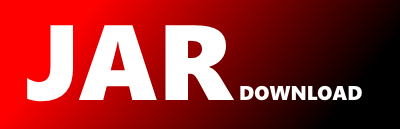
jp.co.yahoo.adsdisplayapi.v15.model.ReportDefinitionServiceCrossCampaignGoal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-display-api-lib Show documentation
Show all versions of ads-display-api-lib Show documentation
Yahoo! JAPAN Ads Display Ads API library for Java
The newest version!
/*
* Yahoo!広告 ディスプレイ広告 API リファレンス / Yahoo! JAPAN Ads Display Ads API Reference
* Yahoo!広告 ディスプレイ広告 APIのWebサービスについて説明します。 Display Ads API Web Services supported in Yahoo! JAPAN Ads API.
*
* The version of the OpenAPI document: v15
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package jp.co.yahoo.adsdisplayapi.v15.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* <div lang=\"ja\"> ReportDefinitionServiceCrossCampaignGoalは、横断リーチレポートの組み合わせの対象となるアカウントおよびキャンペーン目的を示します。<br> このフィールドは、ADD時に省略可能となり、REMOVE時に無視されます。<br> ※ADD時、crossCampaignTypeが<code>CAMPAIGN_GOAL</code>の場合は必須です。 </div> <div lang=\"en\"> ReportDefinitionServiceCrossCampaignGoal indicates account and campaign goal that is subject to Cross-campaign Reach Report combination.<br> This field is optional in ADD operation, and will be ignored in REMOVE operation.<br> *If crossCampaignType is <code>CAMPAIGN_GOAL</code>, this field is required in ADD operation. </div>
*/
@ApiModel(description = " ReportDefinitionServiceCrossCampaignGoalは、横断リーチレポートの組み合わせの対象となるアカウントおよびキャンペーン目的を示します。
このフィールドは、ADD時に省略可能となり、REMOVE時に無視されます。
※ADD時、crossCampaignTypeがCAMPAIGN_GOAL
の場合は必須です。 ReportDefinitionServiceCrossCampaignGoal indicates account and campaign goal that is subject to Cross-campaign Reach Report combination.
This field is optional in ADD operation, and will be ignored in REMOVE operation.
*If crossCampaignType is CAMPAIGN_GOAL
, this field is required in ADD operation. ")
@JsonPropertyOrder({
ReportDefinitionServiceCrossCampaignGoal.JSON_PROPERTY_CAMPAIGN_GOAL
})
@JsonTypeName("ReportDefinitionServiceCrossCampaignGoal")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class ReportDefinitionServiceCrossCampaignGoal {
public static final String JSON_PROPERTY_CAMPAIGN_GOAL = "campaignGoal";
private String campaignGoal;
public ReportDefinitionServiceCrossCampaignGoal() {
}
public ReportDefinitionServiceCrossCampaignGoal campaignGoal(String campaignGoal) {
this.campaignGoal = campaignGoal;
return this;
}
/**
* <div lang=\"ja\"> 横断リーチレポートの組み合わせの対象となるキャンペーン目的です。<br> このフィールドは、ADD時に省略可能となり、REMOVE時に無視されます。<br> ※ADD時、crossCampaignReachTypeが<code>CAMPAIGN_GOAL</code>の場合は必須です。<br> ※BRAND_AWARENESSを指定すると「運用型:ブランド認知」と「予約型:ブランド認知」の両方が対象になります。<br> ※BRAND_AWARENESS_GUARANTEEDは指定できません。<br> ※指定可能な値は、AccountAuthorityServiceのGET操作で得られるAccountAuthorityのauthoritiesフィールドをご確認ください。 </div> <div lang=\"en\"> Campaign goal that is subject to combination of Cross-campaign Reach Report. <br> This field is optional in ADD operation, and will be ignored in REMOVE operation. <br> *If crossCampaignReachType is <code>CAMPAIGN_GOAL</code>, this field is required in ADD operation.<br> *If you specify BRAND_AWARENESS, \"Auction: Brand awareness\" and \"Guaranteed: Brand awareness\" will be targeted. <br> *BRAND_AWARENESS_GUARANTEED cannot be specified.<br> *Available values can be referred to authorities field of AccountAuthority object obtained by GET operation of AccountAuthorityService. </div>
* @return campaignGoal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " 横断リーチレポートの組み合わせの対象となるキャンペーン目的です。
このフィールドは、ADD時に省略可能となり、REMOVE時に無視されます。
※ADD時、crossCampaignReachTypeがCAMPAIGN_GOAL
の場合は必須です。
※BRAND_AWARENESSを指定すると「運用型:ブランド認知」と「予約型:ブランド認知」の両方が対象になります。
※BRAND_AWARENESS_GUARANTEEDは指定できません。
※指定可能な値は、AccountAuthorityServiceのGET操作で得られるAccountAuthorityのauthoritiesフィールドをご確認ください。 Campaign goal that is subject to combination of Cross-campaign Reach Report.
This field is optional in ADD operation, and will be ignored in REMOVE operation.
*If crossCampaignReachType is CAMPAIGN_GOAL
, this field is required in ADD operation.
*If you specify BRAND_AWARENESS, \"Auction: Brand awareness\" and \"Guaranteed: Brand awareness\" will be targeted.
*BRAND_AWARENESS_GUARANTEED cannot be specified.
*Available values can be referred to authorities field of AccountAuthority object obtained by GET operation of AccountAuthorityService. ")
@JsonProperty(JSON_PROPERTY_CAMPAIGN_GOAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCampaignGoal() {
return campaignGoal;
}
@JsonProperty(JSON_PROPERTY_CAMPAIGN_GOAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCampaignGoal(String campaignGoal) {
this.campaignGoal = campaignGoal;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ReportDefinitionServiceCrossCampaignGoal reportDefinitionServiceCrossCampaignGoal = (ReportDefinitionServiceCrossCampaignGoal) o;
return Objects.equals(this.campaignGoal, reportDefinitionServiceCrossCampaignGoal.campaignGoal);
}
@Override
public int hashCode() {
return Objects.hash(campaignGoal);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ReportDefinitionServiceCrossCampaignGoal {\n");
sb.append(" campaignGoal: ").append(toIndentedString(campaignGoal)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy