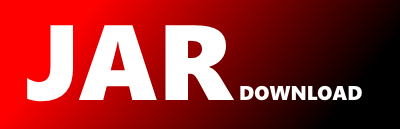
link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package link.thingscloud.freeswitch.esl.inbound.option;
import link.thingscloud.freeswitch.esl.IEslEventListener;
import link.thingscloud.freeswitch.esl.ServerConnectionListener;
import link.thingscloud.freeswitch.esl.inbound.listener.EventListener;
import link.thingscloud.freeswitch.esl.inbound.listener.ServerOptionListener;
import link.thingscloud.freeswitch.esl.util.StringUtils;
import lombok.ToString;
import java.util.ArrayList;
import java.util.List;
/**
* InboundClientOption class.
*
* @author : zhouhailin
* @version 1.0.0
*/
@ToString
public class InboundClientOption {
private int sndBufSize = 65535;
private int rcvBufSize = 65535;
private int workerGroupThread = Runtime.getRuntime().availableProcessors() * 2;
private int publicExecutorThread = Runtime.getRuntime().availableProcessors() * 2;
private int callbackExecutorThread = Runtime.getRuntime().availableProcessors() * 2;
private int defaultTimeoutSeconds = 5;
private String defaultPassword = "ClueCon";
private int readTimeoutSeconds = 30;
private int readerIdleTimeSeconds = 25;
private boolean disablePublicExecutor = false;
private boolean performance = false;
private long performanceCostTime = 200;
private boolean eventPerformance = false;
private long eventPerformanceCostTime = 200;
private ServerOptionListener serverOptionListener = null;
private ServerConnectionListener serverConnectionListener = null;
private final List serverOptions = new ArrayList<>();
private final ServerAddrOption serverAddrOption = new ServerAddrOption(serverOptions);
private final List listeners = new ArrayList<>();
private EventListener eventListener = null;
private final List events = new ArrayList<>();
/**
* sndBufSize.
*
* @return a int.
*/
public int sndBufSize() {
return sndBufSize;
}
/**
* sndBufSize.
*
* @param sndBufSize a int.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption sndBufSize(int sndBufSize) {
this.sndBufSize = sndBufSize;
return this;
}
/**
* rcvBufSize.
*
* @return a int.
*/
public int rcvBufSize() {
return rcvBufSize;
}
/**
* rcvBufSize.
*
* @param rcvBufSize a int.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption rcvBufSize(int rcvBufSize) {
this.rcvBufSize = rcvBufSize;
return this;
}
/**
* workerGroupThread.
*
* @return a int.
*/
public int workerGroupThread() {
return workerGroupThread;
}
/**
* workerGroupThread.
*
* @param workerGroupThread a int.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption workerGroupThread(int workerGroupThread) {
this.workerGroupThread = workerGroupThread;
return this;
}
/**
* publicExecutorThread.
*
* @return a int.
*/
public int publicExecutorThread() {
return publicExecutorThread;
}
/**
* publicExecutorThread.
*
* @param publicExecutorThread a int.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption publicExecutorThread(int publicExecutorThread) {
this.publicExecutorThread = publicExecutorThread;
return this;
}
/**
* callbackExecutorThread.
*
* @return a int.
*/
public int callbackExecutorThread() {
return callbackExecutorThread;
}
/**
* callbackExecutorThread.
*
* @param callbackExecutorThread a int.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption callbackExecutorThread(int callbackExecutorThread) {
this.callbackExecutorThread = callbackExecutorThread;
return this;
}
/**
* defaultTimeoutSeconds.
*
* @return a int.
*/
public int defaultTimeoutSeconds() {
return defaultTimeoutSeconds;
}
/**
* defaultTimeoutSeconds.
*
* @param defaultTimeoutSeconds a int.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption defaultTimeoutSeconds(int defaultTimeoutSeconds) {
this.defaultTimeoutSeconds = defaultTimeoutSeconds;
return this;
}
/**
* defaultPassword.
*
* @return a {@link java.lang.String} object.
*/
public String defaultPassword() {
return defaultPassword;
}
/**
* defaultPassword.
*
* @param defaultPassword a {@link java.lang.String} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption defaultPassword(String defaultPassword) {
this.defaultPassword = defaultPassword;
return this;
}
/**
* readTimeoutSeconds.
*
* @return a {@link java.lang.Integer} object.
*/
public int readTimeoutSeconds() {
return readTimeoutSeconds;
}
/**
* readTimeoutSeconds.
*
* @param readTimeoutSeconds a {@link java.lang.Integer} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption readTimeoutSeconds(int readTimeoutSeconds) {
this.readTimeoutSeconds = readTimeoutSeconds;
return this;
}
/**
* readerIdleTimeSeconds.
*
* @return a {@link java.lang.Integer} object.
*/
public int readerIdleTimeSeconds() {
return readerIdleTimeSeconds;
}
/**
* readerIdleTimeSeconds.
*
* 读空闲时长
*
* @param readerIdleTimeSeconds a {@link java.lang.Integer} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption readerIdleTimeSeconds(int readerIdleTimeSeconds) {
this.readerIdleTimeSeconds = readerIdleTimeSeconds;
return this;
}
/**
*
* disable public executor thread pool then message thread safety.
* 处理事件消息时不使用独立线程池,可以使得消息线程安全
*
*
* @return a boolean.
*/
public boolean disablePublicExecutor() {
return disablePublicExecutor;
}
/**
*
* disable public executor thread pool then message thread safety.
* 处理事件消息时不使用独立线程池,可以使得消息线程安全
*
*
* @param disablePublicExecutor a boolean.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption disablePublicExecutor(boolean disablePublicExecutor) {
this.disablePublicExecutor = disablePublicExecutor;
return this;
}
/**
* performance.
*
* @return a boolean.
*/
public boolean performance() {
return performance;
}
/**
* performance.
*
* @param performance a boolean.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption performance(boolean performance) {
this.performance = performance;
return this;
}
/**
* performanceCostTime.
*
* @return a long.
*/
public long performanceCostTime() {
return performanceCostTime;
}
/**
* performanceCostTime.
*
* @param performanceCostTime a long.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption performanceCostTime(long performanceCostTime) {
this.performanceCostTime = performanceCostTime;
return this;
}
/**
* eventPerformance.
*
* @return a boolean.
*/
public boolean eventPerformance() {
return eventPerformance;
}
/**
* eventPerformance.
*
* @param eventPerformance a boolean.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption eventPerformance(boolean eventPerformance) {
this.eventPerformance = eventPerformance;
return this;
}
/**
* eventPerformanceCostTime.
*
* @return a long.
*/
public long eventPerformanceCostTime() {
return eventPerformanceCostTime;
}
/**
* eventPerformanceCostTime.
*
* @param eventPerformanceCostTime a long.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption eventPerformanceCostTime(long eventPerformanceCostTime) {
this.eventPerformanceCostTime = eventPerformanceCostTime;
return this;
}
/**
* serverOptionListener.
*
* @return a {@link link.thingscloud.freeswitch.esl.inbound.listener.ServerOptionListener} object.
*/
public ServerOptionListener serverOptionListener() {
return serverOptionListener;
}
/**
* serverOptionListener.
*
* @param serverOptionListener a {@link link.thingscloud.freeswitch.esl.inbound.listener.ServerOptionListener} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption serverOptionListener(ServerOptionListener serverOptionListener) {
this.serverOptionListener = serverOptionListener;
return this;
}
/**
* serverConnectionListener.
*
* @return a {@link link.thingscloud.freeswitch.esl.inbound.listener.ServerOptionListener} object.
*/
public ServerConnectionListener serverConnectionListener() {
return serverConnectionListener;
}
/**
* serverConnectionListener.
*
* @param serverConnectionListener a {@link link.thingscloud.freeswitch.esl.ServerConnectionListener} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption serverConnectionListener(ServerConnectionListener serverConnectionListener) {
this.serverConnectionListener = serverConnectionListener;
return this;
}
/**
* serverAddrOption.
*
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.ServerAddrOption} object.
*/
public ServerAddrOption serverAddrOption() {
return serverAddrOption;
}
/**
* serverOptions.
*
* @return a {@link java.util.List} object.
*/
public List serverOptions() {
return serverOptions;
}
/**
* addServerOption.
*
* @param serverOption a {@link link.thingscloud.freeswitch.esl.inbound.option.ServerOption} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption addServerOption(ServerOption serverOption) {
for (ServerOption option : serverOptions) {
if (StringUtils.equals(option.addr(), serverOption.addr())) {
return this;
}
}
serverOptions.add(serverOption);
if (serverOptionListener != null) {
serverOptionListener.onAdded(serverOption);
}
return this;
}
/**
* removeServerOption.
*
* @param serverOption a {@link link.thingscloud.freeswitch.esl.inbound.option.ServerOption} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption removeServerOption(ServerOption serverOption) {
serverOptions.remove(serverOption);
if (serverOptionListener != null) {
serverOptionListener.onRemoved(serverOption);
}
return this;
}
/**
* addListener.
*
* @param listener a {@link link.thingscloud.freeswitch.esl.IEslEventListener} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption addListener(IEslEventListener listener) {
listeners.add(listener);
return this;
}
/**
* removeListener.
*
* @param listener a {@link link.thingscloud.freeswitch.esl.IEslEventListener} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption removeListener(IEslEventListener listener) {
listeners.remove(listener);
return this;
}
/**
* listeners.
*
* @return a {@link java.util.List} object.
*/
public List listeners() {
return listeners;
}
/**
* eventListener.
*
* @return a {@link link.thingscloud.freeswitch.esl.inbound.listener.EventListener} object.
*/
public EventListener eventListener() {
return eventListener;
}
/**
* eventListener.
*
* @param eventListener a {@link link.thingscloud.freeswitch.esl.inbound.listener.EventListener} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption eventListener(EventListener eventListener) {
this.eventListener = eventListener;
return this;
}
/**
* events.
*
* @return a {@link java.util.List} object.
*/
public List events() {
return events;
}
/**
* addEvents.
*
* @param addEvents a {@link java.lang.String} object.
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption addEvents(String... addEvents) {
if (addEvents == null) {
return this;
}
List list = new ArrayList<>();
for (String addEvent : addEvents) {
if (!events().contains(addEvent)) {
list.add(addEvent);
}
}
if (!list.isEmpty()) {
events.addAll(list);
if (eventListener != null) {
eventListener.addEvents(list);
}
}
return this;
}
/**
* cancelEvents.
*
* @return a {@link link.thingscloud.freeswitch.esl.inbound.option.InboundClientOption} object.
*/
public InboundClientOption cancelEvents() {
if (!events.isEmpty()) {
if (eventListener != null) {
eventListener.cancelEvents();
}
events.clear();
}
return this;
}
}