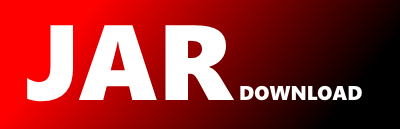
com.sforce.soap.tooling.metadata.Layout Maven / Gradle / Ivy
Show all versions of sforce-tooling-api Show documentation
package com.sforce.soap.tooling.metadata;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import com.sforce.soap.tooling.LayoutHeader;
/**
* Java class for Layout complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Layout">
* <complexContent>
* <extension base="{urn:metadata.tooling.soap.sforce.com}Metadata">
* <sequence>
* <element name="customButtons" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="customConsoleComponents" type="{urn:metadata.tooling.soap.sforce.com}CustomConsoleComponents" minOccurs="0"/>
* <element name="emailDefault" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="excludeButtons" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="feedLayout" type="{urn:metadata.tooling.soap.sforce.com}FeedLayout" minOccurs="0"/>
* <element name="headers" type="{urn:tooling.soap.sforce.com}LayoutHeader" maxOccurs="unbounded" minOccurs="0"/>
* <element name="layoutSections" type="{urn:metadata.tooling.soap.sforce.com}LayoutSection" maxOccurs="unbounded" minOccurs="0"/>
* <element name="miniLayout" type="{urn:metadata.tooling.soap.sforce.com}MiniLayout" minOccurs="0"/>
* <element name="multilineLayoutFields" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="quickActionList" type="{urn:metadata.tooling.soap.sforce.com}QuickActionList" minOccurs="0"/>
* <element name="relatedContent" type="{urn:metadata.tooling.soap.sforce.com}RelatedContent" minOccurs="0"/>
* <element name="relatedLists" type="{urn:metadata.tooling.soap.sforce.com}RelatedListItem" maxOccurs="unbounded" minOccurs="0"/>
* <element name="relatedObjects" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="runAssignmentRulesDefault" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="showEmailCheckbox" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="showHighlightsPanel" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="showInteractionLogPanel" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="showKnowledgeComponent" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="showRunAssignmentRulesCheckbox" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="showSolutionSection" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="showSubmitAndAttachButton" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="summaryLayout" type="{urn:metadata.tooling.soap.sforce.com}SummaryLayout" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Layout", propOrder = {
"customButtons",
"customConsoleComponents",
"emailDefault",
"excludeButtons",
"feedLayout",
"headers",
"layoutSections",
"miniLayout",
"multilineLayoutFields",
"quickActionList",
"relatedContent",
"relatedLists",
"relatedObjects",
"runAssignmentRulesDefault",
"showEmailCheckbox",
"showHighlightsPanel",
"showInteractionLogPanel",
"showKnowledgeComponent",
"showRunAssignmentRulesCheckbox",
"showSolutionSection",
"showSubmitAndAttachButton",
"summaryLayout"
})
public class Layout
extends Metadata
{
protected List customButtons;
protected CustomConsoleComponents customConsoleComponents;
protected Boolean emailDefault;
protected List excludeButtons;
protected FeedLayout feedLayout;
@XmlSchemaType(name = "string")
protected List headers;
protected List layoutSections;
protected MiniLayout miniLayout;
protected List multilineLayoutFields;
protected QuickActionList quickActionList;
protected RelatedContent relatedContent;
protected List relatedLists;
protected List relatedObjects;
protected Boolean runAssignmentRulesDefault;
protected Boolean showEmailCheckbox;
protected Boolean showHighlightsPanel;
protected Boolean showInteractionLogPanel;
protected Boolean showKnowledgeComponent;
protected Boolean showRunAssignmentRulesCheckbox;
protected Boolean showSolutionSection;
protected Boolean showSubmitAndAttachButton;
protected SummaryLayout summaryLayout;
/**
* Gets the value of the customButtons property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the customButtons property.
*
*
* For example, to add a new item, do as follows:
*
* getCustomButtons().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getCustomButtons() {
if (customButtons == null) {
customButtons = new ArrayList();
}
return this.customButtons;
}
/**
* Gets the value of the customConsoleComponents property.
*
* @return
* possible object is
* {@link CustomConsoleComponents }
*
*/
public CustomConsoleComponents getCustomConsoleComponents() {
return customConsoleComponents;
}
/**
* Sets the value of the customConsoleComponents property.
*
* @param value
* allowed object is
* {@link CustomConsoleComponents }
*
*/
public void setCustomConsoleComponents(CustomConsoleComponents value) {
this.customConsoleComponents = value;
}
/**
* Gets the value of the emailDefault property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isEmailDefault() {
return emailDefault;
}
/**
* Sets the value of the emailDefault property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setEmailDefault(Boolean value) {
this.emailDefault = value;
}
/**
* Gets the value of the excludeButtons property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the excludeButtons property.
*
*
* For example, to add a new item, do as follows:
*
* getExcludeButtons().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getExcludeButtons() {
if (excludeButtons == null) {
excludeButtons = new ArrayList();
}
return this.excludeButtons;
}
/**
* Gets the value of the feedLayout property.
*
* @return
* possible object is
* {@link FeedLayout }
*
*/
public FeedLayout getFeedLayout() {
return feedLayout;
}
/**
* Sets the value of the feedLayout property.
*
* @param value
* allowed object is
* {@link FeedLayout }
*
*/
public void setFeedLayout(FeedLayout value) {
this.feedLayout = value;
}
/**
* Gets the value of the headers property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the headers property.
*
*
* For example, to add a new item, do as follows:
*
* getHeaders().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LayoutHeader }
*
*
*/
public List getHeaders() {
if (headers == null) {
headers = new ArrayList();
}
return this.headers;
}
/**
* Gets the value of the layoutSections property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the layoutSections property.
*
*
* For example, to add a new item, do as follows:
*
* getLayoutSections().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LayoutSection }
*
*
*/
public List getLayoutSections() {
if (layoutSections == null) {
layoutSections = new ArrayList();
}
return this.layoutSections;
}
/**
* Gets the value of the miniLayout property.
*
* @return
* possible object is
* {@link MiniLayout }
*
*/
public MiniLayout getMiniLayout() {
return miniLayout;
}
/**
* Sets the value of the miniLayout property.
*
* @param value
* allowed object is
* {@link MiniLayout }
*
*/
public void setMiniLayout(MiniLayout value) {
this.miniLayout = value;
}
/**
* Gets the value of the multilineLayoutFields property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the multilineLayoutFields property.
*
*
* For example, to add a new item, do as follows:
*
* getMultilineLayoutFields().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getMultilineLayoutFields() {
if (multilineLayoutFields == null) {
multilineLayoutFields = new ArrayList();
}
return this.multilineLayoutFields;
}
/**
* Gets the value of the quickActionList property.
*
* @return
* possible object is
* {@link QuickActionList }
*
*/
public QuickActionList getQuickActionList() {
return quickActionList;
}
/**
* Sets the value of the quickActionList property.
*
* @param value
* allowed object is
* {@link QuickActionList }
*
*/
public void setQuickActionList(QuickActionList value) {
this.quickActionList = value;
}
/**
* Gets the value of the relatedContent property.
*
* @return
* possible object is
* {@link RelatedContent }
*
*/
public RelatedContent getRelatedContent() {
return relatedContent;
}
/**
* Sets the value of the relatedContent property.
*
* @param value
* allowed object is
* {@link RelatedContent }
*
*/
public void setRelatedContent(RelatedContent value) {
this.relatedContent = value;
}
/**
* Gets the value of the relatedLists property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the relatedLists property.
*
*
* For example, to add a new item, do as follows:
*
* getRelatedLists().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RelatedListItem }
*
*
*/
public List getRelatedLists() {
if (relatedLists == null) {
relatedLists = new ArrayList();
}
return this.relatedLists;
}
/**
* Gets the value of the relatedObjects property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the relatedObjects property.
*
*
* For example, to add a new item, do as follows:
*
* getRelatedObjects().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getRelatedObjects() {
if (relatedObjects == null) {
relatedObjects = new ArrayList();
}
return this.relatedObjects;
}
/**
* Gets the value of the runAssignmentRulesDefault property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRunAssignmentRulesDefault() {
return runAssignmentRulesDefault;
}
/**
* Sets the value of the runAssignmentRulesDefault property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRunAssignmentRulesDefault(Boolean value) {
this.runAssignmentRulesDefault = value;
}
/**
* Gets the value of the showEmailCheckbox property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isShowEmailCheckbox() {
return showEmailCheckbox;
}
/**
* Sets the value of the showEmailCheckbox property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setShowEmailCheckbox(Boolean value) {
this.showEmailCheckbox = value;
}
/**
* Gets the value of the showHighlightsPanel property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isShowHighlightsPanel() {
return showHighlightsPanel;
}
/**
* Sets the value of the showHighlightsPanel property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setShowHighlightsPanel(Boolean value) {
this.showHighlightsPanel = value;
}
/**
* Gets the value of the showInteractionLogPanel property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isShowInteractionLogPanel() {
return showInteractionLogPanel;
}
/**
* Sets the value of the showInteractionLogPanel property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setShowInteractionLogPanel(Boolean value) {
this.showInteractionLogPanel = value;
}
/**
* Gets the value of the showKnowledgeComponent property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isShowKnowledgeComponent() {
return showKnowledgeComponent;
}
/**
* Sets the value of the showKnowledgeComponent property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setShowKnowledgeComponent(Boolean value) {
this.showKnowledgeComponent = value;
}
/**
* Gets the value of the showRunAssignmentRulesCheckbox property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isShowRunAssignmentRulesCheckbox() {
return showRunAssignmentRulesCheckbox;
}
/**
* Sets the value of the showRunAssignmentRulesCheckbox property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setShowRunAssignmentRulesCheckbox(Boolean value) {
this.showRunAssignmentRulesCheckbox = value;
}
/**
* Gets the value of the showSolutionSection property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isShowSolutionSection() {
return showSolutionSection;
}
/**
* Sets the value of the showSolutionSection property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setShowSolutionSection(Boolean value) {
this.showSolutionSection = value;
}
/**
* Gets the value of the showSubmitAndAttachButton property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isShowSubmitAndAttachButton() {
return showSubmitAndAttachButton;
}
/**
* Sets the value of the showSubmitAndAttachButton property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setShowSubmitAndAttachButton(Boolean value) {
this.showSubmitAndAttachButton = value;
}
/**
* Gets the value of the summaryLayout property.
*
* @return
* possible object is
* {@link SummaryLayout }
*
*/
public SummaryLayout getSummaryLayout() {
return summaryLayout;
}
/**
* Sets the value of the summaryLayout property.
*
* @param value
* allowed object is
* {@link SummaryLayout }
*
*/
public void setSummaryLayout(SummaryLayout value) {
this.summaryLayout = value;
}
}