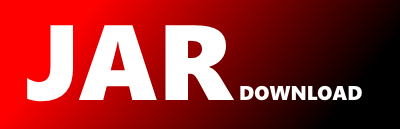
li.rudin.arduino.managed.ManagedInterceptor Maven / Gradle / Ivy
package li.rudin.arduino.managed;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import li.rudin.arduino.api.Arduino;
import li.rudin.arduino.api.converter.TypeConverter;
import net.sf.cglib.proxy.MethodInterceptor;
import net.sf.cglib.proxy.MethodProxy;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ManagedInterceptor implements MethodInterceptor
{
/**
* Local logger
*/
private static final Logger logger = LoggerFactory.getLogger(ManagedInterceptor.class);
public ManagedInterceptor(Class> type, Arduino arduino)
{
this.arduino = arduino;
this.type = type;
}
public final Arduino arduino;
public final Class> type;
@Override
public Object intercept(Object obj, Method method, Object[] args, MethodProxy proxy) throws Throwable
{
//TODO throw exception, if not connected
String methodName = method.getName();
Class> methodOwner = method.getDeclaringClass();
logger.debug("Intercepted method {}.{}", methodOwner.getSimpleName(), methodName);
if (!Modifier.isAbstract(method.getModifiers()))
return proxy.invokeSuper(obj, args);
if (methodOwner == Arduino.class)
//Delegate to device instance
return proxy.invoke(arduino, args);
if (methodName.startsWith("get"))
{
String key = methodName.substring(3, 4).toLowerCase() + methodName.substring(4);
String str = arduino.get(key);
if (str == null)
throw new IllegalArgumentException("null-result from query: " + key);
str = str.trim();
Class> type = method.getReturnType();
return TypeConverter.convertIncoming(str, type);
}
else if (methodName.startsWith("set"))
{
String key = methodName.substring(3, 4).toLowerCase() + methodName.substring(4);
if (args.length == 1)
arduino.send(key, TypeConverter.convertOutgoing(args[0]));
else if (args.length == 0)
arduino.send(key, "");
return null;
}
else if (methodName.startsWith("do"))
{
String key = methodName.substring(2, 3).toLowerCase() + methodName.substring(3);
if (args.length == 1)
arduino.send(key, TypeConverter.convertOutgoing(args[0]));
else if (args.length == 0)
arduino.send(key, "");
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy