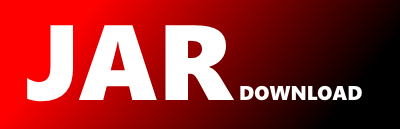
jp.co.yahoo.adsdisplayapi.v15.model.AuditLogServiceJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v15
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v15.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import jp.co.yahoo.adsdisplayapi.v15.model.AuditLogServiceDateRange;
import jp.co.yahoo.adsdisplayapi.v15.model.AuditLogServiceEncoding;
import jp.co.yahoo.adsdisplayapi.v15.model.AuditLogServiceJobStatus;
import jp.co.yahoo.adsdisplayapi.v15.model.AuditLogServiceLang;
import jp.co.yahoo.adsdisplayapi.v15.model.AuditLogServiceOutput;
import jp.co.yahoo.adsdisplayapi.v15.model.AuditLogServiceSourceType;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\">AuditLogServiceJobは操作履歴のダウンロードジョブの情報を保持するオブジェクトです。</div> <div lang=\"en\">AuditLogServiceJob object is a container for storing the download operation history job information.</div>
*/
@ApiModel(description = "AuditLogServiceJobは操作履歴のダウンロードジョブの情報を保持するオブジェクトです。 AuditLogServiceJob object is a container for storing the download operation history job information. ")
@JsonPropertyOrder({
AuditLogServiceJob.JSON_PROPERTY_ACCOUNT_ID,
AuditLogServiceJob.JSON_PROPERTY_AUDIT_LOG_JOB_END_DATE,
AuditLogServiceJob.JSON_PROPERTY_AUDIT_LOG_JOB_ID,
AuditLogServiceJob.JSON_PROPERTY_AUDIT_LOG_JOB_NAME,
AuditLogServiceJob.JSON_PROPERTY_AUDIT_LOG_JOB_START_DATE,
AuditLogServiceJob.JSON_PROPERTY_AUDIT_LOG_JOB_USER_NAME,
AuditLogServiceJob.JSON_PROPERTY_CAMPAIGN_IDS,
AuditLogServiceJob.JSON_PROPERTY_DATE_RANGE,
AuditLogServiceJob.JSON_PROPERTY_ENCODING,
AuditLogServiceJob.JSON_PROPERTY_JOB_STATUS,
AuditLogServiceJob.JSON_PROPERTY_LANG,
AuditLogServiceJob.JSON_PROPERTY_OUTPUT,
AuditLogServiceJob.JSON_PROPERTY_PROGRESS,
AuditLogServiceJob.JSON_PROPERTY_SOURCE_TYPE
})
@JsonTypeName("AuditLogServiceJob")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class AuditLogServiceJob {
public static final String JSON_PROPERTY_ACCOUNT_ID = "accountId";
private Long accountId;
public static final String JSON_PROPERTY_AUDIT_LOG_JOB_END_DATE = "auditLogJobEndDate";
private String auditLogJobEndDate;
public static final String JSON_PROPERTY_AUDIT_LOG_JOB_ID = "auditLogJobId";
private Long auditLogJobId;
public static final String JSON_PROPERTY_AUDIT_LOG_JOB_NAME = "auditLogJobName";
private String auditLogJobName;
public static final String JSON_PROPERTY_AUDIT_LOG_JOB_START_DATE = "auditLogJobStartDate";
private String auditLogJobStartDate;
public static final String JSON_PROPERTY_AUDIT_LOG_JOB_USER_NAME = "auditLogJobUserName";
private String auditLogJobUserName;
public static final String JSON_PROPERTY_CAMPAIGN_IDS = "campaignIds";
private List campaignIds = null;
public static final String JSON_PROPERTY_DATE_RANGE = "dateRange";
private AuditLogServiceDateRange dateRange;
public static final String JSON_PROPERTY_ENCODING = "encoding";
private AuditLogServiceEncoding encoding;
public static final String JSON_PROPERTY_JOB_STATUS = "jobStatus";
private AuditLogServiceJobStatus jobStatus;
public static final String JSON_PROPERTY_LANG = "lang";
private AuditLogServiceLang lang;
public static final String JSON_PROPERTY_OUTPUT = "output";
private AuditLogServiceOutput output;
public static final String JSON_PROPERTY_PROGRESS = "progress";
private Integer progress;
public static final String JSON_PROPERTY_SOURCE_TYPE = "sourceType";
private AuditLogServiceSourceType sourceType;
public AuditLogServiceJob() {
}
public AuditLogServiceJob accountId(Long accountId) {
this.accountId = accountId;
return this;
}
/**
* <div lang=\"ja\"> アカウントIDです。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> Account ID.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " アカウントIDです。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 Account ID.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getAccountId() {
return accountId;
}
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccountId(Long accountId) {
this.accountId = accountId;
}
public AuditLogServiceJob auditLogJobEndDate(String auditLogJobEndDate) {
this.auditLogJobEndDate = auditLogJobEndDate;
return this;
}
/**
* <div lang=\"ja\"> 操作履歴ダウンロードジョブの終了日時です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> The job end date (YYYY-MM-DDTHH:MI:SS+9:00).<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return auditLogJobEndDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 操作履歴ダウンロードジョブの終了日時です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 The job end date (YYYY-MM-DDTHH:MI:SS+9:00).
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_END_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAuditLogJobEndDate() {
return auditLogJobEndDate;
}
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_END_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuditLogJobEndDate(String auditLogJobEndDate) {
this.auditLogJobEndDate = auditLogJobEndDate;
}
public AuditLogServiceJob auditLogJobId(Long auditLogJobId) {
this.auditLogJobId = auditLogJobId;
return this;
}
/**
* <div lang=\"ja\"> 操作履歴のダウンロードジョブIDです。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> The operation history job ID.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return auditLogJobId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 操作履歴のダウンロードジョブIDです。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 The operation history job ID.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getAuditLogJobId() {
return auditLogJobId;
}
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuditLogJobId(Long auditLogJobId) {
this.auditLogJobId = auditLogJobId;
}
public AuditLogServiceJob auditLogJobName(String auditLogJobName) {
this.auditLogJobName = auditLogJobName;
return this;
}
/**
* <div lang=\"ja\"> 操作履歴のダウンロードジョブ名です。<br> このフィールドは、リクエストの場合は省略可能となります。 </div> <div lang=\"en\"> The operation history job name.<br> This field is optional in requests. </div>
* @return auditLogJobName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 操作履歴のダウンロードジョブ名です。
このフィールドは、リクエストの場合は省略可能となります。 The operation history job name.
This field is optional in requests. ")
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAuditLogJobName() {
return auditLogJobName;
}
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuditLogJobName(String auditLogJobName) {
this.auditLogJobName = auditLogJobName;
}
public AuditLogServiceJob auditLogJobStartDate(String auditLogJobStartDate) {
this.auditLogJobStartDate = auditLogJobStartDate;
return this;
}
/**
* <div lang=\"ja\"> 操作履歴ダウンロードジョブの開始日時です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> The job start date (YYYY-MM-DDTHH:MI:SS+9:00).<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return auditLogJobStartDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 操作履歴ダウンロードジョブの開始日時です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 The job start date (YYYY-MM-DDTHH:MI:SS+9:00).
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_START_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAuditLogJobStartDate() {
return auditLogJobStartDate;
}
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_START_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuditLogJobStartDate(String auditLogJobStartDate) {
this.auditLogJobStartDate = auditLogJobStartDate;
}
public AuditLogServiceJob auditLogJobUserName(String auditLogJobUserName) {
this.auditLogJobUserName = auditLogJobUserName;
return this;
}
/**
* <div lang=\"ja\"> 操作履歴ダウンロードジョブの実行ユーザー名です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> The user name who executes the operation history job.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return auditLogJobUserName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 操作履歴ダウンロードジョブの実行ユーザー名です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 The user name who executes the operation history job.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_USER_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAuditLogJobUserName() {
return auditLogJobUserName;
}
@JsonProperty(JSON_PROPERTY_AUDIT_LOG_JOB_USER_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuditLogJobUserName(String auditLogJobUserName) {
this.auditLogJobUserName = auditLogJobUserName;
}
public AuditLogServiceJob campaignIds(List campaignIds) {
this.campaignIds = campaignIds;
return this;
}
public AuditLogServiceJob addCampaignIdsItem(Long campaignIdsItem) {
if (this.campaignIds == null) {
this.campaignIds = new ArrayList<>();
}
this.campaignIds.add(campaignIdsItem);
return this;
}
/**
* <div lang=\"ja\"> ダウンロード対象のキャンペーンIDです。<br> このフィールドは、リクエストの場合は省略可能となります。 </div> <div lang=\"en\"> Campaign ID of the download object.<br> This field is optional in requests. </div>
* @return campaignIds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " ダウンロード対象のキャンペーンIDです。
このフィールドは、リクエストの場合は省略可能となります。 Campaign ID of the download object.
This field is optional in requests. ")
@JsonProperty(JSON_PROPERTY_CAMPAIGN_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCampaignIds() {
return campaignIds;
}
@JsonProperty(JSON_PROPERTY_CAMPAIGN_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCampaignIds(List campaignIds) {
this.campaignIds = campaignIds;
}
public AuditLogServiceJob dateRange(AuditLogServiceDateRange dateRange) {
this.dateRange = dateRange;
return this;
}
/**
* Get dateRange
* @return dateRange
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DATE_RANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AuditLogServiceDateRange getDateRange() {
return dateRange;
}
@JsonProperty(JSON_PROPERTY_DATE_RANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDateRange(AuditLogServiceDateRange dateRange) {
this.dateRange = dateRange;
}
public AuditLogServiceJob encoding(AuditLogServiceEncoding encoding) {
this.encoding = encoding;
return this;
}
/**
* Get encoding
* @return encoding
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ENCODING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AuditLogServiceEncoding getEncoding() {
return encoding;
}
@JsonProperty(JSON_PROPERTY_ENCODING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEncoding(AuditLogServiceEncoding encoding) {
this.encoding = encoding;
}
public AuditLogServiceJob jobStatus(AuditLogServiceJobStatus jobStatus) {
this.jobStatus = jobStatus;
return this;
}
/**
* Get jobStatus
* @return jobStatus
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_JOB_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AuditLogServiceJobStatus getJobStatus() {
return jobStatus;
}
@JsonProperty(JSON_PROPERTY_JOB_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setJobStatus(AuditLogServiceJobStatus jobStatus) {
this.jobStatus = jobStatus;
}
public AuditLogServiceJob lang(AuditLogServiceLang lang) {
this.lang = lang;
return this;
}
/**
* Get lang
* @return lang
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LANG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AuditLogServiceLang getLang() {
return lang;
}
@JsonProperty(JSON_PROPERTY_LANG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLang(AuditLogServiceLang lang) {
this.lang = lang;
}
public AuditLogServiceJob output(AuditLogServiceOutput output) {
this.output = output;
return this;
}
/**
* Get output
* @return output
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_OUTPUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AuditLogServiceOutput getOutput() {
return output;
}
@JsonProperty(JSON_PROPERTY_OUTPUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOutput(AuditLogServiceOutput output) {
this.output = output;
}
public AuditLogServiceJob progress(Integer progress) {
this.progress = progress;
return this;
}
/**
* <div lang=\"ja\"> 処理進捗です。<br> このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 </div> <div lang=\"en\"> Displays progress in integers from 1 to 100.<br> Although this field will be returned in the response, it will be ignored on input. </div>
* @return progress
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 処理進捗です。
このフィールドは、レスポンスの際に返却されますが、リクエストの際には無視されます。 Displays progress in integers from 1 to 100.
Although this field will be returned in the response, it will be ignored on input. ")
@JsonProperty(JSON_PROPERTY_PROGRESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getProgress() {
return progress;
}
@JsonProperty(JSON_PROPERTY_PROGRESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProgress(Integer progress) {
this.progress = progress;
}
public AuditLogServiceJob sourceType(AuditLogServiceSourceType sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* Get sourceType
* @return sourceType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SOURCE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AuditLogServiceSourceType getSourceType() {
return sourceType;
}
@JsonProperty(JSON_PROPERTY_SOURCE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSourceType(AuditLogServiceSourceType sourceType) {
this.sourceType = sourceType;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AuditLogServiceJob auditLogServiceJob = (AuditLogServiceJob) o;
return Objects.equals(this.accountId, auditLogServiceJob.accountId) &&
Objects.equals(this.auditLogJobEndDate, auditLogServiceJob.auditLogJobEndDate) &&
Objects.equals(this.auditLogJobId, auditLogServiceJob.auditLogJobId) &&
Objects.equals(this.auditLogJobName, auditLogServiceJob.auditLogJobName) &&
Objects.equals(this.auditLogJobStartDate, auditLogServiceJob.auditLogJobStartDate) &&
Objects.equals(this.auditLogJobUserName, auditLogServiceJob.auditLogJobUserName) &&
Objects.equals(this.campaignIds, auditLogServiceJob.campaignIds) &&
Objects.equals(this.dateRange, auditLogServiceJob.dateRange) &&
Objects.equals(this.encoding, auditLogServiceJob.encoding) &&
Objects.equals(this.jobStatus, auditLogServiceJob.jobStatus) &&
Objects.equals(this.lang, auditLogServiceJob.lang) &&
Objects.equals(this.output, auditLogServiceJob.output) &&
Objects.equals(this.progress, auditLogServiceJob.progress) &&
Objects.equals(this.sourceType, auditLogServiceJob.sourceType);
}
@Override
public int hashCode() {
return Objects.hash(accountId, auditLogJobEndDate, auditLogJobId, auditLogJobName, auditLogJobStartDate, auditLogJobUserName, campaignIds, dateRange, encoding, jobStatus, lang, output, progress, sourceType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AuditLogServiceJob {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" auditLogJobEndDate: ").append(toIndentedString(auditLogJobEndDate)).append("\n");
sb.append(" auditLogJobId: ").append(toIndentedString(auditLogJobId)).append("\n");
sb.append(" auditLogJobName: ").append(toIndentedString(auditLogJobName)).append("\n");
sb.append(" auditLogJobStartDate: ").append(toIndentedString(auditLogJobStartDate)).append("\n");
sb.append(" auditLogJobUserName: ").append(toIndentedString(auditLogJobUserName)).append("\n");
sb.append(" campaignIds: ").append(toIndentedString(campaignIds)).append("\n");
sb.append(" dateRange: ").append(toIndentedString(dateRange)).append("\n");
sb.append(" encoding: ").append(toIndentedString(encoding)).append("\n");
sb.append(" jobStatus: ").append(toIndentedString(jobStatus)).append("\n");
sb.append(" lang: ").append(toIndentedString(lang)).append("\n");
sb.append(" output: ").append(toIndentedString(output)).append("\n");
sb.append(" progress: ").append(toIndentedString(progress)).append("\n");
sb.append(" sourceType: ").append(toIndentedString(sourceType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy