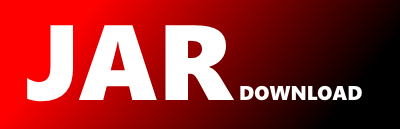
eleme.openapi.h5.sdk.pay.utils.RSA2Util Maven / Gradle / Ivy
package eleme.openapi.h5.sdk.pay.utils;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.io.StringWriter;
import java.io.Writer;
import java.security.PublicKey;
import java.security.spec.X509EncodedKeySpec;
import java.util.*;
import java.security.KeyFactory;
import java.security.PrivateKey;
import java.security.Signature;
import java.security.spec.PKCS8EncodedKeySpec;
import org.springframework.util.StringUtils;
public class RSA2Util {
/**
* rsa2加签
*
* @param content 加签内容
* @param privateKey 私钥
* @param charset 编码
* @return 签名
* @throws Exception 异常信息
*/
public static String rsa256Sign(String content, String privateKey, String charset) throws Exception {
try {
PrivateKey priKey = getPrivateKeyFromPKCS8("RSA", new ByteArrayInputStream(privateKey.getBytes()));
Signature signature = Signature.getInstance("SHA256WithRSA");
signature.initSign(priKey);
if (StringUtils.isEmpty(charset)) {
signature.update(content.getBytes());
} else {
signature.update(content.getBytes(charset));
}
byte[] signed = signature.sign();
return Base64.getEncoder().encodeToString(signed);
} catch (Exception var6) {
throw new Exception("RSAcontent = " + content + "; charset = " + charset, var6);
}
}
/**
* rsa2验签
*
* @param content 验签内容
* @param sign 签名
* @param publicKey 公钥
* @param charset 编码
* @return 验签结果
* @throws Exception 异常信息
*/
public static boolean rsa256Verify(String content, String sign, String publicKey, String charset) throws Exception {
PublicKey pubKey = getPublicKeyFromX509("RSA", new ByteArrayInputStream(publicKey.getBytes()));
Signature signature = Signature.getInstance("SHA256WithRSA");
signature.initVerify(pubKey);
signature.update(content.getBytes(charset));
return signature.verify(Base64.getDecoder().decode(sign));
}
private static PublicKey getPublicKeyFromX509(String algorithm, InputStream ins) throws Exception {
KeyFactory keyFactory = KeyFactory.getInstance(algorithm);
StringWriter writer = new StringWriter();
io(new InputStreamReader(ins), writer, -1);
byte[] encodedKey = writer.toString().getBytes();
encodedKey = Base64.getDecoder().decode(new String(encodedKey));
return keyFactory.generatePublic(new X509EncodedKeySpec(encodedKey));
}
private static PrivateKey getPrivateKeyFromPKCS8(String algorithm, InputStream ins) throws Exception {
if (ins != null && !StringUtils.isEmpty(algorithm)) {
KeyFactory keyFactory = KeyFactory.getInstance(algorithm);
byte[] encodedKey = readText(ins).getBytes();
encodedKey = Base64.getDecoder().decode(new String(encodedKey));
return keyFactory.generatePrivate(new PKCS8EncodedKeySpec(encodedKey));
} else {
return null;
}
}
private static String readText(InputStream in) throws IOException {
return readText(in, (String)null, -1);
}
private static String readText(InputStream in, String encoding, int bufferSize) throws IOException {
Reader reader = encoding == null ? new InputStreamReader(in) : new InputStreamReader(in, encoding);
return readText(reader, bufferSize);
}
private static String readText(Reader reader, int bufferSize) throws IOException {
StringWriter writer = new StringWriter();
io((Reader)reader, (Writer)writer, bufferSize);
return writer.toString();
}
private static void io(Reader in, Writer out, int bufferSize) throws IOException {
if (bufferSize == -1) {
bufferSize = 4096;
}
char[] buffer = new char[bufferSize];
int amount;
while ((amount = in.read(buffer)) >= 0) {
out.write(buffer, 0, amount);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy