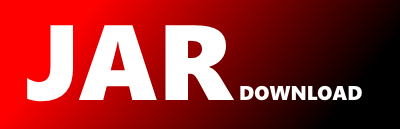
qa.tools.ikeeper.IKeeperInterceptor Maven / Gradle / Ivy
package qa.tools.ikeeper;
import java.io.IOException;
import java.io.InputStream;
import java.lang.annotation.Annotation;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Objects;
import java.util.Properties;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import qa.tools.ikeeper.action.IAction;
import qa.tools.ikeeper.client.ITrackerClient;
import qa.tools.ikeeper.test.IKeeperConnector;
public class IKeeperInterceptor {
private static final Logger LOG = LoggerFactory.getLogger(IKeeperInterceptor.class);
private static final String constraintsPropFileName = "ikeeperConstraints.properties";
private static Map issueConstraints = new HashMap();
static {
loadIssueConstraints();
}
private boolean enabled = true;
public IKeeperInterceptor() {
}
public void intercept(String testName, IAction action, List annotations, ITrackerClient[] clients, Map evaluationProperties) {
if (!enabled) {
return;
}
for (Annotation annotation : annotations) {
for (ITrackerClient c : clients) {
if (c.canHandle(annotation)) {
List detailsList = c.getIssues(annotation);
for (IssueDetails details : detailsList) {
if (details != null) {
intercept(testName, details, evaluationProperties, action);
}
}
break;
}
}
}
}
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
private static void loadIssueConstraints() {
Properties envProps = new Properties();
InputStream inputStream = IKeeperConnector.class.getClassLoader().getResourceAsStream(constraintsPropFileName);
if (inputStream != null) {
try {
envProps.load(inputStream);
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy