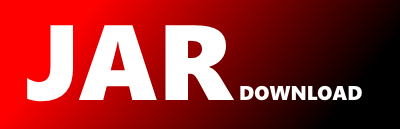
jvmMain.terra.oracle.v1beta1.QueryGrpc Maven / Gradle / Ivy
package terra.oracle.v1beta1;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* Query defines the gRPC querier service.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.63.0)",
comments = "Source: terra/oracle/v1beta1/query.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class QueryGrpc {
private QueryGrpc() {}
public static final java.lang.String SERVICE_NAME = "terra.oracle.v1beta1.Query";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getExchangeRateMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExchangeRate",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExchangeRateMethod() {
io.grpc.MethodDescriptor getExchangeRateMethod;
if ((getExchangeRateMethod = QueryGrpc.getExchangeRateMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getExchangeRateMethod = QueryGrpc.getExchangeRateMethod) == null) {
QueryGrpc.getExchangeRateMethod = getExchangeRateMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExchangeRate"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("ExchangeRate"))
.build();
}
}
}
return getExchangeRateMethod;
}
private static volatile io.grpc.MethodDescriptor getExchangeRatesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExchangeRates",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExchangeRatesMethod() {
io.grpc.MethodDescriptor getExchangeRatesMethod;
if ((getExchangeRatesMethod = QueryGrpc.getExchangeRatesMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getExchangeRatesMethod = QueryGrpc.getExchangeRatesMethod) == null) {
QueryGrpc.getExchangeRatesMethod = getExchangeRatesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExchangeRates"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("ExchangeRates"))
.build();
}
}
}
return getExchangeRatesMethod;
}
private static volatile io.grpc.MethodDescriptor getTobinTaxMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "TobinTax",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getTobinTaxMethod() {
io.grpc.MethodDescriptor getTobinTaxMethod;
if ((getTobinTaxMethod = QueryGrpc.getTobinTaxMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getTobinTaxMethod = QueryGrpc.getTobinTaxMethod) == null) {
QueryGrpc.getTobinTaxMethod = getTobinTaxMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "TobinTax"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("TobinTax"))
.build();
}
}
}
return getTobinTaxMethod;
}
private static volatile io.grpc.MethodDescriptor getTobinTaxesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "TobinTaxes",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getTobinTaxesMethod() {
io.grpc.MethodDescriptor getTobinTaxesMethod;
if ((getTobinTaxesMethod = QueryGrpc.getTobinTaxesMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getTobinTaxesMethod = QueryGrpc.getTobinTaxesMethod) == null) {
QueryGrpc.getTobinTaxesMethod = getTobinTaxesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "TobinTaxes"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("TobinTaxes"))
.build();
}
}
}
return getTobinTaxesMethod;
}
private static volatile io.grpc.MethodDescriptor getActivesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Actives",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryActivesRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryActivesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getActivesMethod() {
io.grpc.MethodDescriptor getActivesMethod;
if ((getActivesMethod = QueryGrpc.getActivesMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getActivesMethod = QueryGrpc.getActivesMethod) == null) {
QueryGrpc.getActivesMethod = getActivesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Actives"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryActivesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryActivesResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("Actives"))
.build();
}
}
}
return getActivesMethod;
}
private static volatile io.grpc.MethodDescriptor getVoteTargetsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "VoteTargets",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getVoteTargetsMethod() {
io.grpc.MethodDescriptor getVoteTargetsMethod;
if ((getVoteTargetsMethod = QueryGrpc.getVoteTargetsMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getVoteTargetsMethod = QueryGrpc.getVoteTargetsMethod) == null) {
QueryGrpc.getVoteTargetsMethod = getVoteTargetsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "VoteTargets"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("VoteTargets"))
.build();
}
}
}
return getVoteTargetsMethod;
}
private static volatile io.grpc.MethodDescriptor getFeederDelegationMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "FeederDelegation",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getFeederDelegationMethod() {
io.grpc.MethodDescriptor getFeederDelegationMethod;
if ((getFeederDelegationMethod = QueryGrpc.getFeederDelegationMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getFeederDelegationMethod = QueryGrpc.getFeederDelegationMethod) == null) {
QueryGrpc.getFeederDelegationMethod = getFeederDelegationMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "FeederDelegation"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("FeederDelegation"))
.build();
}
}
}
return getFeederDelegationMethod;
}
private static volatile io.grpc.MethodDescriptor getMissCounterMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "MissCounter",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getMissCounterMethod() {
io.grpc.MethodDescriptor getMissCounterMethod;
if ((getMissCounterMethod = QueryGrpc.getMissCounterMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getMissCounterMethod = QueryGrpc.getMissCounterMethod) == null) {
QueryGrpc.getMissCounterMethod = getMissCounterMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "MissCounter"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("MissCounter"))
.build();
}
}
}
return getMissCounterMethod;
}
private static volatile io.grpc.MethodDescriptor getAggregatePrevoteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AggregatePrevote",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAggregatePrevoteMethod() {
io.grpc.MethodDescriptor getAggregatePrevoteMethod;
if ((getAggregatePrevoteMethod = QueryGrpc.getAggregatePrevoteMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getAggregatePrevoteMethod = QueryGrpc.getAggregatePrevoteMethod) == null) {
QueryGrpc.getAggregatePrevoteMethod = getAggregatePrevoteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AggregatePrevote"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("AggregatePrevote"))
.build();
}
}
}
return getAggregatePrevoteMethod;
}
private static volatile io.grpc.MethodDescriptor getAggregatePrevotesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AggregatePrevotes",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAggregatePrevotesMethod() {
io.grpc.MethodDescriptor getAggregatePrevotesMethod;
if ((getAggregatePrevotesMethod = QueryGrpc.getAggregatePrevotesMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getAggregatePrevotesMethod = QueryGrpc.getAggregatePrevotesMethod) == null) {
QueryGrpc.getAggregatePrevotesMethod = getAggregatePrevotesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AggregatePrevotes"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("AggregatePrevotes"))
.build();
}
}
}
return getAggregatePrevotesMethod;
}
private static volatile io.grpc.MethodDescriptor getAggregateVoteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AggregateVote",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAggregateVoteMethod() {
io.grpc.MethodDescriptor getAggregateVoteMethod;
if ((getAggregateVoteMethod = QueryGrpc.getAggregateVoteMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getAggregateVoteMethod = QueryGrpc.getAggregateVoteMethod) == null) {
QueryGrpc.getAggregateVoteMethod = getAggregateVoteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AggregateVote"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("AggregateVote"))
.build();
}
}
}
return getAggregateVoteMethod;
}
private static volatile io.grpc.MethodDescriptor getAggregateVotesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AggregateVotes",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAggregateVotesMethod() {
io.grpc.MethodDescriptor getAggregateVotesMethod;
if ((getAggregateVotesMethod = QueryGrpc.getAggregateVotesMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getAggregateVotesMethod = QueryGrpc.getAggregateVotesMethod) == null) {
QueryGrpc.getAggregateVotesMethod = getAggregateVotesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AggregateVotes"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("AggregateVotes"))
.build();
}
}
}
return getAggregateVotesMethod;
}
private static volatile io.grpc.MethodDescriptor getParamsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Params",
requestType = terra.oracle.v1beta1.QueryOuterClass.QueryParamsRequest.class,
responseType = terra.oracle.v1beta1.QueryOuterClass.QueryParamsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getParamsMethod() {
io.grpc.MethodDescriptor getParamsMethod;
if ((getParamsMethod = QueryGrpc.getParamsMethod) == null) {
synchronized (QueryGrpc.class) {
if ((getParamsMethod = QueryGrpc.getParamsMethod) == null) {
QueryGrpc.getParamsMethod = getParamsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Params"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryParamsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
terra.oracle.v1beta1.QueryOuterClass.QueryParamsResponse.getDefaultInstance()))
.setSchemaDescriptor(new QueryMethodDescriptorSupplier("Params"))
.build();
}
}
}
return getParamsMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static QueryStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public QueryStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new QueryStub(channel, callOptions);
}
};
return QueryStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static QueryBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public QueryBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new QueryBlockingStub(channel, callOptions);
}
};
return QueryBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static QueryFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public QueryFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new QueryFutureStub(channel, callOptions);
}
};
return QueryFutureStub.newStub(factory, channel);
}
/**
*
* Query defines the gRPC querier service.
*
*/
public interface AsyncService {
/**
*
* ExchangeRate returns exchange rate of a denom
*
*/
default void exchangeRate(terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExchangeRateMethod(), responseObserver);
}
/**
*
* ExchangeRates returns exchange rates of all denoms
*
*/
default void exchangeRates(terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExchangeRatesMethod(), responseObserver);
}
/**
*
* TobinTax returns tobin tax of a denom
*
*/
default void tobinTax(terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getTobinTaxMethod(), responseObserver);
}
/**
*
* TobinTaxes returns tobin taxes of all denoms
*
*/
default void tobinTaxes(terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getTobinTaxesMethod(), responseObserver);
}
/**
*
* Actives returns all active denoms
*
*/
default void actives(terra.oracle.v1beta1.QueryOuterClass.QueryActivesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getActivesMethod(), responseObserver);
}
/**
*
* VoteTargets returns all vote target denoms
*
*/
default void voteTargets(terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getVoteTargetsMethod(), responseObserver);
}
/**
*
* FeederDelegation returns feeder delegation of a validator
*
*/
default void feederDelegation(terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getFeederDelegationMethod(), responseObserver);
}
/**
*
* MissCounter returns oracle miss counter of a validator
*
*/
default void missCounter(terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getMissCounterMethod(), responseObserver);
}
/**
*
* AggregatePrevote returns an aggregate prevote of a validator
*
*/
default void aggregatePrevote(terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAggregatePrevoteMethod(), responseObserver);
}
/**
*
* AggregatePrevotes returns aggregate prevotes of all validators
*
*/
default void aggregatePrevotes(terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAggregatePrevotesMethod(), responseObserver);
}
/**
*
* AggregateVote returns an aggregate vote of a validator
*
*/
default void aggregateVote(terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAggregateVoteMethod(), responseObserver);
}
/**
*
* AggregateVotes returns aggregate votes of all validators
*
*/
default void aggregateVotes(terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAggregateVotesMethod(), responseObserver);
}
/**
*
* Params queries all parameters.
*
*/
default void params(terra.oracle.v1beta1.QueryOuterClass.QueryParamsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getParamsMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service Query.
*
* Query defines the gRPC querier service.
*
*/
public static abstract class QueryImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return QueryGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service Query.
*
* Query defines the gRPC querier service.
*
*/
public static final class QueryStub
extends io.grpc.stub.AbstractAsyncStub {
private QueryStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected QueryStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new QueryStub(channel, callOptions);
}
/**
*
* ExchangeRate returns exchange rate of a denom
*
*/
public void exchangeRate(terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExchangeRateMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* ExchangeRates returns exchange rates of all denoms
*
*/
public void exchangeRates(terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExchangeRatesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* TobinTax returns tobin tax of a denom
*
*/
public void tobinTax(terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getTobinTaxMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* TobinTaxes returns tobin taxes of all denoms
*
*/
public void tobinTaxes(terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getTobinTaxesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Actives returns all active denoms
*
*/
public void actives(terra.oracle.v1beta1.QueryOuterClass.QueryActivesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getActivesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* VoteTargets returns all vote target denoms
*
*/
public void voteTargets(terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getVoteTargetsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* FeederDelegation returns feeder delegation of a validator
*
*/
public void feederDelegation(terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getFeederDelegationMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* MissCounter returns oracle miss counter of a validator
*
*/
public void missCounter(terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getMissCounterMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* AggregatePrevote returns an aggregate prevote of a validator
*
*/
public void aggregatePrevote(terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAggregatePrevoteMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* AggregatePrevotes returns aggregate prevotes of all validators
*
*/
public void aggregatePrevotes(terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAggregatePrevotesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* AggregateVote returns an aggregate vote of a validator
*
*/
public void aggregateVote(terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAggregateVoteMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* AggregateVotes returns aggregate votes of all validators
*
*/
public void aggregateVotes(terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAggregateVotesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Params queries all parameters.
*
*/
public void params(terra.oracle.v1beta1.QueryOuterClass.QueryParamsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getParamsMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service Query.
*
* Query defines the gRPC querier service.
*
*/
public static final class QueryBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private QueryBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected QueryBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new QueryBlockingStub(channel, callOptions);
}
/**
*
* ExchangeRate returns exchange rate of a denom
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateResponse exchangeRate(terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExchangeRateMethod(), getCallOptions(), request);
}
/**
*
* ExchangeRates returns exchange rates of all denoms
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesResponse exchangeRates(terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExchangeRatesMethod(), getCallOptions(), request);
}
/**
*
* TobinTax returns tobin tax of a denom
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxResponse tobinTax(terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getTobinTaxMethod(), getCallOptions(), request);
}
/**
*
* TobinTaxes returns tobin taxes of all denoms
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesResponse tobinTaxes(terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getTobinTaxesMethod(), getCallOptions(), request);
}
/**
*
* Actives returns all active denoms
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryActivesResponse actives(terra.oracle.v1beta1.QueryOuterClass.QueryActivesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getActivesMethod(), getCallOptions(), request);
}
/**
*
* VoteTargets returns all vote target denoms
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsResponse voteTargets(terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getVoteTargetsMethod(), getCallOptions(), request);
}
/**
*
* FeederDelegation returns feeder delegation of a validator
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationResponse feederDelegation(terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getFeederDelegationMethod(), getCallOptions(), request);
}
/**
*
* MissCounter returns oracle miss counter of a validator
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterResponse missCounter(terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getMissCounterMethod(), getCallOptions(), request);
}
/**
*
* AggregatePrevote returns an aggregate prevote of a validator
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteResponse aggregatePrevote(terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAggregatePrevoteMethod(), getCallOptions(), request);
}
/**
*
* AggregatePrevotes returns aggregate prevotes of all validators
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesResponse aggregatePrevotes(terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAggregatePrevotesMethod(), getCallOptions(), request);
}
/**
*
* AggregateVote returns an aggregate vote of a validator
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteResponse aggregateVote(terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAggregateVoteMethod(), getCallOptions(), request);
}
/**
*
* AggregateVotes returns aggregate votes of all validators
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesResponse aggregateVotes(terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAggregateVotesMethod(), getCallOptions(), request);
}
/**
*
* Params queries all parameters.
*
*/
public terra.oracle.v1beta1.QueryOuterClass.QueryParamsResponse params(terra.oracle.v1beta1.QueryOuterClass.QueryParamsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getParamsMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service Query.
*
* Query defines the gRPC querier service.
*
*/
public static final class QueryFutureStub
extends io.grpc.stub.AbstractFutureStub {
private QueryFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected QueryFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new QueryFutureStub(channel, callOptions);
}
/**
*
* ExchangeRate returns exchange rate of a denom
*
*/
public com.google.common.util.concurrent.ListenableFuture exchangeRate(
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExchangeRateMethod(), getCallOptions()), request);
}
/**
*
* ExchangeRates returns exchange rates of all denoms
*
*/
public com.google.common.util.concurrent.ListenableFuture exchangeRates(
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExchangeRatesMethod(), getCallOptions()), request);
}
/**
*
* TobinTax returns tobin tax of a denom
*
*/
public com.google.common.util.concurrent.ListenableFuture tobinTax(
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getTobinTaxMethod(), getCallOptions()), request);
}
/**
*
* TobinTaxes returns tobin taxes of all denoms
*
*/
public com.google.common.util.concurrent.ListenableFuture tobinTaxes(
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getTobinTaxesMethod(), getCallOptions()), request);
}
/**
*
* Actives returns all active denoms
*
*/
public com.google.common.util.concurrent.ListenableFuture actives(
terra.oracle.v1beta1.QueryOuterClass.QueryActivesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getActivesMethod(), getCallOptions()), request);
}
/**
*
* VoteTargets returns all vote target denoms
*
*/
public com.google.common.util.concurrent.ListenableFuture voteTargets(
terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getVoteTargetsMethod(), getCallOptions()), request);
}
/**
*
* FeederDelegation returns feeder delegation of a validator
*
*/
public com.google.common.util.concurrent.ListenableFuture feederDelegation(
terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getFeederDelegationMethod(), getCallOptions()), request);
}
/**
*
* MissCounter returns oracle miss counter of a validator
*
*/
public com.google.common.util.concurrent.ListenableFuture missCounter(
terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getMissCounterMethod(), getCallOptions()), request);
}
/**
*
* AggregatePrevote returns an aggregate prevote of a validator
*
*/
public com.google.common.util.concurrent.ListenableFuture aggregatePrevote(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAggregatePrevoteMethod(), getCallOptions()), request);
}
/**
*
* AggregatePrevotes returns aggregate prevotes of all validators
*
*/
public com.google.common.util.concurrent.ListenableFuture aggregatePrevotes(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAggregatePrevotesMethod(), getCallOptions()), request);
}
/**
*
* AggregateVote returns an aggregate vote of a validator
*
*/
public com.google.common.util.concurrent.ListenableFuture aggregateVote(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAggregateVoteMethod(), getCallOptions()), request);
}
/**
*
* AggregateVotes returns aggregate votes of all validators
*
*/
public com.google.common.util.concurrent.ListenableFuture aggregateVotes(
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAggregateVotesMethod(), getCallOptions()), request);
}
/**
*
* Params queries all parameters.
*
*/
public com.google.common.util.concurrent.ListenableFuture params(
terra.oracle.v1beta1.QueryOuterClass.QueryParamsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getParamsMethod(), getCallOptions()), request);
}
}
private static final int METHODID_EXCHANGE_RATE = 0;
private static final int METHODID_EXCHANGE_RATES = 1;
private static final int METHODID_TOBIN_TAX = 2;
private static final int METHODID_TOBIN_TAXES = 3;
private static final int METHODID_ACTIVES = 4;
private static final int METHODID_VOTE_TARGETS = 5;
private static final int METHODID_FEEDER_DELEGATION = 6;
private static final int METHODID_MISS_COUNTER = 7;
private static final int METHODID_AGGREGATE_PREVOTE = 8;
private static final int METHODID_AGGREGATE_PREVOTES = 9;
private static final int METHODID_AGGREGATE_VOTE = 10;
private static final int METHODID_AGGREGATE_VOTES = 11;
private static final int METHODID_PARAMS = 12;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_EXCHANGE_RATE:
serviceImpl.exchangeRate((terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXCHANGE_RATES:
serviceImpl.exchangeRates((terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_TOBIN_TAX:
serviceImpl.tobinTax((terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_TOBIN_TAXES:
serviceImpl.tobinTaxes((terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_ACTIVES:
serviceImpl.actives((terra.oracle.v1beta1.QueryOuterClass.QueryActivesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_VOTE_TARGETS:
serviceImpl.voteTargets((terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_FEEDER_DELEGATION:
serviceImpl.feederDelegation((terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_MISS_COUNTER:
serviceImpl.missCounter((terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_AGGREGATE_PREVOTE:
serviceImpl.aggregatePrevote((terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_AGGREGATE_PREVOTES:
serviceImpl.aggregatePrevotes((terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_AGGREGATE_VOTE:
serviceImpl.aggregateVote((terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_AGGREGATE_VOTES:
serviceImpl.aggregateVotes((terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PARAMS:
serviceImpl.params((terra.oracle.v1beta1.QueryOuterClass.QueryParamsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getExchangeRateMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRateResponse>(
service, METHODID_EXCHANGE_RATE)))
.addMethod(
getExchangeRatesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryExchangeRatesResponse>(
service, METHODID_EXCHANGE_RATES)))
.addMethod(
getTobinTaxMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxResponse>(
service, METHODID_TOBIN_TAX)))
.addMethod(
getTobinTaxesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryTobinTaxesResponse>(
service, METHODID_TOBIN_TAXES)))
.addMethod(
getActivesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryActivesRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryActivesResponse>(
service, METHODID_ACTIVES)))
.addMethod(
getVoteTargetsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryVoteTargetsResponse>(
service, METHODID_VOTE_TARGETS)))
.addMethod(
getFeederDelegationMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryFeederDelegationResponse>(
service, METHODID_FEEDER_DELEGATION)))
.addMethod(
getMissCounterMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryMissCounterResponse>(
service, METHODID_MISS_COUNTER)))
.addMethod(
getAggregatePrevoteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevoteResponse>(
service, METHODID_AGGREGATE_PREVOTE)))
.addMethod(
getAggregatePrevotesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryAggregatePrevotesResponse>(
service, METHODID_AGGREGATE_PREVOTES)))
.addMethod(
getAggregateVoteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVoteResponse>(
service, METHODID_AGGREGATE_VOTE)))
.addMethod(
getAggregateVotesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryAggregateVotesResponse>(
service, METHODID_AGGREGATE_VOTES)))
.addMethod(
getParamsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
terra.oracle.v1beta1.QueryOuterClass.QueryParamsRequest,
terra.oracle.v1beta1.QueryOuterClass.QueryParamsResponse>(
service, METHODID_PARAMS)))
.build();
}
private static abstract class QueryBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
QueryBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return terra.oracle.v1beta1.QueryOuterClass.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Query");
}
}
private static final class QueryFileDescriptorSupplier
extends QueryBaseDescriptorSupplier {
QueryFileDescriptorSupplier() {}
}
private static final class QueryMethodDescriptorSupplier
extends QueryBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
QueryMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (QueryGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new QueryFileDescriptorSupplier())
.addMethod(getExchangeRateMethod())
.addMethod(getExchangeRatesMethod())
.addMethod(getTobinTaxMethod())
.addMethod(getTobinTaxesMethod())
.addMethod(getActivesMethod())
.addMethod(getVoteTargetsMethod())
.addMethod(getFeederDelegationMethod())
.addMethod(getMissCounterMethod())
.addMethod(getAggregatePrevoteMethod())
.addMethod(getAggregatePrevotesMethod())
.addMethod(getAggregateVoteMethod())
.addMethod(getAggregateVotesMethod())
.addMethod(getParamsMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy