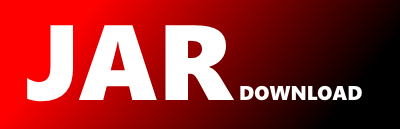
com.sforce.soap.tooling.RunTestFailure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sforce-tooling-api Show documentation
Show all versions of sforce-tooling-api Show documentation
Salesforce Tooling API client
The newest version!
package com.sforce.soap.tooling;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for RunTestFailure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="RunTestFailure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{urn:tooling.soap.sforce.com}ID"/>
* <element name="message" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="methodName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="namespace" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="seeAllData" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="stackTrace" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="time" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="type" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "RunTestFailure", propOrder = {
"id",
"message",
"methodName",
"name",
"namespace",
"seeAllData",
"stackTrace",
"time",
"type"
})
public class RunTestFailure {
@XmlElement(required = true)
protected String id;
@XmlElement(required = true)
protected String message;
@XmlElement(required = true, nillable = true)
protected String methodName;
@XmlElement(required = true)
protected String name;
@XmlElement(required = true, nillable = true)
protected String namespace;
protected Boolean seeAllData;
@XmlElement(required = true, nillable = true)
protected String stackTrace;
protected double time;
@XmlElement(required = true)
protected String type;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the message property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMessage() {
return message;
}
/**
* Sets the value of the message property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMessage(String value) {
this.message = value;
}
/**
* Gets the value of the methodName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMethodName() {
return methodName;
}
/**
* Sets the value of the methodName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMethodName(String value) {
this.methodName = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the namespace property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNamespace() {
return namespace;
}
/**
* Sets the value of the namespace property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNamespace(String value) {
this.namespace = value;
}
/**
* Gets the value of the seeAllData property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isSeeAllData() {
return seeAllData;
}
/**
* Sets the value of the seeAllData property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setSeeAllData(Boolean value) {
this.seeAllData = value;
}
/**
* Gets the value of the stackTrace property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStackTrace() {
return stackTrace;
}
/**
* Sets the value of the stackTrace property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStackTrace(String value) {
this.stackTrace = value;
}
/**
* Gets the value of the time property.
*
*/
public double getTime() {
return time;
}
/**
* Sets the value of the time property.
*
*/
public void setTime(double value) {
this.time = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setType(String value) {
this.type = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy