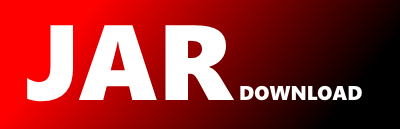
la.renzhen.rtpt.admin.controller.AdminAPIController Maven / Gradle / Ivy
package la.renzhen.rtpt.admin.controller;
import la.renzhen.rtpt.admin.boot.starter.CenterServerProperties;
import la.renzhen.rtpt.admin.source.CenterConfigurationSource;
import la.renzhen.rtpt.admin.source.ConfigElement;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.MediaType;
import org.springframework.web.bind.annotation.*;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* 管理
*
* @author haiker
* @version 14/05/2018 7:48 PM
*/
@RestController
@RequestMapping(value = "${rtpt.admin.context-path:/rtpt}/api", produces = MediaType.APPLICATION_JSON_UTF8_VALUE)
public class AdminAPIController {
@Autowired
CenterConfigurationSource centerConfigurationSource;
@Autowired
CenterServerProperties centerServerProperties;
@GetMapping(value = "/properties")
public Map allProperties(
@RequestParam(value = "prefix", required = false) String prefix,
@RequestParam(value = "env", required = false) String environment) {
String env = Optional.ofNullable(environment).orElse(centerServerProperties.getDefaultEnvironment());
String pre = Optional.ofNullable(prefix).orElse("");
return centerConfigurationSource.loadAll(pre, env).stream()
.collect(Collectors.toMap(ConfigElement::getKey, ConfigElement::getValue));
}
@GetMapping("/property/{key}/")
public String get(
@PathVariable("key") String key,
@RequestParam(value = "env", required = false) String environment
) {
String env = Optional.ofNullable(environment).orElse(centerServerProperties.getDefaultEnvironment());
ConfigElement element = centerConfigurationSource.get(env, key);
if (element == null) {
return null;
}
return element.getValue();
}
}