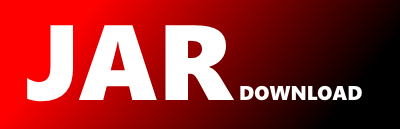
kim.zkp.quick.orm.util.AnnotationSqlBuilderUtils Maven / Gradle / Ivy
/**
* Copyright (c) 2017, ZhuKaipeng 朱开鹏 ([email protected]).
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package kim.zkp.quick.orm.util;
import java.lang.reflect.Field;
import java.util.List;
import kim.zkp.quick.orm.annotation.Condition;
import kim.zkp.quick.orm.annotation.Find;
import kim.zkp.quick.orm.annotation.Join;
import kim.zkp.quick.orm.annotation.OrderBy;
import kim.zkp.quick.orm.cache.ClassCache;
import kim.zkp.quick.orm.common.Constants;
import kim.zkp.quick.orm.exception.SqlBuilderException;
import kim.zkp.quick.orm.model.Schema;
import kim.zkp.quick.orm.sql.convert.FieldConvertProcessor;
public class AnnotationSqlBuilderUtils {
public static String getTableName(Object o) {
return ClassCache.getTableName(o.getClass());
}
public static String getSelect(Object o) {
if (o instanceof Schema) {
return " * ";
}
return ClassCache.getSelect(o.getClass(),true);
}
public static String getCondition(Object o, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy