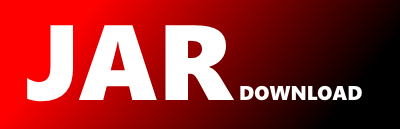
eleme.openapi.sdk.api.entity.order.OGoodsItem Maven / Gradle / Ivy
package eleme.openapi.sdk.api.entity.order;
import eleme.openapi.sdk.api.enumeration.order.*;
import eleme.openapi.sdk.api.entity.order.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class OGoodsItem{
/**
* 食物id
*/
private long id;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
/**
* 规格Id(根据篮子的类型取不同的值)
*/
private Long skuId;
public Long getSkuId() {
return skuId;
}
public void setSkuId(Long skuId) {
this.skuId = skuId;
}
/**
* 商品名称
*/
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
* 原始商品名称
*/
private String originalName;
public String getOriginalName() {
return originalName;
}
public void setOriginalName(String originalName) {
this.originalName = originalName;
}
/**
* 备餐描述
*/
private String mealPreparation;
public String getMealPreparation() {
return mealPreparation;
}
public void setMealPreparation(String mealPreparation) {
this.mealPreparation = mealPreparation;
}
/**
* 订单中商品项的标识(注意,此处不是商品分类Id)
*/
private long categoryId;
public long getCategoryId() {
return categoryId;
}
public void setCategoryId(long categoryId) {
this.categoryId = categoryId;
}
/**
* 商品单价
*/
private double price;
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
/**
* 商品数量
*/
private int quantity;
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
/**
* 总价
*/
private double total;
public double getTotal() {
return total;
}
public void setTotal(double total) {
this.total = total;
}
/**
* 多规格
*/
private List newSpecs;
public List getNewSpecs() {
return newSpecs;
}
public void setNewSpecs(List newSpecs) {
this.newSpecs = newSpecs;
}
/**
* 多属性
*/
private List attributes;
public List getAttributes() {
return attributes;
}
public void setAttributes(List attributes) {
this.attributes = attributes;
}
/**
* 商品扩展码
*/
private String extendCode;
public String getExtendCode() {
return extendCode;
}
public void setExtendCode(String extendCode) {
this.extendCode = extendCode;
}
/**
* 商品条形码
*/
private String barCode;
public String getBarCode() {
return barCode;
}
public void setBarCode(String barCode) {
this.barCode = barCode;
}
/**
* 商品重量(单位克)
*/
private Double weight;
public Double getWeight() {
return weight;
}
public void setWeight(Double weight) {
this.weight = weight;
}
/**
* 用户侧价格
*/
private Double userPrice;
public Double getUserPrice() {
return userPrice;
}
public void setUserPrice(Double userPrice) {
this.userPrice = userPrice;
}
/**
* 商户侧价格
*/
private Double shopPrice;
public Double getShopPrice() {
return shopPrice;
}
public void setShopPrice(Double shopPrice) {
this.shopPrice = shopPrice;
}
/**
* 商品id
*/
private long vfoodId;
public long getVfoodId() {
return vfoodId;
}
public void setVfoodId(long vfoodId) {
this.vfoodId = vfoodId;
}
/**
* food的uniqueId,退款需传此字段
*/
private String uniqueId;
public String getUniqueId() {
return uniqueId;
}
public void setUniqueId(String uniqueId) {
this.uniqueId = uniqueId;
}
/**
* 配料
*/
private List ingredients;
public List getIngredients() {
return ingredients;
}
public void setIngredients(List ingredients) {
this.ingredients = ingredients;
}
/**
* 套餐子商品
*/
private List> foodGroup;
public List> getFoodGroup() {
return foodGroup;
}
public void setFoodGroup(List> foodGroup) {
this.foodGroup = foodGroup;
}
/**
* 商品编码
*/
private String eleItemCode;
public String getEleItemCode() {
return eleItemCode;
}
public void setEleItemCode(String eleItemCode) {
this.eleItemCode = eleItemCode;
}
/**
* 商品UPC
*/
private String upcCode;
public String getUpcCode() {
return upcCode;
}
public void setUpcCode(String upcCode) {
this.upcCode = upcCode;
}
/**
* 商品类型
*/
private int foodType;
public int getFoodType() {
return foodType;
}
public void setFoodType(int foodType) {
this.foodType = foodType;
}
/**
* 商品参与的活动
*/
private List activities;
public List getActivities() {
return activities;
}
public void setActivities(List activities) {
this.activities = activities;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy