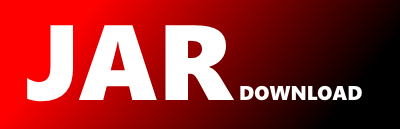
com.atlantbh.jmeter.plugins.jsonutils.jsonpathextractor.JSONPathExtractor Maven / Gradle / Ivy
/*!
* AtlantBH Custom Jmeter Components v1.0.0
* http://www.atlantbh.com/jmeter-components/
*
* Copyright 2011, AtlantBH
*
* Licensed under the under the Apache License, Version 2.0.
*/
package com.atlantbh.jmeter.plugins.jsonutils.jsonpathextractor;
import com.jayway.jsonpath.JsonPath;
import com.jayway.jsonpath.PathNotFoundException;
import net.minidev.json.JSONArray;
import org.apache.jmeter.processor.PostProcessor;
import org.apache.jmeter.samplers.SampleResult;
import org.apache.jmeter.testelement.AbstractTestElement;
import org.apache.jmeter.threads.JMeterContext;
import org.apache.jmeter.threads.JMeterVariables;
import org.apache.jorphan.logging.LoggingManager;
import org.apache.log.Logger;
/**
* This is main class for JSONPath extractor which works on previous sample
* result and extracts value from JSON output using JSONPath
*/
public class JSONPathExtractor extends AbstractTestElement implements PostProcessor {
private static final Logger log = LoggingManager.getLoggerForClass();
private static final long serialVersionUID = 1L;
public static final String JSONPATH = "JSONPATH";
public static final String VAR = "VAR";
public static final String DEFAULT = "DEFAULT";
public static final String SUBJECT = "SUBJECT";
public static final String SRC_VARNAME = "VARIABLE";
public static final String SUBJECT_BODY = "BODY";
public static final String SUBJECT_VARIABLE = "VAR";
public JSONPathExtractor() {
super();
}
public String getJsonPath() {
return getPropertyAsString(JSONPATH);
}
public void setJsonPath(String jsonPath) {
setProperty(JSONPATH, jsonPath);
}
public String getVar() {
return getPropertyAsString(VAR);
}
public void setVar(String var) {
setProperty(VAR, var);
}
public void setDefaultValue(String defaultValue) {
setProperty(DEFAULT, defaultValue);
}
public String getDefaultValue() {
return getPropertyAsString(DEFAULT);
}
public void setSrcVariableName(String defaultValue) {
setProperty(SRC_VARNAME, defaultValue);
}
public String getSrcVariableName() {
return getPropertyAsString(SRC_VARNAME);
}
public void setSubject(String defaultValue) {
setProperty(SUBJECT, defaultValue);
}
public String getSubject() {
return getPropertyAsString(SUBJECT);
}
@Override
public void process() {
JMeterContext context = getThreadContext();
JMeterVariables vars = context.getVariables();
SampleResult previousResult = context.getPreviousResult();
String responseData;
if (getSubject().equals(SUBJECT_VARIABLE)) {
responseData = vars.get(getSrcVariableName());
} else {
responseData = previousResult.getResponseDataAsString();
}
try {
Object jsonPathResult = JsonPath.read(responseData, getJsonPath());
if (jsonPathResult instanceof JSONArray) {
Object[] arr = ((JSONArray) jsonPathResult).toArray();
if (arr.length == 0) {
throw new PathNotFoundException("Extracted array is empty");
}
vars.put(this.getVar(), jsonPathResult.toString());
vars.put(this.getVar() + "_matchNr", String.valueOf(arr.length));
int k = 1;
while (vars.get(this.getVar() + "_" + k) != null) {
vars.remove(this.getVar() + "_" + k);
k++;
}
for (int n = 0; n < arr.length; n++) {
vars.put(this.getVar() + "_" + (n + 1), String.valueOf(arr[n]));
}
} else {
vars.put(this.getVar(), String.valueOf(jsonPathResult));
}
} catch (Exception e) {
log.warn("Extract failed", e);
vars.put(this.getVar(), getDefaultValue());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy