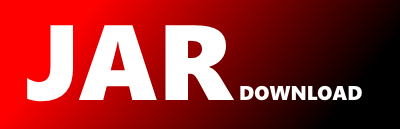
kh.gov.nbc.bakong_khqr.presenter.MerchantPresentedMode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-java Show documentation
Show all versions of sdk-java Show documentation
The standardization of KHQR code specifications will help promote wider use of
mobile retail payments in Cambodia and provide consistent user experience for merchants
and consumers. It can enable interoperability in the payment industry. A common QR code
would facilitate payments among different schemes, e-wallets and banks and would
encourage small merchants to adopt KHQR code as payment method.
KHQR is created for retail or remittance in Cambodia and Cross-Border. It only
requires a single QR for receiving transactions from any payment provider through Bakong
including Bakong App.
The newest version!
package kh.gov.nbc.bakong_khqr.presenter;
import kh.gov.nbc.bakong_khqr.core.CRC;
import kh.gov.nbc.bakong_khqr.model.*;
import kh.gov.nbc.bakong_khqr.core.TagLengthString;
import kh.gov.nbc.bakong_khqr.utils.StringUtils;
import kh.gov.nbc.bakong_khqr.utils.BakongKHQRUtils;
import java.io.Serializable;
import java.nio.charset.StandardCharsets;
import java.util.Calendar;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.TimeZone;
public class MerchantPresentedMode implements Serializable {
// Payload Format Indicator
private TagLengthString payloadFormatIndicator;
// Point of Initiation Method
private TagLengthString pointOfInitiationMethod;
private TagLengthString upiAccountInformation;
// Merchant Account Information
private final Map merchantAccountInformation = new LinkedHashMap<>();
// Merchant Category Code
private TagLengthString merchantCategoryCode;
// Transaction Currency
private TagLengthString transactionCurrency;
// Transaction Amount
private TagLengthString transactionAmount;
private TagLengthString countryCode;
// Merchant Name
private TagLengthString merchantName;
// Merchant City
private TagLengthString merchantCity;
// Additional Data Field Template
private AdditionalDataFieldTemplate additionalDataField;
// Merchant Information Language
private MerchantInformationLanguageTemplate merchantInformationLanguage;
// Additional Data Field Template
private RfuForKhqrTemplate rfuForKhqr;
public final void setPayloadFormatIndicator(final String payloadFormatIndicator) {
this.payloadFormatIndicator = new TagLengthString(KHQRMerchantPresentedCodes.PAYLOAD_FORMAT_INDICATOR, payloadFormatIndicator);
}
public final void setPointOfInitiationMethod(final String pointOfInitiationMethod) {
this.pointOfInitiationMethod = new TagLengthString(KHQRMerchantPresentedCodes.POINT_OF_INITIATION_METHOD, pointOfInitiationMethod);
}
public final void setUpiAccountInformation(final String upiAccountInformation) {
this.upiAccountInformation = new TagLengthString(KHQRMerchantPresentedCodes.UPI_ACCOUNT_INFORMATION, upiAccountInformation);
}
public final void setMerchantCategoryCode(final String merchantCategoryCode) {
this.merchantCategoryCode = new TagLengthString(KHQRMerchantPresentedCodes.MERCHANT_CATEGORY_CODE, merchantCategoryCode);
}
public final void setTransactionCurrency(final String transactionCurrency) {
this.transactionCurrency = new TagLengthString(KHQRMerchantPresentedCodes.TRANSACTION_CURRENCY, transactionCurrency);
}
public final void setTransactionAmount(final String transactionAmount) {
this.transactionAmount = new TagLengthString(KHQRMerchantPresentedCodes.TRANSACTION_AMOUNT, transactionAmount);
}
public final void setCountryCode(final String countryCode) {
this.countryCode = new TagLengthString(KHQRMerchantPresentedCodes.COUNTRY_CODE, countryCode);
}
public final void setMerchantName(final String merchantName) {
this.merchantName = new TagLengthString(KHQRMerchantPresentedCodes.MERCHANT_NAME, merchantName);
}
public final void setMerchantCity(final String merchantCity) {
this.merchantCity = new TagLengthString(KHQRMerchantPresentedCodes.MERCHANT_CITY, merchantCity);
}
public final void addMerchantAccountInformation(final MerchantAccountInformationTemplate merchantAccountInformation) {
this.merchantAccountInformation.put(merchantAccountInformation.getTag(), merchantAccountInformation);
}
public void setAdditionalDataField(final AdditionalDataFieldTemplate additionalDataField) {
this.additionalDataField = additionalDataField;
}
public void setMerchantInformationLanguage(final MerchantInformationLanguageTemplate merchantInformationLanguage) {
this.merchantInformationLanguage = merchantInformationLanguage;
}
private void setRfuForKhqr(){
RfuForKhqr rfuForKhqr = new RfuForKhqr();
rfuForKhqr.setTimeStamp(String.valueOf(Calendar.getInstance(TimeZone.getTimeZone("UTC")).getTimeInMillis()));
RfuForKhqrTemplate rfuForKhqrTemplate = new RfuForKhqrTemplate();
rfuForKhqrTemplate.setValue(rfuForKhqr);
this.rfuForKhqr = rfuForKhqrTemplate;
}
@Override
public String toString() {
setRfuForKhqr();
final StringBuilder sb = new StringBuilder(this.toStringWithoutCrc16());
final String string = sb.toString();
if (StringUtils.isBlank(string)) {
return StringUtils.EMPTY;
}
final int crc16 = CRC.crc16(sb.toString().getBytes(StandardCharsets.UTF_8));
sb.append(String.format("%04X", crc16));
return sb.toString();
}
public String toStringWithoutCrc16() {
final StringBuilder sb = new StringBuilder();
sb.append(BakongKHQRUtils.ofNullable(payloadFormatIndicator));
sb.append(BakongKHQRUtils.ofNullable(pointOfInitiationMethod));
sb.append(BakongKHQRUtils.ofNullable(upiAccountInformation));
for (final Map.Entry entry : merchantAccountInformation.entrySet()) {
sb.append(BakongKHQRUtils.ofNullable(entry.getValue()));
}
sb.append(BakongKHQRUtils.ofNullable(merchantCategoryCode));
sb.append(BakongKHQRUtils.ofNullable(transactionCurrency));
sb.append(BakongKHQRUtils.ofNullable(transactionAmount));
sb.append(BakongKHQRUtils.ofNullable(countryCode));
sb.append(BakongKHQRUtils.ofNullable(merchantName));
sb.append(BakongKHQRUtils.ofNullable(merchantCity));
sb.append(BakongKHQRUtils.ofNullable(additionalDataField));
sb.append(BakongKHQRUtils.ofNullable(merchantInformationLanguage));
sb.append(BakongKHQRUtils.ofNullable(rfuForKhqr));
final String string = sb.toString();
if (StringUtils.isBlank(string)) {
return StringUtils.EMPTY;
}
sb.append(String.format("%s%s", KHQRMerchantPresentedCodes.CRC, "04"));
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy