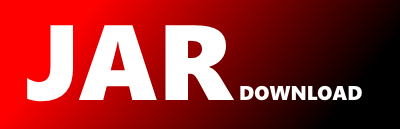
eleme.openapi.sdk.media.common.AuthUtil Maven / Gradle / Ivy
package eleme.openapi.sdk.media.common;
import eleme.openapi.sdk.media.utils.IOUtils;
import org.apache.http.Header;
import org.apache.http.client.methods.HttpEntityEnclosingRequestBase;
import org.apache.http.client.methods.HttpRequestBase;
import org.apache.http.client.utils.DateUtils;
import java.io.IOException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.util.Date;
/**
* User: mulou
* Date: 15-1-2
* Time: 15:49
*/
public class AuthUtil {
private static final String BLANK = " ";
private static final String COLON = ":";
private static final String EMPTY = "";
private static final String QUESTION = "?";
private static final String LINEBREAK = "\n";
private static final String URLENCODED_FORM_CONTENT_TYPE = "application/x-www-form-urlencoded";
private static final String TOP_AUTH_TYPE = "ACL_TOP";
/**
* 一个更加方便的计算签名工具.
* 要求调用这个方法之前必须完成setEntity!!!!
*
* @param request request
* @param ak 用户ak
* @param sk 用户sk
* @param compatible 兼容逻辑,用于兼容之前非form-encoded不计算签名的逻辑
* @throws IOException IO异常
*/
public static void authRequest(HttpRequestBase request, String ak, String sk, boolean compatible) throws IOException {
String body = null;
String date = DateUtils.formatDate(new Date());
request.setHeader("Date", date);
if (request instanceof HttpEntityEnclosingRequestBase && ((HttpEntityEnclosingRequestBase) request).getEntity() != null) {
HttpEntityEnclosingRequestBase entityRequest = (HttpEntityEnclosingRequestBase) request;
Header[] contentTypeHeaders = request.getHeaders("Content-Type");
if (contentTypeHeaders == null) {
throw new IllegalArgumentException("Content-Type header required");
}
boolean isUrlFormEncoded = false;
for (Header header : contentTypeHeaders) {
if (header.getValue().contains(URLENCODED_FORM_CONTENT_TYPE)) {
isUrlFormEncoded = true;
break;
}
}
if (entityRequest.getEntity().getContentType() != null
&& entityRequest.getEntity().getContentType().getValue() != null
&& entityRequest.getEntity().getContentType().getValue().contains(URLENCODED_FORM_CONTENT_TYPE)) {
isUrlFormEncoded = true;
}
if (isUrlFormEncoded) {
body = IOUtils.toString(entityRequest.getEntity().getContent());
} else if (!compatible) {
body = EncodeUtil.encodeWithMD5(entityRequest.getEntity().getContent(), 1024);
}
}
if (request instanceof HttpEntityEnclosingRequestBase && ((HttpEntityEnclosingRequestBase) request).getEntity() == null) {
throw new IllegalArgumentException("entity required");
}
request.setHeader("Authorization", authHeader(request.getURI().getPath().toString(), request.getURI().getQuery(), body, date, ak, sk, TOP_AUTH_TYPE));
}
/**
* 生成加签头信息
*
* @param uri url
* @param queryString 查询参数
* @param httpBody 请求体
* @param date 日期
* @param ak 用户ak
* @param sk 用户sk
* @param authType 审核类型
* @return 签名字符
*/
public static String authHeader(String uri, String queryString, String httpBody, String date, String ak, String sk, String authType) {
String result = authHeader(headerBeforeEncode(uri, queryString, httpBody, date, ak, sk), authType);
return result;
}
/**
* 使用hmac-sha1算法对待加签串进行加密
*
* @param stringBeforeSign 签名字符
* @param sk 用户sk
* @return 签名结果
*/
public static String sign(String stringBeforeSign, String sk) {
checkNotNull(sk);
checkNotNull(stringBeforeSign);
try {
String result = EncodeUtil.encodeWithHmacSha1(stringBeforeSign, sk);
return result;
} catch (InvalidKeyException e) {
//can't happen
} catch (NoSuchAlgorithmException e) {
}
return null;
}
/**
* 使用hmac-sha1算法对参数加密
*
* @param uri uri
* @param queryString 查询字符
* @param httpBody 请求体
* @param date 日期
* @param sk 用户sk
* @return 签名
*/
public static String sign(String uri, String queryString, String httpBody, String date, String sk) {
return sign(stringBeforeSign(uri, queryString, httpBody, date), sk);
}
/**
* 获得待加签串
* uri + queryString + "\n" + httpBody + "\n" + date
*
* @param uri uri
* @param queryString 查询参数
* @param httpBody 请求体
* @param date 日期
* @return 签名
*/
public static String stringBeforeSign(String uri, String queryString, String httpBody, String date) {
checkArgument(isNotBlank(uri));
checkNotNull(date);
StringBuffer sb = new StringBuffer(EMPTY);
sb.append(uri);
if (isNotBlank(queryString)) sb.append(QUESTION).append(queryString);
sb.append(LINEBREAK);
if (isNotBlank(httpBody)) sb.append(httpBody);
sb.append(LINEBREAK);
sb.append(date);
return sb.toString();
}
private static String authHeader(String headerBeforeEncode, String type) {
checkNotNull(type);
checkNotNull(headerBeforeEncode);
String headerAfterEncode = EncodeUtil.encodeWithURLSafeBase64(headerBeforeEncode);
return type + BLANK + headerAfterEncode;
}
private static String headerBeforeEncode(String uri, String queryString, String httpBody, String date, String ak, String sk) {
checkNotNull(ak);
checkNotNull(sk);
return ak + COLON + sign(uri, queryString, httpBody, date, sk);
}
private static void checkArgument(boolean expression) {
if (!expression) {
throw new IllegalArgumentException();
}
}
private static T checkNotNull(T reference) {
if (reference == null) {
throw new NullPointerException();
} else {
return reference;
}
}
private static boolean isNotBlank(CharSequence cs) {
return !isBlank(cs);
}
private static boolean isBlank(CharSequence cs) {
int strLen;
if (cs == null || (strLen = cs.length()) == 0) {
return true;
}
for (int i = 0; i < strLen; i++) {
if (Character.isWhitespace(cs.charAt(i)) == false) {
return false;
}
}
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy