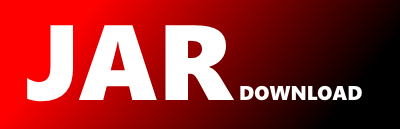
commonMain.terra.treasury.v1beta1.query.converter.kt Maven / Gradle / Ivy
// Transform from terra/treasury/v1beta1/query.proto
@file:GeneratorVersion(version = "0.5.2")
package terra.treasury.v1beta1
import google.protobuf.Any
import java.lang.IllegalStateException
import kr.jadekim.protobuf.`annotation`.GeneratorVersion
import kr.jadekim.protobuf.converter.ProtobufConverter
import kr.jadekim.protobuf.converter.parseProtobuf
public expect object QueryTaxRateRequestConverter : ProtobufConverter
public fun QueryTaxRateRequest.toAny(): Any = Any(QueryTaxRateRequest.TYPE_URL,
with(QueryTaxRateRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTaxRateRequestConverter): QueryTaxRateRequest {
if (typeUrl != QueryTaxRateRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTaxRateRequest.Companion.converter: QueryTaxRateRequestConverter
get() = QueryTaxRateRequestConverter
public expect object QueryTaxRateResponseConverter : ProtobufConverter
public fun QueryTaxRateResponse.toAny(): Any = Any(QueryTaxRateResponse.TYPE_URL,
with(QueryTaxRateResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTaxRateResponseConverter): QueryTaxRateResponse {
if (typeUrl != QueryTaxRateResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTaxRateResponse.Companion.converter: QueryTaxRateResponseConverter
get() = QueryTaxRateResponseConverter
public expect object QueryTaxCapRequestConverter : ProtobufConverter
public fun QueryTaxCapRequest.toAny(): Any = Any(QueryTaxCapRequest.TYPE_URL,
with(QueryTaxCapRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTaxCapRequestConverter): QueryTaxCapRequest {
if (typeUrl != QueryTaxCapRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTaxCapRequest.Companion.converter: QueryTaxCapRequestConverter
get() = QueryTaxCapRequestConverter
public expect object QueryTaxCapResponseConverter : ProtobufConverter
public fun QueryTaxCapResponse.toAny(): Any = Any(QueryTaxCapResponse.TYPE_URL,
with(QueryTaxCapResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTaxCapResponseConverter): QueryTaxCapResponse {
if (typeUrl != QueryTaxCapResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTaxCapResponse.Companion.converter: QueryTaxCapResponseConverter
get() = QueryTaxCapResponseConverter
public expect object QueryTaxCapsRequestConverter : ProtobufConverter
public fun QueryTaxCapsRequest.toAny(): Any = Any(QueryTaxCapsRequest.TYPE_URL,
with(QueryTaxCapsRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTaxCapsRequestConverter): QueryTaxCapsRequest {
if (typeUrl != QueryTaxCapsRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTaxCapsRequest.Companion.converter: QueryTaxCapsRequestConverter
get() = QueryTaxCapsRequestConverter
public expect object QueryTaxCapsResponseItemConverter : ProtobufConverter
public fun QueryTaxCapsResponseItem.toAny(): Any = Any(QueryTaxCapsResponseItem.TYPE_URL,
with(QueryTaxCapsResponseItemConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTaxCapsResponseItemConverter): QueryTaxCapsResponseItem {
if (typeUrl != QueryTaxCapsResponseItem.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTaxCapsResponseItem.Companion.converter: QueryTaxCapsResponseItemConverter
get() = QueryTaxCapsResponseItemConverter
public expect object QueryTaxCapsResponseConverter : ProtobufConverter
public fun QueryTaxCapsResponse.toAny(): Any = Any(QueryTaxCapsResponse.TYPE_URL,
with(QueryTaxCapsResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTaxCapsResponseConverter): QueryTaxCapsResponse {
if (typeUrl != QueryTaxCapsResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTaxCapsResponse.Companion.converter: QueryTaxCapsResponseConverter
get() = QueryTaxCapsResponseConverter
public expect object QueryRewardWeightRequestConverter : ProtobufConverter
public fun QueryRewardWeightRequest.toAny(): Any = Any(QueryRewardWeightRequest.TYPE_URL,
with(QueryRewardWeightRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryRewardWeightRequestConverter): QueryRewardWeightRequest {
if (typeUrl != QueryRewardWeightRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryRewardWeightRequest.Companion.converter: QueryRewardWeightRequestConverter
get() = QueryRewardWeightRequestConverter
public expect object QueryRewardWeightResponseConverter :
ProtobufConverter
public fun QueryRewardWeightResponse.toAny(): Any = Any(QueryRewardWeightResponse.TYPE_URL,
with(QueryRewardWeightResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryRewardWeightResponseConverter): QueryRewardWeightResponse {
if (typeUrl != QueryRewardWeightResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryRewardWeightResponse.Companion.converter: QueryRewardWeightResponseConverter
get() = QueryRewardWeightResponseConverter
public expect object QueryTaxProceedsRequestConverter : ProtobufConverter
public fun QueryTaxProceedsRequest.toAny(): Any = Any(QueryTaxProceedsRequest.TYPE_URL,
with(QueryTaxProceedsRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTaxProceedsRequestConverter): QueryTaxProceedsRequest {
if (typeUrl != QueryTaxProceedsRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTaxProceedsRequest.Companion.converter: QueryTaxProceedsRequestConverter
get() = QueryTaxProceedsRequestConverter
public expect object QueryTaxProceedsResponseConverter : ProtobufConverter
public fun QueryTaxProceedsResponse.toAny(): Any = Any(QueryTaxProceedsResponse.TYPE_URL,
with(QueryTaxProceedsResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryTaxProceedsResponseConverter): QueryTaxProceedsResponse {
if (typeUrl != QueryTaxProceedsResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryTaxProceedsResponse.Companion.converter: QueryTaxProceedsResponseConverter
get() = QueryTaxProceedsResponseConverter
public expect object QuerySeigniorageProceedsRequestConverter :
ProtobufConverter
public fun QuerySeigniorageProceedsRequest.toAny(): Any =
Any(QuerySeigniorageProceedsRequest.TYPE_URL, with(QuerySeigniorageProceedsRequestConverter) {
toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QuerySeigniorageProceedsRequestConverter): QuerySeigniorageProceedsRequest {
if (typeUrl != QuerySeigniorageProceedsRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QuerySeigniorageProceedsRequest.Companion.converter:
QuerySeigniorageProceedsRequestConverter
get() = QuerySeigniorageProceedsRequestConverter
public expect object QuerySeigniorageProceedsResponseConverter :
ProtobufConverter
public fun QuerySeigniorageProceedsResponse.toAny(): Any =
Any(QuerySeigniorageProceedsResponse.TYPE_URL, with(QuerySeigniorageProceedsResponseConverter) {
toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QuerySeigniorageProceedsResponseConverter): QuerySeigniorageProceedsResponse {
if (typeUrl != QuerySeigniorageProceedsResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QuerySeigniorageProceedsResponse.Companion.converter:
QuerySeigniorageProceedsResponseConverter
get() = QuerySeigniorageProceedsResponseConverter
public expect object QueryIndicatorsRequestConverter : ProtobufConverter
public fun QueryIndicatorsRequest.toAny(): Any = Any(QueryIndicatorsRequest.TYPE_URL,
with(QueryIndicatorsRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryIndicatorsRequestConverter): QueryIndicatorsRequest {
if (typeUrl != QueryIndicatorsRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryIndicatorsRequest.Companion.converter: QueryIndicatorsRequestConverter
get() = QueryIndicatorsRequestConverter
public expect object QueryIndicatorsResponseConverter : ProtobufConverter
public fun QueryIndicatorsResponse.toAny(): Any = Any(QueryIndicatorsResponse.TYPE_URL,
with(QueryIndicatorsResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryIndicatorsResponseConverter): QueryIndicatorsResponse {
if (typeUrl != QueryIndicatorsResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryIndicatorsResponse.Companion.converter: QueryIndicatorsResponseConverter
get() = QueryIndicatorsResponseConverter
public expect object QueryParamsRequestConverter : ProtobufConverter
public fun QueryParamsRequest.toAny(): Any = Any(QueryParamsRequest.TYPE_URL,
with(QueryParamsRequestConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryParamsRequestConverter): QueryParamsRequest {
if (typeUrl != QueryParamsRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryParamsRequest.Companion.converter: QueryParamsRequestConverter
get() = QueryParamsRequestConverter
public expect object QueryParamsResponseConverter : ProtobufConverter
public fun QueryParamsResponse.toAny(): Any = Any(QueryParamsResponse.TYPE_URL,
with(QueryParamsResponseConverter) { toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryParamsResponseConverter): QueryParamsResponse {
if (typeUrl != QueryParamsResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryParamsResponse.Companion.converter: QueryParamsResponseConverter
get() = QueryParamsResponseConverter
public expect object QueryBurnTaxExemptionListRequestConverter :
ProtobufConverter
public fun QueryBurnTaxExemptionListRequest.toAny(): Any =
Any(QueryBurnTaxExemptionListRequest.TYPE_URL, with(QueryBurnTaxExemptionListRequestConverter) {
toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryBurnTaxExemptionListRequestConverter): QueryBurnTaxExemptionListRequest {
if (typeUrl != QueryBurnTaxExemptionListRequest.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryBurnTaxExemptionListRequest.Companion.converter:
QueryBurnTaxExemptionListRequestConverter
get() = QueryBurnTaxExemptionListRequestConverter
public expect object QueryBurnTaxExemptionListResponseConverter :
ProtobufConverter
public fun QueryBurnTaxExemptionListResponse.toAny(): Any =
Any(QueryBurnTaxExemptionListResponse.TYPE_URL, with(QueryBurnTaxExemptionListResponseConverter)
{ toByteArray() })
public fun Any.parse(converter: ProtobufConverter =
QueryBurnTaxExemptionListResponseConverter): QueryBurnTaxExemptionListResponse {
if (typeUrl != QueryBurnTaxExemptionListResponse.TYPE_URL) throw
IllegalStateException("Please check the type_url")
return value.parseProtobuf(converter)
}
public val QueryBurnTaxExemptionListResponse.Companion.converter:
QueryBurnTaxExemptionListResponseConverter
get() = QueryBurnTaxExemptionListResponseConverter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy