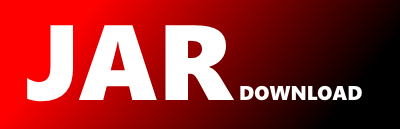
kr.motd.maven.sphinx.dist.lxml.builder$py.class Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sphinx-maven-plugin Show documentation
Show all versions of sphinx-maven-plugin Show documentation
Maven plugin that creates the site with Sphinx
???? 1? f$0 R(Lorg/python/core/PyFrame;Lorg/python/core/ThreadState;)Lorg/python/core/PyObject; __doc__ 9
The ``E`` Element factory for generating XML documents.
org/python/core/PyString fromInterned .(Ljava/lang/String;)Lorg/python/core/PyString;
org/python/core/PyFrame
setglobal /(Ljava/lang/String;Lorg/python/core/PyObject;)V
setline (I)V
lxml.etree org/python/core/imp importOneAs H(Ljava/lang/String;Lorg/python/core/PyFrame;I)Lorg/python/core/PyObject;
ET setlocal !
" functools $ java/lang/String & partial (
importFrom \(Ljava/lang/String;[Ljava/lang/String;Lorg/python/core/PyFrame;I)[Lorg/python/core/PyObject; * +
,
basestring . getname .(Ljava/lang/String;)Lorg/python/core/PyObject; 0 1
2 org/python/core/Py 4 setException M(Ljava/lang/Throwable;Lorg/python/core/PyFrame;)Lorg/python/core/PyException; 6 7
5 8 NameError : org/python/core/PyException < match (Lorg/python/core/PyObject;)Z > ?
= @ str B java/lang/Throwable D unicode F org/python/core/PyObject H object J ElementMaker L ElementMaker$1
__module__ O __name__ QcElement generator factory.
Unlike the ordinary Element factory, the E factory allows you to pass in
more than just a tag and some optional attributes; you can also pass in
text and other elements. The text is added as either text or tail
attributes, and elements are inserted at the right spot. Some small
examples::
>>> from lxml import etree as ET
>>> from lxml.builder import E
>>> ET.tostring(E("tag"))
' '
>>> ET.tostring(E("tag", "text"))
'text '
>>> ET.tostring(E("tag", "text", key="value"))
'text '
>>> ET.tostring(E("tag", E("subtag", "text"), "tail"))
'text tail '
For simple tags, the factory also allows you to write ``E.tag(...)`` instead
of ``E('tag', ...)``::
>>> ET.tostring(E.tag())
' '
>>> ET.tostring(E.tag("text"))
'text '
>>> ET.tostring(E.tag(E.subtag("text"), "tail"))
'text tail '
Here's a somewhat larger example; this shows how to generate HTML
documents, using a mix of prepared factory functions for inline elements,
nested ``E.tag`` calls, and embedded XHTML fragments::
# some common inline elements
A = E.a
I = E.i
B = E.b
def CLASS(v):
# helper function, 'class' is a reserved word
return {'class': v}
page = (
E.html(
E.head(
E.title("This is a sample document")
),
E.body(
E.h1("Hello!", CLASS("title")),
E.p("This is a paragraph with ", B("bold"), " text in it!"),
E.p("This is another paragraph, with a ",
A("link", href="http://www.python.org"), "."),
E.p("Here are some reserved characters: ."),
ET.XML("And finally, here is an embedded XHTML fragment.
"),
)
)
)
print ET.tostring(page)
Here's a prettyprinted version of the output from the above script::
This is a sample document
Hello!
This is a paragraph with bold text in it!
This is another paragraph, with link.
Here are some reserved characters: <spam&egg>.
And finally, here is an embedded XHTML fragment.
For namespace support, you can pass a namespace map (``nsmap``)
and/or a specific target ``namespace`` to the ElementMaker class::
>>> E = ElementMaker(namespace="http://my.ns/")
>>> print(ET.tostring( E.test ))
>>> E = ElementMaker(namespace="http://my.ns/", nsmap={'p':'http://my.ns/'})
>>> print(ET.tostring( E.test ))
S None U org/python/core/PyFunction W f_globals Lorg/python/core/PyObject; Y Z [
__init__$2 to_cell (II)V ^ _
` getlocal (I)Lorg/python/core/PyObject; b c
d getglobal f 1
g _isnot 6(Lorg/python/core/PyObject;)Lorg/python/core/PyObject; i j
I k __nonzero__ ()Z m n
I o { q _add s j
I t } v
_namespace x __setattr__ z
I { dict } __call__ S(Lorg/python/core/ThreadState;Lorg/python/core/PyObject;)Lorg/python/core/PyObject; ?
I ? _nsmap ? __debug__ ? callable ? U Z 5 ? AssertionError ?
makeException S(Lorg/python/core/PyObject;Lorg/python/core/PyObject;)Lorg/python/core/PyException; ? ?
5 ? _makeelement ? Element ? __getattr__ ? 1
I ? getderef ? c
? setderef (ILorg/python/core/PyObject;)V ? ?
? org/python/core/PyDictionary ? EmptyObjects [Lorg/python/core/PyObject; ? ? 5 ? ([Lorg/python/core/PyObject;)V ? ?
? ?
add_text$3
newInteger (I)Lorg/python/core/PyInteger; ? ?
5 ? __getitem__ ? j
I ? tail ? ?
IndexError ? text ? f_lasti I ? ? ? lxml/builder$py ? Lorg/python/core/PyCode; ? ? ? ? j(Lorg/python/core/PyObject;[Lorg/python/core/PyObject;Lorg/python/core/PyCode;Lorg/python/core/PyObject;)V ? ?
X ? ! ?
? add_cdata$4
ValueError ?