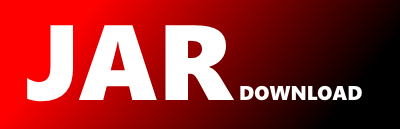
com.sforce.soap.tooling.metadata.LayoutItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sforce-tooling-api Show documentation
Show all versions of sforce-tooling-api Show documentation
Salesforce Tooling API client
The newest version!
package com.sforce.soap.tooling.metadata;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import com.sforce.soap.tooling.UiBehavior;
/**
* Java class for LayoutItem complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="LayoutItem">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="analyticsCloudComponent" type="{urn:metadata.tooling.soap.sforce.com}AnalyticsCloudComponentLayoutItem" minOccurs="0"/>
* <element name="behavior" type="{urn:tooling.soap.sforce.com}UiBehavior" minOccurs="0"/>
* <element name="canvas" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="component" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="customLink" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="emptySpace" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="field" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="height" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="page" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="reportChartComponent" type="{urn:metadata.tooling.soap.sforce.com}ReportChartComponentLayoutItem" minOccurs="0"/>
* <element name="scontrol" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="showLabel" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="showScrollbars" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="width" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "LayoutItem", propOrder = {
"analyticsCloudComponent",
"behavior",
"canvas",
"component",
"customLink",
"emptySpace",
"field",
"height",
"page",
"reportChartComponent",
"scontrol",
"showLabel",
"showScrollbars",
"width"
})
public class LayoutItem {
protected AnalyticsCloudComponentLayoutItem analyticsCloudComponent;
@XmlSchemaType(name = "string")
protected UiBehavior behavior;
protected String canvas;
protected String component;
protected String customLink;
protected Boolean emptySpace;
protected String field;
protected Integer height;
protected String page;
protected ReportChartComponentLayoutItem reportChartComponent;
protected String scontrol;
protected Boolean showLabel;
protected Boolean showScrollbars;
protected String width;
/**
* Gets the value of the analyticsCloudComponent property.
*
* @return
* possible object is
* {@link AnalyticsCloudComponentLayoutItem }
*
*/
public AnalyticsCloudComponentLayoutItem getAnalyticsCloudComponent() {
return analyticsCloudComponent;
}
/**
* Sets the value of the analyticsCloudComponent property.
*
* @param value
* allowed object is
* {@link AnalyticsCloudComponentLayoutItem }
*
*/
public void setAnalyticsCloudComponent(AnalyticsCloudComponentLayoutItem value) {
this.analyticsCloudComponent = value;
}
/**
* Gets the value of the behavior property.
*
* @return
* possible object is
* {@link UiBehavior }
*
*/
public UiBehavior getBehavior() {
return behavior;
}
/**
* Sets the value of the behavior property.
*
* @param value
* allowed object is
* {@link UiBehavior }
*
*/
public void setBehavior(UiBehavior value) {
this.behavior = value;
}
/**
* Gets the value of the canvas property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCanvas() {
return canvas;
}
/**
* Sets the value of the canvas property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCanvas(String value) {
this.canvas = value;
}
/**
* Gets the value of the component property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getComponent() {
return component;
}
/**
* Sets the value of the component property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setComponent(String value) {
this.component = value;
}
/**
* Gets the value of the customLink property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCustomLink() {
return customLink;
}
/**
* Sets the value of the customLink property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCustomLink(String value) {
this.customLink = value;
}
/**
* Gets the value of the emptySpace property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isEmptySpace() {
return emptySpace;
}
/**
* Sets the value of the emptySpace property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setEmptySpace(Boolean value) {
this.emptySpace = value;
}
/**
* Gets the value of the field property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getField() {
return field;
}
/**
* Sets the value of the field property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setField(String value) {
this.field = value;
}
/**
* Gets the value of the height property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getHeight() {
return height;
}
/**
* Sets the value of the height property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setHeight(Integer value) {
this.height = value;
}
/**
* Gets the value of the page property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPage() {
return page;
}
/**
* Sets the value of the page property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPage(String value) {
this.page = value;
}
/**
* Gets the value of the reportChartComponent property.
*
* @return
* possible object is
* {@link ReportChartComponentLayoutItem }
*
*/
public ReportChartComponentLayoutItem getReportChartComponent() {
return reportChartComponent;
}
/**
* Sets the value of the reportChartComponent property.
*
* @param value
* allowed object is
* {@link ReportChartComponentLayoutItem }
*
*/
public void setReportChartComponent(ReportChartComponentLayoutItem value) {
this.reportChartComponent = value;
}
/**
* Gets the value of the scontrol property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getScontrol() {
return scontrol;
}
/**
* Sets the value of the scontrol property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setScontrol(String value) {
this.scontrol = value;
}
/**
* Gets the value of the showLabel property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isShowLabel() {
return showLabel;
}
/**
* Sets the value of the showLabel property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setShowLabel(Boolean value) {
this.showLabel = value;
}
/**
* Gets the value of the showScrollbars property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isShowScrollbars() {
return showScrollbars;
}
/**
* Sets the value of the showScrollbars property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setShowScrollbars(Boolean value) {
this.showScrollbars = value;
}
/**
* Gets the value of the width property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWidth() {
return width;
}
/**
* Sets the value of the width property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWidth(String value) {
this.width = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy