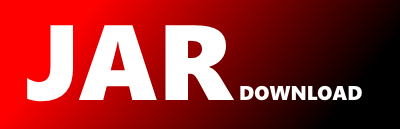
kr.jm.metric.data.Transfer Maven / Gradle / Ivy
package kr.jm.metric.data;
import lombok.AccessLevel;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.ToString;
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
@Getter
@ToString
@NoArgsConstructor(access = AccessLevel.PROTECTED)
public class Transfer {
protected String inputId;
protected T data;
protected long timestamp;
protected Map meta;
public Transfer(String inputId, T data) {
this(inputId, data, null);
}
public Transfer(String inputId, T data, long timestamp) {
this(inputId, data, timestamp, null);
}
public Transfer(String inputId, T data, Map meta) {
this(inputId, data, System.currentTimeMillis(), meta);
}
public Transfer(String inputId, T data, long timestamp,
Map meta) {
this.inputId = inputId;
this.data = data;
this.timestamp = timestamp;
this.meta = Optional.ofNullable(meta).orElseGet(Map::of);
}
public Map getMeta() {
return Objects.requireNonNullElseGet(this.meta,
() -> this.meta = new HashMap<>());
}
public Transfer newWith(D data) {
return newWith(data, this.meta);
}
public Transfer newWith(D data, long timestamp) {
return newWith(data, timestamp, this.meta);
}
public Transfer newWith(D data, Map meta) {
return newWith(data, this.timestamp, meta);
}
public Transfer newWith(D data, long timestamp,
Map meta) {
return new Transfer<>(this.inputId, data, timestamp, meta);
}
public List> newListWith(List dataList) {
return newStreamWith(dataList).collect(Collectors.toList());
}
public Stream> newStreamWith(
List dataList) {
return dataList.stream().map(this::newWith);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy