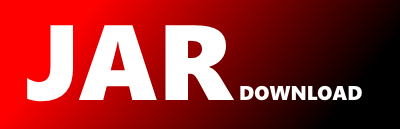
li.rudin.rt.api.observable.list.ObservableList Maven / Gradle / Ivy
package li.rudin.rt.api.observable.list;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import java.util.Set;
import java.util.concurrent.CopyOnWriteArraySet;
public class ObservableList implements List
{
/**
* Creates an observer with backed ArrayList
*/
public ObservableList()
{
this(new ArrayList());
}
/**
* Creates an observer with given default value
* @param list
*/
public ObservableList(List list)
{
this.list = list;
}
/**
* All local listeners
*/
private Set> listListeners = new CopyOnWriteArraySet<>();
/**
* The current value
*/
private final List list;
/**
* Returns the backed list
* @return
*/
public List getList()
{
return list;
}
public void addListListener(ListListener l)
{
listListeners.add(l);
}
public void removeListListener(ListListener l)
{
listListeners.remove(l);
}
@Override
public boolean add(T e)
{
for (ListListener ll: listListeners)
ll.onAdd(e);
return list.add(e);
}
@Override
public void add(int index, T element)
{
for (ListListener ll: listListeners)
ll.onAdd(element, index);
list.add(index, element);
}
@Override
public boolean addAll(Collection extends T> c) {
for (ListListener ll: listListeners)
for (T obj: c)
ll.onAdd(obj);
return list.addAll(c);
}
@Override
public boolean addAll(int index, Collection extends T> c) {
throw new IllegalArgumentException("Sorry, not implemented, yet");
}
@Override
public void clear() {
for (ListListener ll: listListeners)
ll.onClear();
list.clear();
}
@Override
public boolean contains(Object o) {
return list.contains(o);
}
@Override
public boolean containsAll(Collection> c) {
return list.containsAll(c);
}
@Override
public T get(int index) {
return list.get(index);
}
@Override
public int indexOf(Object o) {
return list.indexOf(o);
}
@Override
public boolean isEmpty() {
return list.isEmpty();
}
@Override
public Iterator iterator() {
return list.iterator();
}
@Override
public int lastIndexOf(Object o) {
return list.lastIndexOf(o);
}
@Override
public ListIterator listIterator() {
return list.listIterator();
}
@Override
public ListIterator listIterator(int index) {
return list.listIterator(index);
}
@Override
public boolean remove(Object o) {
throw new IllegalArgumentException("Sorry, not implemented, yet");
}
@Override
public T remove(int index) {
for (ListListener ll: listListeners)
ll.onRemove(index);
return list.remove(index);
}
@Override
public boolean removeAll(Collection> c) {
throw new IllegalArgumentException("Sorry, not implemented, yet");
}
@Override
public boolean retainAll(Collection> c) {
throw new IllegalArgumentException("Sorry, not implemented, yet");
}
@Override
public T set(int index, T element) {
for (ListListener ll: listListeners)
ll.onReplace(element, index);
return list.set(index, element);
}
@Override
public int size() {
return list.size();
}
@Override
public List subList(int fromIndex, int toIndex) {
throw new IllegalArgumentException("Sorry, not implemented, yet");
}
@Override
public Object[] toArray() {
return list.toArray();
}
@Override
public O[] toArray(O[] a) {
return list.toArray(a);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy