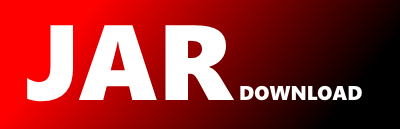
commonMain.kr.jadekim.chameleon.injective.InjectiveTransactionBuilder.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chameleon-injective-jvm Show documentation
Show all versions of chameleon-injective-jvm Show documentation
Crypto Wallet SDK for multiple blockchains
package kr.jadekim.chameleon.injective
import cosmos.base.v1beta1.Coin
import cosmos.crypto.secp256k1.toAny
import cosmos.tx.signing.v1beta1.SignMode
import cosmos.tx.v1beta1.*
import google.protobuf.Any
class InjectiveTransactionBuilder {
private val messages: MutableList = mutableListOf()
private var memo: String = ""
private var timeoutHeight: ULong = 0u
private val extensionOptions: MutableList = mutableListOf()
private val nonCriticalExtensionOptions: MutableList = mutableListOf()
private val signerInfos: MutableList = mutableListOf()
private var fee: Fee = Fee(emptyList(), 0u, "", "")
private var tip: Tip = Tip(emptyList(), "")
private val signatures: MutableList = mutableListOf()
fun message(vararg message: Any): InjectiveTransactionBuilder {
this.messages.addAll(message)
return this
}
fun memo(memo: String): InjectiveTransactionBuilder {
this.memo = memo
return this
}
fun timeoutHeight(timeoutHeight: ULong): InjectiveTransactionBuilder {
this.timeoutHeight = timeoutHeight
return this
}
fun extensionOption(vararg option: Any): InjectiveTransactionBuilder {
this.extensionOptions.addAll(option)
return this
}
fun nonCriticalExtensionOption(vararg option: Any): InjectiveTransactionBuilder {
this.nonCriticalExtensionOptions.addAll(option)
return this
}
fun signer(vararg signerInfo: SignerInfo): InjectiveTransactionBuilder {
this.signerInfos.addAll(signerInfo)
return this
}
fun signer(publicKey: Any, sequence: ULong, signMode: SignMode = SignMode.SIGN_MODE_DIRECT) = signer(
SignerInfo(
publicKey,
ModeInfo(ModeInfo.SumOneOf.Single(ModeInfo.Single(signMode))),
sequence,
)
)
fun signer(publicKey: ByteArray, sequence: ULong, signMode: SignMode = SignMode.SIGN_MODE_DIRECT) = signer(
cosmos.crypto.secp256k1.PubKey(publicKey).toAny(),
sequence,
signMode,
)
fun fee(fee: Fee): InjectiveTransactionBuilder {
this.fee = fee
return this
}
fun fee(gasLimit: ULong, vararg coin: Coin): InjectiveTransactionBuilder {
this.fee = fee.copy(amount = fee.amount + coin, gasLimit = gasLimit)
return this
}
fun tip(tip: Tip): InjectiveTransactionBuilder {
this.tip = tip
return this
}
fun signature(vararg signature: ByteArray): InjectiveTransactionBuilder {
this.signatures.addAll(signature)
return this
}
fun build() = Tx(
body = TxBody(
messages = messages,
memo = memo,
timeoutHeight = timeoutHeight,
extensionOptions = extensionOptions,
nonCriticalExtensionOptions = nonCriticalExtensionOptions,
),
authInfo = AuthInfo(
signerInfos = signerInfos,
fee = fee,
tip = tip,
),
signatures = signatures,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy