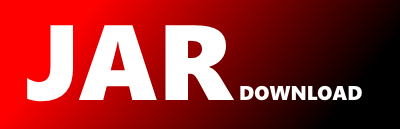
org.jmeterplugins.repository.PluginManagerDialog Maven / Gradle / Ivy
package org.jmeterplugins.repository;
import org.apache.jmeter.gui.action.ActionNames;
import org.apache.jmeter.gui.action.ActionRouter;
import org.apache.jorphan.gui.ComponentUtil;
import org.apache.jorphan.logging.LoggingManager;
import org.apache.log.Logger;
import javax.swing.*;
import javax.swing.border.Border;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.IOException;
public class PluginManagerDialog extends JDialog implements ActionListener {
private static final Logger log = LoggingManager.getLoggerForClass();
public static final Border SPACING = BorderFactory.createEmptyBorder(5, 5, 5, 5);
private final PluginManager manager;
private final JTextPane modifs = new JTextPane();
private final JButton apply = new JButton("Apply Changes and Restart JMeter");
private final PluginsList installed;
private final PluginsList available;
private final PluginUpgradesList upgrades;
private JLabel statusLabel = new JLabel("");
public PluginManagerDialog(PluginManager aManager) {
super((JFrame) null, "JMeter Plugins Manager", true);
setLayout(new BorderLayout());
manager = aManager;
Dimension size = new Dimension(1024, 768);
setSize(size);
setPreferredSize(size);
setIconImage(PluginManagerMenuItem.getPluginsIcon().getImage());
ComponentUtil.centerComponentInWindow(this);
try {
manager.load();
} catch (IOException e) {
log.error("Failed to load plugins manager", e);
}
final GenericCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy