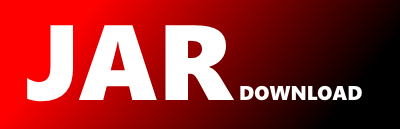
live.radix.gateway.client.StateApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of babylon-gateway-client Show documentation
Show all versions of babylon-gateway-client Show documentation
A generated Client library for Radix Babylon Gateway API.
/*
* Radix Gateway API - Babylon
* This API is exposed by the Babylon Radix Gateway to enable clients to efficiently query current and historic state on the RadixDLT ledger, and intelligently handle transaction submission. It is designed for use by wallets and explorers, and for light queries from front-end dApps. For exchange/asset integrations, back-end dApp integrations, or simple use cases, you should consider using the Core API on a Node. A Gateway is only needed for reading historic snapshots of ledger states or a more robust set-up. The Gateway API is implemented by the [Network Gateway](https://github.com/radixdlt/babylon-gateway), which is configured to read from [full node(s)](https://github.com/radixdlt/babylon-node) to extract and index data from the network. This document is an API reference documentation, visit [User Guide](https://docs.radixdlt.com/) to learn more about how to run a Gateway of your own. ## Migration guide Please see [the latest release notes](https://github.com/radixdlt/babylon-gateway/releases). ## Integration and forward compatibility guarantees All responses may have additional fields added at any release, so clients are advised to use JSON parsers which ignore unknown fields on JSON objects. When the Radix protocol is updated, new functionality may be added, and so discriminated unions returned by the API may need to be updated to have new variants added, corresponding to the updated data. Clients may need to update in advance to be able to handle these new variants when a protocol update comes out. On the very rare occasions we need to make breaking changes to the API, these will be warned in advance with deprecation notices on previous versions. These deprecation notices will include a safe migration path. Deprecation notes or breaking changes will be flagged clearly in release notes for new versions of the Gateway. The Gateway DB schema is not subject to any compatibility guarantees, and may be changed at any release. DB changes will be flagged in the release notes so clients doing custom DB integrations can prepare.
*
* The version of the OpenAPI document: v1.9.2-L
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package live.radix.gateway.client;
import com.fasterxml.jackson.core.type.TypeReference;
import live.radix.gateway.ApiException;
import live.radix.gateway.ApiClient;
import live.radix.gateway.BaseApi;
import live.radix.gateway.Configuration;
import live.radix.gateway.Pair;
import live.radix.gateway.model.ErrorResponse;
import live.radix.gateway.model.StateAccountAuthorizedDepositorsPageRequest;
import live.radix.gateway.model.StateAccountAuthorizedDepositorsPageResponse;
import live.radix.gateway.model.StateAccountLockerPageVaultsRequest;
import live.radix.gateway.model.StateAccountLockerPageVaultsResponse;
import live.radix.gateway.model.StateAccountLockersTouchedAtRequest;
import live.radix.gateway.model.StateAccountLockersTouchedAtResponse;
import live.radix.gateway.model.StateAccountResourcePreferencesPageRequest;
import live.radix.gateway.model.StateAccountResourcePreferencesPageResponse;
import live.radix.gateway.model.StateEntityDetailsRequest;
import live.radix.gateway.model.StateEntityDetailsResponse;
import live.radix.gateway.model.StateEntityFungibleResourceVaultsPageRequest;
import live.radix.gateway.model.StateEntityFungibleResourceVaultsPageResponse;
import live.radix.gateway.model.StateEntityFungiblesPageRequest;
import live.radix.gateway.model.StateEntityFungiblesPageResponse;
import live.radix.gateway.model.StateEntityMetadataPageRequest;
import live.radix.gateway.model.StateEntityMetadataPageResponse;
import live.radix.gateway.model.StateEntityNonFungibleIdsPageRequest;
import live.radix.gateway.model.StateEntityNonFungibleIdsPageResponse;
import live.radix.gateway.model.StateEntityNonFungibleResourceVaultsPageRequest;
import live.radix.gateway.model.StateEntityNonFungibleResourceVaultsPageResponse;
import live.radix.gateway.model.StateEntityNonFungiblesPageRequest;
import live.radix.gateway.model.StateEntityNonFungiblesPageResponse;
import live.radix.gateway.model.StateEntitySchemaPageRequest;
import live.radix.gateway.model.StateEntitySchemaPageResponse;
import live.radix.gateway.model.StateKeyValueStoreDataRequest;
import live.radix.gateway.model.StateKeyValueStoreDataResponse;
import live.radix.gateway.model.StateKeyValueStoreKeysRequest;
import live.radix.gateway.model.StateKeyValueStoreKeysResponse;
import live.radix.gateway.model.StateNonFungibleDataRequest;
import live.radix.gateway.model.StateNonFungibleDataResponse;
import live.radix.gateway.model.StateNonFungibleIdsRequest;
import live.radix.gateway.model.StateNonFungibleIdsResponse;
import live.radix.gateway.model.StateNonFungibleLocationRequest;
import live.radix.gateway.model.StateNonFungibleLocationResponse;
import live.radix.gateway.model.StatePackageBlueprintPageRequest;
import live.radix.gateway.model.StatePackageBlueprintPageResponse;
import live.radix.gateway.model.StatePackageCodePageRequest;
import live.radix.gateway.model.StatePackageCodePageResponse;
import live.radix.gateway.model.StateValidatorsListRequest;
import live.radix.gateway.model.StateValidatorsListResponse;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-12-12T19:38:04.213407200+02:00[Europe/Kiev]", comments = "Generator version: 7.7.0")
public class StateApi extends BaseApi {
public StateApi() {
super(Configuration.getDefaultApiClient());
}
public StateApi(ApiClient apiClient) {
super(apiClient);
}
/**
* Get Account authorized depositors
* Returns paginable collection of authorized depositors for given account.
* @param stateAccountAuthorizedDepositorsPageRequest (required)
* @return StateAccountAuthorizedDepositorsPageResponse
* @throws ApiException if fails to make API call
*/
public StateAccountAuthorizedDepositorsPageResponse accountAuthorizedDepositorsPage(StateAccountAuthorizedDepositorsPageRequest stateAccountAuthorizedDepositorsPageRequest) throws ApiException {
return this.accountAuthorizedDepositorsPage(stateAccountAuthorizedDepositorsPageRequest, Collections.emptyMap());
}
/**
* Get Account authorized depositors
* Returns paginable collection of authorized depositors for given account.
* @param stateAccountAuthorizedDepositorsPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateAccountAuthorizedDepositorsPageResponse
* @throws ApiException if fails to make API call
*/
public StateAccountAuthorizedDepositorsPageResponse accountAuthorizedDepositorsPage(StateAccountAuthorizedDepositorsPageRequest stateAccountAuthorizedDepositorsPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateAccountAuthorizedDepositorsPageRequest;
// verify the required parameter 'stateAccountAuthorizedDepositorsPageRequest' is set
if (stateAccountAuthorizedDepositorsPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateAccountAuthorizedDepositorsPageRequest' when calling accountAuthorizedDepositorsPage");
}
// create path and map variables
String localVarPath = "/state/account/page/authorized-depositors";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Account Locker Vaults Page
* Returns all the resource vaults associated with a given account locker. The returned response is in a paginated format, ordered by the most recent resource vault creation on the ledger.
* @param stateAccountLockerPageVaultsRequest (required)
* @return StateAccountLockerPageVaultsResponse
* @throws ApiException if fails to make API call
*/
public StateAccountLockerPageVaultsResponse accountLockerVaultsPage(StateAccountLockerPageVaultsRequest stateAccountLockerPageVaultsRequest) throws ApiException {
return this.accountLockerVaultsPage(stateAccountLockerPageVaultsRequest, Collections.emptyMap());
}
/**
* Get Account Locker Vaults Page
* Returns all the resource vaults associated with a given account locker. The returned response is in a paginated format, ordered by the most recent resource vault creation on the ledger.
* @param stateAccountLockerPageVaultsRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateAccountLockerPageVaultsResponse
* @throws ApiException if fails to make API call
*/
public StateAccountLockerPageVaultsResponse accountLockerVaultsPage(StateAccountLockerPageVaultsRequest stateAccountLockerPageVaultsRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateAccountLockerPageVaultsRequest;
// verify the required parameter 'stateAccountLockerPageVaultsRequest' is set
if (stateAccountLockerPageVaultsRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateAccountLockerPageVaultsRequest' when calling accountLockerVaultsPage");
}
// create path and map variables
String localVarPath = "/state/account-locker/page/vaults";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Most Recent Touch of Account Lockers
* Returns most recent state version given account locker has been touched. Touch refers to the creation of the account locker itself as well as any modification to its contents, such as resource claim, airdrop or store.
* @param stateAccountLockersTouchedAtRequest (required)
* @return StateAccountLockersTouchedAtResponse
* @throws ApiException if fails to make API call
*/
public StateAccountLockersTouchedAtResponse accountLockersTouchedAt(StateAccountLockersTouchedAtRequest stateAccountLockersTouchedAtRequest) throws ApiException {
return this.accountLockersTouchedAt(stateAccountLockersTouchedAtRequest, Collections.emptyMap());
}
/**
* Get Most Recent Touch of Account Lockers
* Returns most recent state version given account locker has been touched. Touch refers to the creation of the account locker itself as well as any modification to its contents, such as resource claim, airdrop or store.
* @param stateAccountLockersTouchedAtRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateAccountLockersTouchedAtResponse
* @throws ApiException if fails to make API call
*/
public StateAccountLockersTouchedAtResponse accountLockersTouchedAt(StateAccountLockersTouchedAtRequest stateAccountLockersTouchedAtRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateAccountLockersTouchedAtRequest;
// verify the required parameter 'stateAccountLockersTouchedAtRequest' is set
if (stateAccountLockersTouchedAtRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateAccountLockersTouchedAtRequest' when calling accountLockersTouchedAt");
}
// create path and map variables
String localVarPath = "/state/account-lockers/touched-at";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Account resource preferences
* Returns paginable collection of resource preference rules for given account.
* @param stateAccountResourcePreferencesPageRequest (required)
* @return StateAccountResourcePreferencesPageResponse
* @throws ApiException if fails to make API call
*/
public StateAccountResourcePreferencesPageResponse accountResourcePreferencesPage(StateAccountResourcePreferencesPageRequest stateAccountResourcePreferencesPageRequest) throws ApiException {
return this.accountResourcePreferencesPage(stateAccountResourcePreferencesPageRequest, Collections.emptyMap());
}
/**
* Get Account resource preferences
* Returns paginable collection of resource preference rules for given account.
* @param stateAccountResourcePreferencesPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateAccountResourcePreferencesPageResponse
* @throws ApiException if fails to make API call
*/
public StateAccountResourcePreferencesPageResponse accountResourcePreferencesPage(StateAccountResourcePreferencesPageRequest stateAccountResourcePreferencesPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateAccountResourcePreferencesPageRequest;
// verify the required parameter 'stateAccountResourcePreferencesPageRequest' is set
if (stateAccountResourcePreferencesPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateAccountResourcePreferencesPageRequest' when calling accountResourcePreferencesPage");
}
// create path and map variables
String localVarPath = "/state/account/page/resource-preferences";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get page of Global Entity Fungible Resource Vaults
* Returns vaults for fungible resource owned by a given global entity. The returned response is in a paginated format, ordered by the resource's first appearance on the ledger.
* @param stateEntityFungibleResourceVaultsPageRequest (required)
* @return StateEntityFungibleResourceVaultsPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityFungibleResourceVaultsPageResponse entityFungibleResourceVaultPage(StateEntityFungibleResourceVaultsPageRequest stateEntityFungibleResourceVaultsPageRequest) throws ApiException {
return this.entityFungibleResourceVaultPage(stateEntityFungibleResourceVaultsPageRequest, Collections.emptyMap());
}
/**
* Get page of Global Entity Fungible Resource Vaults
* Returns vaults for fungible resource owned by a given global entity. The returned response is in a paginated format, ordered by the resource's first appearance on the ledger.
* @param stateEntityFungibleResourceVaultsPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateEntityFungibleResourceVaultsPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityFungibleResourceVaultsPageResponse entityFungibleResourceVaultPage(StateEntityFungibleResourceVaultsPageRequest stateEntityFungibleResourceVaultsPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateEntityFungibleResourceVaultsPageRequest;
// verify the required parameter 'stateEntityFungibleResourceVaultsPageRequest' is set
if (stateEntityFungibleResourceVaultsPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateEntityFungibleResourceVaultsPageRequest' when calling entityFungibleResourceVaultPage");
}
// create path and map variables
String localVarPath = "/state/entity/page/fungible-vaults/";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get page of Global Entity Fungible Resource Balances
* Returns the total amount of each fungible resource owned by a given global entity. Result can be aggregated globally or per vault. The returned response is in a paginated format, ordered by the resource's first appearance on the ledger.
* @param stateEntityFungiblesPageRequest (required)
* @return StateEntityFungiblesPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityFungiblesPageResponse entityFungiblesPage(StateEntityFungiblesPageRequest stateEntityFungiblesPageRequest) throws ApiException {
return this.entityFungiblesPage(stateEntityFungiblesPageRequest, Collections.emptyMap());
}
/**
* Get page of Global Entity Fungible Resource Balances
* Returns the total amount of each fungible resource owned by a given global entity. Result can be aggregated globally or per vault. The returned response is in a paginated format, ordered by the resource's first appearance on the ledger.
* @param stateEntityFungiblesPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateEntityFungiblesPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityFungiblesPageResponse entityFungiblesPage(StateEntityFungiblesPageRequest stateEntityFungiblesPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateEntityFungiblesPageRequest;
// verify the required parameter 'stateEntityFungiblesPageRequest' is set
if (stateEntityFungiblesPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateEntityFungiblesPageRequest' when calling entityFungiblesPage");
}
// create path and map variables
String localVarPath = "/state/entity/page/fungibles/";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Entity Metadata Page
* Returns all the metadata properties associated with a given global entity. The returned response is in a paginated format, ordered by first appearance on the ledger.
* @param stateEntityMetadataPageRequest (required)
* @return StateEntityMetadataPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityMetadataPageResponse entityMetadataPage(StateEntityMetadataPageRequest stateEntityMetadataPageRequest) throws ApiException {
return this.entityMetadataPage(stateEntityMetadataPageRequest, Collections.emptyMap());
}
/**
* Get Entity Metadata Page
* Returns all the metadata properties associated with a given global entity. The returned response is in a paginated format, ordered by first appearance on the ledger.
* @param stateEntityMetadataPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateEntityMetadataPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityMetadataPageResponse entityMetadataPage(StateEntityMetadataPageRequest stateEntityMetadataPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateEntityMetadataPageRequest;
// verify the required parameter 'stateEntityMetadataPageRequest' is set
if (stateEntityMetadataPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateEntityMetadataPageRequest' when calling entityMetadataPage");
}
// create path and map variables
String localVarPath = "/state/entity/page/metadata";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get page of Non-Fungibles in Vault
* Returns all non-fungible IDs of a given non-fungible resource owned by a given entity. The returned response is in a paginated format, ordered by the resource's first appearence on the ledger.
* @param stateEntityNonFungibleIdsPageRequest (required)
* @return StateEntityNonFungibleIdsPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityNonFungibleIdsPageResponse entityNonFungibleIdsPage(StateEntityNonFungibleIdsPageRequest stateEntityNonFungibleIdsPageRequest) throws ApiException {
return this.entityNonFungibleIdsPage(stateEntityNonFungibleIdsPageRequest, Collections.emptyMap());
}
/**
* Get page of Non-Fungibles in Vault
* Returns all non-fungible IDs of a given non-fungible resource owned by a given entity. The returned response is in a paginated format, ordered by the resource's first appearence on the ledger.
* @param stateEntityNonFungibleIdsPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateEntityNonFungibleIdsPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityNonFungibleIdsPageResponse entityNonFungibleIdsPage(StateEntityNonFungibleIdsPageRequest stateEntityNonFungibleIdsPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateEntityNonFungibleIdsPageRequest;
// verify the required parameter 'stateEntityNonFungibleIdsPageRequest' is set
if (stateEntityNonFungibleIdsPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateEntityNonFungibleIdsPageRequest' when calling entityNonFungibleIdsPage");
}
// create path and map variables
String localVarPath = "/state/entity/page/non-fungible-vault/ids";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get page of Global Entity Non-Fungible Resource Vaults
* Returns vaults for non fungible resource owned by a given global entity. The returned response is in a paginated format, ordered by the resource's first appearance on the ledger.
* @param stateEntityNonFungibleResourceVaultsPageRequest (required)
* @return StateEntityNonFungibleResourceVaultsPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityNonFungibleResourceVaultsPageResponse entityNonFungibleResourceVaultPage(StateEntityNonFungibleResourceVaultsPageRequest stateEntityNonFungibleResourceVaultsPageRequest) throws ApiException {
return this.entityNonFungibleResourceVaultPage(stateEntityNonFungibleResourceVaultsPageRequest, Collections.emptyMap());
}
/**
* Get page of Global Entity Non-Fungible Resource Vaults
* Returns vaults for non fungible resource owned by a given global entity. The returned response is in a paginated format, ordered by the resource's first appearance on the ledger.
* @param stateEntityNonFungibleResourceVaultsPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateEntityNonFungibleResourceVaultsPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityNonFungibleResourceVaultsPageResponse entityNonFungibleResourceVaultPage(StateEntityNonFungibleResourceVaultsPageRequest stateEntityNonFungibleResourceVaultsPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateEntityNonFungibleResourceVaultsPageRequest;
// verify the required parameter 'stateEntityNonFungibleResourceVaultsPageRequest' is set
if (stateEntityNonFungibleResourceVaultsPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateEntityNonFungibleResourceVaultsPageRequest' when calling entityNonFungibleResourceVaultPage");
}
// create path and map variables
String localVarPath = "/state/entity/page/non-fungible-vaults/";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get page of Global Entity Non-Fungible Resource Balances
* Returns the total amount of each non-fungible resource owned by a given global entity. Result can be aggregated globally or per vault. The returned response is in a paginated format, ordered by the resource's first appearance on the ledger.
* @param stateEntityNonFungiblesPageRequest (required)
* @return StateEntityNonFungiblesPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityNonFungiblesPageResponse entityNonFungiblesPage(StateEntityNonFungiblesPageRequest stateEntityNonFungiblesPageRequest) throws ApiException {
return this.entityNonFungiblesPage(stateEntityNonFungiblesPageRequest, Collections.emptyMap());
}
/**
* Get page of Global Entity Non-Fungible Resource Balances
* Returns the total amount of each non-fungible resource owned by a given global entity. Result can be aggregated globally or per vault. The returned response is in a paginated format, ordered by the resource's first appearance on the ledger.
* @param stateEntityNonFungiblesPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateEntityNonFungiblesPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntityNonFungiblesPageResponse entityNonFungiblesPage(StateEntityNonFungiblesPageRequest stateEntityNonFungiblesPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateEntityNonFungiblesPageRequest;
// verify the required parameter 'stateEntityNonFungiblesPageRequest' is set
if (stateEntityNonFungiblesPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateEntityNonFungiblesPageRequest' when calling entityNonFungiblesPage");
}
// create path and map variables
String localVarPath = "/state/entity/page/non-fungibles/";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Entity Schema Page
* Returns all the schemas associated with a given global entity. The returned response is in a paginated format, ordered by first appearance on the ledger.
* @param stateEntitySchemaPageRequest (required)
* @return StateEntitySchemaPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntitySchemaPageResponse entitySchemaPage(StateEntitySchemaPageRequest stateEntitySchemaPageRequest) throws ApiException {
return this.entitySchemaPage(stateEntitySchemaPageRequest, Collections.emptyMap());
}
/**
* Get Entity Schema Page
* Returns all the schemas associated with a given global entity. The returned response is in a paginated format, ordered by first appearance on the ledger.
* @param stateEntitySchemaPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateEntitySchemaPageResponse
* @throws ApiException if fails to make API call
*/
public StateEntitySchemaPageResponse entitySchemaPage(StateEntitySchemaPageRequest stateEntitySchemaPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateEntitySchemaPageRequest;
// verify the required parameter 'stateEntitySchemaPageRequest' is set
if (stateEntitySchemaPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateEntitySchemaPageRequest' when calling entitySchemaPage");
}
// create path and map variables
String localVarPath = "/state/entity/page/schemas";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get KeyValueStore Data
* Returns data (value) associated with a given key of a given key-value store. [Check detailed documentation for explanation](#section/How-to-query-the-content-of-a-key-value-store-inside-a-component)
* @param stateKeyValueStoreDataRequest (required)
* @return StateKeyValueStoreDataResponse
* @throws ApiException if fails to make API call
*/
public StateKeyValueStoreDataResponse keyValueStoreData(StateKeyValueStoreDataRequest stateKeyValueStoreDataRequest) throws ApiException {
return this.keyValueStoreData(stateKeyValueStoreDataRequest, Collections.emptyMap());
}
/**
* Get KeyValueStore Data
* Returns data (value) associated with a given key of a given key-value store. [Check detailed documentation for explanation](#section/How-to-query-the-content-of-a-key-value-store-inside-a-component)
* @param stateKeyValueStoreDataRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateKeyValueStoreDataResponse
* @throws ApiException if fails to make API call
*/
public StateKeyValueStoreDataResponse keyValueStoreData(StateKeyValueStoreDataRequest stateKeyValueStoreDataRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateKeyValueStoreDataRequest;
// verify the required parameter 'stateKeyValueStoreDataRequest' is set
if (stateKeyValueStoreDataRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateKeyValueStoreDataRequest' when calling keyValueStoreData");
}
// create path and map variables
String localVarPath = "/state/key-value-store/data";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get KeyValueStore Keys
* Allows to iterate over key value store keys.
* @param stateKeyValueStoreKeysRequest (required)
* @return StateKeyValueStoreKeysResponse
* @throws ApiException if fails to make API call
*/
public StateKeyValueStoreKeysResponse keyValueStoreKeys(StateKeyValueStoreKeysRequest stateKeyValueStoreKeysRequest) throws ApiException {
return this.keyValueStoreKeys(stateKeyValueStoreKeysRequest, Collections.emptyMap());
}
/**
* Get KeyValueStore Keys
* Allows to iterate over key value store keys.
* @param stateKeyValueStoreKeysRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateKeyValueStoreKeysResponse
* @throws ApiException if fails to make API call
*/
public StateKeyValueStoreKeysResponse keyValueStoreKeys(StateKeyValueStoreKeysRequest stateKeyValueStoreKeysRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateKeyValueStoreKeysRequest;
// verify the required parameter 'stateKeyValueStoreKeysRequest' is set
if (stateKeyValueStoreKeysRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateKeyValueStoreKeysRequest' when calling keyValueStoreKeys");
}
// create path and map variables
String localVarPath = "/state/key-value-store/keys";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Non-Fungible Data
* Returns data associated with a given non-fungible ID of a given non-fungible resource.
* @param stateNonFungibleDataRequest (required)
* @return StateNonFungibleDataResponse
* @throws ApiException if fails to make API call
*/
public StateNonFungibleDataResponse nonFungibleData(StateNonFungibleDataRequest stateNonFungibleDataRequest) throws ApiException {
return this.nonFungibleData(stateNonFungibleDataRequest, Collections.emptyMap());
}
/**
* Get Non-Fungible Data
* Returns data associated with a given non-fungible ID of a given non-fungible resource.
* @param stateNonFungibleDataRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateNonFungibleDataResponse
* @throws ApiException if fails to make API call
*/
public StateNonFungibleDataResponse nonFungibleData(StateNonFungibleDataRequest stateNonFungibleDataRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateNonFungibleDataRequest;
// verify the required parameter 'stateNonFungibleDataRequest' is set
if (stateNonFungibleDataRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateNonFungibleDataRequest' when calling nonFungibleData");
}
// create path and map variables
String localVarPath = "/state/non-fungible/data";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get page of Non-Fungible Ids in Resource Collection
* Returns the non-fungible IDs of a given non-fungible resource. Returned response is in a paginated format, ordered by their first appearance on the ledger.
* @param stateNonFungibleIdsRequest (required)
* @return StateNonFungibleIdsResponse
* @throws ApiException if fails to make API call
*/
public StateNonFungibleIdsResponse nonFungibleIds(StateNonFungibleIdsRequest stateNonFungibleIdsRequest) throws ApiException {
return this.nonFungibleIds(stateNonFungibleIdsRequest, Collections.emptyMap());
}
/**
* Get page of Non-Fungible Ids in Resource Collection
* Returns the non-fungible IDs of a given non-fungible resource. Returned response is in a paginated format, ordered by their first appearance on the ledger.
* @param stateNonFungibleIdsRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateNonFungibleIdsResponse
* @throws ApiException if fails to make API call
*/
public StateNonFungibleIdsResponse nonFungibleIds(StateNonFungibleIdsRequest stateNonFungibleIdsRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateNonFungibleIdsRequest;
// verify the required parameter 'stateNonFungibleIdsRequest' is set
if (stateNonFungibleIdsRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateNonFungibleIdsRequest' when calling nonFungibleIds");
}
// create path and map variables
String localVarPath = "/state/non-fungible/ids";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Non-Fungible Location
* Returns location of a given non-fungible ID.
* @param stateNonFungibleLocationRequest (required)
* @return StateNonFungibleLocationResponse
* @throws ApiException if fails to make API call
*/
public StateNonFungibleLocationResponse nonFungibleLocation(StateNonFungibleLocationRequest stateNonFungibleLocationRequest) throws ApiException {
return this.nonFungibleLocation(stateNonFungibleLocationRequest, Collections.emptyMap());
}
/**
* Get Non-Fungible Location
* Returns location of a given non-fungible ID.
* @param stateNonFungibleLocationRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateNonFungibleLocationResponse
* @throws ApiException if fails to make API call
*/
public StateNonFungibleLocationResponse nonFungibleLocation(StateNonFungibleLocationRequest stateNonFungibleLocationRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateNonFungibleLocationRequest;
// verify the required parameter 'stateNonFungibleLocationRequest' is set
if (stateNonFungibleLocationRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateNonFungibleLocationRequest' when calling nonFungibleLocation");
}
// create path and map variables
String localVarPath = "/state/non-fungible/location";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Package Blueprints Page
* Returns all the blueprints associated with a given package entity. The returned response is in a paginated format, ordered by first appearance on the ledger.
* @param statePackageBlueprintPageRequest (required)
* @return StatePackageBlueprintPageResponse
* @throws ApiException if fails to make API call
*/
public StatePackageBlueprintPageResponse packageBlueprintPage(StatePackageBlueprintPageRequest statePackageBlueprintPageRequest) throws ApiException {
return this.packageBlueprintPage(statePackageBlueprintPageRequest, Collections.emptyMap());
}
/**
* Get Package Blueprints Page
* Returns all the blueprints associated with a given package entity. The returned response is in a paginated format, ordered by first appearance on the ledger.
* @param statePackageBlueprintPageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StatePackageBlueprintPageResponse
* @throws ApiException if fails to make API call
*/
public StatePackageBlueprintPageResponse packageBlueprintPage(StatePackageBlueprintPageRequest statePackageBlueprintPageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = statePackageBlueprintPageRequest;
// verify the required parameter 'statePackageBlueprintPageRequest' is set
if (statePackageBlueprintPageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'statePackageBlueprintPageRequest' when calling packageBlueprintPage");
}
// create path and map variables
String localVarPath = "/state/package/page/blueprints";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Package Codes Page
* Returns all the codes associated with a given package entity. The returned response is in a paginated format, ordered by first appearance on the ledger.
* @param statePackageCodePageRequest (required)
* @return StatePackageCodePageResponse
* @throws ApiException if fails to make API call
*/
public StatePackageCodePageResponse packageCodePage(StatePackageCodePageRequest statePackageCodePageRequest) throws ApiException {
return this.packageCodePage(statePackageCodePageRequest, Collections.emptyMap());
}
/**
* Get Package Codes Page
* Returns all the codes associated with a given package entity. The returned response is in a paginated format, ordered by first appearance on the ledger.
* @param statePackageCodePageRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StatePackageCodePageResponse
* @throws ApiException if fails to make API call
*/
public StatePackageCodePageResponse packageCodePage(StatePackageCodePageRequest statePackageCodePageRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = statePackageCodePageRequest;
// verify the required parameter 'statePackageCodePageRequest' is set
if (statePackageCodePageRequest == null) {
throw new ApiException(400, "Missing the required parameter 'statePackageCodePageRequest' when calling packageCodePage");
}
// create path and map variables
String localVarPath = "/state/package/page/codes";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Entity Details
* Returns detailed information for collection of entities. Aggregate resources globally by default.
* @param stateEntityDetailsRequest (required)
* @return StateEntityDetailsResponse
* @throws ApiException if fails to make API call
*/
public StateEntityDetailsResponse stateEntityDetails(StateEntityDetailsRequest stateEntityDetailsRequest) throws ApiException {
return this.stateEntityDetails(stateEntityDetailsRequest, Collections.emptyMap());
}
/**
* Get Entity Details
* Returns detailed information for collection of entities. Aggregate resources globally by default.
* @param stateEntityDetailsRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateEntityDetailsResponse
* @throws ApiException if fails to make API call
*/
public StateEntityDetailsResponse stateEntityDetails(StateEntityDetailsRequest stateEntityDetailsRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateEntityDetailsRequest;
// verify the required parameter 'stateEntityDetailsRequest' is set
if (stateEntityDetailsRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateEntityDetailsRequest' when calling stateEntityDetails");
}
// create path and map variables
String localVarPath = "/state/entity/details";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Validators List
*
* @param stateValidatorsListRequest (required)
* @return StateValidatorsListResponse
* @throws ApiException if fails to make API call
*/
public StateValidatorsListResponse stateValidatorsList(StateValidatorsListRequest stateValidatorsListRequest) throws ApiException {
return this.stateValidatorsList(stateValidatorsListRequest, Collections.emptyMap());
}
/**
* Get Validators List
*
* @param stateValidatorsListRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @return StateValidatorsListResponse
* @throws ApiException if fails to make API call
*/
public StateValidatorsListResponse stateValidatorsList(StateValidatorsListRequest stateValidatorsListRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = stateValidatorsListRequest;
// verify the required parameter 'stateValidatorsListRequest' is set
if (stateValidatorsListRequest == null) {
throw new ApiException(400, "Missing the required parameter 'stateValidatorsListRequest' when calling stateValidatorsList");
}
// create path and map variables
String localVarPath = "/state/validators/list";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List