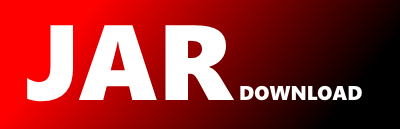
jp.gopay.sdk.builders.bankaccount.AbstractBankAccountsBuilders Maven / Gradle / Ivy
package jp.gopay.sdk.builders.bankaccount;
import jp.gopay.sdk.builders.IdempotentRetrofitRequestBuilder;
import jp.gopay.sdk.builders.RetrofitRequestBuilder;
import jp.gopay.sdk.builders.RetrofitRequestBuilderPaginated;
import jp.gopay.sdk.models.common.BankAccountId;
import jp.gopay.sdk.models.common.Void;
import jp.gopay.sdk.models.common.bankaccounts.BaseBankAccount;
import jp.gopay.sdk.models.response.bankaccount.BankAccount;
import retrofit2.Retrofit;
public abstract class AbstractBankAccountsBuilders {
public static abstract class AbstractCreateBankAccountRequestBuilder
extends IdempotentRetrofitRequestBuilder {
protected BaseBankAccount bankAccount;
public BaseBankAccount getBankAccount() {
return bankAccount;
}
public AbstractCreateBankAccountRequestBuilder(Retrofit retrofit, BaseBankAccount bankAccount) {
super(retrofit);
this.bankAccount = bankAccount;
}
}
public static abstract class AbstractDeleteBankAccountRequestBuilder
extends RetrofitRequestBuilder {
protected BankAccountId bankAccountId;
protected BankAccountId getBankAccountId() {
return bankAccountId;
}
public AbstractDeleteBankAccountRequestBuilder(Retrofit retrofit, BankAccountId bankAccountId) {
super(retrofit);
this.bankAccountId = bankAccountId;
}
}
public static abstract class AbstractGetBankAccountRequestBuilder
extends RetrofitRequestBuilder {
protected BankAccountId bankAccountId;
protected BankAccountId getBankAccountId() {
return bankAccountId;
}
public AbstractGetBankAccountRequestBuilder(Retrofit retrofit, BankAccountId bankAccountId) {
super(retrofit);
this.bankAccountId = bankAccountId;
}
}
public static abstract class AbstractListAllBankAccountsRequestBuilder
extends RetrofitRequestBuilderPaginated {
public AbstractListAllBankAccountsRequestBuilder(Retrofit retrofit) {
super(retrofit);
}
}
public static abstract class AbstractGetPrimaryBankAccountRequestBuilder
extends RetrofitRequestBuilder {
public AbstractGetPrimaryBankAccountRequestBuilder(Retrofit retrofit) {
super(retrofit);
}
}
public static abstract class AbstractUpdateBankAccountRequestBuilder
extends IdempotentRetrofitRequestBuilder {
protected BankAccountId bankAccountId;
protected Boolean isPrimary;
protected String holderName;
protected String bankName;
protected String branchName;
protected String bankAddress;
protected String currency;
protected String accountNumber;
protected String swiftCode;
protected String routingNumber;
protected BankAccountId getBankAccountId() {
return bankAccountId;
}
protected Boolean getIsPrimary() {
return isPrimary;
}
protected String getHolderName() {
return holderName;
}
protected String getBankName() {
return bankName;
}
protected String getBranchName() {
return branchName;
}
protected String getBankAddress() {
return bankAddress;
}
protected String getCurrency() {
return currency;
}
protected String getAccountNumber() {
return accountNumber;
}
protected String getSwiftCode() {
return swiftCode;
}
protected String getRoutingNumber() {
return routingNumber;
}
public AbstractUpdateBankAccountRequestBuilder(Retrofit retrofit, BankAccountId bankAccountId) {
super(retrofit);
this.bankAccountId = bankAccountId;
}
public B withIsPrimary(Boolean isPrimary) {
this.isPrimary = isPrimary;
return (B)this;
}
public B withHolderName(String holderName) {
this.holderName = holderName;
return (B)this;
}
public B withBankName(String bankName) {
this.bankName = bankName;
return (B)this;
}
public B withBranchName(String branchName) {
this.branchName = branchName;
return (B)this;
}
public B withBankAddress(String bankAddress) {
this.bankAddress = bankAddress;
return (B)this;
}
public B withCurrency(String currency) {
this.currency = currency;
return (B)this;
}
public B withAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return (B)this;
}
public B withSwiftCode(String swiftCode) {
this.swiftCode = swiftCode;
return (B)this;
}
public B withRoutingNumber(String routingNumber) {
this.routingNumber = routingNumber;
return (B)this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy