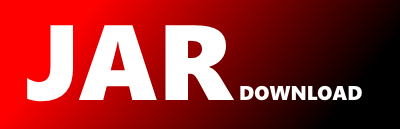
commonMain.terra.oracle.v1beta1.query.converter.kt Maven / Gradle / Ivy
// Transform from terra/oracle/v1beta1/query.proto
@file:GeneratorVersion(version = "0.5.2")
package terra.oracle.v1beta1
import google.protobuf.Any
import java.lang.IllegalStateException
import kr.jadekim.protobuf.`annotation`.GeneratorVersion
import kr.jadekim.protobuf.converter.ProtobufConverter
import kr.jadekim.protobuf.converter.parseProtobuf
public expect object QueryExchangeRateRequestConverter : ProtobufConverter
public fun QueryExchangeRateRequest.toAny(): Any = Any(QueryExchangeRateRequest.TYPE_URL,
with(QueryExchangeRateRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryExchangeRateRequestConverter): QueryExchangeRateRequest {
if (typeUrl != QueryExchangeRateRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryExchangeRateRequest.Companion.converter: QueryExchangeRateRequestConverter
get() = QueryExchangeRateRequestConverter
public expect object QueryExchangeRateResponseConverter :
ProtobufConverter
public fun QueryExchangeRateResponse.toAny(): Any = Any(QueryExchangeRateResponse.TYPE_URL,
with(QueryExchangeRateResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryExchangeRateResponseConverter): QueryExchangeRateResponse {
if (typeUrl != QueryExchangeRateResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryExchangeRateResponse.Companion.converter: QueryExchangeRateResponseConverter
get() = QueryExchangeRateResponseConverter
public expect object QueryExchangeRatesRequestConverter :
ProtobufConverter
public fun QueryExchangeRatesRequest.toAny(): Any = Any(QueryExchangeRatesRequest.TYPE_URL,
with(QueryExchangeRatesRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryExchangeRatesRequestConverter): QueryExchangeRatesRequest {
if (typeUrl != QueryExchangeRatesRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryExchangeRatesRequest.Companion.converter: QueryExchangeRatesRequestConverter
get() = QueryExchangeRatesRequestConverter
public expect object QueryExchangeRatesResponseConverter :
ProtobufConverter
public fun QueryExchangeRatesResponse.toAny(): Any = Any(QueryExchangeRatesResponse.TYPE_URL,
with(QueryExchangeRatesResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryExchangeRatesResponseConverter): QueryExchangeRatesResponse {
if (typeUrl != QueryExchangeRatesResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryExchangeRatesResponse.Companion.converter: QueryExchangeRatesResponseConverter
get() = QueryExchangeRatesResponseConverter
public expect object QueryTobinTaxRequestConverter : ProtobufConverter
public fun QueryTobinTaxRequest.toAny(): Any = Any(QueryTobinTaxRequest.TYPE_URL,
with(QueryTobinTaxRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTobinTaxRequestConverter): QueryTobinTaxRequest {
if (typeUrl != QueryTobinTaxRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTobinTaxRequest.Companion.converter: QueryTobinTaxRequestConverter
get() = QueryTobinTaxRequestConverter
public expect object QueryTobinTaxResponseConverter : ProtobufConverter
public fun QueryTobinTaxResponse.toAny(): Any = Any(QueryTobinTaxResponse.TYPE_URL,
with(QueryTobinTaxResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTobinTaxResponseConverter): QueryTobinTaxResponse {
if (typeUrl != QueryTobinTaxResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTobinTaxResponse.Companion.converter: QueryTobinTaxResponseConverter
get() = QueryTobinTaxResponseConverter
public expect object QueryTobinTaxesRequestConverter : ProtobufConverter
public fun QueryTobinTaxesRequest.toAny(): Any = Any(QueryTobinTaxesRequest.TYPE_URL,
with(QueryTobinTaxesRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTobinTaxesRequestConverter): QueryTobinTaxesRequest {
if (typeUrl != QueryTobinTaxesRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTobinTaxesRequest.Companion.converter: QueryTobinTaxesRequestConverter
get() = QueryTobinTaxesRequestConverter
public expect object QueryTobinTaxesResponseConverter : ProtobufConverter
public fun QueryTobinTaxesResponse.toAny(): Any = Any(QueryTobinTaxesResponse.TYPE_URL,
with(QueryTobinTaxesResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTobinTaxesResponseConverter): QueryTobinTaxesResponse {
if (typeUrl != QueryTobinTaxesResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTobinTaxesResponse.Companion.converter: QueryTobinTaxesResponseConverter
get() = QueryTobinTaxesResponseConverter
public expect object QueryActivesRequestConverter : ProtobufConverter
public fun QueryActivesRequest.toAny(): Any = Any(QueryActivesRequest.TYPE_URL,
with(QueryActivesRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryActivesRequestConverter): QueryActivesRequest {
if (typeUrl != QueryActivesRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryActivesRequest.Companion.converter: QueryActivesRequestConverter
get() = QueryActivesRequestConverter
public expect object QueryActivesResponseConverter : ProtobufConverter
public fun QueryActivesResponse.toAny(): Any = Any(QueryActivesResponse.TYPE_URL,
with(QueryActivesResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryActivesResponseConverter): QueryActivesResponse {
if (typeUrl != QueryActivesResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryActivesResponse.Companion.converter: QueryActivesResponseConverter
get() = QueryActivesResponseConverter
public expect object QueryVoteTargetsRequestConverter : ProtobufConverter
public fun QueryVoteTargetsRequest.toAny(): Any = Any(QueryVoteTargetsRequest.TYPE_URL,
with(QueryVoteTargetsRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryVoteTargetsRequestConverter): QueryVoteTargetsRequest {
if (typeUrl != QueryVoteTargetsRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryVoteTargetsRequest.Companion.converter: QueryVoteTargetsRequestConverter
get() = QueryVoteTargetsRequestConverter
public expect object QueryVoteTargetsResponseConverter : ProtobufConverter
public fun QueryVoteTargetsResponse.toAny(): Any = Any(QueryVoteTargetsResponse.TYPE_URL,
with(QueryVoteTargetsResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryVoteTargetsResponseConverter): QueryVoteTargetsResponse {
if (typeUrl != QueryVoteTargetsResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryVoteTargetsResponse.Companion.converter: QueryVoteTargetsResponseConverter
get() = QueryVoteTargetsResponseConverter
public expect object QueryFeederDelegationRequestConverter :
ProtobufConverter
public fun QueryFeederDelegationRequest.toAny(): Any = Any(QueryFeederDelegationRequest.TYPE_URL,
with(QueryFeederDelegationRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryFeederDelegationRequestConverter): QueryFeederDelegationRequest {
if (typeUrl != QueryFeederDelegationRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryFeederDelegationRequest.Companion.converter: QueryFeederDelegationRequestConverter
get() = QueryFeederDelegationRequestConverter
public expect object QueryFeederDelegationResponseConverter :
ProtobufConverter
public fun QueryFeederDelegationResponse.toAny(): Any = Any(QueryFeederDelegationResponse.TYPE_URL,
with(QueryFeederDelegationResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryFeederDelegationResponseConverter): QueryFeederDelegationResponse {
if (typeUrl != QueryFeederDelegationResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryFeederDelegationResponse.Companion.converter: QueryFeederDelegationResponseConverter
get() = QueryFeederDelegationResponseConverter
public expect object QueryMissCounterRequestConverter : ProtobufConverter
public fun QueryMissCounterRequest.toAny(): Any = Any(QueryMissCounterRequest.TYPE_URL,
with(QueryMissCounterRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryMissCounterRequestConverter): QueryMissCounterRequest {
if (typeUrl != QueryMissCounterRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryMissCounterRequest.Companion.converter: QueryMissCounterRequestConverter
get() = QueryMissCounterRequestConverter
public expect object QueryMissCounterResponseConverter : ProtobufConverter
public fun QueryMissCounterResponse.toAny(): Any = Any(QueryMissCounterResponse.TYPE_URL,
with(QueryMissCounterResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryMissCounterResponseConverter): QueryMissCounterResponse {
if (typeUrl != QueryMissCounterResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryMissCounterResponse.Companion.converter: QueryMissCounterResponseConverter
get() = QueryMissCounterResponseConverter
public expect object QueryAggregatePrevoteRequestConverter :
ProtobufConverter
public fun QueryAggregatePrevoteRequest.toAny(): Any = Any(QueryAggregatePrevoteRequest.TYPE_URL,
with(QueryAggregatePrevoteRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryAggregatePrevoteRequestConverter): QueryAggregatePrevoteRequest {
if (typeUrl != QueryAggregatePrevoteRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryAggregatePrevoteRequest.Companion.converter: QueryAggregatePrevoteRequestConverter
get() = QueryAggregatePrevoteRequestConverter
public expect object QueryAggregatePrevoteResponseConverter :
ProtobufConverter
public fun QueryAggregatePrevoteResponse.toAny(): Any = Any(QueryAggregatePrevoteResponse.TYPE_URL,
with(QueryAggregatePrevoteResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryAggregatePrevoteResponseConverter): QueryAggregatePrevoteResponse {
if (typeUrl != QueryAggregatePrevoteResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryAggregatePrevoteResponse.Companion.converter: QueryAggregatePrevoteResponseConverter
get() = QueryAggregatePrevoteResponseConverter
public expect object QueryAggregatePrevotesRequestConverter :
ProtobufConverter
public fun QueryAggregatePrevotesRequest.toAny(): Any = Any(QueryAggregatePrevotesRequest.TYPE_URL,
with(QueryAggregatePrevotesRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryAggregatePrevotesRequestConverter): QueryAggregatePrevotesRequest {
if (typeUrl != QueryAggregatePrevotesRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryAggregatePrevotesRequest.Companion.converter: QueryAggregatePrevotesRequestConverter
get() = QueryAggregatePrevotesRequestConverter
public expect object QueryAggregatePrevotesResponseConverter :
ProtobufConverter
public fun QueryAggregatePrevotesResponse.toAny(): Any =
Any(QueryAggregatePrevotesResponse.TYPE_URL, with(QueryAggregatePrevotesResponseConverter) {
toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryAggregatePrevotesResponseConverter): QueryAggregatePrevotesResponse {
if (typeUrl != QueryAggregatePrevotesResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryAggregatePrevotesResponse.Companion.converter:
QueryAggregatePrevotesResponseConverter
get() = QueryAggregatePrevotesResponseConverter
public expect object QueryAggregateVoteRequestConverter :
ProtobufConverter
public fun QueryAggregateVoteRequest.toAny(): Any = Any(QueryAggregateVoteRequest.TYPE_URL,
with(QueryAggregateVoteRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryAggregateVoteRequestConverter): QueryAggregateVoteRequest {
if (typeUrl != QueryAggregateVoteRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryAggregateVoteRequest.Companion.converter: QueryAggregateVoteRequestConverter
get() = QueryAggregateVoteRequestConverter
public expect object QueryAggregateVoteResponseConverter :
ProtobufConverter
public fun QueryAggregateVoteResponse.toAny(): Any = Any(QueryAggregateVoteResponse.TYPE_URL,
with(QueryAggregateVoteResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryAggregateVoteResponseConverter): QueryAggregateVoteResponse {
if (typeUrl != QueryAggregateVoteResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryAggregateVoteResponse.Companion.converter: QueryAggregateVoteResponseConverter
get() = QueryAggregateVoteResponseConverter
public expect object QueryAggregateVotesRequestConverter :
ProtobufConverter
public fun QueryAggregateVotesRequest.toAny(): Any = Any(QueryAggregateVotesRequest.TYPE_URL,
with(QueryAggregateVotesRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryAggregateVotesRequestConverter): QueryAggregateVotesRequest {
if (typeUrl != QueryAggregateVotesRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryAggregateVotesRequest.Companion.converter: QueryAggregateVotesRequestConverter
get() = QueryAggregateVotesRequestConverter
public expect object QueryAggregateVotesResponseConverter :
ProtobufConverter
public fun QueryAggregateVotesResponse.toAny(): Any = Any(QueryAggregateVotesResponse.TYPE_URL,
with(QueryAggregateVotesResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryAggregateVotesResponseConverter): QueryAggregateVotesResponse {
if (typeUrl != QueryAggregateVotesResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryAggregateVotesResponse.Companion.converter: QueryAggregateVotesResponseConverter
get() = QueryAggregateVotesResponseConverter
public expect object QueryParamsRequestConverter : ProtobufConverter
public fun QueryParamsRequest.toAny(): Any = Any(QueryParamsRequest.TYPE_URL,
with(QueryParamsRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryParamsRequestConverter): QueryParamsRequest {
if (typeUrl != QueryParamsRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryParamsRequest.Companion.converter: QueryParamsRequestConverter
get() = QueryParamsRequestConverter
public expect object QueryParamsResponseConverter : ProtobufConverter
public fun QueryParamsResponse.toAny(): Any = Any(QueryParamsResponse.TYPE_URL,
with(QueryParamsResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryParamsResponseConverter): QueryParamsResponse {
if (typeUrl != QueryParamsResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryParamsResponse.Companion.converter: QueryParamsResponseConverter
get() = QueryParamsResponseConverter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy