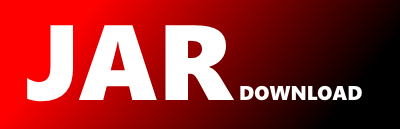
com.simbot.component.mirai.collections.SingletonMap.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of component-mirai Show documentation
Show all versions of component-mirai Show documentation
a simple-robot component for mirai
/*
*
* Copyright (c) 2020. ForteScarlet All rights reserved.
* Project component-mirai
* File collections.kt
*
* You can contact the author through the following channels:
* github https://github.com/ForteScarlet
* gitee https://gitee.com/ForteScarlet
* email [email protected]
* QQ 1149159218
*
*
*/
package com.simbot.component.mirai.collections
import java.io.Serializable
/**
* 一个单值Map, 只可以改变value值.
* key不可为null
* value不可为null
*/
@Suppress("MemberVisibilityCanBePrivate")
class SingletonMap(val key: K, var value: V) : Map, Serializable, Cloneable {
constructor(entry: Map.Entry): this(entry.key, entry.value)
private val keySet: Set = setOf(key)
private val singletonEntry = SingletonMapEntry()
/**
* 判断是否与key相同
*/
private fun isKey(other: Any?): Boolean {
return key == other
}
/**
* 判断是否与value相同
*/
private fun isValue(other: Any?): Boolean {
return value == other
}
/**
* 大小固定为1
*/
override val size: Int = 1
/**
* 得到一个entries
*/
override val entries: MutableSet>
get() = mutableSetOf(singletonEntry)
/**
* keys
*/
override val keys: MutableSet
get() = keySet.toMutableSet()
/**
* values
*/
override val values: MutableCollection
get() = mutableListOf(value)
/**
* 是否存在key
*/
override fun containsKey(key: K): Boolean = isKey(key)
override fun containsValue(value: V): Boolean = isValue(value)
override fun get(key: K): V? = if(isKey(key)) value else null
override fun isEmpty(): Boolean = false
/**
* entry
*/
inner class SingletonMapEntry: MutableMap.MutableEntry {
/**
* set value for [super map value][SingletonMap.value]
*/
override fun setValue(newValue: V): V {
val oldValue = value
[email protected] = newValue
return oldValue
}
/**
* Returns the key of this key/value pair.
* just return [super map key][SingletonMap.key]
*/
override val key: K = [email protected]
/**
* Returns the value of this key/value pair.
* just return [super map value][SingletonMap.value]
*/
override val value: V
get() = [email protected]
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy