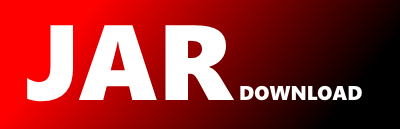
eleme.openapi.h5.sdk.pay.utils.PaySignUtil Maven / Gradle / Ivy
package eleme.openapi.h5.sdk.pay.utils;
import java.util.HashMap;
import java.util.Map;
import java.util.TreeMap;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.alibaba.fastjson.TypeReference;
import com.google.common.base.Joiner;
import com.google.common.collect.Maps;
import org.apache.commons.lang3.RandomStringUtils;
import org.apache.commons.lang3.StringUtils;
/**
* 支付签约工具类
*/
public class PaySignUtil {
/**
* 支付验签
*
* @param content 请求内容
* @param elemePublicKey 饿了么公钥
* @return 验签结果
*/
public static boolean verify(String content, String elemePublicKey) {
try {
Map contentMap = JSONObject.parseObject(content,
new TypeReference>() {});
String sign = contentMap.get("sign");
// sign和内容为空的字段不参与签名
contentMap = Maps.filterEntries(contentMap,
p -> StringUtils.isNotBlank(p.getKey()) && StringUtils.isNotBlank(p.getValue()) && !StringUtils.equals(
"sign", p.getKey()));
String signContent = Joiner.on("&").withKeyValueSeparator("=").join(contentMap);
return RSA2Util.rsa256Verify(signContent, sign, elemePublicKey, "utf-8");
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
/**
* 对业务结果加签并生成最终响应结果
*
* @param obj 加签对象
* @param privateKey 机构私钥
* @return 加签后的内容
*/
public static String sign(Object obj, String privateKey) {
try {
Map contentMap = JSONObject.parseObject(JSON.toJSONString(obj),
new TypeReference>() {});
// 生成随机串
String nonceStr = RandomStringUtils.randomAlphabetic(32);
contentMap.put("nonceStr", nonceStr);
// 生成签名
contentMap = Maps.filterEntries(contentMap,
p -> StringUtils.isNotBlank(p.getKey()) && StringUtils.isNotBlank(p.getValue()));
String signContent = Joiner.on("&").withKeyValueSeparator("=").join(contentMap);
String sign = RSA2Util.rsa256Sign(signContent, privateKey, "utf-8");
contentMap.put("sign", sign);
return JSON.toJSONString(contentMap);
} catch (Exception e) {
e.printStackTrace();
JSONObject jsonObject = new JSONObject();
jsonObject.put("returnCode", "FAIL");
jsonObject.put("returnMsg", "加签失败");
return JSON.toJSONString(jsonObject);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy