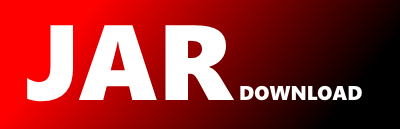
eleme.openapi.h5.sdk.pay.service.base.BaseCallbackService Maven / Gradle / Ivy
package eleme.openapi.h5.sdk.pay.service.base;
import java.nio.charset.StandardCharsets;
import javax.net.ssl.SSLContext;
import eleme.openapi.h5.sdk.pay.model.request.PayCallbackRequest;
import eleme.openapi.h5.sdk.pay.model.request.RefundCallbackRequest;
import eleme.openapi.h5.sdk.pay.service.CallbackService;
import eleme.openapi.h5.sdk.pay.utils.PaySignUtil;
import org.apache.commons.lang3.StringUtils;
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.conn.ssl.NoopHostnameVerifier;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.ssl.SSLContextBuilder;
import org.apache.http.util.EntityUtils;
import org.apache.logging.log4j.util.Strings;
/**
* 饿了么回调base实现,机构侧继承该类
*/
public abstract class BaseCallbackService implements CallbackService {
@Override
public boolean payCallback(PayCallbackRequest request, String notifyUrl) throws Exception {
return callback(request, notifyUrl);
}
@Override
public boolean refundCallback(RefundCallbackRequest request, String notifyUrl) throws Exception {
return callback(request, notifyUrl);
}
/**
* 结果回调,仅支付/退款成功需要回调
*
* @param request
* @param notifyUrl 通知地址
* @return 回调是否成功
*/
private boolean callback(Object request, String notifyUrl) throws Exception {
// 1.加签
String reqContent = PaySignUtil.sign(request, getPrivateKey());
// 2.http请求
String respContent = httpExecute(reqContent, notifyUrl);
// 3.结果处理
return "SUCCESS".equals(respContent);
}
/**
* http基本调用
* @param reqContent 请求内容
* @param url 请求url
* @return http响应内容
* @throws Exception 异常信息
*/
protected String httpExecute(String reqContent, String url) throws Exception {
CloseableHttpClient httpClient = null;
CloseableHttpResponse response = null;
try {
// 创建SSL上下文
SSLContext sslContext = new SSLContextBuilder().loadTrustMaterial(null, (chain, authType) -> true).build();
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(sslContext,
NoopHostnameVerifier.INSTANCE);
// 创建HttpClient实例
httpClient = HttpClients.custom().setSSLSocketFactory(sslsf).build();
// 创建HttpPost实例
HttpPost httpPost = new HttpPost(url);
// 设置请求头
httpPost.setHeader("Content-Type", "application/json");
// 设置请求体
StringEntity entity = new StringEntity(reqContent, "UTF-8");
httpPost.setEntity(entity);
// 发送请求并接收响应
response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
// 检查响应状态码
if (response.getStatusLine().getStatusCode() >= 200 && response.getStatusLine().getStatusCode() < 300) {
// 输出响应状态码和响应体
return EntityUtils.toString(responseEntity, StandardCharsets.UTF_8);
}
return Strings.EMPTY;
} finally {
if (response != null) {
response.close();
}
if (httpClient != null) {
httpClient.close();
}
}
}
/**
* 获取机构私钥
*
* @return 机构私钥
*/
public abstract String getPrivateKey();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy