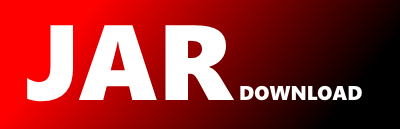
eleme.openapi.sdk.api.service.DecorationService Maven / Gradle / Ivy
package eleme.openapi.sdk.api.service;
import eleme.openapi.sdk.api.annotation.Service;
import eleme.openapi.sdk.api.base.BaseNopService;
import eleme.openapi.sdk.api.exception.ServiceException;
import eleme.openapi.sdk.oauth.response.Token;
import eleme.openapi.sdk.config.Config;
import eleme.openapi.sdk.api.entity.decoration.*;
import eleme.openapi.sdk.api.enumeration.decoration.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.*;
/**
* 门店装修服务
*/
@Service("eleme.decoration")
public class DecorationService extends BaseNopService {
public DecorationService(Config config,Token token) {
super(config, token, DecorationService.class);
}
/**
* 创建招贴
*
* @param sign 招贴信息和其关联门店ID集合
* @return 招贴ID
* @throws ServiceException 服务异常
*/
public long createSign(OSaveShopSignRequest sign) throws ServiceException {
Map params = new HashMap();
params.put("sign", sign);
return call("eleme.decoration.sign.createSign", params);
}
/**
* 修改招贴
*
* @param signId 招贴ID
* @param sign 招贴信息和其关联门店ID
* @return 招贴ID
* @throws ServiceException 服务异常
*/
public long updateSign(long signId, OSaveShopSignRequest sign) throws ServiceException {
Map params = new HashMap();
params.put("signId", signId);
params.put("sign", sign);
return call("eleme.decoration.sign.updateSign", params);
}
/**
* 作废招贴
*
* @param signId 招贴ID
* @throws ServiceException 服务异常
*/
public void invalidSign(long signId) throws ServiceException {
Map params = new HashMap();
params.put("signId", signId);
call("eleme.decoration.sign.invalidSign", params);
}
/**
* 获取历史上传过的招贴图片
*
* @param sign 查询条件
* @return 历史招贴图片集合
* @throws ServiceException 服务异常
*/
public List getSignHistoryImage(OQuerySignRequest sign) throws ServiceException {
Map params = new HashMap();
params.put("sign", sign);
return call("eleme.decoration.sign.getSignHistoryImage", params);
}
/**
* 查询有效招贴集合
*
* @return 当前店铺招贴集合
* @throws ServiceException 服务异常
*/
public List querySign() throws ServiceException {
Map params = new HashMap();
return call("eleme.decoration.sign.querySign", params);
}
/**
* 根据招贴ID查询店铺招贴详情
*
* @param signId 招贴ID
* @return 招贴信息
* @throws ServiceException 服务异常
*/
public OShopSignDetailResponse getSignById(long signId) throws ServiceException {
Map params = new HashMap();
params.put("signId", signId);
return call("eleme.decoration.sign.getSignById", params);
}
/**
* 新增品牌故事
*
* @param story 品牌故事信息和其关联连锁店子店ID
* @return 品牌故事ID
* @throws ServiceException 服务异常
*/
public long createBrandStory(OSaveBrandStoryRequest story) throws ServiceException {
Map params = new HashMap();
params.put("story", story);
return call("eleme.decoration.story.createBrandStory", params);
}
/**
* 更新品牌故事
*
* @param brandStoryId 品牌故事ID
* @param story 品牌故事信息和其关联连锁店子店ID
* @return 品牌故事ID
* @throws ServiceException 服务异常
*/
public long updateBrandStory(Long brandStoryId, OSaveBrandStoryRequest story) throws ServiceException {
Map params = new HashMap();
params.put("brandStoryId", brandStoryId);
params.put("story", story);
return call("eleme.decoration.story.updateBrandStory", params);
}
/**
* 删除品牌故事
*
* @param brandStoryId 品牌故事ID
* @return 品牌故事ID
* @throws ServiceException 服务异常
*/
public long deleteBrandStory(Long brandStoryId) throws ServiceException {
Map params = new HashMap();
params.put("brandStoryId", brandStoryId);
return call("eleme.decoration.story.deleteBrandStory", params);
}
/**
* 查询品牌故事列表
*
* @return 品牌故事集合
* @throws ServiceException 服务异常
*/
public List queryBrandStoryList() throws ServiceException {
Map params = new HashMap();
return call("eleme.decoration.story.queryBrandStoryList", params);
}
/**
* 查询当前设置的品牌故事信息
*
* @param brandStoryId 品牌故事ID
* @return 品牌故事信息
* @throws ServiceException 服务异常
*/
public OBrandStoryResponse getBrandStoryById(long brandStoryId) throws ServiceException {
Map params = new HashMap();
params.put("brandStoryId", brandStoryId);
return call("eleme.decoration.story.getBrandStoryById", params);
}
/**
* 保存精准分类
*
* @param category 精准分类信息
* @throws ServiceException 服务异常
*/
public void saveCategory(OSaveAccurateCategoryRequest category) throws ServiceException {
Map params = new HashMap();
params.put("category", category);
call("eleme.decoration.accurateCategory.saveCategory", params);
}
/**
* 根据门店ID获取精准分类
*
* @param category 查询参数
* @return 精准分类信息
* @throws ServiceException 服务异常
*/
public OShopAccurateCategory getAccurateCategory(OGetAccurateCategoryRequest category) throws ServiceException {
Map params = new HashMap();
params.put("category", category);
return call("eleme.decoration.accurateCategory.getAccurateCategory", params);
}
/**
* 查询精准分类
*
* @param category 查询参数
* @return 精准分类信息集合
* @throws ServiceException 服务异常
*/
public List queryAccurateCategoryList(OQueryAccurateCategoryRequest category) throws ServiceException {
Map params = new HashMap();
params.put("category", category);
return call("eleme.decoration.accurateCategory.queryAccurateCategoryList", params);
}
/**
* 创建海报
*
* @param poster 海报信息和其关联门店ID和门店商品
* @return 新建的海报ID
* @throws ServiceException 服务异常
*/
public Long createPoster(OSavePosterRequest poster) throws ServiceException {
Map params = new HashMap();
params.put("poster", poster);
return call("eleme.decoration.poster.createPoster", params);
}
/**
* 修改海报
*
* @param posterId 海报ID
* @param poster 海报信息和其关联门店ID和门店商品
* @throws ServiceException 服务异常
*/
public void updatePoster(Long posterId, OSavePosterRequest poster) throws ServiceException {
Map params = new HashMap();
params.put("posterId", posterId);
params.put("poster", poster);
call("eleme.decoration.poster.updatePoster", params);
}
/**
* 作废海报
*
* @param poster 作废海报信息
* @throws ServiceException 服务异常
*/
public void invalidPoster(OInvalidPosterRequest poster) throws ServiceException {
Map params = new HashMap();
params.put("poster", poster);
call("eleme.decoration.poster.invalidPoster", params);
}
/**
* 根据海报ID获取海报详情
*
* @param posterId 海报ID
* @return 海报和海报商品详情
* @throws ServiceException 服务异常
*/
public OShopPosterDetailResponse getPosterDetailById(Long posterId) throws ServiceException {
Map params = new HashMap();
params.put("posterId", posterId);
return call("eleme.decoration.poster.getPosterDetailById", params);
}
/**
* 根据海报ID获取海报详情 V2
*
* @param posterId 海报ID
* @param shopId 当前店铺ID,默认情况下和当前帐号一致,如果是连锁分店想切换到子店进行操作,那么使用子店的店铺id进行设置
* @return 海报和海报商品详情
* @throws ServiceException 服务异常
*/
public OShopPosterDetailResponse getPosterDetailByIdV2(Long posterId, Long shopId) throws ServiceException {
Map params = new HashMap();
params.put("posterId", posterId);
params.put("shopId", shopId);
return call("eleme.decoration.poster.getPosterDetailByIdV2", params);
}
/**
* 查询有效的海报信息集合
*
* @return 海报信息集合
* @throws ServiceException 服务异常
*/
public List queryEffectivePosters() throws ServiceException {
Map params = new HashMap();
return call("eleme.decoration.poster.queryEffectivePosters", params);
}
/**
* 查询有效的海报信息集合 V2
*
* @param shopId 当前店铺ID,默认情况下和当前帐号一致,如果是连锁分店想切换到子店进行操作,那么使用子店的店铺id进行设置
* @return 海报信息集合
* @throws ServiceException 服务异常
*/
public List queryEffectivePostersV2(Long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.decoration.poster.queryEffectivePostersV2", params);
}
/**
* 获取历史上传过的海报图片
*
* @return 历史图片列表
* @throws ServiceException 服务异常
*/
public List getPosterHistoryImage() throws ServiceException {
Map params = new HashMap();
return call("eleme.decoration.poster.getPosterHistoryImage", params);
}
/**
* 保存爆款橱窗
*
* @param burstWindow 爆款橱窗信息
* @throws ServiceException 服务异常
*/
public void saveBurstWindow(OSaveShopBurstWindow burstWindow) throws ServiceException {
Map params = new HashMap();
params.put("burstWindow", burstWindow);
call("eleme.decoration.burstWindow.saveBurstWindow", params);
}
/**
* 根据门店ID关闭店铺爆款橱窗
*
* @param shopId 门店ID
* @throws ServiceException 服务异常
*/
public void closeBurstWindowByShopId(Long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
call("eleme.decoration.burstWindow.closeBurstWindowByShopId", params);
}
/**
* 根据店铺ID查询该店铺的爆款橱窗信息
*
* @param shopId 店铺ID
* @return 爆款橱窗信息
* @throws ServiceException 服务异常
*/
public OShopBurstWindow getBurstWindowByShopId(Long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.decoration.burstWindow.getBurstWindowByShopId", params);
}
/**
* 根据门店ID集合查询店铺爆款橱窗信息集合
*
* @param shopIds 查询条件
* @return 爆款橱窗信息集合
* @throws ServiceException 服务异常
*/
public List queryBurstWindowList(List shopIds) throws ServiceException {
Map params = new HashMap();
params.put("shopIds", shopIds);
return call("eleme.decoration.burstWindow.queryBurstWindowList", params);
}
/**
* 上传图片
*
* @param image 文件内容base64编码值
* @return 图片信息
* @throws ServiceException 服务异常
*/
public OImage upload(String image) throws ServiceException {
Map params = new HashMap();
params.put("image", image);
return call("eleme.decoration.image.upload", params);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy