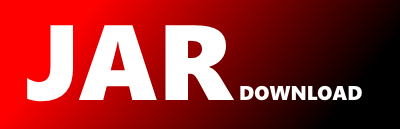
ratpack.kotlin.handling.KChainAction.kt Maven / Gradle / Ivy
package ratpack.kotlin.handling
import ratpack.file.FileHandlerSpec
import ratpack.func.Action
import ratpack.func.Predicate
import ratpack.handling.Chain
import ratpack.handling.Context
import ratpack.handling.Handler
import ratpack.registry.Registry
import ratpack.registry.RegistrySpec
import ratpack.server.ServerConfig
/**
* Allow for the kotlin DSL to be used from classes extending KChainAction.
*/
abstract class KChainAction : Action {
var delegate: ThreadLocal = ThreadLocal()
abstract fun execute()
override fun execute(chain: Chain) {
delegate.set(KChain(chain))
execute()
delegate.remove()
}
//convenience to get delegate value
fun dg(): KChain = delegate.get()
//mirror of available functions in GroovyChain
//misc
fun getRegistry(): Registry = dg().registry
fun getServerConfig(): ServerConfig = dg().serverConfig
//prefix
inline fun prefix(path: String = "", crossinline cb: KChain.(KChain) -> Unit): KChain = dg().prefix(path, cb)
fun prefix(path: String = "", action: Action): KChain = dg().prefix(path, action)
fun prefix(path: String = "", action: Class>): KChain = dg().prefix(path, action)
//all
inline fun all(crossinline cb: KContext.(KContext) -> Unit): KChain = dg().all(cb)
fun all(handler: Handler): KChain = dg().all(handler)
fun all(handler: Class): KChain = dg().all(handler)
//path
inline fun path(path: String = "", crossinline cb: KContext.(KContext) -> Unit): KChain = dg().path(path, cb)
fun path(path: String = "", handler: Handler): KChain = dg().path(path, handler)
fun path(path: String = "", handler: Class): KChain = dg().path(path, handler)
//chain
fun chain(cb: KChain.(KChain) -> Unit): Handler = dg().chain(cb)
fun chain(action: Class>): Handler = dg().chain(action)
//get
inline fun get(path: String = "", crossinline cb: KContext.(KContext) -> Unit): KChain = dg().get(path, cb)
fun get(path: String = "", handler: Handler): KChain = dg().get(path, handler)
fun get(path: String = "", handler: Class): KChain = dg().get(path, handler)
//put
inline fun put(path: String = "", crossinline cb: KContext.(KContext) -> Unit): KChain = dg().put(path, cb)
fun put(path: String = "", handler: Handler): KChain = dg().put(path, handler)
fun put(path: String = "", handler: Class): KChain = dg().put(path, handler)
//post
inline fun post(path: String = "", crossinline cb: KContext.(KContext) -> Unit): KChain = dg().post(path, cb)
fun post(path: String = "", handler: Handler): KChain = dg().post(path, handler)
fun post(path: String = "", handler: Class): KChain = dg().post(path, handler)
//delete
inline fun delete(path: String = "", crossinline cb: KContext.(KContext) -> Unit): KChain = dg().delete(path, cb)
fun delete(path: String = "", handler: Handler): KChain = dg().delete(path, handler)
fun delete(path: String = "", handler: Class): KChain = dg().delete(path, handler)
//patch
inline fun patch(path: String = "", crossinline cb: KContext.(KContext) -> Unit): KChain = dg().patch(path, cb)
fun patch(path: String = "", handler: Handler): KChain = dg().patch(path, handler)
fun patch(path: String = "", handler: Class): KChain = dg().patch(path, handler)
//options
inline fun options(path: String = "", crossinline cb: KContext.(KContext) -> Unit): KChain = dg().options(path, cb)
fun options(path: String = "", handler: Handler): KChain = dg().options(path, handler)
fun options(path: String = "", handler: Class): KChain = dg().options(path, handler)
//fileSystem
inline fun fileSystem(path: String = "", crossinline cb: KChain.(KChain) -> Unit): KChain = dg().fileSystem(path, cb)
fun fileSystem(path: String = "", action: Action): KChain = dg().fileSystem(path, action)
fun fileSystem(path: String = "", action: Class>): KChain = dg().fileSystem(path, action)
//files
inline fun files(crossinline cb: FileHandlerSpec.(FileHandlerSpec) -> Unit): KChain = dg().files(cb)
fun files(): KChain = dg().files()
fun files(action: Action): KChain = dg().files(action)
//host
inline fun host(hostName: String, crossinline cb: KChain.(KChain) -> Unit): KChain = dg().host(hostName, cb)
fun host(hostName: String, action: Action): KChain = dg().host(hostName, action)
fun host(hostName: String, action: Class>): KChain = dg().host(hostName, action)
//insert
inline fun insert(crossinline cb: KChain.(KChain) -> Unit): KChain = dg().insert(cb)
fun insert(action: Action): KChain = dg().insert(action)
fun insert(action: Class>): KChain = dg().insert(getRegistry().get(action))
//redirect
fun redirect(code: Int, location: String): KChain = dg().redirect(code, location)
//register
fun register(registry: Registry): KChain = dg().register(registry)
fun register(action: Action): KChain = dg().register(action)
fun register(registry: Registry, action: Action): KChain = dg().register(registry, action)
fun register(registry: Registry, action: Class>): KChain = dg().register(registry, action)
fun register(registryAction: Action, chainAction: Action): KChain = dg().register(registryAction, chainAction)
fun register(registryAction: Action, chainAction: Class>): KChain = dg().register(registryAction, chainAction)
inline fun register(crossinline registryAction: RegistrySpec.(RegistrySpec) -> Unit, crossinline cb: KChain.(KChain) -> Unit): KChain = dg().register(registryAction, cb)
inline fun register(registry: Registry, crossinline cb: KChain.(KChain) -> Unit): KChain = dg().register(registry, cb)
inline fun register(crossinline registryAction: RegistrySpec.(RegistrySpec) -> Unit): KChain = dg().register(registryAction)
//when
inline fun `when`(test: Predicate, crossinline cb: KChain.(KChain) -> Unit): KChain = dg().`when`(test, cb)
fun `when`(test: Predicate, action: Action): KChain = dg().`when`(test, action)
fun `when`(test: Predicate, action: Class>): KChain = dg().`when`(test, action)
inline fun `when`(test: Boolean, crossinline cb: KChain.(KChain) -> Unit): KChain = dg().`when`(test, cb)
fun `when`(test: Boolean, action: Action): KChain = dg().`when`(test, action)
fun `when`(test: Boolean, action: Class>): KChain = dg().`when`(test, action)
//onlyIf
fun onlyIf(test: Predicate, cb: KContext.(KContext) -> Unit): KChain = dg().onlyIf(test, cb)
fun onlyIf(test: Predicate, handler: Handler): KChain = dg().onlyIf(test, handler)
fun onlyIf(test: Predicate, handler: Class): KChain = dg().onlyIf(test, handler)
//notFound
fun notFound(): KChain = dg().notFound()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy