList {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" deliveryStatus: ").append(toIndentedString(deliveryStatus)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" isRemoveDescription: ").append(toIndentedString(isRemoveDescription)).append("\n");
sb.append(" reach: ").append(toIndentedString(reach)).append("\n");
sb.append(" audienceListContent: ").append(toIndentedString(audienceListContent)).append("\n");
sb.append(" audienceListId: ").append(toIndentedString(audienceListId)).append("\n");
sb.append(" audienceListName: ").append(toIndentedString(audienceListName)).append("\n");
sb.append(" sourceAccountId: ").append(toIndentedString(sourceAccountId)).append("\n");
sb.append(" sourceAccountName: ").append(toIndentedString(sourceAccountName)).append("\n");
sb.append(" sourceType: ").append(toIndentedString(sourceType)).append("\n");
sb.append(" isShared: ").append(toIndentedString(isShared)).append("\n");
sb.append(" dataConnectionStopped: ").append(toIndentedString(dataConnectionStopped)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy