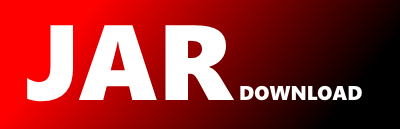
com.sforce.soap.tooling.RunTestsResult Maven / Gradle / Ivy
Show all versions of sforce-tooling-api Show documentation
package com.sforce.soap.tooling;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for RunTestsResult complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="RunTestsResult">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="apexLogId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="codeCoverage" type="{urn:tooling.soap.sforce.com}CodeCoverageResult" maxOccurs="unbounded" minOccurs="0"/>
* <element name="codeCoverageWarnings" type="{urn:tooling.soap.sforce.com}CodeCoverageWarning" maxOccurs="unbounded" minOccurs="0"/>
* <element name="failures" type="{urn:tooling.soap.sforce.com}RunTestFailure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="numFailures" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="numTestsRun" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="successes" type="{urn:tooling.soap.sforce.com}RunTestSuccess" maxOccurs="unbounded" minOccurs="0"/>
* <element name="totalTime" type="{http://www.w3.org/2001/XMLSchema}double"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "RunTestsResult", propOrder = {
"apexLogId",
"codeCoverage",
"codeCoverageWarnings",
"failures",
"numFailures",
"numTestsRun",
"successes",
"totalTime"
})
public class RunTestsResult {
protected String apexLogId;
protected List codeCoverage;
protected List codeCoverageWarnings;
protected List failures;
protected int numFailures;
protected int numTestsRun;
protected List successes;
protected double totalTime;
/**
* Gets the value of the apexLogId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getApexLogId() {
return apexLogId;
}
/**
* Sets the value of the apexLogId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setApexLogId(String value) {
this.apexLogId = value;
}
/**
* Gets the value of the codeCoverage property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the codeCoverage property.
*
*
* For example, to add a new item, do as follows:
*
* getCodeCoverage().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CodeCoverageResult }
*
*
*/
public List getCodeCoverage() {
if (codeCoverage == null) {
codeCoverage = new ArrayList();
}
return this.codeCoverage;
}
/**
* Gets the value of the codeCoverageWarnings property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the codeCoverageWarnings property.
*
*
* For example, to add a new item, do as follows:
*
* getCodeCoverageWarnings().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CodeCoverageWarning }
*
*
*/
public List getCodeCoverageWarnings() {
if (codeCoverageWarnings == null) {
codeCoverageWarnings = new ArrayList();
}
return this.codeCoverageWarnings;
}
/**
* Gets the value of the failures property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the failures property.
*
*
* For example, to add a new item, do as follows:
*
* getFailures().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RunTestFailure }
*
*
*/
public List getFailures() {
if (failures == null) {
failures = new ArrayList();
}
return this.failures;
}
/**
* Gets the value of the numFailures property.
*
*/
public int getNumFailures() {
return numFailures;
}
/**
* Sets the value of the numFailures property.
*
*/
public void setNumFailures(int value) {
this.numFailures = value;
}
/**
* Gets the value of the numTestsRun property.
*
*/
public int getNumTestsRun() {
return numTestsRun;
}
/**
* Sets the value of the numTestsRun property.
*
*/
public void setNumTestsRun(int value) {
this.numTestsRun = value;
}
/**
* Gets the value of the successes property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the successes property.
*
*
* For example, to add a new item, do as follows:
*
* getSuccesses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RunTestSuccess }
*
*
*/
public List getSuccesses() {
if (successes == null) {
successes = new ArrayList();
}
return this.successes;
}
/**
* Gets the value of the totalTime property.
*
*/
public double getTotalTime() {
return totalTime;
}
/**
* Sets the value of the totalTime property.
*
*/
public void setTotalTime(double value) {
this.totalTime = value;
}
}