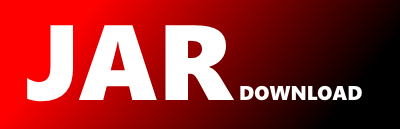
com.sforce.soap.partner.SingleEmailMessage Maven / Gradle / Ivy
package com.sforce.soap.partner;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for SingleEmailMessage complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="SingleEmailMessage">
* <complexContent>
* <extension base="{urn:partner.soap.sforce.com}Email">
* <sequence>
* <element name="bccAddresses" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="25" minOccurs="0"/>
* <element name="ccAddresses" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="25" minOccurs="0"/>
* <element name="charset" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="documentAttachments" type="{urn:partner.soap.sforce.com}ID" maxOccurs="unbounded" minOccurs="0"/>
* <element name="entityAttachments" type="{urn:partner.soap.sforce.com}ID" maxOccurs="unbounded" minOccurs="0"/>
* <element name="fileAttachments" type="{urn:partner.soap.sforce.com}EmailFileAttachment" maxOccurs="unbounded" minOccurs="0"/>
* <element name="htmlBody" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="inReplyTo" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="optOutPolicy" type="{urn:partner.soap.sforce.com}SendEmailOptOutPolicy"/>
* <element name="orgWideEmailAddressId" type="{urn:partner.soap.sforce.com}ID" minOccurs="0"/>
* <element name="plainTextBody" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="references" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="targetObjectId" type="{urn:partner.soap.sforce.com}ID"/>
* <element name="templateId" type="{urn:partner.soap.sforce.com}ID"/>
* <element name="toAddresses" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="100" minOccurs="0"/>
* <element name="treatBodiesAsTemplate" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="treatTargetObjectAsRecipient" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="whatId" type="{urn:partner.soap.sforce.com}ID"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SingleEmailMessage", propOrder = {
"bccAddresses",
"ccAddresses",
"charset",
"documentAttachments",
"entityAttachments",
"fileAttachments",
"htmlBody",
"inReplyTo",
"optOutPolicy",
"orgWideEmailAddressId",
"plainTextBody",
"references",
"targetObjectId",
"templateId",
"toAddresses",
"treatBodiesAsTemplate",
"treatTargetObjectAsRecipient",
"whatId"
})
public class SingleEmailMessage
extends Email
{
@XmlElement(nillable = true)
protected List bccAddresses;
@XmlElement(nillable = true)
protected List ccAddresses;
@XmlElement(required = true, nillable = true)
protected String charset;
protected List documentAttachments;
protected List entityAttachments;
protected List fileAttachments;
@XmlElement(required = true, nillable = true)
protected String htmlBody;
@XmlElement(nillable = true)
protected String inReplyTo;
@XmlElement(required = true, nillable = true)
@XmlSchemaType(name = "string")
protected SendEmailOptOutPolicy optOutPolicy;
@XmlElement(nillable = true)
protected String orgWideEmailAddressId;
@XmlElement(required = true, nillable = true)
protected String plainTextBody;
@XmlElement(nillable = true)
protected String references;
@XmlElement(required = true, nillable = true)
protected String targetObjectId;
@XmlElement(required = true, nillable = true)
protected String templateId;
@XmlElement(nillable = true)
protected List toAddresses;
@XmlElement(required = true, type = Boolean.class, nillable = true)
protected Boolean treatBodiesAsTemplate;
@XmlElement(required = true, type = Boolean.class, nillable = true)
protected Boolean treatTargetObjectAsRecipient;
@XmlElement(required = true, nillable = true)
protected String whatId;
/**
* Gets the value of the bccAddresses property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the bccAddresses property.
*
*
* For example, to add a new item, do as follows:
*
* getBccAddresses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getBccAddresses() {
if (bccAddresses == null) {
bccAddresses = new ArrayList();
}
return this.bccAddresses;
}
/**
* Gets the value of the ccAddresses property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ccAddresses property.
*
*
* For example, to add a new item, do as follows:
*
* getCcAddresses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getCcAddresses() {
if (ccAddresses == null) {
ccAddresses = new ArrayList();
}
return this.ccAddresses;
}
/**
* Gets the value of the charset property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCharset() {
return charset;
}
/**
* Sets the value of the charset property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCharset(String value) {
this.charset = value;
}
/**
* Gets the value of the documentAttachments property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the documentAttachments property.
*
*
* For example, to add a new item, do as follows:
*
* getDocumentAttachments().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getDocumentAttachments() {
if (documentAttachments == null) {
documentAttachments = new ArrayList();
}
return this.documentAttachments;
}
/**
* Gets the value of the entityAttachments property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the entityAttachments property.
*
*
* For example, to add a new item, do as follows:
*
* getEntityAttachments().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getEntityAttachments() {
if (entityAttachments == null) {
entityAttachments = new ArrayList();
}
return this.entityAttachments;
}
/**
* Gets the value of the fileAttachments property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the fileAttachments property.
*
*
* For example, to add a new item, do as follows:
*
* getFileAttachments().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link EmailFileAttachment }
*
*
*/
public List getFileAttachments() {
if (fileAttachments == null) {
fileAttachments = new ArrayList();
}
return this.fileAttachments;
}
/**
* Gets the value of the htmlBody property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHtmlBody() {
return htmlBody;
}
/**
* Sets the value of the htmlBody property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHtmlBody(String value) {
this.htmlBody = value;
}
/**
* Gets the value of the inReplyTo property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getInReplyTo() {
return inReplyTo;
}
/**
* Sets the value of the inReplyTo property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setInReplyTo(String value) {
this.inReplyTo = value;
}
/**
* Gets the value of the optOutPolicy property.
*
* @return
* possible object is
* {@link SendEmailOptOutPolicy }
*
*/
public SendEmailOptOutPolicy getOptOutPolicy() {
return optOutPolicy;
}
/**
* Sets the value of the optOutPolicy property.
*
* @param value
* allowed object is
* {@link SendEmailOptOutPolicy }
*
*/
public void setOptOutPolicy(SendEmailOptOutPolicy value) {
this.optOutPolicy = value;
}
/**
* Gets the value of the orgWideEmailAddressId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrgWideEmailAddressId() {
return orgWideEmailAddressId;
}
/**
* Sets the value of the orgWideEmailAddressId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrgWideEmailAddressId(String value) {
this.orgWideEmailAddressId = value;
}
/**
* Gets the value of the plainTextBody property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPlainTextBody() {
return plainTextBody;
}
/**
* Sets the value of the plainTextBody property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPlainTextBody(String value) {
this.plainTextBody = value;
}
/**
* Gets the value of the references property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReferences() {
return references;
}
/**
* Sets the value of the references property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReferences(String value) {
this.references = value;
}
/**
* Gets the value of the targetObjectId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTargetObjectId() {
return targetObjectId;
}
/**
* Sets the value of the targetObjectId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTargetObjectId(String value) {
this.targetObjectId = value;
}
/**
* Gets the value of the templateId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTemplateId() {
return templateId;
}
/**
* Sets the value of the templateId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTemplateId(String value) {
this.templateId = value;
}
/**
* Gets the value of the toAddresses property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the toAddresses property.
*
*
* For example, to add a new item, do as follows:
*
* getToAddresses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getToAddresses() {
if (toAddresses == null) {
toAddresses = new ArrayList();
}
return this.toAddresses;
}
/**
* Gets the value of the treatBodiesAsTemplate property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTreatBodiesAsTemplate() {
return treatBodiesAsTemplate;
}
/**
* Sets the value of the treatBodiesAsTemplate property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTreatBodiesAsTemplate(Boolean value) {
this.treatBodiesAsTemplate = value;
}
/**
* Gets the value of the treatTargetObjectAsRecipient property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTreatTargetObjectAsRecipient() {
return treatTargetObjectAsRecipient;
}
/**
* Sets the value of the treatTargetObjectAsRecipient property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTreatTargetObjectAsRecipient(Boolean value) {
this.treatTargetObjectAsRecipient = value;
}
/**
* Gets the value of the whatId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWhatId() {
return whatId;
}
/**
* Sets the value of the whatId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWhatId(String value) {
this.whatId = value;
}
}