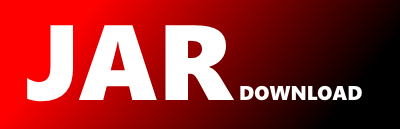
com.sforce.soap.tooling.Scontrol Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sforce-tooling-api Show documentation
Show all versions of sforce-tooling-api Show documentation
Salesforce Tooling API client
The newest version!
package com.sforce.soap.tooling;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for Scontrol complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Scontrol">
* <complexContent>
* <extension base="{urn:tooling.soap.sforce.com}sObject">
* <sequence>
* <element name="ContentSource" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="CreatedBy" type="{urn:tooling.soap.sforce.com}User" minOccurs="0"/>
* <element name="CreatedById" type="{urn:tooling.soap.sforce.com}ID" minOccurs="0"/>
* <element name="CreatedDate" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="Description" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="DeveloperName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="EncodingKey" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="LastModifiedBy" type="{urn:tooling.soap.sforce.com}User" minOccurs="0"/>
* <element name="LastModifiedById" type="{urn:tooling.soap.sforce.com}ID" minOccurs="0"/>
* <element name="LastModifiedDate" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="ManageableState" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Name" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="NamespacePrefix" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="SupportsCaching" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Scontrol", propOrder = {
"contentSource",
"createdBy",
"createdById",
"createdDate",
"description",
"developerName",
"encodingKey",
"lastModifiedBy",
"lastModifiedById",
"lastModifiedDate",
"manageableState",
"name",
"namespacePrefix",
"supportsCaching"
})
public class Scontrol
extends SObject
{
@XmlElement(name = "ContentSource", nillable = true)
protected String contentSource;
@XmlElement(name = "CreatedBy", nillable = true)
protected User createdBy;
@XmlElement(name = "CreatedById", nillable = true)
protected String createdById;
@XmlElement(name = "CreatedDate", nillable = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar createdDate;
@XmlElement(name = "Description", nillable = true)
protected String description;
@XmlElement(name = "DeveloperName", nillable = true)
protected String developerName;
@XmlElement(name = "EncodingKey", nillable = true)
protected String encodingKey;
@XmlElement(name = "LastModifiedBy", nillable = true)
protected User lastModifiedBy;
@XmlElement(name = "LastModifiedById", nillable = true)
protected String lastModifiedById;
@XmlElement(name = "LastModifiedDate", nillable = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar lastModifiedDate;
@XmlElement(name = "ManageableState", nillable = true)
protected String manageableState;
@XmlElement(name = "Name", nillable = true)
protected String name;
@XmlElement(name = "NamespacePrefix", nillable = true)
protected String namespacePrefix;
@XmlElement(name = "SupportsCaching", nillable = true)
protected Boolean supportsCaching;
/**
* Gets the value of the contentSource property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getContentSource() {
return contentSource;
}
/**
* Sets the value of the contentSource property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setContentSource(String value) {
this.contentSource = value;
}
/**
* Gets the value of the createdBy property.
*
* @return
* possible object is
* {@link User }
*
*/
public User getCreatedBy() {
return createdBy;
}
/**
* Sets the value of the createdBy property.
*
* @param value
* allowed object is
* {@link User }
*
*/
public void setCreatedBy(User value) {
this.createdBy = value;
}
/**
* Gets the value of the createdById property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCreatedById() {
return createdById;
}
/**
* Sets the value of the createdById property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCreatedById(String value) {
this.createdById = value;
}
/**
* Gets the value of the createdDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getCreatedDate() {
return createdDate;
}
/**
* Sets the value of the createdDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setCreatedDate(XMLGregorianCalendar value) {
this.createdDate = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the developerName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeveloperName() {
return developerName;
}
/**
* Sets the value of the developerName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeveloperName(String value) {
this.developerName = value;
}
/**
* Gets the value of the encodingKey property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEncodingKey() {
return encodingKey;
}
/**
* Sets the value of the encodingKey property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEncodingKey(String value) {
this.encodingKey = value;
}
/**
* Gets the value of the lastModifiedBy property.
*
* @return
* possible object is
* {@link User }
*
*/
public User getLastModifiedBy() {
return lastModifiedBy;
}
/**
* Sets the value of the lastModifiedBy property.
*
* @param value
* allowed object is
* {@link User }
*
*/
public void setLastModifiedBy(User value) {
this.lastModifiedBy = value;
}
/**
* Gets the value of the lastModifiedById property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLastModifiedById() {
return lastModifiedById;
}
/**
* Sets the value of the lastModifiedById property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLastModifiedById(String value) {
this.lastModifiedById = value;
}
/**
* Gets the value of the lastModifiedDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getLastModifiedDate() {
return lastModifiedDate;
}
/**
* Sets the value of the lastModifiedDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setLastModifiedDate(XMLGregorianCalendar value) {
this.lastModifiedDate = value;
}
/**
* Gets the value of the manageableState property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getManageableState() {
return manageableState;
}
/**
* Sets the value of the manageableState property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setManageableState(String value) {
this.manageableState = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the namespacePrefix property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNamespacePrefix() {
return namespacePrefix;
}
/**
* Sets the value of the namespacePrefix property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNamespacePrefix(String value) {
this.namespacePrefix = value;
}
/**
* Gets the value of the supportsCaching property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isSupportsCaching() {
return supportsCaching;
}
/**
* Sets the value of the supportsCaching property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setSupportsCaching(Boolean value) {
this.supportsCaching = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy