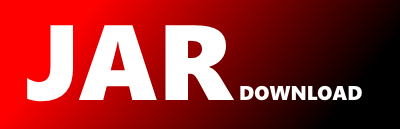
live.radix.gateway.model.StreamTransactionsRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of babylon-gateway-client Show documentation
Show all versions of babylon-gateway-client Show documentation
A generated Client library for Radix Babylon Gateway API.
/*
* Radix Gateway API - Babylon
* This API is exposed by the Babylon Radix Gateway to enable clients to efficiently query current and historic state on the RadixDLT ledger, and intelligently handle transaction submission. It is designed for use by wallets and explorers, and for light queries from front-end dApps. For exchange/asset integrations, back-end dApp integrations, or simple use cases, you should consider using the Core API on a Node. A Gateway is only needed for reading historic snapshots of ledger states or a more robust set-up. The Gateway API is implemented by the [Network Gateway](https://github.com/radixdlt/babylon-gateway), which is configured to read from [full node(s)](https://github.com/radixdlt/babylon-node) to extract and index data from the network. This document is an API reference documentation, visit [User Guide](https://docs.radixdlt.com/) to learn more about how to run a Gateway of your own. ## Migration guide Please see [the latest release notes](https://github.com/radixdlt/babylon-gateway/releases). ## Integration and forward compatibility guarantees All responses may have additional fields added at any release, so clients are advised to use JSON parsers which ignore unknown fields on JSON objects. When the Radix protocol is updated, new functionality may be added, and so discriminated unions returned by the API may need to be updated to have new variants added, corresponding to the updated data. Clients may need to update in advance to be able to handle these new variants when a protocol update comes out. On the very rare occasions we need to make breaking changes to the API, these will be warned in advance with deprecation notices on previous versions. These deprecation notices will include a safe migration path. Deprecation notes or breaking changes will be flagged clearly in release notes for new versions of the Gateway. The Gateway DB schema is not subject to any compatibility guarantees, and may be changed at any release. DB changes will be flagged in the release notes so clients doing custom DB integrations can prepare.
*
* The version of the OpenAPI document: v1.9.2-L
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package live.radix.gateway.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import live.radix.gateway.model.LedgerStateSelector;
import live.radix.gateway.model.StreamTransactionsRequestAllOfManifestClassFilter;
import live.radix.gateway.model.StreamTransactionsRequestEventFilterItem;
import live.radix.gateway.model.TransactionDetailsOptIns;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* StreamTransactionsRequest
*/
@JsonPropertyOrder({
StreamTransactionsRequest.JSON_PROPERTY_AT_LEDGER_STATE,
StreamTransactionsRequest.JSON_PROPERTY_FROM_LEDGER_STATE,
StreamTransactionsRequest.JSON_PROPERTY_CURSOR,
StreamTransactionsRequest.JSON_PROPERTY_LIMIT_PER_PAGE,
StreamTransactionsRequest.JSON_PROPERTY_KIND_FILTER,
StreamTransactionsRequest.JSON_PROPERTY_MANIFEST_ACCOUNTS_WITHDRAWN_FROM_FILTER,
StreamTransactionsRequest.JSON_PROPERTY_MANIFEST_ACCOUNTS_DEPOSITED_INTO_FILTER,
StreamTransactionsRequest.JSON_PROPERTY_MANIFEST_BADGES_PRESENTED_FILTER,
StreamTransactionsRequest.JSON_PROPERTY_MANIFEST_RESOURCES_FILTER,
StreamTransactionsRequest.JSON_PROPERTY_AFFECTED_GLOBAL_ENTITIES_FILTER,
StreamTransactionsRequest.JSON_PROPERTY_AFFECTED_GLOBAL_ENTITIES_FILTER_TYPE,
StreamTransactionsRequest.JSON_PROPERTY_EVENTS_FILTER,
StreamTransactionsRequest.JSON_PROPERTY_ACCOUNTS_WITH_MANIFEST_OWNER_METHOD_CALLS,
StreamTransactionsRequest.JSON_PROPERTY_ACCOUNTS_WITHOUT_MANIFEST_OWNER_METHOD_CALLS,
StreamTransactionsRequest.JSON_PROPERTY_MANIFEST_CLASS_FILTER,
StreamTransactionsRequest.JSON_PROPERTY_EVENT_GLOBAL_EMITTERS_FILTER,
StreamTransactionsRequest.JSON_PROPERTY_ORDER,
StreamTransactionsRequest.JSON_PROPERTY_OPT_INS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-12-12T19:38:04.213407200+02:00[Europe/Kiev]", comments = "Generator version: 7.7.0")
public class StreamTransactionsRequest {
public static final String JSON_PROPERTY_AT_LEDGER_STATE = "at_ledger_state";
private LedgerStateSelector atLedgerState;
public static final String JSON_PROPERTY_FROM_LEDGER_STATE = "from_ledger_state";
private LedgerStateSelector fromLedgerState;
public static final String JSON_PROPERTY_CURSOR = "cursor";
private String cursor;
public static final String JSON_PROPERTY_LIMIT_PER_PAGE = "limit_per_page";
private JsonNullable limitPerPage = JsonNullable.undefined();
/**
* Limit returned transactions by their kind. Defaults to `user`.
*/
public enum KindFilterEnum {
USER("User"),
EPOCH_CHANGE("EpochChange"),
ALL("All");
private String value;
KindFilterEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static KindFilterEnum fromValue(String value) {
for (KindFilterEnum b : KindFilterEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_KIND_FILTER = "kind_filter";
private KindFilterEnum kindFilter;
public static final String JSON_PROPERTY_MANIFEST_ACCOUNTS_WITHDRAWN_FROM_FILTER = "manifest_accounts_withdrawn_from_filter";
private List manifestAccountsWithdrawnFromFilter = new ArrayList<>();
public static final String JSON_PROPERTY_MANIFEST_ACCOUNTS_DEPOSITED_INTO_FILTER = "manifest_accounts_deposited_into_filter";
private List manifestAccountsDepositedIntoFilter = new ArrayList<>();
public static final String JSON_PROPERTY_MANIFEST_BADGES_PRESENTED_FILTER = "manifest_badges_presented_filter";
private List manifestBadgesPresentedFilter = new ArrayList<>();
public static final String JSON_PROPERTY_MANIFEST_RESOURCES_FILTER = "manifest_resources_filter";
private List manifestResourcesFilter = new ArrayList<>();
public static final String JSON_PROPERTY_AFFECTED_GLOBAL_ENTITIES_FILTER = "affected_global_entities_filter";
private List affectedGlobalEntitiesFilter = new ArrayList<>();
/**
* Whether the filter should require all addresses (AND) or any of them (OR) to be present in the transaction. Default - \"And\".
*/
public enum AffectedGlobalEntitiesFilterTypeEnum {
AND("And"),
OR("Or");
private String value;
AffectedGlobalEntitiesFilterTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static AffectedGlobalEntitiesFilterTypeEnum fromValue(String value) {
for (AffectedGlobalEntitiesFilterTypeEnum b : AffectedGlobalEntitiesFilterTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_AFFECTED_GLOBAL_ENTITIES_FILTER_TYPE = "affected_global_entities_filter_type";
private AffectedGlobalEntitiesFilterTypeEnum affectedGlobalEntitiesFilterType = AffectedGlobalEntitiesFilterTypeEnum.AND;
public static final String JSON_PROPERTY_EVENTS_FILTER = "events_filter";
private List eventsFilter = new ArrayList<>();
public static final String JSON_PROPERTY_ACCOUNTS_WITH_MANIFEST_OWNER_METHOD_CALLS = "accounts_with_manifest_owner_method_calls";
private List accountsWithManifestOwnerMethodCalls = new ArrayList<>();
public static final String JSON_PROPERTY_ACCOUNTS_WITHOUT_MANIFEST_OWNER_METHOD_CALLS = "accounts_without_manifest_owner_method_calls";
private List accountsWithoutManifestOwnerMethodCalls = new ArrayList<>();
public static final String JSON_PROPERTY_MANIFEST_CLASS_FILTER = "manifest_class_filter";
private StreamTransactionsRequestAllOfManifestClassFilter manifestClassFilter;
public static final String JSON_PROPERTY_EVENT_GLOBAL_EMITTERS_FILTER = "event_global_emitters_filter";
private List eventGlobalEmittersFilter = new ArrayList<>();
/**
* Configures the order of returned result set. Defaults to `desc`.
*/
public enum OrderEnum {
ASC("Asc"),
DESC("Desc");
private String value;
OrderEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static OrderEnum fromValue(String value) {
for (OrderEnum b : OrderEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_ORDER = "order";
private OrderEnum order;
public static final String JSON_PROPERTY_OPT_INS = "opt_ins";
private TransactionDetailsOptIns optIns;
public StreamTransactionsRequest() {
}
public StreamTransactionsRequest atLedgerState(LedgerStateSelector atLedgerState) {
this.atLedgerState = atLedgerState;
return this;
}
/**
* Get atLedgerState
* @return atLedgerState
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AT_LEDGER_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LedgerStateSelector getAtLedgerState() {
return atLedgerState;
}
@JsonProperty(JSON_PROPERTY_AT_LEDGER_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAtLedgerState(LedgerStateSelector atLedgerState) {
this.atLedgerState = atLedgerState;
}
public StreamTransactionsRequest fromLedgerState(LedgerStateSelector fromLedgerState) {
this.fromLedgerState = fromLedgerState;
return this;
}
/**
* Get fromLedgerState
* @return fromLedgerState
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_FROM_LEDGER_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LedgerStateSelector getFromLedgerState() {
return fromLedgerState;
}
@JsonProperty(JSON_PROPERTY_FROM_LEDGER_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFromLedgerState(LedgerStateSelector fromLedgerState) {
this.fromLedgerState = fromLedgerState;
}
public StreamTransactionsRequest cursor(String cursor) {
this.cursor = cursor;
return this;
}
/**
* This cursor allows forward pagination, by providing the cursor from the previous request.
* @return cursor
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CURSOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCursor() {
return cursor;
}
@JsonProperty(JSON_PROPERTY_CURSOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCursor(String cursor) {
this.cursor = cursor;
}
public StreamTransactionsRequest limitPerPage(Integer limitPerPage) {
this.limitPerPage = JsonNullable.of(limitPerPage);
return this;
}
/**
* The page size requested.
* @return limitPerPage
*/
@javax.annotation.Nullable
@JsonIgnore
public Integer getLimitPerPage() {
return limitPerPage.orElse(null);
}
@JsonProperty(JSON_PROPERTY_LIMIT_PER_PAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getLimitPerPage_JsonNullable() {
return limitPerPage;
}
@JsonProperty(JSON_PROPERTY_LIMIT_PER_PAGE)
public void setLimitPerPage_JsonNullable(JsonNullable limitPerPage) {
this.limitPerPage = limitPerPage;
}
public void setLimitPerPage(Integer limitPerPage) {
this.limitPerPage = JsonNullable.of(limitPerPage);
}
public StreamTransactionsRequest kindFilter(KindFilterEnum kindFilter) {
this.kindFilter = kindFilter;
return this;
}
/**
* Limit returned transactions by their kind. Defaults to `user`.
* @return kindFilter
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_KIND_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public KindFilterEnum getKindFilter() {
return kindFilter;
}
@JsonProperty(JSON_PROPERTY_KIND_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setKindFilter(KindFilterEnum kindFilter) {
this.kindFilter = kindFilter;
}
public StreamTransactionsRequest manifestAccountsWithdrawnFromFilter(List manifestAccountsWithdrawnFromFilter) {
this.manifestAccountsWithdrawnFromFilter = manifestAccountsWithdrawnFromFilter;
return this;
}
public StreamTransactionsRequest addManifestAccountsWithdrawnFromFilterItem(String manifestAccountsWithdrawnFromFilterItem) {
if (this.manifestAccountsWithdrawnFromFilter == null) {
this.manifestAccountsWithdrawnFromFilter = new ArrayList<>();
}
this.manifestAccountsWithdrawnFromFilter.add(manifestAccountsWithdrawnFromFilterItem);
return this;
}
/**
* Allows specifying an array of account addresses. If specified, the response will contain only transactions with a manifest containing withdrawals from the given accounts.
* @return manifestAccountsWithdrawnFromFilter
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MANIFEST_ACCOUNTS_WITHDRAWN_FROM_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getManifestAccountsWithdrawnFromFilter() {
return manifestAccountsWithdrawnFromFilter;
}
@JsonProperty(JSON_PROPERTY_MANIFEST_ACCOUNTS_WITHDRAWN_FROM_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setManifestAccountsWithdrawnFromFilter(List manifestAccountsWithdrawnFromFilter) {
this.manifestAccountsWithdrawnFromFilter = manifestAccountsWithdrawnFromFilter;
}
public StreamTransactionsRequest manifestAccountsDepositedIntoFilter(List manifestAccountsDepositedIntoFilter) {
this.manifestAccountsDepositedIntoFilter = manifestAccountsDepositedIntoFilter;
return this;
}
public StreamTransactionsRequest addManifestAccountsDepositedIntoFilterItem(String manifestAccountsDepositedIntoFilterItem) {
if (this.manifestAccountsDepositedIntoFilter == null) {
this.manifestAccountsDepositedIntoFilter = new ArrayList<>();
}
this.manifestAccountsDepositedIntoFilter.add(manifestAccountsDepositedIntoFilterItem);
return this;
}
/**
* Similar to `manifest_accounts_withdrawn_from_filter`, but will return only transactions with a manifest containing deposits to the given accounts.
* @return manifestAccountsDepositedIntoFilter
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MANIFEST_ACCOUNTS_DEPOSITED_INTO_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getManifestAccountsDepositedIntoFilter() {
return manifestAccountsDepositedIntoFilter;
}
@JsonProperty(JSON_PROPERTY_MANIFEST_ACCOUNTS_DEPOSITED_INTO_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setManifestAccountsDepositedIntoFilter(List manifestAccountsDepositedIntoFilter) {
this.manifestAccountsDepositedIntoFilter = manifestAccountsDepositedIntoFilter;
}
public StreamTransactionsRequest manifestBadgesPresentedFilter(List manifestBadgesPresentedFilter) {
this.manifestBadgesPresentedFilter = manifestBadgesPresentedFilter;
return this;
}
public StreamTransactionsRequest addManifestBadgesPresentedFilterItem(String manifestBadgesPresentedFilterItem) {
if (this.manifestBadgesPresentedFilter == null) {
this.manifestBadgesPresentedFilter = new ArrayList<>();
}
this.manifestBadgesPresentedFilter.add(manifestBadgesPresentedFilterItem);
return this;
}
/**
* Allows specifying array of badge resource addresses. If specified, the response will contain only transactions where the given badges were presented.
* @return manifestBadgesPresentedFilter
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MANIFEST_BADGES_PRESENTED_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getManifestBadgesPresentedFilter() {
return manifestBadgesPresentedFilter;
}
@JsonProperty(JSON_PROPERTY_MANIFEST_BADGES_PRESENTED_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setManifestBadgesPresentedFilter(List manifestBadgesPresentedFilter) {
this.manifestBadgesPresentedFilter = manifestBadgesPresentedFilter;
}
public StreamTransactionsRequest manifestResourcesFilter(List manifestResourcesFilter) {
this.manifestResourcesFilter = manifestResourcesFilter;
return this;
}
public StreamTransactionsRequest addManifestResourcesFilterItem(String manifestResourcesFilterItem) {
if (this.manifestResourcesFilter == null) {
this.manifestResourcesFilter = new ArrayList<>();
}
this.manifestResourcesFilter.add(manifestResourcesFilterItem);
return this;
}
/**
* Allows specifying array of resource addresses. If specified, the response will contain only transactions containing the given resources in the manifest (regardless of their usage).
* @return manifestResourcesFilter
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MANIFEST_RESOURCES_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getManifestResourcesFilter() {
return manifestResourcesFilter;
}
@JsonProperty(JSON_PROPERTY_MANIFEST_RESOURCES_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setManifestResourcesFilter(List manifestResourcesFilter) {
this.manifestResourcesFilter = manifestResourcesFilter;
}
public StreamTransactionsRequest affectedGlobalEntitiesFilter(List affectedGlobalEntitiesFilter) {
this.affectedGlobalEntitiesFilter = affectedGlobalEntitiesFilter;
return this;
}
public StreamTransactionsRequest addAffectedGlobalEntitiesFilterItem(String affectedGlobalEntitiesFilterItem) {
if (this.affectedGlobalEntitiesFilter == null) {
this.affectedGlobalEntitiesFilter = new ArrayList<>();
}
this.affectedGlobalEntitiesFilter.add(affectedGlobalEntitiesFilterItem);
return this;
}
/**
* Allows specifying an array of global addresses. If specified, the response will contain transactions that affected all of the given global entities. A global entity is marked as \"affected\" by a transaction if any of its state (or its descendents' state) was modified as a result of the transaction. For performance reasons consensus manager and transaction tracker are excluded from that filter.
* @return affectedGlobalEntitiesFilter
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AFFECTED_GLOBAL_ENTITIES_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAffectedGlobalEntitiesFilter() {
return affectedGlobalEntitiesFilter;
}
@JsonProperty(JSON_PROPERTY_AFFECTED_GLOBAL_ENTITIES_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAffectedGlobalEntitiesFilter(List affectedGlobalEntitiesFilter) {
this.affectedGlobalEntitiesFilter = affectedGlobalEntitiesFilter;
}
public StreamTransactionsRequest affectedGlobalEntitiesFilterType(AffectedGlobalEntitiesFilterTypeEnum affectedGlobalEntitiesFilterType) {
this.affectedGlobalEntitiesFilterType = affectedGlobalEntitiesFilterType;
return this;
}
/**
* Whether the filter should require all addresses (AND) or any of them (OR) to be present in the transaction. Default - \"And\".
* @return affectedGlobalEntitiesFilterType
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AFFECTED_GLOBAL_ENTITIES_FILTER_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AffectedGlobalEntitiesFilterTypeEnum getAffectedGlobalEntitiesFilterType() {
return affectedGlobalEntitiesFilterType;
}
@JsonProperty(JSON_PROPERTY_AFFECTED_GLOBAL_ENTITIES_FILTER_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAffectedGlobalEntitiesFilterType(AffectedGlobalEntitiesFilterTypeEnum affectedGlobalEntitiesFilterType) {
this.affectedGlobalEntitiesFilterType = affectedGlobalEntitiesFilterType;
}
public StreamTransactionsRequest eventsFilter(List eventsFilter) {
this.eventsFilter = eventsFilter;
return this;
}
public StreamTransactionsRequest addEventsFilterItem(StreamTransactionsRequestEventFilterItem eventsFilterItem) {
if (this.eventsFilter == null) {
this.eventsFilter = new ArrayList<>();
}
this.eventsFilter.add(eventsFilterItem);
return this;
}
/**
* Filters the transaction stream to transactions which emitted at least one event matching each filter (each filter can be satisfied by a different event). Currently *only* deposit and withdrawal events emitted by an internal vault entity are tracked. For the purpose of filtering, the emitter address is replaced by the global ancestor of the emitter, for example, the top-level account / component which contains the vault which emitted the event.
* @return eventsFilter
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_EVENTS_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getEventsFilter() {
return eventsFilter;
}
@JsonProperty(JSON_PROPERTY_EVENTS_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEventsFilter(List eventsFilter) {
this.eventsFilter = eventsFilter;
}
public StreamTransactionsRequest accountsWithManifestOwnerMethodCalls(List accountsWithManifestOwnerMethodCalls) {
this.accountsWithManifestOwnerMethodCalls = accountsWithManifestOwnerMethodCalls;
return this;
}
public StreamTransactionsRequest addAccountsWithManifestOwnerMethodCallsItem(String accountsWithManifestOwnerMethodCallsItem) {
if (this.accountsWithManifestOwnerMethodCalls == null) {
this.accountsWithManifestOwnerMethodCalls = new ArrayList<>();
}
this.accountsWithManifestOwnerMethodCalls.add(accountsWithManifestOwnerMethodCallsItem);
return this;
}
/**
* Allows specifying an array of account addresses. If specified, the response will contain only transactions that, for all specified accounts, contain manifest method calls to that account which require the owner role. See the [account docs](https://docs.radixdlt.com/docs/account) for more information.
* @return accountsWithManifestOwnerMethodCalls
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ACCOUNTS_WITH_MANIFEST_OWNER_METHOD_CALLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAccountsWithManifestOwnerMethodCalls() {
return accountsWithManifestOwnerMethodCalls;
}
@JsonProperty(JSON_PROPERTY_ACCOUNTS_WITH_MANIFEST_OWNER_METHOD_CALLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccountsWithManifestOwnerMethodCalls(List accountsWithManifestOwnerMethodCalls) {
this.accountsWithManifestOwnerMethodCalls = accountsWithManifestOwnerMethodCalls;
}
public StreamTransactionsRequest accountsWithoutManifestOwnerMethodCalls(List accountsWithoutManifestOwnerMethodCalls) {
this.accountsWithoutManifestOwnerMethodCalls = accountsWithoutManifestOwnerMethodCalls;
return this;
}
public StreamTransactionsRequest addAccountsWithoutManifestOwnerMethodCallsItem(String accountsWithoutManifestOwnerMethodCallsItem) {
if (this.accountsWithoutManifestOwnerMethodCalls == null) {
this.accountsWithoutManifestOwnerMethodCalls = new ArrayList<>();
}
this.accountsWithoutManifestOwnerMethodCalls.add(accountsWithoutManifestOwnerMethodCallsItem);
return this;
}
/**
* Allows specifying an array of account addresses. If specified, the response will contain only transactions that, for all specified accounts, do NOT contain manifest method calls to that account which require owner role. See the [account docs](https://docs.radixdlt.com/docs/account) for more information.
* @return accountsWithoutManifestOwnerMethodCalls
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ACCOUNTS_WITHOUT_MANIFEST_OWNER_METHOD_CALLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAccountsWithoutManifestOwnerMethodCalls() {
return accountsWithoutManifestOwnerMethodCalls;
}
@JsonProperty(JSON_PROPERTY_ACCOUNTS_WITHOUT_MANIFEST_OWNER_METHOD_CALLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccountsWithoutManifestOwnerMethodCalls(List accountsWithoutManifestOwnerMethodCalls) {
this.accountsWithoutManifestOwnerMethodCalls = accountsWithoutManifestOwnerMethodCalls;
}
public StreamTransactionsRequest manifestClassFilter(StreamTransactionsRequestAllOfManifestClassFilter manifestClassFilter) {
this.manifestClassFilter = manifestClassFilter;
return this;
}
/**
* Get manifestClassFilter
* @return manifestClassFilter
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MANIFEST_CLASS_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public StreamTransactionsRequestAllOfManifestClassFilter getManifestClassFilter() {
return manifestClassFilter;
}
@JsonProperty(JSON_PROPERTY_MANIFEST_CLASS_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setManifestClassFilter(StreamTransactionsRequestAllOfManifestClassFilter manifestClassFilter) {
this.manifestClassFilter = manifestClassFilter;
}
public StreamTransactionsRequest eventGlobalEmittersFilter(List eventGlobalEmittersFilter) {
this.eventGlobalEmittersFilter = eventGlobalEmittersFilter;
return this;
}
public StreamTransactionsRequest addEventGlobalEmittersFilterItem(String eventGlobalEmittersFilterItem) {
if (this.eventGlobalEmittersFilter == null) {
this.eventGlobalEmittersFilter = new ArrayList<>();
}
this.eventGlobalEmittersFilter.add(eventGlobalEmittersFilterItem);
return this;
}
/**
* Allows specifying an array of global addresses. If specified, the response will contain transactions in which all entities emitted events. If an event was published by an internal entity, it is going to be indexed as it is a global ancestor. For performance reasons events published by consensus manager and native XRD resource are excluded from that filter.
* @return eventGlobalEmittersFilter
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_EVENT_GLOBAL_EMITTERS_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getEventGlobalEmittersFilter() {
return eventGlobalEmittersFilter;
}
@JsonProperty(JSON_PROPERTY_EVENT_GLOBAL_EMITTERS_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEventGlobalEmittersFilter(List eventGlobalEmittersFilter) {
this.eventGlobalEmittersFilter = eventGlobalEmittersFilter;
}
public StreamTransactionsRequest order(OrderEnum order) {
this.order = order;
return this;
}
/**
* Configures the order of returned result set. Defaults to `desc`.
* @return order
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ORDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OrderEnum getOrder() {
return order;
}
@JsonProperty(JSON_PROPERTY_ORDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOrder(OrderEnum order) {
this.order = order;
}
public StreamTransactionsRequest optIns(TransactionDetailsOptIns optIns) {
this.optIns = optIns;
return this;
}
/**
* Get optIns
* @return optIns
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OPT_INS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TransactionDetailsOptIns getOptIns() {
return optIns;
}
@JsonProperty(JSON_PROPERTY_OPT_INS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOptIns(TransactionDetailsOptIns optIns) {
this.optIns = optIns;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
StreamTransactionsRequest streamTransactionsRequest = (StreamTransactionsRequest) o;
return Objects.equals(this.atLedgerState, streamTransactionsRequest.atLedgerState) &&
Objects.equals(this.fromLedgerState, streamTransactionsRequest.fromLedgerState) &&
Objects.equals(this.cursor, streamTransactionsRequest.cursor) &&
equalsNullable(this.limitPerPage, streamTransactionsRequest.limitPerPage) &&
Objects.equals(this.kindFilter, streamTransactionsRequest.kindFilter) &&
Objects.equals(this.manifestAccountsWithdrawnFromFilter, streamTransactionsRequest.manifestAccountsWithdrawnFromFilter) &&
Objects.equals(this.manifestAccountsDepositedIntoFilter, streamTransactionsRequest.manifestAccountsDepositedIntoFilter) &&
Objects.equals(this.manifestBadgesPresentedFilter, streamTransactionsRequest.manifestBadgesPresentedFilter) &&
Objects.equals(this.manifestResourcesFilter, streamTransactionsRequest.manifestResourcesFilter) &&
Objects.equals(this.affectedGlobalEntitiesFilter, streamTransactionsRequest.affectedGlobalEntitiesFilter) &&
Objects.equals(this.affectedGlobalEntitiesFilterType, streamTransactionsRequest.affectedGlobalEntitiesFilterType) &&
Objects.equals(this.eventsFilter, streamTransactionsRequest.eventsFilter) &&
Objects.equals(this.accountsWithManifestOwnerMethodCalls, streamTransactionsRequest.accountsWithManifestOwnerMethodCalls) &&
Objects.equals(this.accountsWithoutManifestOwnerMethodCalls, streamTransactionsRequest.accountsWithoutManifestOwnerMethodCalls) &&
Objects.equals(this.manifestClassFilter, streamTransactionsRequest.manifestClassFilter) &&
Objects.equals(this.eventGlobalEmittersFilter, streamTransactionsRequest.eventGlobalEmittersFilter) &&
Objects.equals(this.order, streamTransactionsRequest.order) &&
Objects.equals(this.optIns, streamTransactionsRequest.optIns);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(atLedgerState, fromLedgerState, cursor, hashCodeNullable(limitPerPage), kindFilter, manifestAccountsWithdrawnFromFilter, manifestAccountsDepositedIntoFilter, manifestBadgesPresentedFilter, manifestResourcesFilter, affectedGlobalEntitiesFilter, affectedGlobalEntitiesFilterType, eventsFilter, accountsWithManifestOwnerMethodCalls, accountsWithoutManifestOwnerMethodCalls, manifestClassFilter, eventGlobalEmittersFilter, order, optIns);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class StreamTransactionsRequest {\n");
sb.append(" atLedgerState: ").append(toIndentedString(atLedgerState)).append("\n");
sb.append(" fromLedgerState: ").append(toIndentedString(fromLedgerState)).append("\n");
sb.append(" cursor: ").append(toIndentedString(cursor)).append("\n");
sb.append(" limitPerPage: ").append(toIndentedString(limitPerPage)).append("\n");
sb.append(" kindFilter: ").append(toIndentedString(kindFilter)).append("\n");
sb.append(" manifestAccountsWithdrawnFromFilter: ").append(toIndentedString(manifestAccountsWithdrawnFromFilter)).append("\n");
sb.append(" manifestAccountsDepositedIntoFilter: ").append(toIndentedString(manifestAccountsDepositedIntoFilter)).append("\n");
sb.append(" manifestBadgesPresentedFilter: ").append(toIndentedString(manifestBadgesPresentedFilter)).append("\n");
sb.append(" manifestResourcesFilter: ").append(toIndentedString(manifestResourcesFilter)).append("\n");
sb.append(" affectedGlobalEntitiesFilter: ").append(toIndentedString(affectedGlobalEntitiesFilter)).append("\n");
sb.append(" affectedGlobalEntitiesFilterType: ").append(toIndentedString(affectedGlobalEntitiesFilterType)).append("\n");
sb.append(" eventsFilter: ").append(toIndentedString(eventsFilter)).append("\n");
sb.append(" accountsWithManifestOwnerMethodCalls: ").append(toIndentedString(accountsWithManifestOwnerMethodCalls)).append("\n");
sb.append(" accountsWithoutManifestOwnerMethodCalls: ").append(toIndentedString(accountsWithoutManifestOwnerMethodCalls)).append("\n");
sb.append(" manifestClassFilter: ").append(toIndentedString(manifestClassFilter)).append("\n");
sb.append(" eventGlobalEmittersFilter: ").append(toIndentedString(eventGlobalEmittersFilter)).append("\n");
sb.append(" order: ").append(toIndentedString(order)).append("\n");
sb.append(" optIns: ").append(toIndentedString(optIns)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `at_ledger_state` to the URL query string
if (getAtLedgerState() != null) {
joiner.add(getAtLedgerState().toUrlQueryString(prefix + "at_ledger_state" + suffix));
}
// add `from_ledger_state` to the URL query string
if (getFromLedgerState() != null) {
joiner.add(getFromLedgerState().toUrlQueryString(prefix + "from_ledger_state" + suffix));
}
// add `cursor` to the URL query string
if (getCursor() != null) {
try {
joiner.add(String.format("%scursor%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCursor()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `limit_per_page` to the URL query string
if (getLimitPerPage() != null) {
try {
joiner.add(String.format("%slimit_per_page%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getLimitPerPage()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `kind_filter` to the URL query string
if (getKindFilter() != null) {
try {
joiner.add(String.format("%skind_filter%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getKindFilter()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `manifest_accounts_withdrawn_from_filter` to the URL query string
if (getManifestAccountsWithdrawnFromFilter() != null) {
for (int i = 0; i < getManifestAccountsWithdrawnFromFilter().size(); i++) {
try {
joiner.add(String.format("%smanifest_accounts_withdrawn_from_filter%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getManifestAccountsWithdrawnFromFilter().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `manifest_accounts_deposited_into_filter` to the URL query string
if (getManifestAccountsDepositedIntoFilter() != null) {
for (int i = 0; i < getManifestAccountsDepositedIntoFilter().size(); i++) {
try {
joiner.add(String.format("%smanifest_accounts_deposited_into_filter%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getManifestAccountsDepositedIntoFilter().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `manifest_badges_presented_filter` to the URL query string
if (getManifestBadgesPresentedFilter() != null) {
for (int i = 0; i < getManifestBadgesPresentedFilter().size(); i++) {
try {
joiner.add(String.format("%smanifest_badges_presented_filter%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getManifestBadgesPresentedFilter().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `manifest_resources_filter` to the URL query string
if (getManifestResourcesFilter() != null) {
for (int i = 0; i < getManifestResourcesFilter().size(); i++) {
try {
joiner.add(String.format("%smanifest_resources_filter%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getManifestResourcesFilter().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `affected_global_entities_filter` to the URL query string
if (getAffectedGlobalEntitiesFilter() != null) {
for (int i = 0; i < getAffectedGlobalEntitiesFilter().size(); i++) {
try {
joiner.add(String.format("%saffected_global_entities_filter%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getAffectedGlobalEntitiesFilter().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `affected_global_entities_filter_type` to the URL query string
if (getAffectedGlobalEntitiesFilterType() != null) {
try {
joiner.add(String.format("%saffected_global_entities_filter_type%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAffectedGlobalEntitiesFilterType()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `events_filter` to the URL query string
if (getEventsFilter() != null) {
for (int i = 0; i < getEventsFilter().size(); i++) {
if (getEventsFilter().get(i) != null) {
joiner.add(getEventsFilter().get(i).toUrlQueryString(String.format("%sevents_filter%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `accounts_with_manifest_owner_method_calls` to the URL query string
if (getAccountsWithManifestOwnerMethodCalls() != null) {
for (int i = 0; i < getAccountsWithManifestOwnerMethodCalls().size(); i++) {
try {
joiner.add(String.format("%saccounts_with_manifest_owner_method_calls%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getAccountsWithManifestOwnerMethodCalls().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `accounts_without_manifest_owner_method_calls` to the URL query string
if (getAccountsWithoutManifestOwnerMethodCalls() != null) {
for (int i = 0; i < getAccountsWithoutManifestOwnerMethodCalls().size(); i++) {
try {
joiner.add(String.format("%saccounts_without_manifest_owner_method_calls%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getAccountsWithoutManifestOwnerMethodCalls().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `manifest_class_filter` to the URL query string
if (getManifestClassFilter() != null) {
joiner.add(getManifestClassFilter().toUrlQueryString(prefix + "manifest_class_filter" + suffix));
}
// add `event_global_emitters_filter` to the URL query string
if (getEventGlobalEmittersFilter() != null) {
for (int i = 0; i < getEventGlobalEmittersFilter().size(); i++) {
try {
joiner.add(String.format("%sevent_global_emitters_filter%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getEventGlobalEmittersFilter().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `order` to the URL query string
if (getOrder() != null) {
try {
joiner.add(String.format("%sorder%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getOrder()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `opt_ins` to the URL query string
if (getOptIns() != null) {
joiner.add(getOptIns().toUrlQueryString(prefix + "opt_ins" + suffix));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy