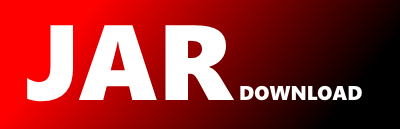
com.sforce.soap.tooling.RelationshipInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sforce-tooling-api Show documentation
Show all versions of sforce-tooling-api Show documentation
Salesforce Tooling API client
The newest version!
package com.sforce.soap.tooling;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for RelationshipInfo complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="RelationshipInfo">
* <complexContent>
* <extension base="{urn:tooling.soap.sforce.com}sObject">
* <sequence>
* <element name="ChildSobject" type="{urn:tooling.soap.sforce.com}EntityDefinition" minOccurs="0"/>
* <element name="ChildSobjectId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="DurableId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Field" type="{urn:tooling.soap.sforce.com}FieldDefinition" minOccurs="0"/>
* <element name="FieldId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="IsCascadeDelete" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="IsDeprecatedAndHidden" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="IsRestrictedDelete" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="JunctionIdListName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="RelationshipDomains" type="{urn:tooling.soap.sforce.com}QueryResult" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "RelationshipInfo", propOrder = {
"childSobject",
"childSobjectId",
"durableId",
"field",
"fieldId",
"isCascadeDelete",
"isDeprecatedAndHidden",
"isRestrictedDelete",
"junctionIdListName",
"relationshipDomains"
})
public class RelationshipInfo
extends SObject
{
@XmlElement(name = "ChildSobject", nillable = true)
protected EntityDefinition childSobject;
@XmlElement(name = "ChildSobjectId", nillable = true)
protected String childSobjectId;
@XmlElement(name = "DurableId", nillable = true)
protected String durableId;
@XmlElement(name = "Field", nillable = true)
protected FieldDefinition field;
@XmlElement(name = "FieldId", nillable = true)
protected String fieldId;
@XmlElement(name = "IsCascadeDelete", nillable = true)
protected Boolean isCascadeDelete;
@XmlElement(name = "IsDeprecatedAndHidden", nillable = true)
protected Boolean isDeprecatedAndHidden;
@XmlElement(name = "IsRestrictedDelete", nillable = true)
protected Boolean isRestrictedDelete;
@XmlElement(name = "JunctionIdListName", nillable = true)
protected String junctionIdListName;
@XmlElement(name = "RelationshipDomains", nillable = true)
protected QueryResult relationshipDomains;
/**
* Gets the value of the childSobject property.
*
* @return
* possible object is
* {@link EntityDefinition }
*
*/
public EntityDefinition getChildSobject() {
return childSobject;
}
/**
* Sets the value of the childSobject property.
*
* @param value
* allowed object is
* {@link EntityDefinition }
*
*/
public void setChildSobject(EntityDefinition value) {
this.childSobject = value;
}
/**
* Gets the value of the childSobjectId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getChildSobjectId() {
return childSobjectId;
}
/**
* Sets the value of the childSobjectId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setChildSobjectId(String value) {
this.childSobjectId = value;
}
/**
* Gets the value of the durableId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDurableId() {
return durableId;
}
/**
* Sets the value of the durableId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDurableId(String value) {
this.durableId = value;
}
/**
* Gets the value of the field property.
*
* @return
* possible object is
* {@link FieldDefinition }
*
*/
public FieldDefinition getField() {
return field;
}
/**
* Sets the value of the field property.
*
* @param value
* allowed object is
* {@link FieldDefinition }
*
*/
public void setField(FieldDefinition value) {
this.field = value;
}
/**
* Gets the value of the fieldId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFieldId() {
return fieldId;
}
/**
* Sets the value of the fieldId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFieldId(String value) {
this.fieldId = value;
}
/**
* Gets the value of the isCascadeDelete property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsCascadeDelete() {
return isCascadeDelete;
}
/**
* Sets the value of the isCascadeDelete property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsCascadeDelete(Boolean value) {
this.isCascadeDelete = value;
}
/**
* Gets the value of the isDeprecatedAndHidden property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsDeprecatedAndHidden() {
return isDeprecatedAndHidden;
}
/**
* Sets the value of the isDeprecatedAndHidden property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsDeprecatedAndHidden(Boolean value) {
this.isDeprecatedAndHidden = value;
}
/**
* Gets the value of the isRestrictedDelete property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsRestrictedDelete() {
return isRestrictedDelete;
}
/**
* Sets the value of the isRestrictedDelete property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsRestrictedDelete(Boolean value) {
this.isRestrictedDelete = value;
}
/**
* Gets the value of the junctionIdListName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJunctionIdListName() {
return junctionIdListName;
}
/**
* Sets the value of the junctionIdListName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJunctionIdListName(String value) {
this.junctionIdListName = value;
}
/**
* Gets the value of the relationshipDomains property.
*
* @return
* possible object is
* {@link QueryResult }
*
*/
public QueryResult getRelationshipDomains() {
return relationshipDomains;
}
/**
* Sets the value of the relationshipDomains property.
*
* @param value
* allowed object is
* {@link QueryResult }
*
*/
public void setRelationshipDomains(QueryResult value) {
this.relationshipDomains = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy